文章目录
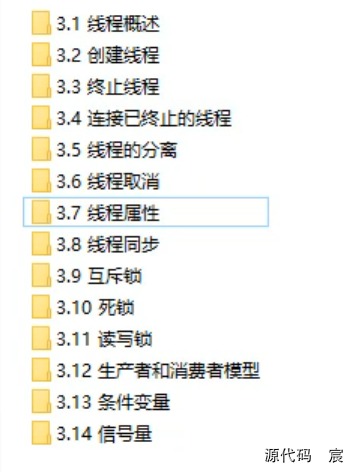
线程概述
线程和进程的区别
线程之间共享和非共享资源
NPTL
创建线程
线程操作
pthread_create函数
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
- 功能:创建一个子线程
- 参数:
- thread:传出参数, 线程创建成功后, 子线程的线程ID被写到该变量中
- attr : 设置线程的属性, 一般使用默认值, NULL
- start_routine : 函数指针, 这个函数是子线程需要处理的逻辑代码
- arg : 给第三个参数使用, 传参
- 返回值:
成功:0
失败:返回错误号。这个错误号和之前errno不太一样。
获取错误号的信息: char * strerror(int errnum);
man pthread_create
pthread_create() 函数在调用进程中启动一个新线程。新线程通过调用start_routine()开始执行; arg 作为 start_routine() 的唯一参数传递。
strerror函数
man strerror
创建线程小案例
pthread_create.c
/*
一般情况下, main函数所在的线程我们称之为主线程(main线程), 其余创建的线程称之为子线程。
程序中默认只有一个进程, fork()函数调用, 2个进程
程序中默认只有一个线程, pthread_create()函数调用, 2个线程
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
- 功能:创建一个子线程
- 参数:
- thread:传出参数, 线程创建成功后, 子线程的线程ID被写到该变量中
- attr : 设置线程的属性, 一般使用默认值, NULL
- start_routine : 函数指针, 这个函数是子线程需要处理的逻辑代码
- arg : 给第三个参数使用, 传参
- 返回值:
成功:0
失败:返回错误号。这个错误号和之前errno不太一样。
获取错误号的信息: char * strerror(int errnum);
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void * arg) {
printf("child thread...\n");
return NULL;
}
int main() {
pthread_t tid;
// 创建一个子线程
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char* errstr = strerror(ret);
printf("error: %s\n", errstr);
}
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
sleep(1);
return 0; // exit(0);
}
回调函数有参数
pthread_create.c
/*
一般情况下, main函数所在的线程我们称之为主线程(main线程), 其余创建的线程称之为子线程。
程序中默认只有一个进程, fork()函数调用, 2个进程
程序中默认只有一个线程, pthread_create()函数调用, 2个线程
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
- 功能:创建一个子线程
- 参数:
- thread:传出参数, 线程创建成功后, 子线程的线程ID被写到该变量中
- attr : 设置线程的属性, 一般使用默认值, NULL
- start_routine : 函数指针, 这个函数是子线程需要处理的逻辑代码
- arg : 给第三个参数使用, 传参
- 返回值:
成功:0
失败:返回错误号。这个错误号和之前errno不太一样。
获取错误号的信息: char * strerror(int errnum);
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void * arg) {
printf("child thread...\n");
printf("arg value: %d\n", *(int *)arg);
return NULL;
}
int main() {
pthread_t tid;
int num = 10;
// 创建一个子线程
int ret = pthread_create(&tid, NULL, callback, (void *)&num);
if(ret != 0) {
char* errstr = strerror(ret);
printf("error: %s\n", errstr);
}
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
sleep(1);
return 0; // exit(0);
}
终止线程
pthread_t就是长整型
pthread_self函数
pthread_exit函数
man pthread_exit
终止线程小案例
pthread_exit.c
/*
#include <pthread.h>
void pthread_exit(void *retval);
功能:终止一个线程, 在哪个线程中调用, 就表示终止哪个线程
参数:
retval:需要传递一个指针, 作为一个返回值, 可以在pthread_join()中获取到
没有返回值
pthread_t pthread_self(void);
功能:获取当前的线程的线程ID
This function always succeeds, returning the calling thread's ID.
int pthread_equal(pthread_t t1, pthread_t t2);
功能:比较两个线程ID是否相等
不同的操作系统, pthread_t类型的实现不一样, 有的是无符号的长整型, 有的
是使用结构体去实现的。
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
void * callback(void *arg) {
printf("child thread is : %ld\n", pthread_self());
return NULL; // pthread_exit(NULL);
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 主线程
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
printf("tid: %ld, main thread is : %ld\n", tid, pthread_self());
// 让主线程退出,当主线程退出时,不会影响其他正常运行的线程。
pthread_exit(NULL);
printf("main thread exit\n");
return 0; // exit(0);
}
当main线程执行退出以后,当这个main所属的进程的所有线程都终止了,这个进程就自动退出了
主线程的LWP与PID相同,子线程的LWP与PID不相同
pthread_exit.c
/*
#include <pthread.h>
void pthread_exit(void *retval);
功能:终止一个线程, 在哪个线程中调用, 就表示终止哪个线程
参数:
retval:需要传递一个指针, 作为一个返回值, 可以在pthread_join()中获取到
没有返回值
pthread_t pthread_self(void);
功能:获取当前的线程的线程ID
This function always succeeds, returning the calling thread's ID.
