实名认证工具类(身份证二要素+手机号,姓名)两种
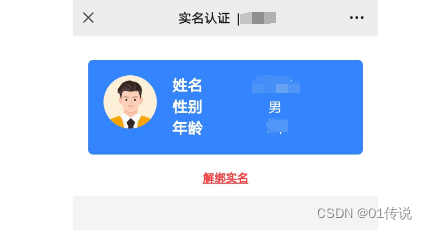
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.common.exception.TencentCloudSDKException;
import com.tencentcloudapi.common.profile.ClientProfile;
import com.tencentcloudapi.common.profile.HttpProfile;
import com.tencentcloudapi.faceid.v20180301.FaceidClient;
import com.tencentcloudapi.faceid.v20180301.models.CheckPhoneAndNameRequest;
import com.tencentcloudapi.faceid.v20180301.models.CheckPhoneAndNameResponse;
import com.tencentcloudapi.faceid.v20180301.models.IdCardVerificationRequest;
import com.tencentcloudapi.faceid.v20180301.models.IdCardVerificationResponse;
import io.micrometer.common.util.StringUtils;
import lombok.Builder;
import lombok.Data;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class IDCardUtil {
private final String secretId;
private final String secretKey;
private final String endpoint;
public IDCardUtil(String secretId, String secretKey, String endpoint) {
this.secretId = secretId;
this.secretKey = secretKey;
this.endpoint = endpoint;
}
public Result check(String idCard, String name) {
Credential cred = new Credential(secretId, secretKey);
HttpProfile httpProfile = new HttpProfile();
httpProfile.setEndpoint(endpoint);
ClientProfile clientProfile = new ClientProfile();
clientProfile.setHttpProfile(httpProfile);
FaceidClient client = new FaceidClient(cred, "", clientProfile);
IdCardVerificationRequest req = new IdCardVerificationRequest();
req.setIdCard(idCard);
req.setName(name);
Result.ResultBuilder builder = Result.builder();
try {
IdCardVerificationResponse resp = client.IdCardVerification(req);
String json = IdCardVerificationResponse.toJsonString(resp);
if (StringUtils.isNotBlank(json)) {
JSONObject jsonObject = JSON.parseObject(json);
return builder.result(jsonObject.getString("Result")).description(jsonObject.getString("Description")).build();
}
} catch (TencentCloudSDKException e) {
log.error("IDCardUtil.check Failed. ", e);
throw new WangyaoException("身份证校验异常!");
}
return builder.build();
}
public Result checkPhoneNumber(String phoneNum, String name) {
Credential cred = new Credential(secretId, secretKey);
HttpProfile httpProfile = new HttpProfile();
httpProfile.setEndpoint(endpoint);
ClientProfile clientProfile = new ClientProfile();
clientProfile.setHttpProfile(httpProfile);
FaceidClient client = new FaceidClient(cred, "", clientProfile);
CheckPhoneAndNameRequest req = new CheckPhoneAndNameRequest();
req.setMobile(phoneNum);
req.setName(name);
Result.ResultBuilder builder = Result.builder();
try {
CheckPhoneAndNameResponse resp = client.CheckPhoneAndName(req);
String json = CheckPhoneAndNameResponse.toJsonString(resp);
if (StringUtils.isNotBlank(json)) {
JSONObject jsonObject = JSON.parseObject(json);
return builder.result(jsonObject.getString("Result")).description(jsonObject.getString("Description")).build();
}
} catch (TencentCloudSDKException e) {
log.error("IDCardUtil.check Failed. ", e);
throw new WangyaoException("身份证校验异常!");
}
return builder.build();
}
@Builder
@Data
public static class Result {
private String result;
private String description;
}
}