1 比赛规则
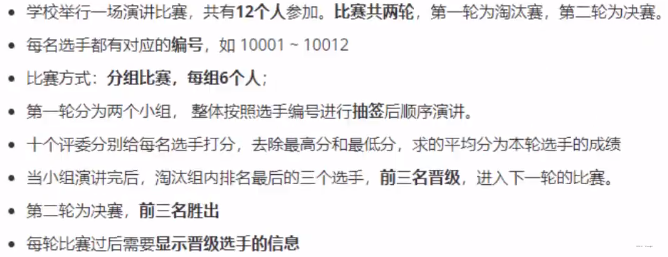
2 系统功能

3 实现过程
3.1 speechManager.h
#pragma once
#include<iostream>
#include<vector>
#include<deque>
#include<map>
#include<algorithm>
#include<functional>
#include<numeric>
#include<ctime>
#include<string>
#include<fstream>
#include"speaker.h"
using namespace std;
class speechManager {
public:
speechManager();
void show_menu();
void initSpeech();
vector<int> v1;
vector<int> v2;
vector<int> v3;
map<int, speaker> m;
int index;
void creatSpeaker();
void startspeech();
void speechchouqian();
void speeching();
void showScore();
void saveScore();
void loadScore();
bool fileIsEmpty;
map<int, vector<string>> Score;
void showAllScore();
void clearScore();
void exitSystem();
~speechManager();
};
3.2 speaker.h
#pragma once
#include<iostream>
using namespace std;
class speaker {
public:
string m_name;
double m_score[2];
};
3.3 speechManager.cpp
#include"speechManager.h"
speechManager::speechManager() {
this->initSpeech();
this->creatSpeaker();
this->loadScore();
};
void speechManager::show_menu() {
cout << "**********************" << endl;
cout << "**********************" << endl;
cout << "***演讲比赛管理系统***" << endl;
cout << "*** 1 开始演讲比赛 ***" << endl;
cout << "*** 2 往届比赛记录 ***" << endl;
cout << "*** 3 清空比赛记录 ***" << endl;
cout << "*** 4 退出管理系统 ***" << endl;
cout << "**********************" << endl;
cout << "**********************" << endl;
}
void speechManager::initSpeech() {
this->v1.clear();
this->v2.clear();
this->v3.clear();
this->m.clear();
this->index = 1;
}
void speechManager::creatSpeaker() {
string nameSeed = "ABCDEFGHIJKL";
for (int i = 0; i < nameSeed.size(); i++) {
speaker sp;
sp.m_name = nameSeed[i];
sp.m_score[0] = 0;
sp.m_score[1] = 0;
this->v1.push_back(10001 + i);
this->m.insert(make_pair(10001 + i, sp));
}
}
void speechManager::startspeech() {
this->speechchouqian();
this->speeching();
this->showScore();
this->index++;
this->speechchouqian();
this->speeching();
this->showScore();
this->saveScore();
cout << "本届比赛已结束" << endl;
system("pause");
}
void speechManager::speechchouqian() {
cout << "--------------- 第" << index << "轮抽签结果如下 ---------------" << endl;
if (index == 1) {
random_shuffle(v1.begin(), v1.end());
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
else {
random_shuffle(v2.begin(), v2.end());
for (vector<int>::iterator it = v2.begin(); it != v2.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
system("pause");
cout << endl;
}
void speechManager::speeching() {
cout << "--------------- 第" << index << "轮比赛结果如下 ---------------" << endl;
vector<int> v_src;
srand((unsigned int)time(NULL));
if (this->index == 1) {
v_src = v1;
}
else {
v_src = v2;
}
multimap<double, int,greater<double>> groupScore;
int num = 0;
for (vector<int>::iterator it = v_src.begin(); it != v_src.end(); it++) {
deque<double> d;
for (int i = 0; i < 10; i++) {
double score = (rand() % 401 + 600) / 10.f;
d.push_back(score);
}
sort(d.begin(), d.end(), greater<double>());
d.pop_back();
d.pop_front();
double sum = accumulate(d.begin(), d.end(), 0.0f);
double avg = sum / (double)d.size();
this->m[*it].m_score[this->index - 1] = avg;
groupScore.insert(make_pair(avg, *it));
num++;
if (num % 6 == 0) {
cout << "--------------- 第" << num / 6 << "组成绩如下 ---------------" << endl;
