1.标准IO函数时候讲解的时钟代码,要求输入quit字符串后,结束进程
#include<stdio.h>
#include <string.h>
#include <time.h>
#include <unistd.h>
#include <pthread.h>
void* callBack(void* arg)
{
time_t t;
struct tm *p;
while(1)
{
t = time(NULL);
p = localtime(&t);
printf("%4d-%02d-%02d %02d:%02d:%02d\n",\
p->tm_year+1900,p->tm_mon+1,p->tm_mday,\
p->tm_hour,p->tm_min,p->tm_sec);
sleep(3);
}
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
pthread_t tid;
if(pthread_create(&tid, NULL, callBack, NULL) != 0)
{
fprintf(stderr, "pthread_create failed %d\n", __LINE__);
return -1;
}
printf("实时时间:\n");
char str[20];
scanf("%s", str);
if(strcmp(str,"quit") == 0)
{
pthread_cancel(tid);
}
return 0;
}
结果:
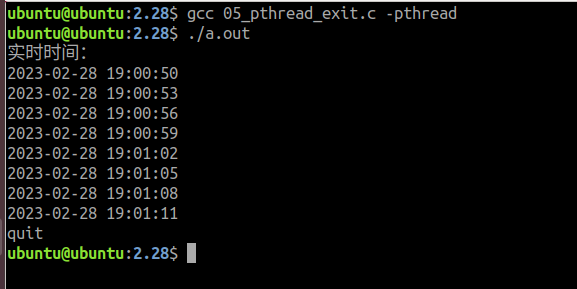
2.要求定义一个全局变量char bufD = "1234567", 创建两个线程,不考虑退出条件。
a. A线程循环打印buf字符串,
b. B线程循环倒置buf字符串,即buf中本来存储1234567, 倒置后buf中存储7654321.不打印! !
c.倒置不允许使用辅助数组。
d.要求A线程打印出来的结果只能为1234567或者7654321
e.不允许使用sleep函数
#include<stdio.h>
#include <unistd.h>
#include <pthread.h>
#include <string.h>
char buf[] = "1234567";
void* callBaackA(void* arg)
{
while(1)
{
printf("%s\n", buf);
sleep(1);
}
pthread_exit(NULL);
}
void* callBaackB(void* arg)
{
char t;
int i = 0;
int j = strlen(buf)-1;
while(i < j)
{
t=buf[i];buf[i]=buf[j];buf[j]=t;
i++;j--;
}
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
pthread_t tid1,tid2;
if(pthread_create(&tid1, NULL, callBaackA, NULL) != 0)
{
fprintf(stderr, "pthread_create1 failed %d\n", __LINE__);
return -1;
}
if(pthread_create(&tid2, NULL, callBaackB, NULL) != 0)
{
fprintf(stderr, "pthread_create2 failed %d\n", __LINE__);
return -1;
}
printf("%s\n", buf);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
3.用两个线程拷贝一张图片,A线程拷贝前半部分,B线程拷贝后半部分
#include<stdio.h>
#include <unistd.h>
#include <pthread.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <fcntl.h>
void* callBackA(void* arg)
{
int fd = open("./1.png", O_RDONLY);
if(fd < 0)
{
perror("open");
return NULL;
}
int fd1 = open("./3.png", O_WRONLY|O_CREAT|O_TRUNC, 0664);
if(fd1 < 0)
{
perror("open");
return NULL;
}
struct stat buf;
if(stat("./1.png", &buf) < 0)
{
perror("stat");
return NULL;
}
printf("%ld\n", buf.st_size);
off_t size = buf.st_size;
off_t mid = size/2;
int flag = size%2;
lseek(fd, 0, SEEK_SET);
lseek(fd1, 0, SEEK_SET);
char c = 0;
for(int i=0; i<mid; i++)
{
read(fd, &c, 1);
write(fd1, &c, 1);
}
printf("前半部分拷贝完成\n");
pthread_exit(NULL);
}
void* callBackB(void* arg)
{
int fd = open("./1.png", O_RDONLY);
if(fd < 0)
{
perror("open");
return NULL;
}
int fd1 = open("./3.png", O_WRONLY|O_CREAT|O_TRUNC, 0664);
if(fd1 < 0)
{
perror("open");
return NULL;
}
struct stat buf;
if(stat("./1.png", &buf) < 0)
{
perror("stat");
return NULL;
}
printf("%ld\n", buf.st_size);
off_t size = buf.st_size;
off_t mid = size/2;
int flag = size%2;
sleep(1);
lseek(fd, mid, SEEK_SET);
lseek(fd1, mid, SEEK_SET);
char c = 0;
for(int i=0; i<mid+flag; i++)
{
read(fd, &c, 1);
write(fd1, &c, 1);
}
printf("后半部分拷贝完成\n");
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
pthread_t tid1,tid2;
if(pthread_create(&tid1, NULL, callBackA, NULL) != 0)
{
fprintf(stderr,"pthread_create failed\n");
return -1;
}
if(pthread_create(&tid2, NULL, callBackB, NULL) != 0)
{
fprintf(stderr,"pthread_create failed\n");
return -1;
}
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}