调度算法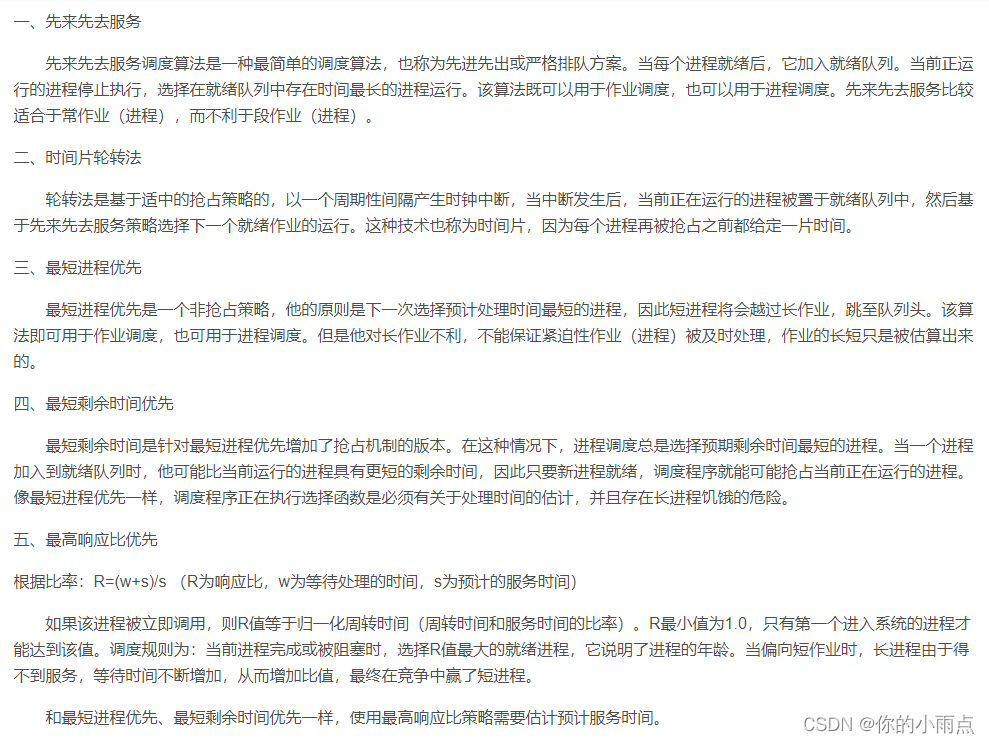
#include<iostream>
using namespace std;
#define MAX_PEOC_ID 65536
#define TIME_SLICE 2
//进程控制块
typedef struct PCB
{
char name[10];//进程名 id
char state; //进程状态 W/R
int ArriveTime; //进程到达时间
int StartTime; //进程开始时间
int FinshTime;//进程结束时间
int ServiceTime;//进程服务时间
float WholeTime; //周转时间
float Weight_WholeTime;//带权周转时间
double Average_WholeTime;//平均周转时间
double Average_Weight_WholeTime;//带权平均周转时间
int RunTime;//已经占用CPU时间
int NeedTime;//还要占用CPU时间
int Prio;//优先级
struct PCB *next;
}pcb;
double Sum_WholeTime=0,Sum_Weight_WholeTime=0;
int time=0;
int Proc_Num=0;
pcb *head = NULL;
pcb *tail=NULL;
void FCFS_RunProccess(pcb *proc)
{
proc->StartTime=time;
cout<<"时刻 "<<time<<" 开始执行当前作业 "<<proc->name<<endl;
time+=proc->ServiceTime;
proc->state='R';
proc->FinshTime=time;
proc->WholeTime=proc->FinshTime-proc->ArriveTime;
proc->Weight_WholeTime=proc->WholeTime/proc->ServiceTime;
Sum_WholeTime+=proc->WholeTime;
Sum_Weight_WholeTime+=proc->Weight_WholeTime;
proc->Average_WholeTime=Sum_WholeTime/Proc_Num;
proc->Average_Weight_WholeTime=Sum_Weight_WholeTime/Proc_Num;
printf(" 到达时间 开始时间 服务时间 完成时间 周转时间 带权周转时间\n");
printf("%6d %6d %6d %6d %8.1f %8.2f\n",
proc->ArriveTime,proc->StartTime,proc->ServiceTime,
proc->FinshTime,proc->WholeTime,proc->Weight_WholeTime);
//printf("共 %d 个进程\n",Proc_Num);
printf(" 平均周转时间 平均带权周转时间 \n");
printf(" %10.2f %10.2f\n",proc->Average_WholeTime,
proc->Average_Weight_WholeTime);
}
void FCFS()
{
pcb* cur_proc=head;
pcb*new_proc=NULL;
while(cur_proc)
{
if(cur_proc->state == 'W')
{
new_proc=cur_proc;
FCFS_RunProccess(new_proc);
}
cur_proc=cur_proc->next;
head=cur_proc;
free (new_proc);
new_proc=NULL;
}
}
void FCFS_CreateProccess()
{
cout<<"请输入进程的个数: ";
cin>>Proc_Num;
if(Proc_Num > MAX_PEOC_ID)
{
cout<<"sorry I can't give you PCB \n";
return;
}
for(int i=1;i<=Proc_Num;++i)
{
pcb*new_proc=NULL;
if((new_proc=(pcb*)malloc(sizeof(pcb))) == NULL)
{
perror("malloc");
return;
}
cout<<"请输入第"<<i<<"个进程名: ";
cin>>new_proc->name;
cout<<"请输入第"<<i<<"个进程到达时间: ";
cin>>new_proc->ArriveTime;
cout<<"请输入第"<<i<<"个进程服务时间:";
cin>>new_proc->ServiceTime;
new_proc->next=NULL;
if(head == NULL)
{
new_proc->next=head;
head=new_proc;
tail=head;
time=new_proc->ArriveTime;
}
else
{
if(head->ArriveTime>new_proc->ArriveTime)
{
new_proc->next=head;
head=new_proc;
}
else
{
pcb* cur_proc=head;
while(cur_proc->next != NULL && cur_proc->next->ArriveTime<new_proc->ArriveTime)
{
cur_proc=cur_proc->next;
}
if(cur_proc->next==NULL)
{
tail=new_proc;
