先为人物添加Box Collider 2D(2D物体的碰撞体)和Rigidbody 2D(2D物体的刚体)更为具体的可以查阅unity手册
动画制作
为人物添加Animatior组件并添加动画
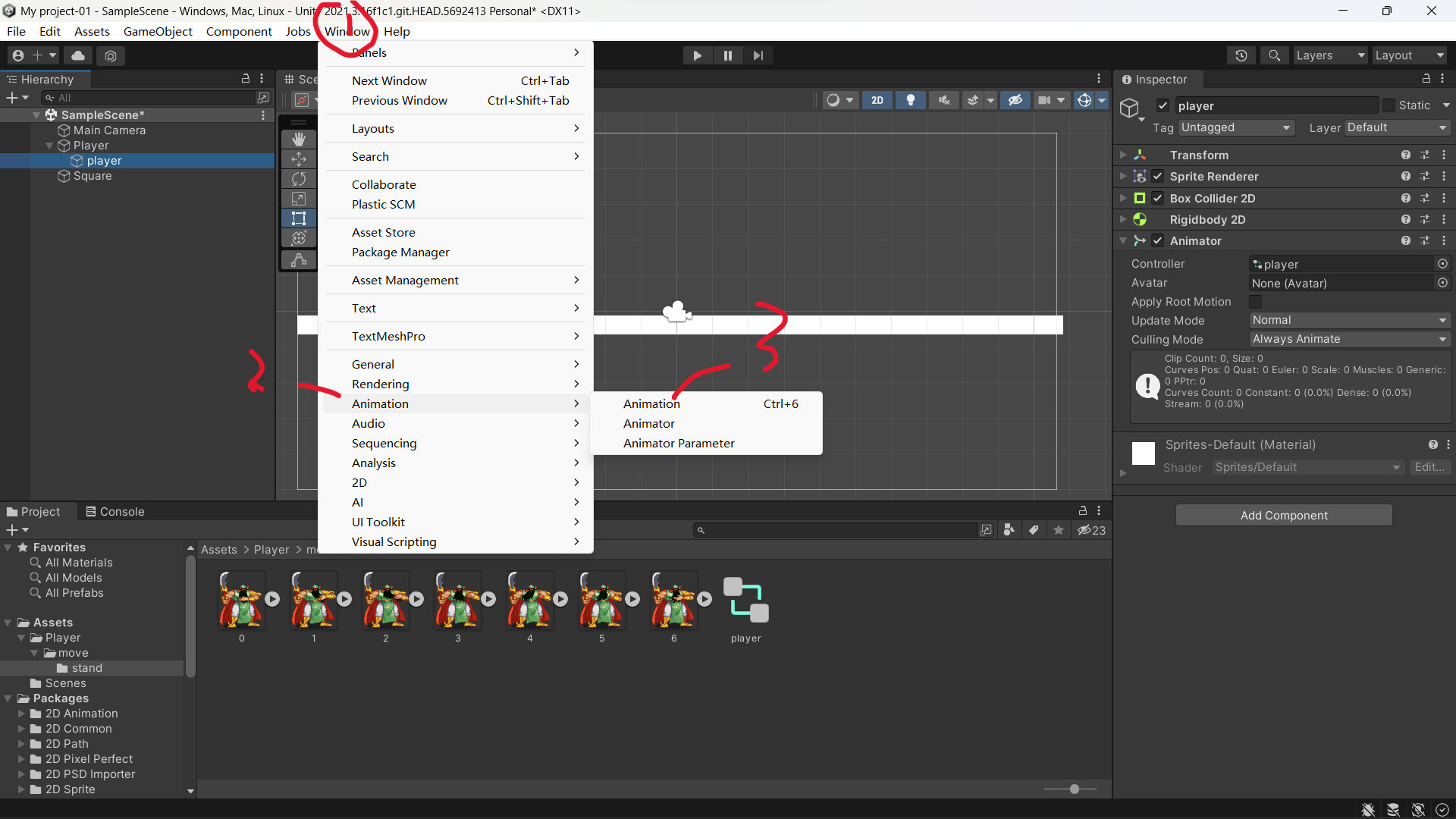
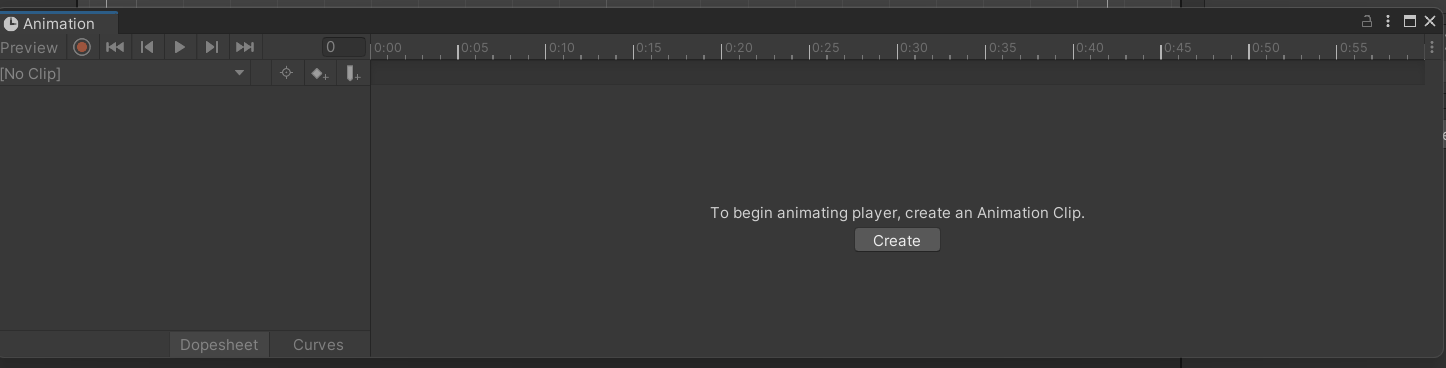
点击Creata创建动画,并将序列帧图片放入
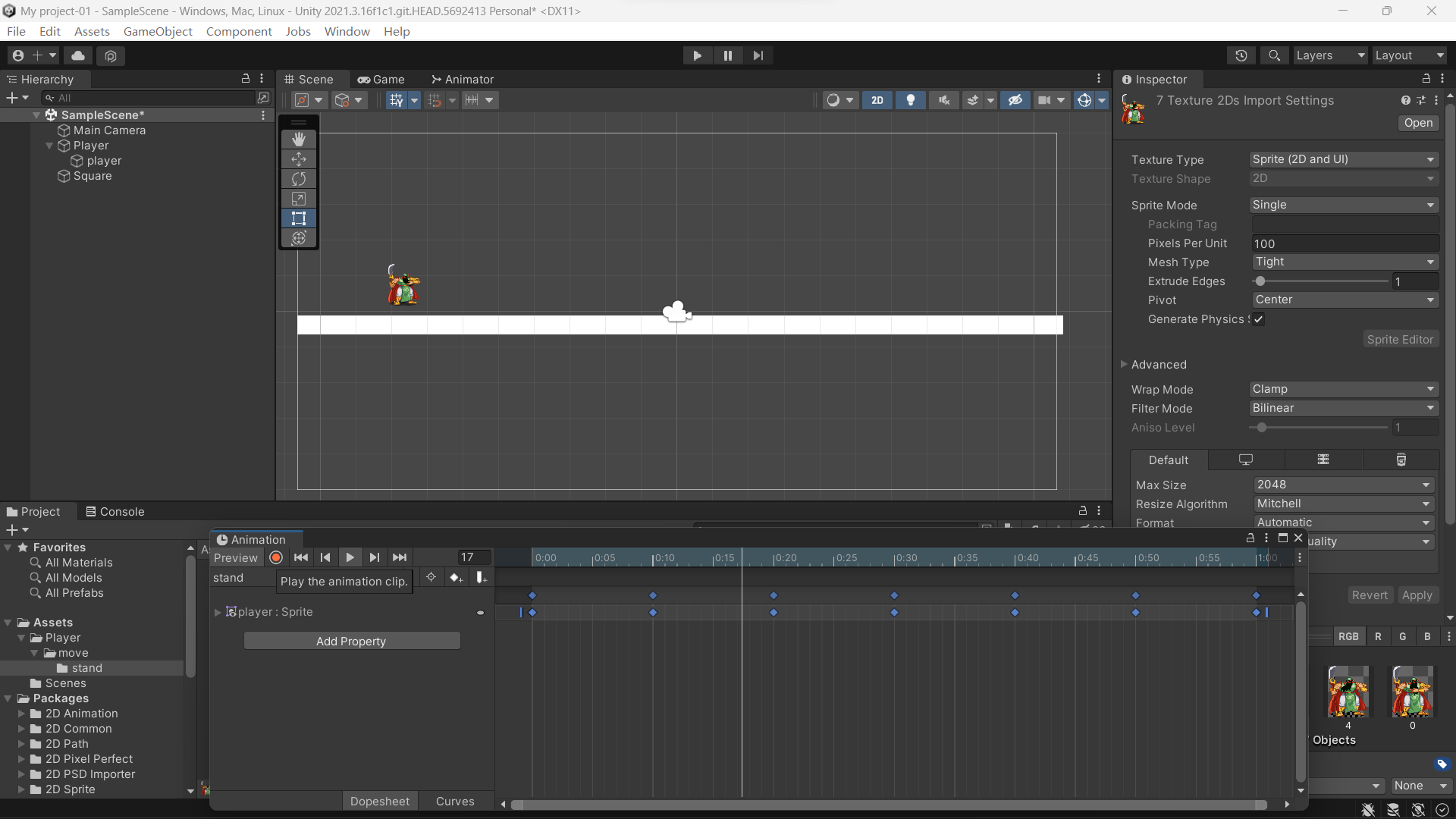
点击播放是可以预览的,并将Animatior Contorller托到人物对应位置上。
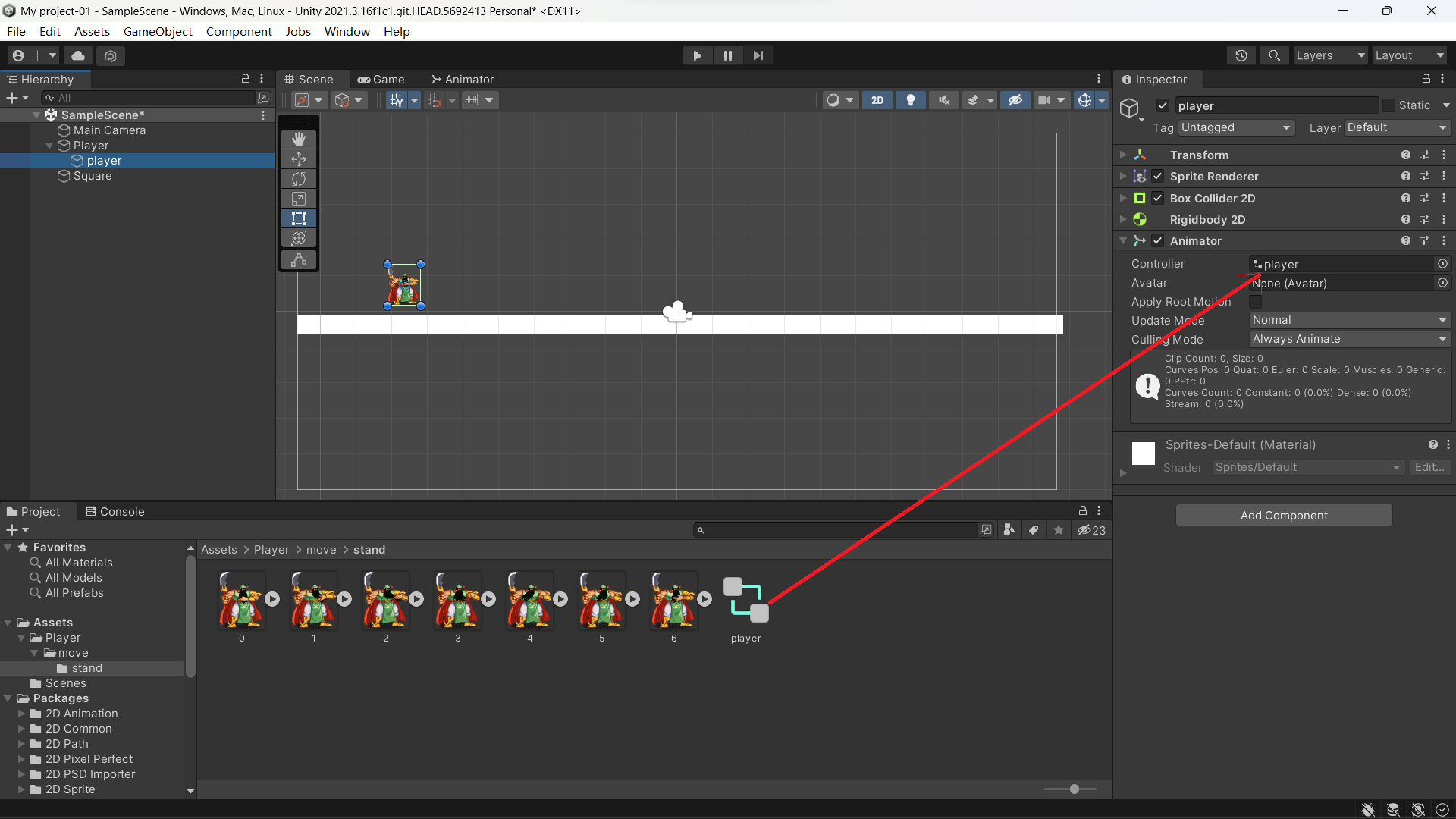
一个物体的Animatior Contorller是用来进行动画播放控制的文件,可以实现本物体的动画转换。
人物的移动
移动
通过物体水平方向上的位置来移动
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Move : MonoBehaviour
{
public Rigidbody2D rb;//获取刚体
public float speed = 1.0f;//速度
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody2D>();//自动获取unity的对应组件;
}
// Update is called once per frame
void Update()
{
move();
}
public void move()
{
//Horizontal是键盘上的上、下、左、右键
float mo = Input.GetAxis("Horizontal");//GetAxis 返回值为[-1,1]拥有平缓过度的功能
float x = Input.GetAxisRaw("Horizontal");//GetAxisRaw 返回值为-1 0 1,三种情况;
//移动
if(mo != 0)
{
rb.transform.position = new Vector2(transform.position.x + speed * mo *
