- Class类是一个泛型类,Class类的对象表示一个运行时类型。
- 加载到内存中的类,会缓存一定的时间,在此期间内可以通过不同的方式获取这个类的信息。
- 类的加载过程:
- 加载。将类的class文件读入内存,并由类加载器为之创建一个java.lang.Class对象。
- 链接。将类的二进制数据合并到jre中。
- 初始化。jvm负责类的初始化。
- 利用反射实例化类的对象通常是通过调用类的空参构造器实现的,因此需要保证类具有空参构造器,且是public的。
import java.lang.annotation.Annotation;
import java.lang.reflect.*;
public class ReflectionTest {
public static void main(String[] args) throws Exception {
Class personClass = Class.forName("Person");
Constructor constructor = personClass.getConstructor(String.class);
Person person1 = (Person) constructor.newInstance("Tom");
System.out.println(person1);
Field name = personClass.getField("name");
name.set(person1, "Tim");
System.out.println(person1);
Method setName = personClass.getMethod("setName", String.class);
setName.invoke(person1, "Tem");
System.out.println(person1);
System.out.println();
Constructor declaredConstructor = personClass.getDeclaredConstructor(String.class, int.class);
declaredConstructor.setAccessible(true);
Person person2 = (Person) declaredConstructor.newInstance("Jerry", 18);
System.out.println(person2);
Field age = personClass.getDeclaredField("age");
age.setAccessible(true);
age.set(person2, 24);
System.out.println(person2);
Method setAge = personClass.getDeclaredMethod("setAge", int.class);
setAge.setAccessible(true);
setAge.invoke(person2, 35);
System.out.println(person2);
System.out.println();
Method[] declaredMethods = personClass.getDeclaredMethods();
for (Method method : declaredMethods) {
Annotation[] annotations = method.getDeclaredAnnotations();
for (Annotation annotation : annotations) {
System.out.println("注解:" + annotation);
}
System.out.println("权限修饰符:" + Modifier.toString(method.getModifiers()));
System.out.println("返回值类型:" + method.getReturnType().getName());
System.out.println("方法名:" + method.getName());
Parameter[] parameters = method.getParameters();
for (Parameter parameter : parameters) {
System.out.println("参数:" + parameter);
}
Class[] exceptionTypes = method.getExceptionTypes();
for (Class exceptionType : exceptionTypes) {
System.out.println("异常:" + exceptionType.getName());
}
System.out.println();
}
}
}
@interface MyAnnotation {
String value() default "Annotation";
}
@MyAnnotation()
class Person {
@MyAnnotation(value = "publicField")
public String name;
@MyAnnotation(value = "privateField")
private int age;
@MyAnnotation(value = "publicConstructor")
public Person(String name) {
this.name = name;
}
@MyAnnotation(value = "privateConstructor")
private Person(String name, int age) {
this.name = name;
this.age = age;
}
@MyAnnotation(value = "publicMethod")
public void setName(String name) throws RuntimeException {
this.name = name;
}
@MyAnnotation(value = "privateMethod")
private void setAge(int age) throws IndexOutOfBoundsException, IllegalArgumentException {
this.age = age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
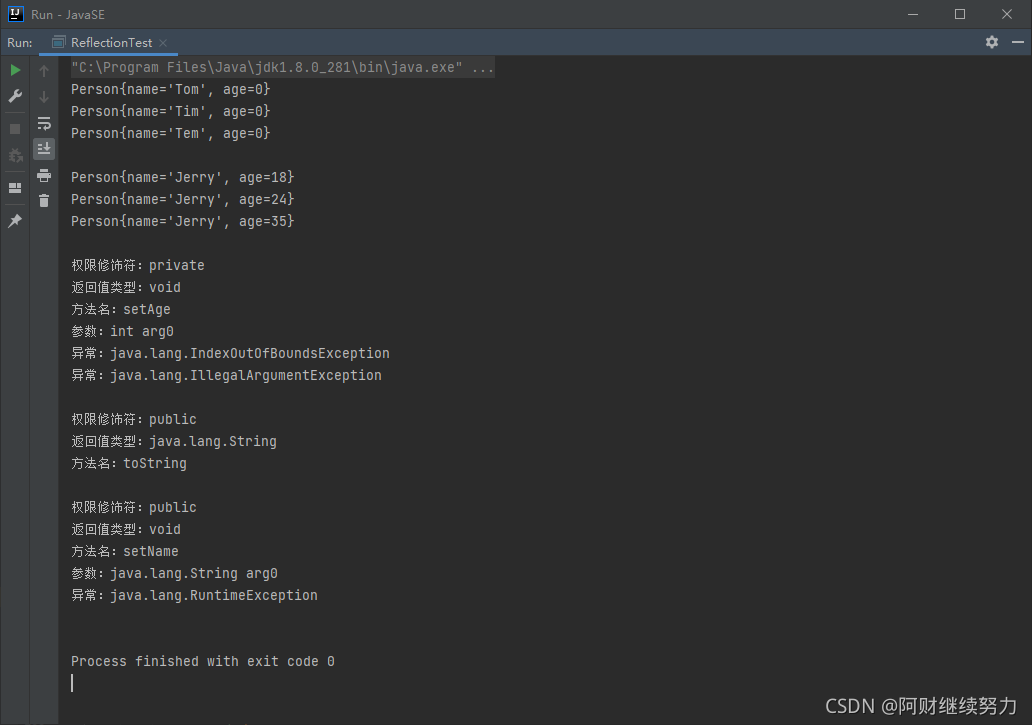