1. 题目
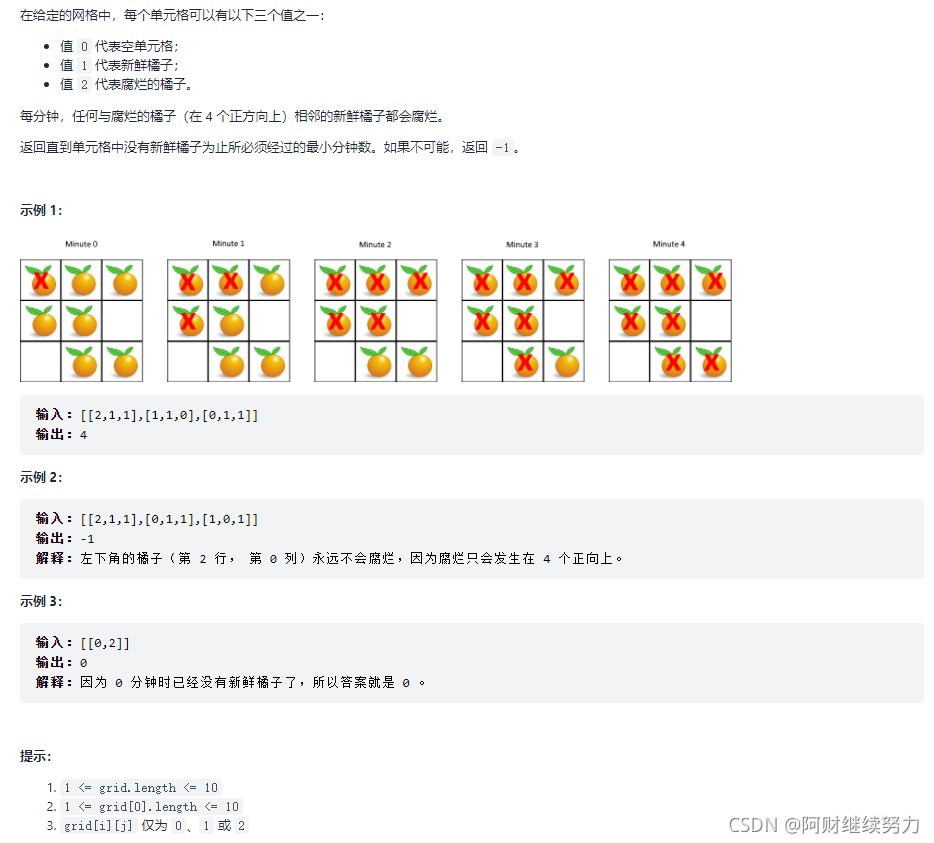
2. 思路
(1) 多源BFS
- 假设有一个腐烂的橘子存在于网格之外,当第0分钟时,这个橘子使得网格中的一些橘子腐烂,此时网格中所有橘子的状态正好满足题目条件,那么称网格之外的这个橘子为超级源点。
- 将第0分钟时腐烂的橘子全部加入队列,看作一个整体,然后进行广度优先搜索,即为多源广度优先搜索。
- 创建两个与原网格相同大小的二维数组,time用于记录每个新鲜橘子被腐烂的最短时间,初始时新鲜橘子设置为-1,腐烂橘子和空格子默认为0;flag用于标记每个网格是否被访问过,初始时腐烂橘子和空格子设置为true,新鲜橘子默认为false。
- 搜索完毕后,遍历time数组,若还存在-1,表示该新鲜橘子不会被腐烂,直接返回-1即可,否则返回最大值。
(2) 多源BFS优化
- 可以利用变量count记录当前网格中新鲜橘子的剩余数量,直接在原数组中修改,当发现新鲜橘子时,将其改为腐烂橘子,并将count减1。
- 按照搜索的轮数表示最短时间,搜索完毕后若count大于0,表示还存在新鲜橘子,直接返回-1即可,否则返回搜索的轮数。
3. 代码
import java.util.LinkedList;
import java.util.Queue;
public class Test {
public static void main(String[] args) {
}
}
class Solution {
public int orangesRotting(int[][] grid) {
int res = 0;
int row = grid.length;
int col = grid[0].length;
int[][] time = new int[row][col];
boolean[][] flag = new boolean[row][col];
Queue<int[]> queue = new LinkedList<>();
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (grid[i][j] == 2) {
queue.offer(new int[]{i, j});
flag[i][j] = true;
} else if (grid[i][j] == 0) {
flag[i][j] = true;
} else {
time[i][j] = -1;
}
}
}
while (!queue.isEmpty()) {
int[] index = queue.poll();
int x = index[0];
int y = index[1];
if (x > 0 && !flag[x - 1][y]) {
queue.offer(new int[]{x - 1, y});
time[x - 1][y] = time[x][y] + 1;
flag[x - 1][y] = true;
}
if (x < row - 1 && !flag[x + 1][y]) {
queue.offer(new int[]{x + 1, y});
time[x + 1][y] = time[x][y] + 1;
flag[x + 1][y] = true;
}
if (y > 0 && !flag[x][y - 1]) {
queue.offer(new int[]{x, y - 1});
time[x][y - 1] = time[x][y] + 1;
flag[x][y - 1] = true;
}
if (y < col - 1 && !flag[x][y + 1]) {
queue.offer(new int[]{x, y + 1});
time[x][y + 1] = time[x][y] + 1;
flag[x][y + 1] = true;
}
}
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (time[i][j] == -1) {
return -1;
} else {
res = Math.max(res, time[i][j]);
}
}
}
return res;
}
}
class Solution1 {
public int orangesRotting(int[][] grid) {
int res = 0;
int row = grid.length;
int col = grid[0].length;
int count = 0;
Queue<int[]> queue = new LinkedList<>();
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (grid[i][j] == 2) {
queue.offer(new int[]{i, j});
} else if (grid[i][j] == 1) {
count++;
}
}
}
while (count > 0 && !queue.isEmpty()) {
int size = queue.size();
for (int i = 0; i < size; i++) {
int[] index = queue.poll();
int x = index[0];
int y = index[1];
if (x > 0 && grid[x - 1][y] == 1) {
grid[x - 1][y] = 2;
count--;
queue.offer(new int[]{x - 1, y});
}
if (x < row - 1 && grid[x + 1][y] == 1) {
grid[x + 1][y] = 2;
count--;
queue.offer(new int[]{x + 1, y});
}
if (y > 0 && grid[x][y - 1] == 1) {
grid[x][y - 1] = 2;
count--;
queue.offer(new int[]{x, y - 1});
}
if (y < col - 1 && grid[x][y + 1] == 1) {
grid[x][y + 1] = 2;
count--;
queue.offer(new int[]{x, y + 1});
}
}
res++;
}
if (count > 0) {
return -1;
}
return res;
}
}