Time.deltaTime, transform.position.y); //transform.position可以直接设置物体的位置
}
//转身
if (x != 0)
{
rb.transform.localScale = new Vector2(x, 1);
//transform.localScale可以设置物体的比例
}
}
}
通过水平方向上的速度来移动
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Move : MonoBehaviour
{
public Rigidbody2D rb;
public float speed = 1.0F;
public float jump = 1.0f;
// Start is called before the first frame update
void Start()
{
rb= GetComponent<Rigidbody2D>();
}
// Update is called once per frame
void Update()
{
move();
}
public void move()
{
float me = Input.GetAxis("Horizontal");//GetAxis 返回值为[-1,1]拥有平缓过度的功能
float x = Input.GetAxisRaw("Horizontal");//GetAxisRaw 返回值为-1 0 1,三种情况
if(me != 0)
{
rb.velocity = new Vector2(me * speed * Time.deltaTime, rb.velocity.y);
// velocity 为物体所拥有到的速度;
}
if(x != 0)
{
transform.localScale = new Vector3(x,1,1);
}
}
}
动画转换
先添加好人物的走路动画,然后设置Animatior Contorller
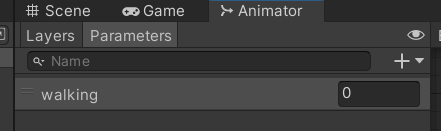