}
new_proc->next=cur_proc->next;
cur_proc->next=new_proc;
}
if(new_proc->ArriveTime < time)
{
time=new_proc->ArriveTime;
}
}
new_proc->StartTime=0;
new_proc->FinshTime=0;
new_proc->WholeTime=0;
new_proc->Weight_WholeTime=0;
new_proc->Average_Weight_WholeTime=0;
new_proc->Average_WholeTime=0;
new_proc->state= 'W';
new_proc->RunTime=0;
new_proc->NeedTime=0;
}
}
void ShortJobsCreateProccess()
{
cout<<"请输入进程的个数: ";
cin>>Proc_Num;
if(Proc_Num > MAX_PEOC_ID)
{
cout<<"sorry I can't give you PCB \n";
return;
}
for(int i=1;i<=Proc_Num;++i)
{
pcb*new_proc=NULL;
if((new_proc=(pcb*)malloc(sizeof(pcb))) == NULL)
{
perror("malloc");
return;
}
cout<<"请输入第"<<i<<"个进程名: ";
cin>>new_proc->name;
cout<<"请输入第"<<i<<"个进程到达时间: ";
cin>>new_proc->ArriveTime;
cout<<"请输入第"<<i<<"个进程服务时间:";
cin>>new_proc->ServiceTime;
new_proc->next=NULL;
if(head == NULL)
{
new_proc->next=head;
head=new_proc;
tail=head;
time=new_proc->ArriveTime;
}
else
{
if(head->ServiceTime>new_proc->ServiceTime)
{
new_proc->next=head;
head=new_proc;
}
else
{
pcb* cur_proc=head;
while(cur_proc->next != NULL && cur_proc->next->ServiceTime<new_proc->ServiceTime)
{
cur_proc=cur_proc->next;
}
if(cur_proc->next==NULL)
{
tail=new_proc;
}
new_proc->next=cur_proc->next;
cur_proc->next=new_proc;
}
if(new_proc->ArriveTime < time)
{
time=new_proc->ArriveTime;
}
}
new_proc->StartTime=0;
new_proc->FinshTime=0;
new_proc->WholeTime=0;
new_proc->Weight_WholeTime=0;
new_proc->Average_Weight_WholeTime=0;
new_proc->Average_WholeTime=0;
new_proc->state= 'W';
new_proc->RunTime=0;
new_proc->NeedTime=0;
}
}
void PrioCreateProccess()
{
cout<<"请输入进程的个数: ";
cin>>Proc_Num;
if(Proc_Num > MAX_PEOC_ID)
{
cout<<"sorry I can't give you PCB \n";
return;
}
for(int i=1;i<=Proc_Num;++i)
{
pcb*new_proc=NULL;
if((new_proc=(pcb*)malloc(sizeof(pcb))) == NULL)
{
perror("malloc");
return;
}
cout<<"请输入第"<<i<<"个进程名: ";
cin>>new_proc->name;
cout<<"请输入第"<<i<<"个进程到达时间: ";
cin>>new_proc->ArriveTime;
cout<<"请输入第"<<i<<"个进程服务时间:";
cin>>new_proc->ServiceTime;
cout<<"请输入第"<<i<<"个进程优先级:(值越小优先级越高) ";
cin>>new_proc->Prio;
new_proc->next=NULL;
if(head == NULL)
{
new_proc->next=head;
head=new_proc;
tail=head;
time=new_proc->ArriveTime;
}
else
{
if(head->Prio>new_proc->Prio)
{
new_proc->next=head;
head=new_proc;
}
else
{
pcb* cur_proc=head;
while(cur_proc->next != NULL && cur_proc->next->Prio<new_proc->Prio)
{
cur_proc=cur_proc->next;
}
if(cur_proc->next==NULL)
{
tail=new_proc;
}
new_proc->next=cur_proc->next;
cur_proc->next=new_proc;
}
if(new_proc->ArriveTime < time)
