链栈,自己实现一遍,但是节点存储不是整数,存储学生信息(年龄,分数,姓名)三级引用。
1、建立学生信息结构体,将data改为学生信息结构体类型。
2、循环入栈和入队。
代码展示
#include <myhead.h>
#define MAX 4
typedef struct{
int age;
float score;
char name[20];
}stu;
typedef struct node{
stu s;
struct node *next;
}Node;
typedef struct{
int len;
Node *top;
}Stack,*Pstack;
typedef struct{
Node *rear;
Node *front;
int len;
}Queue,*Pqueue;
Pstack creat_stack(){
Pstack p = malloc(sizeof(Stack));
if(p==NULL){
printf("申请空间失败!\n");
return NULL;
}
p->len = 0;
p->top = NULL;
return p;
}
Pqueue creat_queue(){
Pqueue p = malloc(sizeof(Queue));
if(p==NULL){
printf("申请空间失败!\n");
return NULL;
}
p->len = 0;
p->rear = NULL;
p->front = NULL;
return p;
}
int stack_insert(Pstack p,stu s){
if(p==NULL){
printf("链表不存在!\n");
return -1;
}
Node *t = malloc(sizeof(Node));
t->s.age = s.age;
t->s.score = s.score;
strcpy(t->s.name,s.name);
t->next = p->top;
p->top = t;
p->len++;
return 0;
}
int queue_insert(Pqueue p,stu s){
if(p==NULL){
printf("队列不存在!\n");
return -1;
}
Node *t = malloc(sizeof(Node));
t->s.age = s.age;
t->s.score = s.score;
strcpy(t->s.name,s.name);
if(p->rear==NULL){
p->rear = t;
p->front = t;
}else{
p->rear->next = t;
p->rear = t;
}
t->next = NULL;
p->len++;
return 0;
}
int stack_output(Pstack p){
if( p==NULL || p->len == 0 ){
printf("链表不存在或者为空!\n");
return -1;
}
printf("学生信息如下:\n");
Node *t = p->top;
for(int i=0;i<p->len;i++){
printf("年龄:%d\t成绩:%.2f\t姓名:%s\n",t->s.age,t->s.score,t->s.name);
t = t->next;
}
return 0;
}
int queue_output(Pqueue p){
if(p==NULL || p->len == 0 ){
printf("队列不存在或者为空!\n");
return -1;
}
Node *t = p->front;
printf("学生信息如下:\n");
for(int i=0;i<p->len;i++){
printf("年龄:%d\t成绩:%.2f\t姓名:%s\n",t->s.age,t->s.score,t->s.name);
t = t->next;
}
return 0;
}
int main(int argc, const char *argv[])
{
printf("链栈的输入输出:\n");
Pstack L = creat_stack();
stu s[MAX] = {{18,89,"王五"},
{20,90,"张三"},
{19,96,"李玲"},
{18,88,"赵柳"}};
for(int i=0;i<MAX;i++){
stack_insert(L,s[i]);
}
stack_output(L);
printf("链式队列的输入输出:\n");
Pqueue P = creat_queue();
for(int i=0;i<MAX;i++){
queue_insert(P,s[i]);
}
queue_output(P);
return 0;
}
运行结果
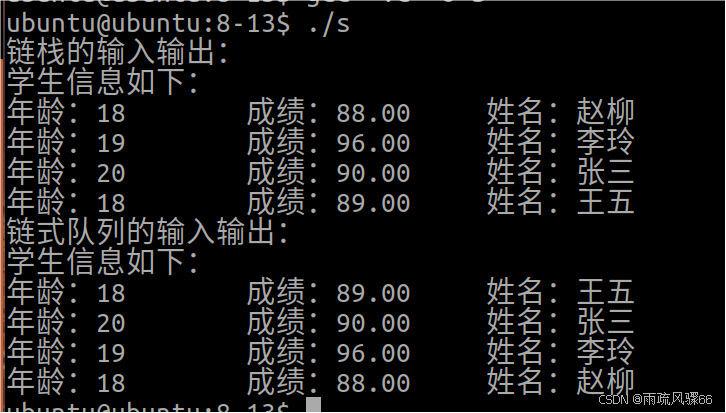