创建名为walking的float类型动画参数(也可以用bool类型)
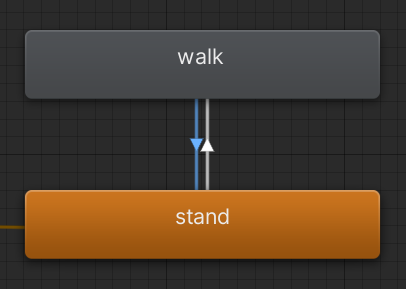
连接好切换的两个动画
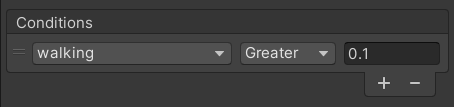
设置好切换条件,stand->walk 当速度大于0.1切换为walk动画
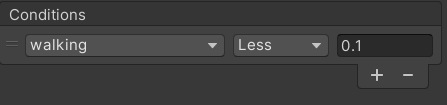
walk->walk 当速度小于0.1切换为stand动画
在代码中获取Animator组件去设置动画的播放参数
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class move : MonoBehaviour
{
public Rigidbody2D rb;
public Animator anim;
public float speed = 1000;
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody2D>();//自动获取对应组件
anim = GetComponent<Animator>();
}
// Update is called once per frame
void Update()
{
Move();
}
public void Move()
{
float movex = Input.GetAxis("Horizontal");
float mo = Input.GetAxisRaw("Horizontal");
if(movex != 0)
{
rb.velocity = new Vector2(speed * movex * Time.deltaTime, rb.velocity.y);
anim.SetFloat("walking", Mathf.Abs(mo)); //Set 设置这个动画的状态
//因为GetAxisRaw 只有-1 0 1,所以切换动画很迅速,而且只要不为0就会播放walk动画;
}
if(mo != 0)
{