{
time=new_proc->ArriveTime;
}
}
new_proc->StartTime=0;
new_proc->FinshTime=0;
new_proc->WholeTime=0;
new_proc->Weight_WholeTime=0;
new_proc->Average_Weight_WholeTime=0;
new_proc->Average_WholeTime=0;
new_proc->state= 'W';
new_proc->RunTime=0;
new_proc->NeedTime=0;
}
}
void RR_RunProccess(PCB *proc)
{
proc->StartTime=time;
cout<<"时刻 "<<time<<" 开始执行当前作业 "<<proc->name<<endl;
proc->RunTime+=TIME_SLICE;
time+=TIME_SLICE;
proc->NeedTime=proc->ServiceTime-proc->RunTime;
if(proc->NeedTime <=0)
{
cout<<"时刻 "<<time<<" 结束作业 "<<proc->name<<endl;
head=proc->next;
free(proc);
proc=NULL;
if(head==NULL)
{
tail=head;
}
}
else
{
cout<<"时刻 "<<time<<" 挂起作业 "<<proc->name<<endl;
cout<<"已经运行了"<<proc->RunTime<<"还需要执行"<<proc->NeedTime<<endl;
if(proc->next != NULL)
{
head=proc->next;
proc->next=NULL;
tail->next=proc;
tail=proc;
}
}
}
void RoundRobin()
{
pcb* cur_proc=head;
pcb* new_proc=NULL;
while(cur_proc)
{
if(cur_proc->state == 'W')
{
new_proc=cur_proc;
RR_RunProccess(new_proc);
}
cur_proc=head;
}
}
void Sort_By_NeedTime()
{
pcb* prev=head;
pcb*cur=head->next;
prev->next=NULL;
pcb*nxt=NULL;
if( cur && head->NeedTime <= cur->NeedTime)
{
cur->next=head;
head=cur;
}
else if(cur)
{
while(cur && prev->next != NULL && prev->next->NeedTime <= cur->NeedTime)
{
prev=prev->next;
}
nxt=cur->next;
cur->next=prev->next;
prev->next=cur;
cur=nxt;
}
}
void ShortNeed_Time_RunProccess(pcb *proc)
{
proc->StartTime=time;
cout<<"时刻 "<<time<<" 开始执行当前作业 "<<proc->name<<endl;
proc->RunTime+=TIME_SLICE;
time+=TIME_SLICE;
proc->NeedTime=proc->ServiceTime-proc->RunTime;
if(proc->NeedTime <=0)
{
cout<<"时刻 "<<time<<" 结束作业 "<<proc->name<<endl;
head=proc->next;
free(proc);
proc=NULL;
if(head==NULL)
{
tail=head;
}
}
else
{
cout<<"时刻 "<<time<<" 挂起作业 "<<proc->name<<endl;
cout<<"已经运行了"<<proc->RunTime<<"还需要执行"<<proc->NeedTime<<endl;
Sort_By_NeedTime();
}
}
void ShortNeed_Time()
{
pcb* cur_proc=head;
pcb* new_proc=NULL;
while(cur_proc)
{
if(cur_proc->state == 'W')
{
new_proc=cur_proc;
ShortNeed_Time_RunProccess(new_proc);
}
cur_proc=head;
}
}
void main()
{
int select=1;
while(select)
{
cout<<"******************************************\n";
cout<<"*****1.******* 先来先服务算法 ************\n";
cout<<"*****2.******** 时间片轮转 ************\n";
cout<<"*****3.******** 短作业优先 ************\n";
cout<<"*****4.******** 优先级调度 ************\n";
cout<<"*****5.******** 最短剩余时间 ************\n";
cout<<"*****0.**********退出*********************\n";
cout<<"请选择:> ";
cin>>select;
switch(select)
{
case 1:
FCFS_CreateProccess();
FCFS();