for (multimap<double, int, greater<double>>::iterator it = groupScore.begin(); it != groupScore.end(); it++) {
cout << "编号:" << it->second
<< " 姓名:" << this->m[it->second].m_name
<< " 成绩:" << this->m[it->second].m_score[this->index - 1] << endl;
}
int count = 0;
for (multimap<double, int, greater<double>>::iterator it = groupScore.begin(); it != groupScore.end() && count < 3; it++, count++) {
if (this->index == 1) {
v2.push_back(it->second);
}
else {
v3.push_back(it->second);
}
}
groupScore.clear();
}
}
cout << "--------------- 第" << this->index << "轮比赛结束 ---------------" << endl;
system("pause");
}
void speechManager::showScore() {
cout << "--------------- 第" << this->index << "轮晋级结果如下 ---------------" << endl;
vector<int> v;
if (this->index == 1) {
v = v2;
}
else {
v = v3;
}
for (vector<int>::iterator it = v.begin(); it != v.end(); it++) {
cout << "编号:" << *it
<< " 姓名:" << this->m[*it].m_name
<< " 成绩:" << this->m[*it].m_score[this->index-1] << endl;
}
system("pause");
system("cls");
this->show_menu();
}
void speechManager::saveScore() {
ofstream ofs;
ofs.open("speechScore.csv", ios::out | ios::app);
for (vector<int>::iterator it = v3.begin(); it != v3.end(); it++) {
ofs << *it << "," << this->m[*it].m_score[1] << ",";
}
ofs << endl;
ofs.close();
cout << "--------------- 成绩已保存 ---------------" << endl;
this->fileIsEmpty = false;
}
void speechManager::loadScore() {
ifstream ifs;
ifs.open("speechScore.csv", ios::in);
if (!ifs.is_open()) {
this->fileIsEmpty = true;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof()) {
this->fileIsEmpty = true;
ifs.close();
return;
}
this->fileIsEmpty = false;
ifs.putback(ch);
string data;
int s_index = 0;
while (ifs >> data) {
vector<string> v;
int pos = -1;
int start = 0;
while (true) {
pos = data.find(",", start);
if (pos == -1) {
break;
}
string temp = data.substr(start, pos - start);
v.push_back(temp);
start = pos + 1;
}
this->Score.insert(make_pair(s_index, v));
s_index++;
}
ifs.close();
}
void speechManager::showAllScore() {
if (this->fileIsEmpty) {
cout << "文件为空或不存在" << endl;
system("pause");
return;
}
for (int i = 0; i < this->Score.size(); i++) {
cout << "第" << i + 1 << "届:" << endl;
cout << "冠军 编号:" << this->Score[i][0] << "\t得分:" << this->Score[i][1] << ""
<< " 亚军 编号:" << this->Score[i][2] << "\t得分:" << this->Score[i][3] << ""
<< " 季军 编号:" << this->Score[i][4] << "\t得分:" << this->Score[i][5] << endl << endl;
}
system("pause");
}
void speechManager::clearScore() {
cout << "确认清空吗" << endl;
cout << "1、确认" << endl;
cout << "2、返回" << endl;
int choice = 0;
cin >> choice;
if (choice == 1) {
ofstream ofs("speechScore.csv", ios::trunc);
ofs.close();
this->initSpeech();
this->creatSpeaker();
this->loadScore();
cout << "清空成功" << endl;
system("pause");
}
}
void speechManager::exitSystem() {
cout << "欢迎下次使用" << endl;
exit(0);
}
speechManager::~speechManager() {};
3.4 演讲比赛管理系统.cpp
#include<iostream>
#include<algorithm>
#include<string>
#include"speechManager.h"
using namespace std;
int main() {
while (true) {
speechManager sm;
sm.show_menu();
int func;
cout << "请选择功能:" << endl;
cin >> func;
switch (func)
{
case 1:
sm.startspeech();
system("cls");
break;
case 2:
sm.showAllScore();
system("cls");
break;
case 3:
sm.clearScore();
system("cls");
break;
default:
sm.exitSystem();
break;
}
}
}