rb.transform.localScale = new Vector3(mo, 1, 1);
}
}
}
由于刚体的特性,在松开方向键后物体仍会向前移动一小段(这里涉及到物理材质和材质间的摩擦力,后面再细讲)
跳跃
添加起跳动画和下落动画后给地面添加名为wall的图层(用来做碰撞检测)
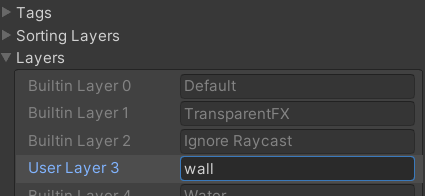
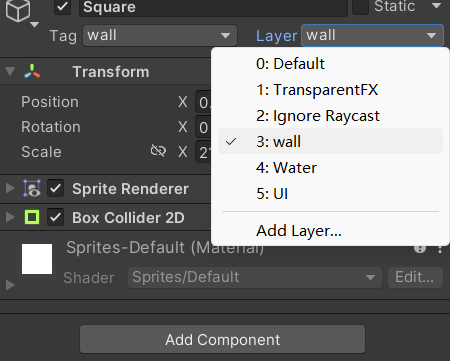
设置跳跃动画间的关系
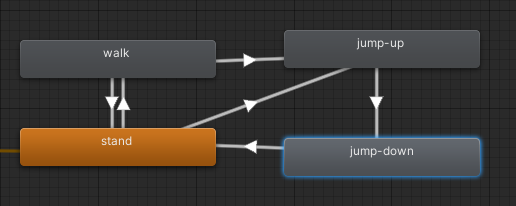
添加起跳和下落的动画参数
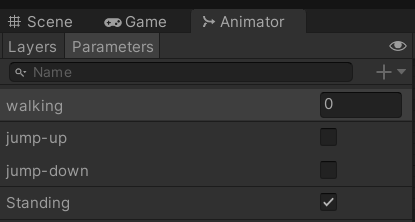
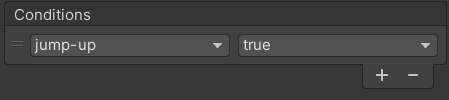
walk->jump-up在走路状态下可以跳跃

stand->jump-up在站立状态下可以跳跃
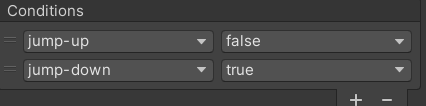
jump-up->jump-down该状态启用后起跳动画关闭,下落动画播放
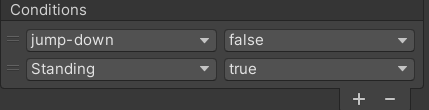
jump-down->stand同上
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class move : MonoBehaviour
{
public Rigidbody2D rb;
public Animator anim;
public float speed = 1000;
public float jump = 3;
public LayerMask wall; //获取地面标签
public Collider2D coll; // 获取碰撞体
// Start is called before the first frame update
void Start()
{
//自动获取对应组件(这类代码尽量少用,在unity拖动获取组件会更准确也会更便于修改)
rb = GetComponent<Rigidbody2D>();
