一、新建短信微服务
1、在service模块下创建子模块service-msm、创建controller和service代码
2、配置application.properties
#服务端口
server:
port: 8005
#服务名
spring:
application:
name: service-cms
#服务地址
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
#返回json的全局时间格式
jackson:
date-format: yyyy-MM-dd HH:mm:ss
time-zone: GMT+8
#Redis配置
redis:
host: 127.0.0.1
port: 6379
database: 0
timeout: 1800000
lettuce:
pool:
max-active: 20
max-wait: -1
#最大阻塞等待时间(负数表示没有限制)
max-idle: 5
min-idle: 0
3、创建启动类
创建MsmApplication.java
package com.atguigu.msmservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
import org.springframework.context.annotation.ComponentScan;
/**
* @Author panghl
* @Date 2021/2/2 22:10
* @Description TODO
**/
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
//@EnableDiscoveryClient
//@EnableFeignClients
@ComponentScan(basePackages = {"com.atguigu"})
public class MsmApplication {
public static void main(String[] args) {
SpringApplication.run(MsmApplication.class,args);
}
}
二、阿里云短信服务
帮助文档:
https://help.aliyun.com/product/44282.html?spm=5176.10629532.0.0.38311cbeYzBm73
1、开通阿里云短信服务
阿里云短信服务 新用户可以0元免费试用一百条!!!
2、添加签名管理与模板管理
(1)添加模板管理
选择 国内消息 - 模板管理 - 添加模板

点击 添加模板,进入到添加页面,输入模板信息
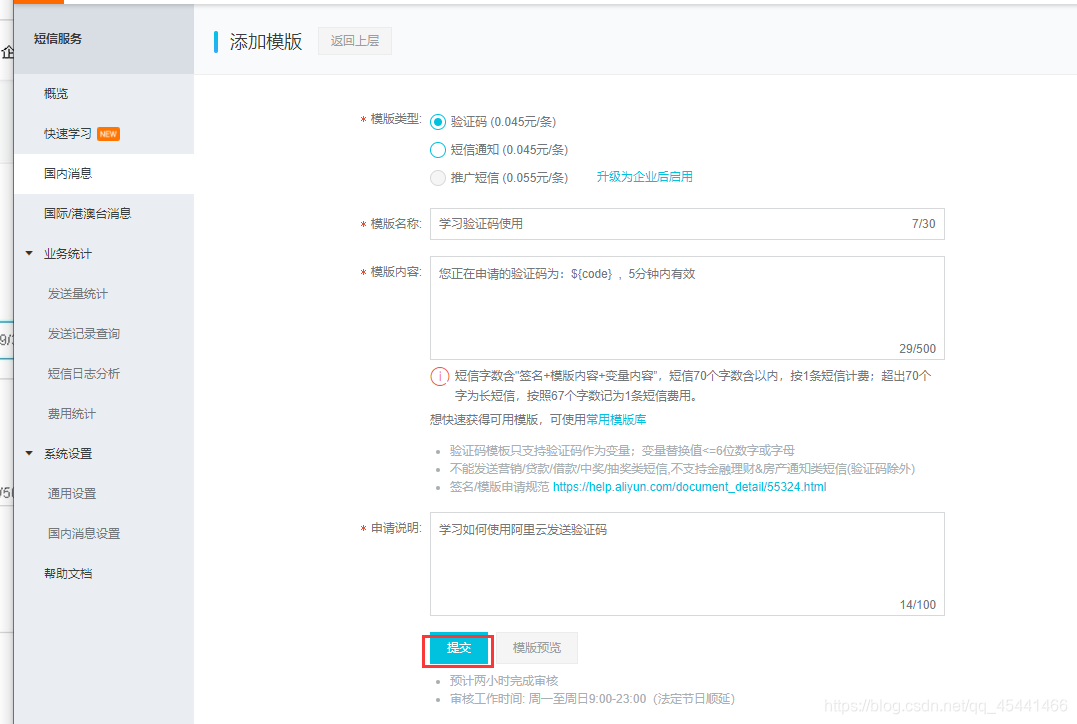
点击提交,等待审核,审核通过后可以使用
(2)添加签名管理
选择 国内消息 - 签名管理 - 添加签名
点击添加签名,进入添加页面,填入相关信息
注意:签名要写的有实际意义
点击提交,等待审核,审核通过后可以使用
由于没有申请到签名-我用email代替了。(包含整合freemarker、thymeleaf发送邮件)
可查看我另一篇播客详解:https://blog.csdn.net/qq_45441466/article/details/113611153
三、编写发送短信接口
1、在service-msm的pom中引入依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
</dependency>
2、编写controller,根据手机号发送短信
package com.atguigu.msmservice.controller;
import com.atguigu.common.R;
import com.atguigu.msmservice.service.MsmService;
import com.atguigu.msmservice.utils.RandomUtil;
import io.swagger.annotations.Api;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.HashMap;
import java.util.Map;
/**
* @Author panghl
* @Date 2021/2/2 22:11
* @Description TODO
**/
@RestController
@CrossOrigin
@Api(tags = {"短信服务"})
@RequestMapping("/edumsm/msm")
public class MsmController {
@Autowired
private MsmService msmService;
//发送短信的方法
@GetMapping("/send/{phone}")
public R sendMsm(@PathVariable("phone") String phone) {
//生成随机值,传递阿里云进行发送
String code = RandomUtil.getFourBitRandom();
Map<String, Object> param = new HashMap<>();
param.put("code", code);
param.put("phone", phone);
boolean isSend = msmService.sendMsm(param,phone);
if (isSend) {
return R.ok();
} else {
return R.error();
}
}
}
3、编写service
package com.atguigu.msmservice.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.aliyuncs.CommonRequest;
import com.aliyuncs.CommonResponse;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.profile.DefaultProfile;
import com.atguigu.msmservice.service.MsmService;
import com.atguigu.msmservice.utils.ConstantPropertiesUtils;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import java.util.Map;
/**
* @Author panghl
* @Date 2021/2/2 22:12
* @Description TODO
**/
@Service
public class MsmServiceImpl implements MsmService {
/**
* 发送短信
* @param param
* @return
*/
@Override
public boolean sendMsm(Map<String, Object> param,String phone) {
if(StringUtils.isEmpty(phone)) {return false;}
DefaultProfile profile = DefaultProfile.getProfile("default", ConstantPropertiesUtils.KEY_ID, ConstantPropertiesUtils.KEY_SECRET);
IAcsClient client = new DefaultAcsClient(profile);
//设置相关参数
CommonRequest request = new CommonRequest();
request.setSysMethod(MethodType.POST);
request.setSysDomain("dysmsapi.aliyuncs.com");
request.setSysVersion("2017-05-15");
request.setSysAction("SendSms");
//设置要发送相关的参数
request.putQueryParameter("RegionId", "cn-hangzhou");
request.putQueryParameter("PhoneNumbers",phone);//设置要发送的手机号
request.putQueryParameter("SignName", "待补充"); //申请阿里云 签名名称
request.putQueryParameter("TemplateCode", "SMS_211220037"); //申请阿里云 模板code
request.putQueryParameter("TemplateParam", JSONObject.toJSONString(param)); //验证码数据,转换json数据传递
//最终发送
try {
//最终发送
CommonResponse commonResponse = client.getCommonResponse(request);
boolean success = commonResponse.getHttpResponse().isSuccess();
return success;
} catch (ClientException e) {
e.printStackTrace();
return false;
}
}
}