这是一个手机生成验证吗的小demo,
图一仿写写,图二老师代码
思路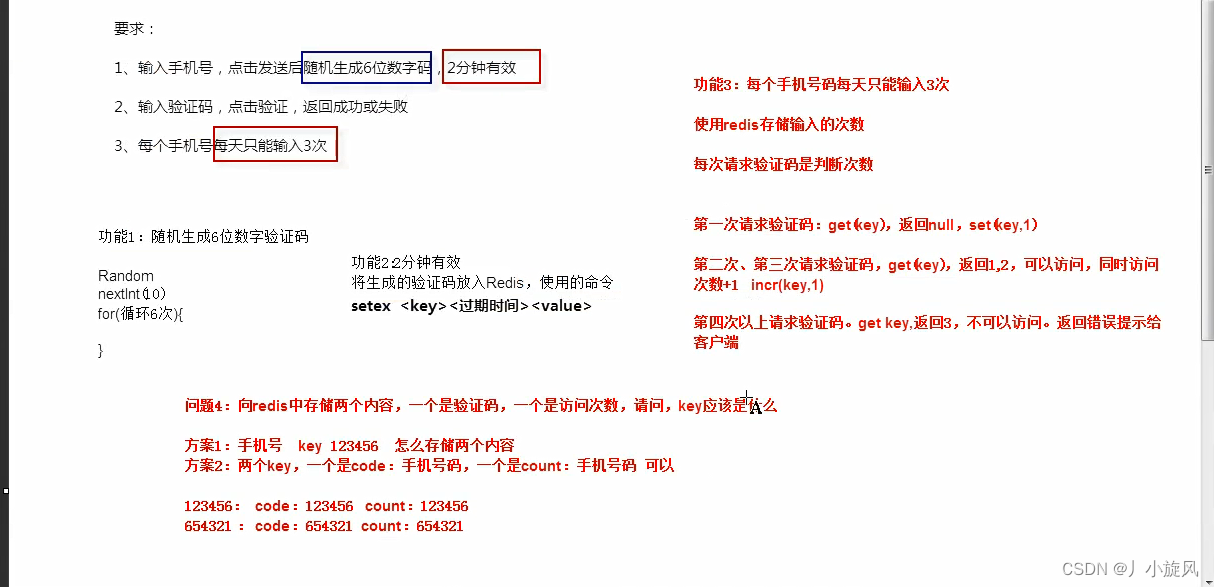
前提,在pom中导入redis相关依赖
<dependencies>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.2.0</version>
</dependency>
</dependencies>
在import com.sun.org.apache.bcel.internal.classfile.Code;
import redis.clients.jedis.Jedis;
import java.util.Random;
import java.util.Scanner;
/**
* @author jph
* @version 1.0
* @Description
* @date 2022/8/18 16:07
*/
public class Sjyzm {
public static void main(String[] args) {
//1.请输入手机验证码
Scanner scanner = new Scanner(System.in);
System.out.println("请输入手机号");
int phone = scanner.nextInt();
//2.输入1完成发送,稍后会接受到验证码,需要我们对验证码进行处理
System.out.println("输入1完成发送");
//这一步起一个暂停效果,如果我们不输入数组,程序就不往下走.
scanner.next();
//会出现两种情况,一种是次数不超,生成验证码,另一种是次数超了.直接返回一个-1
String yzmCode=getCode(phone);
//超了就结束了,不会往下进行了.