int pthread_equal(pthread_t t1, pthread_t t2);
功能:比较两个线程ID是否相等
不同的操作系统, pthread_t类型的实现不一样, 有的是无符号的长整型, 有的
是使用结构体去实现的。
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
void * callback(void *arg) {
int num = 1;
while(1) {
if(num % 5000000) {
printf("child thread is : %ld\n", pthread_self());
}
num++;
}
return NULL; // pthread_exit(NULL);
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 主线程
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
printf("tid: %ld, main thread is : %ld\n", tid, pthread_self());
// 让主线程退出,当主线程退出时,不会影响其他正常运行的线程。
pthread_exit(NULL);
printf("main thread exit\n");
return 0; // exit(0);
}
连接已终止的线程
pthread_join函数
int pthread_join(pthread_t thread, void **retval);
- 功能: 和一个已经终止的线程进行连接
回收子线程的资源
这个函数是阻塞函数,调用一次只能回收一个子线程
一般在主线程中使用
- 参数:
- thread: 需要回收的子线程的ID
- retval: 接收子线程退出时的返回值
- 返回值:
0 : 成功
非0 : 失败,返回的错误号
man pthread_join
回收子线程资源的小案例
pthread_join.c
/*
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
- 功能: 和一个已经终止的线程进行连接
回收子线程的资源
这个函数是阻塞函数,调用一次只能回收一个子线程
一般在主线程中使用
- 参数:
- thread: 需要回收的子线程的ID
- retval: 接收子线程退出时的返回值
- 返回值:
0 : 成功
非0 : 失败,返回的错误号
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void *arg) {
printf("child thread is : %ld\n", pthread_self());
sleep(3);
return NULL; // pthread_exit(NULL);
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 主线程
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
printf("tid: %ld, main thread is : %ld\n", tid, pthread_self());
// 主线程调用pthread_join()回收子线程的资源
ret = pthread_join(tid, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
printf("Recycling sub-thread resources successfully\n");
// 让主线程退出,当主线程退出时,不会影响其他正常运行的线程。
pthread_exit(NULL);
return 0; // exit(0);
}
获取接收子线程退出时的返回值的案例
pthread_join.c
/*
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
- 功能: 和一个已经终止的线程进行连接
回收子线程的资源
这个函数是阻塞函数,调用一次只能回收一个子线程
一般在主线程中使用
- 参数:
- thread: 需要回收的子线程的ID
- retval: 接收子线程退出时的返回值
- 返回值:
0 : 成功
非0 : 失败,返回的错误号
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
int value = 10;
void * callback(void *arg) {
printf("child thread is : %ld\n", pthread_self());
// sleep(3);
// return NULL; // pthread_exit(NULL);
// int value = 10; 局部变量
pthread_exit((void *)&value);
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 主线程
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
printf("tid: %ld, main thread is : %ld\n", tid, pthread_self());
// 主线程调用pthread_join()回收子线程的资源
int * thread_retval;
ret = pthread_join(tid, (void **)&thread_retval);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error: %s\n", errstr);
}
printf("exit data: %d\n", *thread_retval);
printf("Recycling sub-thread resources successfully\n");
// 让主线程退出,当主线程退出时,不会影响其他正常运行的线程。
pthread_exit(NULL);
return 0; // exit(0);
}
函数callback返回的是一个指针,要想接收这个返回值需要一个指针类型。
所以定义了 int *thread_retval去接收返回的指针。
但是如果直接将 thread_retval传入函数pthread_join,它就是一个局部参数,当结束函数pthread_join回到主线程,thread_retval的值是不会改变的。这样起不到用thread_retval接收返回值的作用。
要改变值,就要传递地址,所以得传&thread_retval,因为thread_retval是一个指针,而&是取地址,所以就成了**的参数类型。
线程的分离
pthread_detach函数
int pthread_detach(pthread_t thread);
- 功能:分离一个线程。被分离的线程在终止的时候, 会自动释放资源返回给系统
1.不能多次分离, 会产生不可预料的行为。
2.不能去连接一个已经分离的线程, 会报错。
- 参数:需要分离的线程的ID
- 返回值:
成功:0
失败:返回错误号
man pthread_detach
线程分离的小案例
pthread_detach.c
/*
#include <pthread.h>
int pthread_detach(pthread_t thread);
- 功能:分离一个线程。被分离的线程在终止的时候, 会自动释放资源返回给系统
1.不能多次分离, 会产生不可预料的行为。
2.不能去连接一个已经分离的线程, 会报错。
- 参数:需要分离的线程的ID
- 返回值:
成功:0
失败:返回错误号
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void *arg) {
printf("child thread id : %ld\n", pthread_self());
return NULL;
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char *errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 输出主线程和子线程的id
printf("tid: %ld, main thread id : %ld\n", tid, pthread_self());
// 设置子线程分离,子线程分离后,子线程结束时对应的资源就不需要主线程释放
pthread_detach(tid);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error2 : %s\n", errstr);
}
// 设置分离后,对分离的子线程进行连接 pthread_join()
pthread_exit(NULL);
return 0;
}
验证线程是否分离
pthread_detach.c
/*
#include <pthread.h>
int pthread_detach(pthread_t thread);
- 功能:分离一个线程。被分离的线程在终止的时候, 会自动释放资源返回给系统
1.不能多次分离, 会产生不可预料的行为。
2.不能去连接一个已经分离的线程, 会报错。
- 参数:需要分离的线程的ID
- 返回值:
成功:0
失败:返回错误号
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void *arg) {
printf("child thread id : %ld\n", pthread_self());
return NULL;
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char *errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 输出主线程和子线程的id
