1、if语句
if
语句的三种形态
形态1:如果。。。那么。。。
#include <iostream>using namespace std;int main( void ) {int salary;cout << " 你月薪多少 ?" ;cin >> salary;if (salary < 20000) {
cout << " 你是一个好人 , 我还不想谈恋爱 ." << endl;}system( "pause" );return 0;}
形态2:如果。。。那么。。。,否则。。
#include <iostream>using namespace std;int main( void ) {int salary;cout << " 你月薪多少 ?" << endl ;cin >> salary;if (salary < 20000) {cout << " 你是一个好人 , 我还不想谈恋爱 ." << endl;} else {cout << " 我没有什么要求 , 只要你对我好就行 ." << endl;}system( "pause" );return 0;}
形态
3
#include <iostream>#include <string>#include <stdio.h>using namespace std;int main( void ){int salary;string houseRet; // 是否有房string carRet; // 是否有车cout << " 你的月薪是多少 ?" << endl;cin >> salary;cout << " 你有房吗? " << endl;cin >> houseRet;cout << " 你有车吗? " << endl;cin >> carRet;if (salary < 20000) {cout << " 你是一个好人, 我现在还不想谈恋爱 ." << endl;} else if (houseRet == " 有 " ) {cout << " 我其实没有什么要求,只要你对我好 ." << endl;} else if (carRet == " 有 " ) {cout << " 还不错哦, 以后再联系 ." << endl;} else {cout << " 有缘再见 ." << endl;}system( "pause" );return 0;}
if 语句的嵌套
实例:
求
3
个数的最大值。
#include <iostream>#include <windows.h>using namespace std;int main( void ) {int x, y, z;cout << " 请输入 3 个整数 : " << endl;cin >> x >> y >> z;if (x > y) {if (x > z) {cout << " 最大值是: " << x << endl;} else {cout << " 最大值是: " << z << endl;}} else {if (y > z) {cout << " 最大值是: " << y << endl;} else {cout << " 最大值是: " << z << endl;}}system( "pause" );return 0;}
嵌套的常见错误(配对错误)
与前面最近的,而且还没有配对的 if 匹配
错误避免方法:
1 )严格使用 { }2 )先写{}再写里面的内容3 )保持良好的“缩进”
2、switch 语句
switch
的基本使用
语法:
switch (x) {case 表达式 1 :语句 1break;case 表达式 2 :语句 2break;case 表达式 3 :语句 3break;default 表达式 1 :语句 1break;}
注意:switch 表达式,这个表达式只能取整数类型!!
流程图:
demo
#include <iostream>#include <windows.h>using namespace std;int main(void) {int num;cout << " 请输入一个数字 : ";cin >> num;switch (num) {case 1:cout << " 星期一:包子 " << endl; break;case 2:cout << " 星期二:馒头 " << endl;break;case 3:cout << " 星期三:稀饭 " << endl; break;case 4:cout << " 星期四:白菜 " << endl; break;case 5:cout << " 星期五:土豆 " << endl; break;case 6:case 7:cout << " 周末:休息 " << endl; break;default:cout << " 请输入 1-7" << endl; break;}system("pause");return 0;}
switch
和
if
的选择
switch
: 用于
int/char/long/long long
类型的变量,和多个特定常量的判断处理。 (float 和 double 类型不可以),
注意:
即
case
表达式,这个表达式只
能取整数,整数表达式,不能取
其它类型的表达式!!
if:
适用于各种逻辑判断
-常见错误总结
switch 错误
以下错误,在
vs/vc
中有提示,但是仍可以通过编译。
在
gcc
编译器中,不能通过编译。
#include <stdio.h>
int main(void) {
int c;
scanf("%d", &c);
switch (c) {
case 1:
int x = 0; //错误!
printf("c=1");
break;
case 2:
printf("c=2");
break;
default:
printf("other");
break;
}
return 0;
}
case语句里不能直接定义变量!!