if("-1".equals(yzmCode)){
System.out.println("手机输入验证码已经超过3次");
return;
}
System.out.println("验证码是"+yzmCode);
//3. 请输入验证码
System.out.println("请输入验证码");
String inYzm = scanner.next();
System.out.println("请输入1完成发送");
scanner.nextInt();
boolean flag=verifyCode(inYzm,yzmCode);
if(flag)
{
System.out.println("验证码输入正确");
}else{
System.out.println("验证码输入错误");
}
//4.发送验证码,获取检验结果并输
}
private static boolean verifyCode(String inYzm, String yzmCode) {
boolean flag=false;
if(yzmCode.equals(inYzm)){
flag=true;
}
return flag;
}
private static String getCode(int phone) {
//生成验证码
String produceCode = produce();
//验证码时长不能超过2分钟 , 进一步联想连接服务器就要记得关闭linux的防护墙
Jedis jedis = new Jedis("192.168.245.131",6379);
//到这时redis中创建出一个来 produceCode"+phone ,key值来
jedis.setex("produceCode"+phone, 120, produceCode);
//判断本手机验证码是否超过3次
//count"+phone 在redis中是没有的,所以第一次访问必定为空
//在访问次数上下功夫.
String count=jedis.get("count"+phone);
if(count==null){
//相当于又开辟了一个key
jedis.set("count:"+phone, "1");
}else if(Integer.parseInt(count)<3){
//为什么不增?
jedis.incr("count:"+phone);
}else{
//这个是大于3的情况,咱们就把这个值符为-1
produceCode = "-1";
}
return produceCode;
}
public static String produce(){
Random random = new Random();
String s="";
for (int i = 0; i < 6; i++) {
int i1 = random.nextInt(10);
s+=i1;
}
return s;
}
}
代码分界线
import redis.clients.jedis.Jedis;
import java.util.Random;
import java.util.Scanner;
public class PhoneCode {
public static void main(String[] args) {
//1、输入手机号
Scanner input = new Scanner(System.in);
System.out.println("请输入手机号码:");
String phone = input.next();
//2.点击发送后随机生成6位数字码,2分钟有效
System.out.println("输入1完成发送:");
input.next();
String code = sendPhone(phone);
if("-1".equals(code)){
System.out.println("今天输入验证码已经超过3次,请明天再试");
return;
}
System.out.println("验证码是:"+code);
//3、输入验证码,点击验证,返回成功或失败
System.out.println("请输入验证码:");
String myCode = input.next();
System.out.println("输入1进行验证");
input.next();
boolean flag = verifyCode(phone,myCode);
//4.输出结果
if(flag){
System.out.println("成功");
}else{
System.out.println("失败");
}
}
/** * 进行验证 */
private static boolean verifyCode(String phone, String myCode) {
boolean flag = false;//默认失败
Jedis jedis = new Jedis("192.168.20.200",6379);
//1.获取保存在redis中的正确验证码
String codeKey = "code:"+phone;
String code = jedis.get(codeKey);
//2.
if(myCode.equals(code)){
flag = true;
}
jedis.close();
return flag;
}
/**
* 发送手机号码,返回验证码
*/
public static String sendPhone(String phone) {
Jedis jedis = new Jedis("192.168.20.200",6379);
//1.获取验证码
String code = getCode();
//2.将验证码保存在redis中,两分钟有效
String codeKey = "code:"+phone;
jedis.setex(codeKey,120,code);
//3.每个手机号每天只能输入3次
String countKey = "count:"+phone;
String count = jedis.get(countKey);
if(count==null){ //如果是第一次,设置次数为1次
jedis.setex(countKey,24*60*60,"1");
}else if(Integer.parseInt(count)<=2){ //如果是第二次或者第三次,设置次数+1
jedis.incr(countKey);
}else{//如果超过3次,给出次数超过的提示
//code ="今天输入验证码已经超过3次,请明天再试";
code="-1";
}
jedis.close();
//4.返回结果(验证码或者超过3次提示)
return code;
}
//生成6位数字验证码
public static String getCode() {
Random random = new Random();
String code = "";
for(int i=0;i<6;i++) {
int rand = random.nextInt(10);
code += rand;
}
return code;
}
}
其中总结小失误
Linux 防火墙问题,总是在访问Linux时忘记关闭防火墙,
查看防火墙systemctl status firewalld
关闭防火墙 systemctl stop firewalld