printf("tid: %ld, main thread id : %ld\n", tid, pthread_self());
// 设置子线程分离,子线程分离后,子线程结束时对应的资源就不需要主线程释放
pthread_detach(tid);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error2 : %s\n", errstr);
}
// 设置分离后,对分离的子线程进行连接 pthread_join()
ret = pthread_join(tid, NULL);
if(ret != 0) {
char * errstr = strerror(ret);
printf("error3 : %s\n", errstr);
}
pthread_exit(NULL);
return 0;
}
main线程创建了子线程,线程的默认属性是非分离状态,main线程等待子线程结束。只有当pthread_join()函数返回时,创建的线程才算终止,才能释放自己占用的系统资源。分离状态指线程不被其他线程所等待,自己运行完后,线程也就结束了,马上释放系统资源。
我的理解是如果线程先结束,再运行到pthread_detach()函数,该函数也会起作用,因为线程运行完了,并不代表就释放资源了。如果没有调用pthread_join()函数,那就需要变成分离状态释放资源。
线程分离不代表结束,pthread_detach函数是非阻塞的,无论先调用还是后调用该函数,子进程一样能够继续正常运行,只是能够让子线程在结束时无需pthread_join回收资源。
线程取消
pthread_cancel函数
man pthread_cancel
pthread_cancel()函数向线程thread发送取消请求。 目标线程是否以及何时对取消请求作出反应取决于该线程控制下的两个属性:其可取消性状态和类型。
线程的可取消状态由 pthread_setcancelstate(3) 确定,可以启用(新线程的默认设置)或禁用。 如果线程禁用了取消,则取消请求将保持排队状态,直到该线程启用取消。 如果线程启用了取消,则其可取消类型决定何时发生取消。
线程的取消类型由 pthread_setcanceltype(3) 确定,可以是异步的或延迟的(新线程的默认值)。 异步可取消性意味着线程可以随时取消(通常是立即取消,但系统不保证这一点)。 延迟可取消性意味着取消将被延迟,直到线程下一次调用作为取消点的函数为止。 pthreads(7) 中提供了作为或可能作为取消点的函数列表。
pthreads取消点
man pthreads
POSIX.1 规定某些函数必须是取消点,而某些其他函数可以是取消点。 如果线程是可取消的,其可取消性类型是延迟的,并且线程的取消请求处于待处理状态,则当线程调用作为取消点的函数时,该线程将被取消。
线程取消小案例
pthread_cancel.c
/*
#include <pthread.h>
int pthread_cancel(pthread_t thread);
- 功能:取消线程(让线程终止)
取消某个线程,可以终止某个线程的运行,
但是并不是立马终止,而是当子线程执行到一个取消点,线程才会终止。
取消点:系统规定好的一些系统调用,我们可以粗略的理解为从用户区到内核区的切换,这个位置称之为取消点。
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void *arg) {
printf("child thread id : %ld\n", pthread_self());
for(int i = 0; i < 5; i++) {
printf("child : %d\n", i);
}
return NULL;
}
int main() {
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, NULL, callback, NULL);
if(ret != 0) {
char *errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 取消线程
pthread_cancel(tid);
for(int i = 0; i < 5; i++) {
printf("%d\n", i);
}
// 输出主线程和子线程的id
printf("tid: %ld, main thread id : %ld\n", tid, pthread_self());
pthread_exit(NULL);
return 0;
}
线程属性
pthread_attr_getdetachstate函数
man pthread_attr_getdetachstate
PTHREAD_CREATE_DETACHED:使用 attr 创建的线程将以分离状态创建。
PTHREAD_CREATE_JOINABLE:使用 attr 创建的线程将被创建为可连接状态。
pthread_attr_getdetachstate函数
man pthread_attr_getdetachstate
pthread_attr.c
/*
int pthread_attr_init(pthread_attr_t *attr);
- 初始化线程属性变量
int pthread_attr_destroy(pthread_attr_t *attr);
- 释放线程属性的资源
int pthread_attr_getdetachstate(const pthread_attr_t *attr, int *detachstate);
- 获取线程分离的状态属性
On success, these functions return 0; on error, they return a nonzero error number.
int pthread_attr_setdetachstate(pthread_attr_t *attr, int detachstate);
- 设置线程分离的状态属性
*/
#include <stdio.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
void * callback(void *arg) {
printf("child thread id : %ld\n", pthread_self());
return NULL;
}
int main() {
// 创建一个线程属性变量
pthread_attr_t attr;
// 初始化属性变量
pthread_attr_init(&attr);
// 设置属性
pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_DETACHED);
// 创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid, &attr, callback, NULL);
if(ret != 0) {
char *errstr = strerror(ret);
printf("error: %s\n", errstr);
}
// 获取线程的栈的大小
size_t size;
pthread_attr_getstacksize(&attr, &size);
printf("thread stack size : %ld\n", size);
// 输出主线程和子线程的id
printf("tid: %ld, main thread id : %ld\n", tid, pthread_self());
// 释放线程属性资源
pthread_attr_destroy(&attr);
pthread_exit(NULL);
return 0;
}
一般系统默认为每个线程分配8MB的栈空间
即 8 MB = 8388608 B
线程同步
usleep函数
man usleep
卖门票小案例
selltickets.c
/*
使用多线程实现卖票的案例。
有3个窗口,一共是100张票。
*/
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 全局变量,所有的线程都共享这一份资源
int tickets = 100;
void * sellticket(void *arg) {
// 卖票
while(tickets > 0) {
usleep(6000);
printf("%ld 正在卖第 %d 张门票\n", pthread_self(), tickets);
tickets--;
}
return NULL;
}
int main() {
// 创建3个子线程
pthread_t tid1, tid2, tid3;
pthread_create(&tid1, NULL, sellticket, NULL);
pthread_create(&tid2, NULL, sellticket, NULL);
pthread_create(&tid3, NULL, sellticket, NULL);
// 回收子线程的资源,阻塞
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_join(tid3, NULL);
// 设置线程分离
// pthread_detach(tid1);
// pthread_detach(tid2);
// pthread_detach(tid3);
// 退出主线程
pthread_exit(NULL);
return 0;
}
互斥锁
互斥量
互斥量相关操作函数
pthread_mutex_init函数
int pthread_mutex_init(pthread_mutex_t *restrict mutex, const pthread_mutexattr_t *restrict attr);
- 初始化互斥量
- 参数 :
- mutex : 需要初始化的互斥量变量
- attr : 互斥量相关的属性, NULL
