实现效果
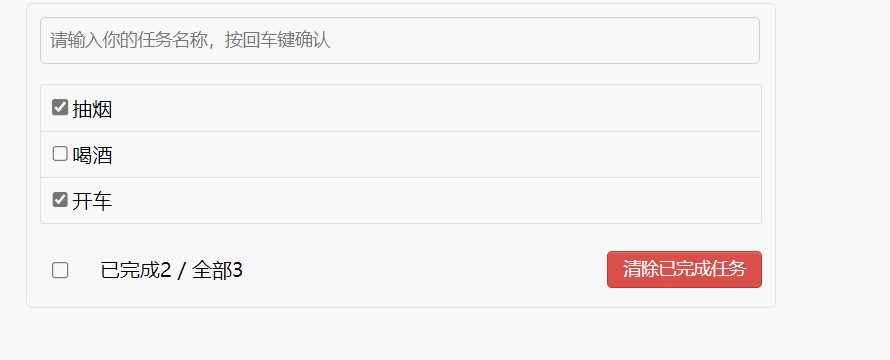
代码展示
main.js
//引入vue
import Vue from "vue";
//引入组件App
import App from "./App.vue";
//关闭vue的生产提示
Vue.config.productionTip = false;
//创建vm
new Vue({
el: "#app",
render: (h) => h(App),
});
App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<todo-head :addTodo="addTodo" />
<todo-list
:todoList="todoList"
:deleteTodo="deleteTodo"
:updateFlag="updateFlag"
/>
<todo-fotter
:todoList="todoList"
:updateFlagAll="updateFlagAll"
:deleteFlagAll="deleteFlagAll"
/>
</div>
</div>
</div>
</template>
<script>
import TodoList from "./components/todo-list.vue";
import TodoHead from "./components/todo-head.vue";
import TodoFotter from "./components/todo-fotter.vue";
//引入组件
export default {
components: { TodoHead, TodoList, TodoFotter },
name: "App",
data() {
return {
todoList: [
{ id: "001", name: "yb", flag: true },
{ id: "002", name: "yg", flag: false },
{ id: "003", name: "yf", flag: true },
],
};
},
methods: {
//添加
addTodo(todo) {
this.todoList.unshift(todo); //在数组最前方添加
},
//删除
deleteTodo(id) {
//过滤出 不是要删除id的 所有值
this.todoList = this.todoList.filter((todo) => todo.id != id);
},
//删除全部已选的
deleteFlagAll() {
this.todoList = this.todoList.filter((todo) => todo.flag === false);
},
//修改指定事 是否完成
updateFlag(id) {
this.todoList.forEach((todo) => {
if (todo.id === id) todo.flag = !todo.flag;
});
},
//修改全部的 是否完成
updateFlagAll(flag) {
this.todoList.forEach((todo) => {
todo.flag = flag;
});
},
//删除指定的
},
};
</script>
<style>
/*base*/
body {
background: plum;
}
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2),
0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
components里的 todo-head.vue
<template>
<div class="todo-header">
<input
type="text"
placeholder="请输入你的任务名称,按回车键确认"
@keyup.enter="add"
v-model="todoName"
/>
</div>
</template>
<script scoped>
//引入nanoid
import { nanoid } from "nanoid";
export default {
props: ["addTodo"],
data() {
return {
todoName: "",
};
},
methods: {
add() {
//去除前后空格判断不为空 进行添加
if (this.todoName.trim() != "" && this.todoName.trim() != null) {
const todo = { id: nanoid(), name: this.todoName, flag: false };
this.addTodo(todo);
this.todoName = "";
}
},
},
};
</script>
<style>
/*header*/
.todo-header input {
width: 560px;
height: 28px;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 4px;
padding: 4px 7px;
}
.todo-header input:focus {
outline: none;
border-color: rgba(82, 168, 236, 0.8);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075),
0 0 8px rgba(82, 168, 236, 0.6);
}
</style>
components里的todo-list.v