break;
case 2:
FCFS_CreateProccess();
RoundRobin();
break;
case 3:
ShortJobsCreateProccess();
FCFS();
break;
case 4:
PrioCreateProccess();
FCFS();
break;
case 5:
ShortJobsCreateProccess();
ShortNeed_Time();
break;
default:
break;
}
}
}
测试用例:
3
a 1 4
b 5 2
c 2 5
4
a 0 7
b 2 4
c 4 1
d 5 4
调度
置换算法
#include <iostream>
#include <algorithm>
#include <cstring>
#include <queue>
#include <unordered_set>
#include <ctime>
using namespace std;
typedef pair<int, int> PII;
const int N = 1010;//执行序列最大长度
const int M = 10;//内存块最大数量
int len;//执行序列长度
int seq[N];//执行序列
int sz;//内存块数量
PII flag[M];
void OPT()
{
unordered_set<int> st;//记录所有内存块中的页面号
int cnt = 0;//缺页中断次数
int idx = 0;
for (int i = 0; i < len; i++)
if (!st.count(seq[i]))//当前要访问的页面不在内存块中
{
if (st.size() < sz) st.insert(seq[i]), flag[idx++] = { i, seq[i] };
else
for (int j = i + 1; j < len; j++)
{
int t = 0;
if (st.count(seq[j]))
for (int k = 0; k < idx; k++)
if (flag[k].second == seq[j])
{
flag[k].first = j;
t++;
break;
}
if (t == 3 || j == len - 1)
{
sort(flag, flag + idx);
st.erase(flag[0].second);
st.insert(seq[i]);
flag[0] = { i, seq[i] };
}
}
cnt++;
}
cout << "最佳置换算法产生的缺页中断次数为 : " << cnt << "次\n";
}
void FIFO()
{
unordered_set<int> st;
queue<int> Q;
int cnt = 0;
for (int i = 0; i < len; i++)
if (!st.count(seq[i]))
{
if (st.size() < sz) st.insert(seq[i]), Q.push(seq[i]);
else
{
st.erase(Q.front());
st.insert(seq[i]);
Q.pop();
Q.push(seq[i]);
}
cnt++;
}
cout << "先进先出置换算法产生的缺页中断次数为 : " << cnt << "次\n";
}
void LRU()
{
unordered_set<int> st;
int cnt = 0;
int idx = 0;
for (int i = 0; i < len; i++)
if (!st.count(seq[i]))
{
if (st.size() < sz) st.insert(seq[i]), flag[idx++] = { i, seq[i] };
else
{
sort(flag, flag + idx);
st.erase(flag[0].second);
st.insert(seq[i]);
flag[0] = { i, seq[i] };
}
cnt++;
}
else
for (int j = 0; j < idx; j++)
if (flag[j].second == seq[i]) flag[j].first = i;
cout << "最近最久未使用置换算法产生的缺页中断次数为 : " << cnt << "次\n";
}
int main()
{
srand(time(0));
len = rand() % 981 + 20;//随机产生20~1000的序列长度
for (int i = 0; i < len; i++) seq[i] = rand() % 20;
/*len = 20;
seq[0] = 1, seq[1] = 2, seq[2] = 3, seq[3] = 4, seq[4] = 2
, seq[5] = 1, seq[6] = 5, seq[7] = 6, seq[8] = 2, seq[9] = 1, seq[10] = 2
, seq[11] = 3, seq[12] = 7, seq[13] = 6, seq[14] = 3, seq[15] = 2
, seq[16] = 1, seq[17] = 2, seq[18] = 3, seq[19] = 6;*/
cout << "页面执行序列 : ";
for (int i = 0; i < len; i++) cout << seq[i] << ' ';
for (int i = 0; i < 3; i++)
{
cout << endl << "请输入内存块数量 : ";
cin >> sz;
cout << endl;
OPT();
FIFO();
LRU();
}
return 0;
}