- restrict : C语言的修饰符, 被修饰的指针, 不能由另外的一个指针进行操作。
pthread_mutex_t *restrict mutex = xxx;
pthread_mutex_t * mutex1 = mutex; 会报错
函数 pthread_mutex_init 用于初始化互斥锁。这是 POSIX 线程(pthread)库中的一个基础功能,广泛应用于操作系统级别的多线程编程中以保证数据访问的同步和避免竞态条件。这里是该函数的参数和返回值的详细解释:
函数原型
int pthread_mutex_init(pthread_mutex_t *mutex, const pthread_mutexattr_t *attr);
pthread_mutex_destroy函数
man pthread_mutex_destroy
pthread_mutex_lock函数
man pthread_mutex_lock
pthread_mutex_unlock函数
man pthread_mutex_unlock
卖门票升级版小案例
mutex.c
/*
互斥量的类型 pthread_mutex_t
int pthread_mutex_init(pthread_mutex_t *restrict mutex, const pthread_mutexattr_t *restrict attr);
- 初始化互斥量
- 参数 :
- mutex : 需要初始化的互斥量变量
- attr : 互斥量相关的属性, NULL
- restrict : C语言的修饰符, 被修饰的指针, 不能由另外的一个指针进行操作。
pthread_mutex_t *restrict mutex = xxx;
pthread_mutex_t * mutex1 = mutex; 会报错
int pthread_mutex_destroy(pthread_mutex_t *mutex);
- 释放互斥量的资源
int pthread_mutex_lock(pthread_mutex_t *mutex);
- 加锁, 阻塞的, 如果有一个线程加锁了, 那么其他的线程只能阻塞等待
int pthread_mutex_trylock(pthread_mutex_t *mutex);
- 尝试加锁, 如果加锁失败, 不会阻塞, 会直接返回。
int pthread_mutex_unlock(pthread_mutex_t *mutex);
- 解锁
*/
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 全局变量,所有的线程都共享这一份资源
int tickets = 100;
// 创建一个互斥量
pthread_mutex_t mutex;
void * sellticket(void *arg) {
// 卖票
while(1) {
usleep(60000);
// 加锁
pthread_mutex_lock(&mutex);
if(tickets > 0) {
printf("%ld 正在卖第 %d 张门票\n", pthread_self(), tickets);
tickets--;
} else {
// 解锁
pthread_mutex_unlock(&mutex);
break;
}
// 解锁
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
// 初始化互斥量
pthread_mutex_init(&mutex, NULL);
// 创建3个子线程
pthread_t tid1, tid2, tid3;
pthread_create(&tid1, NULL, sellticket, NULL);
pthread_create(&tid2, NULL, sellticket, NULL);
pthread_create(&tid3, NULL, sellticket, NULL);
// 回收子线程的资源,阻塞
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_join(tid3, NULL);
// 释放互斥量资源
pthread_mutex_destroy(&mutex);
// 退出主线程
pthread_exit(NULL);
return 0;
}
死锁
忘记释放锁
deadlock.c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 全局变量,所有的线程都共享这一份资源。
int tickets = 100;
// 创建一个互斥量
pthread_mutex_t mutex;
void * sellticket(void * arg) {
// 卖票
while(1) {
// 加锁
// pthread_mutex_lock(&mutex);
pthread_mutex_lock(&mutex);
if(tickets > 0) {
usleep(6000);
printf("%ld 正在卖第 %d 张门票\n", pthread_self(), tickets);
tickets--;
}else {
// 解锁
pthread_mutex_unlock(&mutex);
break;
}
// 解锁
// pthread_mutex_unlock(&mutex);
// pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
// 初始化互斥量
pthread_mutex_init(&mutex, NULL);
// 创建3个子线程
pthread_t tid1, tid2, tid3;
pthread_create(&tid1, NULL, sellticket, NULL);
pthread_create(&tid2, NULL, sellticket, NULL);
pthread_create(&tid3, NULL, sellticket, NULL);
// 回收子线程的资源,阻塞
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_join(tid3, NULL);
pthread_exit(NULL); // 退出主线程
// 释放互斥量资源
pthread_mutex_destroy(&mutex);
return 0;
}
重复加锁
deadlock.c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 全局变量,所有的线程都共享这一份资源。
int tickets = 100;
// 创建一个互斥量
pthread_mutex_t mutex;
void * sellticket(void * arg) {
// 卖票
while(1) {
// 加锁
pthread_mutex_lock(&mutex);
pthread_mutex_lock(&mutex);
if(tickets > 0) {
usleep(6000);
printf("%ld 正在卖第 %d 张门票\n", pthread_self(), tickets);
tickets--;
}else {
// 解锁
pthread_mutex_unlock(&mutex);
break;
}
// 解锁
pthread_mutex_unlock(&mutex);
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
// 初始化互斥量
pthread_mutex_init(&mutex, NULL);
// 创建3个子线程
pthread_t tid1, tid2, tid3;
pthread_create(&tid1, NULL, sellticket, NULL);
pthread_create(&tid2, NULL, sellticket, NULL);
pthread_create(&tid3, NULL, sellticket, NULL);
// 回收子线程的资源,阻塞
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_join(tid3, NULL);
pthread_exit(NULL); // 退出主线程
// 释放互斥量资源
pthread_mutex_destroy(&mutex);
return 0;
}
多线程多锁,抢占锁资源
deadlock1.c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 创建2个互斥量
pthread_mutex_t mutex1, mutex2;
void * workA(void * arg) {
pthread_mutex_lock(&mutex1);
sleep(1);
pthread_mutex_lock(&mutex2);
printf("workA...\n");
pthread_mutex_unlock(&mutex2);
pthread_mutex_unlock(&mutex1);
return NULL;
}
void * workB(void * arg) {
pthread_mutex_lock(&mutex2);
sleep(1);
pthread_mutex_lock(&mutex1);
printf("workB...\n");
pthread_mutex_unlock(&mutex1);
pthread_mutex_unlock(&mutex2);
return NULL;
}
int main() {
// 初始化互斥量
pthread_mutex_init(&mutex1, NULL);
pthread_mutex_init(&mutex2, NULL);
// 创建2个子线程
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, workA, NULL);