应该修改为:
#include <stdio.h>int main(void) {int c;scanf("%d", &c);switch(c) {case 1:{int x = 0; //错误!printf("c=1");}break;case 2:printf("c=2");break;default:printf("other");break;}return 0;}
case语句里定义变量的话,要加{ }来括起来!!
不安全函数(
scanf
等)
#include <iostream>#include <string>#include <stdio.h>using namespace std;int main( void ){int num;scanf( "%d" , &num);system( "pause" );return 0;}
vs
中不能直接使用
scanf
等
C
标准库函数
因为
vs
使用更安全的
c11
标准
,
认为这类函数不安全。
注明,这类函数正常使用时,是没有任何问题的但是,部分黑客可能会利用其中的缺陷,开发恶意软件,对系统造成影响
解决方案:
1.
方法
1
:使用修改项目的属性,直接使用这些“不安全”的函数。
添加:
/D _CRT_SECURE_NO_WARNINGS
2.
方法
2
:使用
c11
标准中的“更安全”的函数 scanf_s
gets 不能使用,使用 gets_s
gets
是老标准
C
语言函数,
vs
使用更安全的
c11
标准
,
使用对应的
gets_s
char line[32];
gets_s(line,
sizeof
(line));
scanf 不能使用
原因同上,改用 scanf_s
int x;scanf_s("%d", &x); // 不需要使用第 3 个参数,用法和 scanf 相同float f;scanf_s("%f", &f); // 不需要使用第 3 个参数 , 用法和 scanf 相同char c;scanf_s("%c", &c, sizeof(c)); //需要使用第 3 个参数, 否则有告警char name[16];scanf_s("%s", name, sizeof(name)); // 需要使用第 3 个参数int age;char name[16];scanf_s("%d%s", &age, name, sizeof(name));
使用scanf_s函数在输入字符类型,或者字符数组时,要加第三个参数,否则会出现编译警告。
cin >> 的返回值
#include <iostream>#include <string>#include <Windows.h>using namespace std;int main( void ) {string word;int count = 0;int length = 0;cout << " 请输入任意多个单词: " ;while (1) {if ((cin >> word) == 0) { // 在 vs 中不能通过编译break ;}count++;length += word.length();}cout << " 一共有 " << count << " 单词 " << endl;cout << " 总长度: " << length << endl;system( "pause" );return 0;}
把
if
((cin >> word) == 0)
修改为:
if ((bool)(std::cin >> word) == 0) {或者修改为: if (!(cin >> word)) {第二种改法是个重点,以后用到的多!重中之重!!一定要熟悉
getline 的返回值#include <iostream>#include <string>#include <Windows.h>using namespace std;int main( void ) {string line;int lineCount = 0;int length = 0;cout << " 请输入任意多行: " ;while (1) {// 遇到文件结束符时, 返回 NULL ( 0 )if (getline(cin, line) == 0) {//在 vs 中不能通过编译break ;}lineCount++;length += line.length();}cout << " 一共有 " << lineCount << " 行 " << endl;cout << " 总长度 : " << length << endl;system( "pause" );return 0;}
修改:
if (getline(cin, line) == 0) {
修改为:
if ((bool)getline(cin, line) == 0) {或者修改为: if (!getline(cin, line)) {这两个修改都是重中之重!! 重中之重!!一定要熟悉
if 语句后面误加分号
int age;cout << "请输入年龄: " ;cin >> age;if (age > 40); {cout << "大叔" << endl;}
严格遵循代码规范,做到零警告。以上代码在 VS
中编译时,会有警告
warning
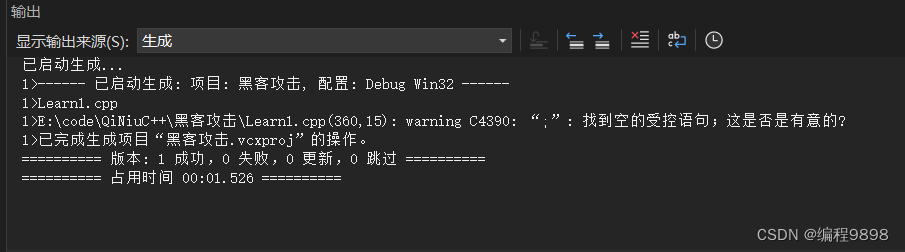
3、计算机英语加油站
bool
|
布尔
逻辑类型
|
if
|
如果
|
else
|
否则
|
switch
|
开关
|
case
|
情况
|
default
|
默认
|
4、职场修炼:怎样优雅地避免加班
996 工作制?9-6-5制定合理的项目计划提高工作效率(要有一定的技术积累)养成良好的工作习惯【不加班】每天有目标的学习 1 小时以上
5、练习
习题
1.