anim = GetComponent<Animator>();
}
// Update is called once per frame
void Update()
{
Move();
SwitchAnim();
}
public void Move()
{
float movex = Input.GetAxis("Horizontal");
float mo = Input.GetAxisRaw("Horizontal");
if(movex != 0)
{
rb.velocity = new Vector2(speed * movex * Time.deltaTime, rb.velocity.y);
anim.SetFloat("walking", Mathf.Abs(mo));
}
if(mo != 0)
{
rb.transform.localScale = new Vector3(mo, 1, 1);
}
if (Input.GetButtonDown("Jump") && coll.IsTouchingLayers(wall))
//跳跃的改良,只有碰到地面才能起跳(不然连按空格会在空中起飞)
{
rb.velocity = new Vector2(rb.velocity.x, jump);
anim.SetBool("jump-up", true);
}
}
//动画转换
public void SwitchAnim()
{
if (anim.GetBool("jump-up") && rb.velocity.y < 0)//Get 获取动画状态
{
//当起跳动画正在播放时且y上的速度小于0,那么关闭起跳动画播放下落动画;
anim.SetBool("jump-up", false);
anim.SetBool("jump-down", true);
}
else if (coll.IsTouchingLayers(wall))// 当碰到地面时,将下落动画关闭
{
anim.SetBool("jump-down", false);
anim.SetBool("Standing", true);
}
}
}