pthread_create(&tid2, NULL, workB, NULL);
// 回收子线程资源
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
// 释放互斥量资源
pthread_mutex_destroy(&mutex1);
pthread_mutex_destroy(&mutex2);
return 0;
}
读写锁
读写锁小案例
rwlock.c
/*
读写锁的类型 pthread_rwlock_t
int pthread_rwlock_init(pthread_rwlock_t *restrict rwlock, const pthread_rwlockattr_t *restrict attr);
int pthread_rwlock_destroy(pthread_rwlock_t *rwlock);
int pthread_rwlock_rdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_tryrdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_wrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_trywrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_unlock(pthread_rwlock_t *rwlock);
案例:8个线程操作同一个全局变量。
3个线程不定时写这个全局变量, 5个线程不定时的读这个全局变量
*/
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 创建一个共享数据
int num = 1;
// pthread_mutex_t mutex;
pthread_rwlock_t rwlock;
void * writeNum(void *arg) {
while(1) {
pthread_rwlock_wrlock(&rwlock);
num++;
printf("++write, tid: %ld, num: %d\n", pthread_self(), num);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
return NULL;
}
void * readNum(void *arg) {
while(1) {
pthread_rwlock_rdlock(&rwlock);
printf("===read, tid: %ld, num: %d\n", pthread_self(), num);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
return NULL;
}
int main() {
pthread_rwlock_init(&rwlock, NULL);
// 创建3个写线程,5个读线程
pthread_t wtids[3], rtids[5];
for(int i = 0; i < 3; i++) {
pthread_create(&wtids[i], NULL, writeNum, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_create(&rtids[i], NULL, readNum, NULL);
}
// 设置线程分离
for(int i = 0; i < 3; i++) {
pthread_detach(wtids[i]);
}
for(int i = 0; i < 5; i++) {
pthread_detach(rtids[i]);
}
pthread_rwlock_destroy(&rwlock);
pthread_exit(NULL);
return 0;
}
按照老师的代码,如果destory在先,因为上面的detach运行后子线程可能没有运行完,所以可能会在子线程运行过程中destory互斥量,这样会出错,如果destory在后,那么主线程exit后也不会去destory互斥量,所以我建议上面回收子线程使用join,因为join是阻塞的,会等所有的子进程资源回收完了再继续,然后再destory互斥量,最后exit主线程。
rwlock.c
/*
读写锁的类型 pthread_rwlock_t
int pthread_rwlock_init(pthread_rwlock_t *restrict rwlock, const pthread_rwlockattr_t *restrict attr);
int pthread_rwlock_destroy(pthread_rwlock_t *rwlock);
int pthread_rwlock_rdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_tryrdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_wrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_trywrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_unlock(pthread_rwlock_t *rwlock);
案例:8个线程操作同一个全局变量。
3个线程不定时写这个全局变量, 5个线程不定时的读这个全局变量
*/
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 创建一个共享数据
int num = 1;
// pthread_mutex_t mutex;
pthread_rwlock_t rwlock;
void * writeNum(void *arg) {
while(1) {
pthread_rwlock_wrlock(&rwlock);
num++;
printf("++write, tid: %ld, num: %d\n", pthread_self(), num);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
return NULL;
}
void * readNum(void *arg) {
while(1) {
pthread_rwlock_rdlock(&rwlock);
printf("===read, tid: %ld, num: %d\n", pthread_self(), num);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
return NULL;
}
int main() {
pthread_rwlock_init(&rwlock, NULL);
// 创建3个写线程,5个读线程
pthread_t wtids[3], rtids[5];
for(int i = 0; i < 3; i++) {
pthread_create(&wtids[i], NULL, writeNum, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_create(&rtids[i], NULL, readNum, NULL);
}
// 设置线程分离
for(int i = 0; i < 3; i++) {
pthread_join(wtids[i], NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(rtids[i], NULL);
}
pthread_rwlock_destroy(&rwlock);
pthread_exit(NULL);
return 0;
}
读写锁相关操作函数
比较【读写锁】和【互斥锁】在读操作上的时间效率
test.c
/*
此程序是为了比较
互斥锁和读写锁在 高并发读操作上的效率差异
创建两个进程,父进程中使用互斥锁读取,子进程使用读写锁读取
每个进程中,创建10个读线程,对全局变量区的某个变量进行读取
每个线程读取 COUNT 次,总计完成10*COUNT次读取
利用 gettimeofday 函数,对整个读取过程精确到微秒的计时
*/
#include <pthread.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <time.h>
#include <sys/time.h>
#include <sys/wait.h>
#include <stdlib.h>
//创建互斥锁
pthread_mutex_t mutex;
//创建读写锁
pthread_rwlock_t rwlock;
//全局变量, 父子进程、线程间都是相同的
int data = 123456;
//读取次数
long COUNT = 10000000;
//互斥读函数
void *mFunc(void *arg)
{
int read = 0;
for (long i = 0; i < COUNT; ++i)
{
//加锁
pthread_mutex_lock(&mutex);
read = data;
//解锁
pthread_mutex_unlock(&mutex);
}
//结束线程
pthread_exit(NULL);
}
//读写锁读
void *rwFunc(void *arg)
{
int read = 0;
for (long i = 0; i < COUNT; ++i)
{
//加锁
pthread_rwlock_rdlock(&rwlock);
read = data;
//解锁
pthread_rwlock_unlock(&rwlock);
}
//结束线程
pthread_exit(NULL);
}
int main()
{
//创建两个进程,父进程中使用互斥锁读取,子进程使用读写锁读取