让用户输入一个字符, 然后进行转换:
如果是大写字母,就转换为小写字母
如果是小写字母,就转换为大写字母
如果是其它字符,不做任何转换
#include <iostream>#include <string>#include <Windows.h>using namespace std;int main( void ) {char c;cout << " 请输入一个字符 : " << endl;cin >> c;if (c >= 'a' && c <= 'z' ) {c = c - 'a' + 'A' ;} else if (c >= 'A' && c <= 'Z' ) {c = c - 'A' + 'a' ;}cout << c << endl;system( "pause" );return 0;}
习题
2.
让用户输入一个数字(
0-9
),然后输出对应的大写汉字。
注:
零 壹 贰 叁 肆 伍 陆 柒 捌 玖
例如,用户输入
3
, 输出“叁”
#include <iostream>#include <string>#include <Windows.h>using namespace std;// 零 壹 贰 叁 肆 伍 陆 柒 捌 玖int main( void ) {int num;string ret;cout << " 请输入一个数字 [0-9]: " ;cin >> num;switch (num) {case 0:cout << " 零 " ;break ;case 1:cout << " 壹 " ;break ;case 2:cout << " 贰 " ;break ;case 3:cout << " 叁 " ;break ;case 4:cout << " 肆 " ;break ;case 5:cout << " 伍 " ;break ;case 6:cout << " 陆 " ;break ;case 7:cout << " 柒 " ;break ;case 8:cout << " 捌 " ;break ;case 9:cout << " 玖 " ;break ;default :break ;}cout << endl;system( "pause" );return 0;}
方法
2
:
用空间换速度。
#include <iostream>#include <string>#include <Windows.h>using namespace std;int main( void ) {int num;string ret[10] = { " 零 " , " 壹 " , " 贰 " , " 叁 " , " 肆 " , " 伍 " , " 陆 " , " 柒 " , " 捌 " ," 玖 " };cout << " 请输入一个数字 [0-9]: " ;cin >> num;if (num >=0 && num <=9) {cout << ret[num] << endl;}system( "pause" );return 0;}
习题
3.
让用户输入年份和月份,然后输出这个月有多少天。
说明:
闰年的
2
月份有
29
天
普通闰年: 能被 4 整除但不能被 100 整除的年份为世纪闰年: 能被 400 整除
#include <iostream>#include <Windows.h>#include <string>using namespace std;/*闰年的 2 月份有 29 天普通闰年 : 能被 4 整除但不能被 100 整除的年份为世纪闰年 : 能被 400 整除*/int main( void ) {int year;int month;bool flag = false ;int days;cout << " 请输入年份: " ;cin >> year;cout << " 请输入月份: " ;cin >> month;if (year % 400 == 0) {flag = true ;} else if (year % 4 == 0 && year % 100 != 0) {flag = true ;} else {flag = false ;}switch (month) {case 1:case 3:case 5:case 7:case 8:case 10:case 12:
days = 31;break ;case 2:days = flag ? 29 : 28;break ;case 4:case 6:case 9:case 11:days = 30;break ;default :std::cout << " 无效月份 " << std::endl;break ;}cout << year << " 年 " << month << " 月一共有: " << days << " 天 " << endl;system( "pause" );return 0;}