int pid = fork();
// 父进程
if (pid > 0)
{
//parent process
//初始化互斥锁
pthread_mutex_init(&mutex, NULL);
//创建十个线程,并开始计时
pthread_t mtids[10];
struct timeval start;
gettimeofday(&start, NULL);
for (int i = 0; i < 10; ++i)
{
pthread_create(&mtids[i], NULL, mFunc, NULL);
}
//在主线程中,调用join函数,回收线程,线程回收完成后,结束计时
for (int i = 0; i < 10; ++i)
{
pthread_join(mtids[i], NULL);
}
struct timeval end;
gettimeofday(&end, NULL);
long timediff = (end.tv_sec - start.tv_sec) * 1000000 + end.tv_usec - start.tv_usec;
printf("互斥锁 读全部线程执行完毕,总耗时: %ld us\n", timediff);
//回收子进程
wait(NULL);
}
else if (pid == 0)
{
//子进程
//初始化读写锁
pthread_rwlock_init(&rwlock, NULL);
//创建
//创建十个线程,并开始计时
pthread_t rwtids[10];
struct timeval start;
gettimeofday(&start, NULL);
for (int i = 0; i < 10; ++i)
{
pthread_create(&rwtids[i], NULL, rwFunc, NULL);
}
//在主线程中,调用join函数,回收线程,线程回收完成后,结束计时
for (int i = 0; i < 10; ++i)
{
pthread_join(rwtids[i], NULL);
}
struct timeval end;
gettimeofday(&end, NULL);
long timediff = (end.tv_sec - start.tv_sec) * 1000000 + end.tv_usec - start.tv_usec;
printf("读写锁 读全部线程执行完毕,总耗时: %ld us\n", timediff);
//结束进程
exit(0);
}
return 0;
}
在服务器或桌面 Linux 上安装手册页(Installing man pages on server or desktop Linux)
sudo apt update
sudo apt install man-db manpages-posix
安装软件开发的手册页(Install man pages for software development)
sudo apt install manpages-dev manpages-posix-dev
生产者和消费者模型
生产者消费者案例
prodcust.c
/*
生产者消费者模型(粗略的版本)
*/
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
typedef struct node
{
/* data */
int num;
struct node *next;
}Node;
// 头结点
struct node * head = NULL;
// 创建一个互斥量
pthread_mutex_t mutex;
void * producer(void *arg) {
srand((unsigned int)time(NULL));
// 不断的创建新的节点,添加到链表中
while(1) {
pthread_mutex_lock(&mutex);
Node * newNode = (Node*)malloc(sizeof(Node));
newNode->next = head;
head = newNode;
newNode->num = rand() % 1000;
printf("add node, num : %d, tid: %ld\n", newNode->num, pthread_self());
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
void * customer(void *arg) {
while(1) {
pthread_mutex_lock(&mutex);
// 保存头结点的指针
Node *tmp = head;
// 判断是否有数据
if(head != NULL) {
// 有数据
head = head->next;
printf("del node, num: %d, tid: %ld\n", tmp->num, pthread_self());
free(tmp);
pthread_mutex_unlock(&mutex);
usleep(100);
} else {
pthread_mutex_unlock(&mutex);
}
}
return NULL;
}
int main() {
// 创建5个生产者线程,和5个消费者线程
pthread_t ptids[5], ctids[5];
for(int i = 0; i < 5; i++) {
pthread_create(&ptids[i], NULL, producer, NULL);
pthread_create(&ctids[i], NULL, customer, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(ptids[i], NULL);
pthread_join(ctids[i], NULL);
}
// while(1) {
// sleep(10);
// }
pthread_exit(NULL);
return 0;
}
原子操作
条件变量
pthread_cond_init函数
man pthread_cond_init
pthread_cond_wait函数
man pthread_cond_wait
pthread_cond_signal函数
pthread_cond_destroy函数
pthread_cond_timedwait函数
man pthread_cond_timedwait
pthread_cond_broadcast函数
man pthread_cond_broadcast
生产者消费者案例升级版
cond.c
/*
条件变量的类型 pthread_cond_t
int pthread_cond_init(pthread_cond_t *restrict cond, const pthread_condattr_t *restrict attr);
int pthread_cond_destroy(pthread_cond_t *cond);
int pthread_cond_wait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex);
- 等待,调用了该函数,线程会阻塞。
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
- 等待多长时间,调用了这个函数,线程会阻塞,直到指定的时间结束。
int pthread_cond_signal(pthread_cond_t *cond);
- 唤醒一个或者多个等待的线程
int pthread_cond_broadcast(pthread_cond_t *cond);
- 唤醒所有的等待的线程
*/
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
typedef struct node
{
/* data */
int num;
struct node *next;
}Node;
// 头结点
struct node * head = NULL;
// 创建一个互斥量
pthread_mutex_t mutex;
// 创建条件变量
pthread_cond_t cond;
void * producer(void *arg) {
srand((unsigned int)time(NULL));
// 不断的创建新的节点,添加到链表中
while(1) {
pthread_mutex_lock(&mutex);
Node * newNode = (Node*)malloc(sizeof(Node));
newNode->next = head;
head = newNode;
newNode->num = rand() % 1000;
printf("add node, num : %d, tid: %ld\n", newNode->num, pthread_self());
// 只要生产了一个,就通知消费者消费
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
void * customer(void *arg) {
while(1) {
pthread_mutex_lock(&mutex);
// 保存头结点的指针
Node *tmp = head;
// 判断是否有数据
if(head != NULL) {
// 有数据
head = head->next;
printf("del node, num: %d, tid: %ld\n", tmp->num, pthread_self());
free(tmp);
pthread_mutex_unlock(&mutex);
usleep(100);
} else {
// 没有数据,需要等待
// 当这个函数调用阻塞的时候,会对互斥锁进行解锁,当不阻塞的,继续向下执行,会重新加锁。
pthread_cond_wait(&cond, &mutex);
pthread_mutex_unlock(&mutex);
}
}
return NULL;
}
int main() {
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 创建5个生产者线程,和5个消费者线程
pthread_t ptids[5], ctids[5];
for(int i = 0; i < 5; i++) {
pthread_create(&ptids[i], NULL, producer, NULL);
pthread_create(&ctids[i], NULL, customer, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(ptids[i], NULL);
pthread_join(ctids[i], NULL);
}
// while(1) {
// sleep(10);
// }
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
pthread_exit(NULL);
return 0;
}
生产者中的pthread_cond_signal(&cond)信号是唤醒一个或者多个睡眠的线程,如果容器中的数据足够多,消费者不执行pthread_cond_wait(&cond,&mutex),即如果数据足够多,线程没有休眠,即使收到了信号也不会做任何的处理。
当缓冲区为空,不用条件变量时,消费者处于无意义的循环,此时只执行加锁和解锁,直到时间片完才发生线程切换,浪费cpu资源。而加入条件变量,一旦发现缓存区是空的,会立刻从运行态进入阻塞态,此时一定会发生线程切换,把cpu资源让出。互斥锁和条件变量都可以实现线程同步,但使用条件变量效率更高。
生产者消费者案例改良版
cond.c
/*
条件变量的类型 pthread_cond_t
int pthread_cond_init(pthread_cond_t *restrict cond, const pthread_condattr_t *restrict attr);
int pthread_cond_destroy(pthread_cond_t *cond);
int pthread_cond_wait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex);
- 等待,调用了该函数,线程会阻塞。
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
- 等待多长时间,调用了这个函数,线程会阻塞,直到指定的时间结束。
int pthread_cond_signal(pthread_cond_t *cond);
- 唤醒一个或者多个等待的线程
int pthread_cond_broadcast(pthread_cond_t *cond);
- 唤醒所有的等待的线程
*/
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
typedef struct node
{
/* data */
int num;
struct node *next;
}Node;
// 头结点
struct node * head = NULL;
// 创建一个互斥量
pthread_mutex_t mutex;
// 创建条件变量
pthread_cond_t cond;
void * producer(void *arg) {
srand((unsigned int)time(NULL));
// 不断的创建新的节点,添加到链表中
while(1) {
pthread_mutex_lock(&mutex);
Node * newNode = (Node*)malloc(sizeof(Node));
newNode->next = head;
head = newNode;
newNode->num = rand() % 1000;
printf("add node, num : %d, tid: %ld\n", newNode->num, pthread_self());
// 只要生产了一个,就通知消费者消费
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
void * customer(void *arg) {
while(1) {
pthread_mutex_lock(&mutex);
// 判断是否有数据
while(head == NULL) {
// 没有数据,需要等待
// 当这个函数调用阻塞的时候,会对互斥锁进行解锁,当不阻塞的,继续向下执行,会重新加锁。
pthread_cond_wait(&cond, &mutex);
}
// 保存头结点的指针
Node *tmp = head;
assert(head != NULL);
// 有数据
head = head->next;
printf("del node, num: %d, tid: %ld\n", tmp->num, pthread_self());
free(tmp);
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
int main() {
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 创建5个生产者线程,和5个消费者线程
pthread_t ptids[5], ctids[5];
for(int i = 0; i < 5; i++) {
pthread_create(&ptids[i], NULL, producer, NULL);
pthread_create(&ctids[i], NULL, customer, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(ptids[i], NULL);
pthread_join(ctids[i], NULL);
}
// while(1) {
// sleep(10);
// }
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
pthread_exit(NULL);
return 0;
}
生产者消费者案例修改版
cond.c
/*
条件变量的类型 pthread_cond_t
int pthread_cond_init(pthread_cond_t *restrict cond, const pthread_condattr_t *restrict attr);
int pthread_cond_destroy(pthread_cond_t *cond);
int pthread_cond_wait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex);
- 等待,调用了该函数,线程会阻塞。
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
- 等待多长时间,调用了这个函数,线程会阻塞,直到指定的时间结束。
int pthread_cond_signal(pthread_cond_t *cond);
- 唤醒一个或者多个等待的线程
int pthread_cond_broadcast(pthread_cond_t *cond);
- 唤醒所有的等待的线程
*/
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
typedef struct node
{
/* data */
int num;
struct node *next;
}Node;
// 头结点
Node * head = NULL;
// 创建一个互斥量
pthread_mutex_t mutex;
// 创建条件变量
pthread_cond_t cond;
void * producer(void *arg) {
srand((unsigned int)time(NULL));
// 不断的创建新的节点,添加到链表中
while(1) {
pthread_mutex_lock(&mutex);
Node * newNode = (Node*)malloc(sizeof(Node));
newNode->next = head;
head = newNode;
newNode->num = rand() % 1000;
printf("add node, num : %d, tid: %ld\n", newNode->num, pthread_self());
// 只要生产了一个,就通知消费者消费
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
void * customer(void *arg) {
while (1)
{
pthread_mutex_lock(&mutex);
pthread_cond_wait(&cond, &mutex);
// 保存头结点的指针
Node *tmp = head;
// 有数据
head = head->next;
printf("del node, num : %d, tid : %ld\n", tmp->num, pthread_self());
free(tmp);
pthread_mutex_unlock(&mutex);
usleep(100);
// 等待
}
return NULL;
}
int main() {
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 创建5个生产者线程,和5个消费者线程
pthread_t ptids[5], ctids[5];
for(int i = 0; i < 5; i++) {
pthread_create(&ptids[i], NULL, producer, NULL);
pthread_create(&ctids[i], NULL, customer, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(ptids[i], NULL);
pthread_join(ctids[i], NULL);
}
// while(1) {
// sleep(10);
// }
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
pthread_exit(NULL);
return 0;
}
信号量
sem_init函数
man sem_init
sem_init() 在 sem 指向的地址处初始化未命名信号量。 value 参数指定信号量的初始值。
pshared 参数指示该信号量是在进程的线程之间还是在进程之间共享。
如果 pshared 的值为 0,则信号量在进程的线程之间共享,并且应该位于所有线程可见的某个地址(例如,全局变量或在堆上动态分配的变量)。
如果 pshared 非零,则信号量在进程之间共享,并且应该位于共享内存区域中(请参阅 shm_open(3)、mmap(2) 和 shmget(2))。 (由于 fork(2) 创建的子进程继承其父进程的内存映射,因此它也可以访问信号量。)任何可以访问共享内存区域的进程都可以使用 sem_post(3)、sem_wait(3) 和 sem_wait(3)等 对信号量进行操作 。
sem_wait函数
man sem_wait
sem_wait() 递减(锁定)sem 指向的信号量。 如果信号量的值大于零,则继续递减,并且函数立即返回。 如果信号量当前的值为零,则调用将阻塞,直到可以执行递减(即信号量值上升到零以上),或者信号处理程序中断调用。
sem_post函数
man sem_post
sem_post() 递增(解锁)sem 指向的信号量。 如果信号量的值因此变得大于零,则在 sem_wait(3) 调用中阻塞的另一个进程或线程将被唤醒并继续锁定信号量。
信号量的大小决定了容器的大小
sem_destroy函数
man sem_destroy
信号量小案例
semaphore.c
/*
信号量的类型 sem_t
int sem_init(sem_t *sem, int pshared, unsigned int value);
- 初始化信号量
- 参数:
- sem : 信号量变量的地址
- pshared : 0 用在线程间 ,非0 用在进程间
- value : 信号量中的值
int sem_destroy(sem_t *sem);
- 释放资源
int sem_wait(sem_t *sem);
- 对信号量加锁,调用一次对信号量的值-1,如果值为0,就阻塞
int sem_trywait(sem_t *sem);
int sem_timedwait(sem_t *sem, const struct timespec *abs_timeout);
int sem_post(sem_t *sem);
- 对信号量解锁,调用一次对信号量的值+1
int sem_getvalue(sem_t *sem, int *sval);
sem_t psem;
sem_t csem;
init(psem, 0, 8);
init(csem, 0, 0);
producer() {
sem_wait(&psem);
sem_post(&csem)
}
customer() {
sem_wait(&csem);
sem_post(&psem)
}
*/
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <unistd.h>
#include <semaphore.h>
#include <time.h>
typedef struct node
{
/* data */
int num;
struct node *next;
}Node;
// 头结点
Node * head = NULL;
// 创建一个互斥量
pthread_mutex_t mutex;
// 创建两个信号量
sem_t psem;
sem_t csem;
void * producer(void *arg) {
srand((unsigned int)time(NULL));
// 不断的创建新的节点,添加到链表中
while(1) {
sem_wait(&psem);
pthread_mutex_lock(&mutex);
Node * newNode = (Node*)malloc(sizeof(Node));
newNode->next = head;
head = newNode;
newNode->num = rand() % 1000;
printf("add node, num : %d, tid: %ld\n", newNode->num, pthread_self());
pthread_mutex_unlock(&mutex);
sem_post(&csem);
}
return NULL;
}
void * customer(void *arg) {
while (1)
{
sem_wait(&csem);
pthread_mutex_lock(&mutex);
// 保存头结点的指针
Node *tmp = head;
// 有数据
head = head->next;
printf("del node, num : %d, tid : %ld\n", tmp->num, pthread_self());
free(tmp);
pthread_mutex_unlock(&mutex);
sem_post(&psem);
}
return NULL;
}
int main() {
pthread_mutex_init(&mutex, NULL);
sem_init(&psem, 0, 8);
sem_init(&csem, 0, 0);
// 创建5个生产者线程,和5个消费者线程
pthread_t ptids[5], ctids[5];
for(int i = 0; i < 5; i++) {
pthread_create(&ptids[i], NULL, producer, NULL);
pthread_create(&ctids[i], NULL, customer, NULL);
}
for(int i = 0; i < 5; i++) {
pthread_join(ptids[i], NULL);
pthread_join(ctids[i], NULL);
}
// while(1) {
// sleep(10);
// }
pthread_mutex_destroy(&mutex);
pthread_exit(NULL);
return 0;
}
有信号量也要用互斥锁,因为信号量实现进程/线程间的同步,互斥锁实现对共享资源的互斥访问
使用linux线程库的信号量函数,完成经典的生产者和消费者模型
prodcust.c
#include <pthread.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <time.h>
#include <sys/time.h>
#include <sys/wait.h>
#include <stdlib.h>
#include <semaphore.h>
//缓冲区
int *buf;
int bufSize = 100;
int bufPtr;
int count;
//三个信号量
sem_t full, empty, mutex;
//生产者线程
void *producer(void *arg)
{
while (bufPtr < bufSize)
{
//信号量模型
sem_wait(&full);
sem_wait(&mutex);
buf[++bufPtr] = bufPtr;
printf("Producer: pid[%ld], produce data[%d]\n", pthread_self(), buf[bufPtr]);
sem_post(&mutex);
sem_post(&empty);
}
}
//消费者线程
void *consumer(void *arg)
{
while (1)
{
//信号量模型
sem_wait(&empty);
sem_wait(&mutex);
count = (count + 1) % __INT32_MAX__;
printf("Consumer: pid[%ld], count[%d], data[%d]\n", pthread_self(), count, buf[bufPtr--]);
sem_post(&mutex);
sem_post(&full);
}
}
int main()
{
//初始化三个信号量
sem_init(&full, 0, bufSize);
sem_init(&empty, 0, 0);
sem_init(&mutex, 0, 1);
//初始化读写指针、缓冲区
bufPtr = -1;
count = 0;
buf = (int *)malloc(sizeof(int) * bufSize);
//创建6个线程,一个作生产者,5个消费者
pthread_t ppid, cpids[5];
pthread_create(&ppid, NULL, producer, NULL);
for (int i = 0; i < 5; ++i)
{
pthread_create(&cpids[i], NULL, consumer, NULL);
}
pthread_join(ppid, NULL);
for (int i = 0; i < 5; ++i)
{
pthread_join(cpids[i], NULL);
}
//主线程结束
pthread_exit(NULL);
return 0;
}
之后我会持续更新,如果喜欢我的文章,请记得一键三连哦,点赞关注收藏,你的每一个赞每一份关注每一次收藏都将是我前进路上的无限动力 !!!↖(▔▽▔)↗感谢支持!