一、头文件
1.stack.h 栈头文件
#pragma once
#include <stdio.h>
#include <iostream>
#include "tree.h"
using namespace std;
#define MaxSize 128
typedef struct _SqStack
{
Bnode *base;
Bnode *top;
}SqStack;
bool InitStack(SqStack &S)
{
S.base = new Bnode[MaxSize];
if(!S.base)
return false;
S.top = S.base;
return true;
}
bool PushStack(SqStack &S,Bnode e)
{
if(S.top-S.base == MaxSize)
return false;
*(S.top++)=e;
return true;
}
bool PopStack(SqStack &S,Bnode &e)
{
if(S.base == S.top)
return false;
e = *(--S.top);
return true;
}
bool PopStack1(SqStack &S)
{
if(S.base == S.top)
return false;
S.top--;
return true;
}
Bnode* GetTop(SqStack &S)
{
if(S.top != S.base)
return S.top-1;
else
return NULL;
}
int GetSize(SqStack &S)
{
return (S.top-S.base);
}
bool IsEmpty(SqStack &S)
{
if(S.top == S.base)
return true;
else
return false;
}
void DestroyStack(SqStack &S)
{
if(S.base)
free(S.base);
S.base = NULL;
S.top = NULL;
}
2.tree.h 树头文件
#ifndef __TREE_H__
#define __TREE_H__
#define MAX_NODE 1024
#define isLess(a, b) (a<b)
#define isEqual(a, b) (a==b)
typedef int ElemType;
typedef struct _Bnode {
ElemType data;
struct _Bnode* lchild, * rchild;
}Bnode, Btree;
#endif
二、前中序遍历
1.前序遍历(递归)
void PreOrderRec(Btree* root)
{
if (root == NULL)
{
return;
}
printf("- %d -", root->data);
PreOrderRec(root->lchild);
PreOrderRec(root->rchild);
}
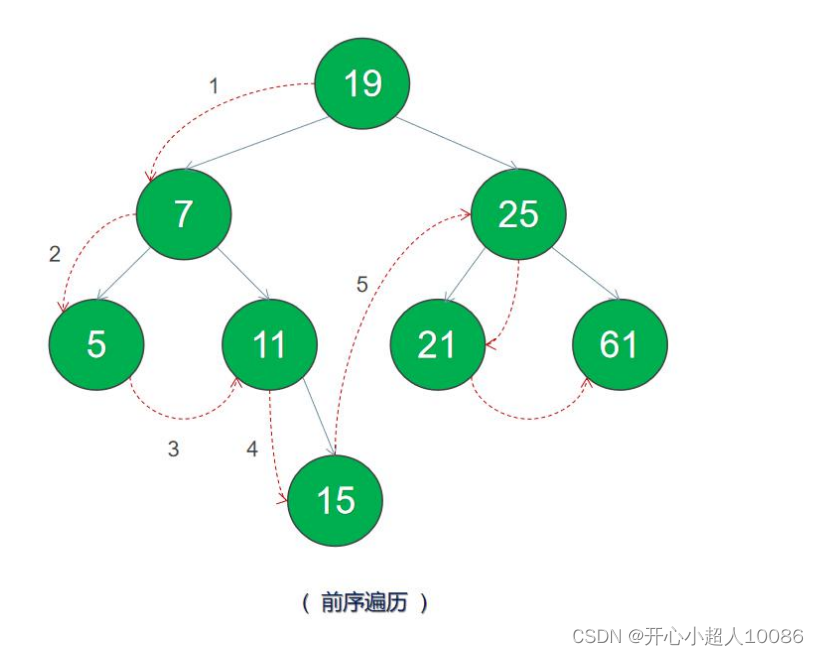
2.前序遍历----借助栈
void PreOrder(Btree* root)
{
Bnode cur;
if (root == NULL)
{
return;
}
SqStack stack;
InitStack(stack);
PushStack(stack, *root);
while (!(IsEmpty(stack)))
{
PopStack(stack, cur);
printf("- %d -", cur.data);
if (cur.rchild != NULL)
{
PushStack(stack, *(cur.rchild));
}
if (cur.lchild != NULL)
{
PushStack(stack, *(cur.lchild));
}
}
DestroyStack(stack);
}
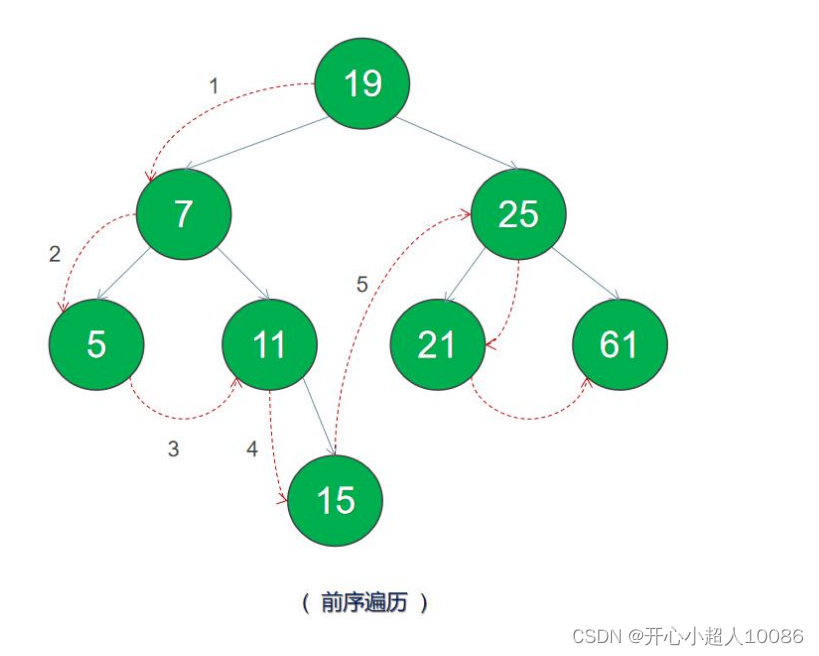
3.中序遍历(递归)
void PreOrderRec2(Btree *root)
{
if(root == NULL)
return ;
PreOrderRec2(root->lchild);
printf("-%d-",root->data);
PreOrderRec2(root->rchild);
}
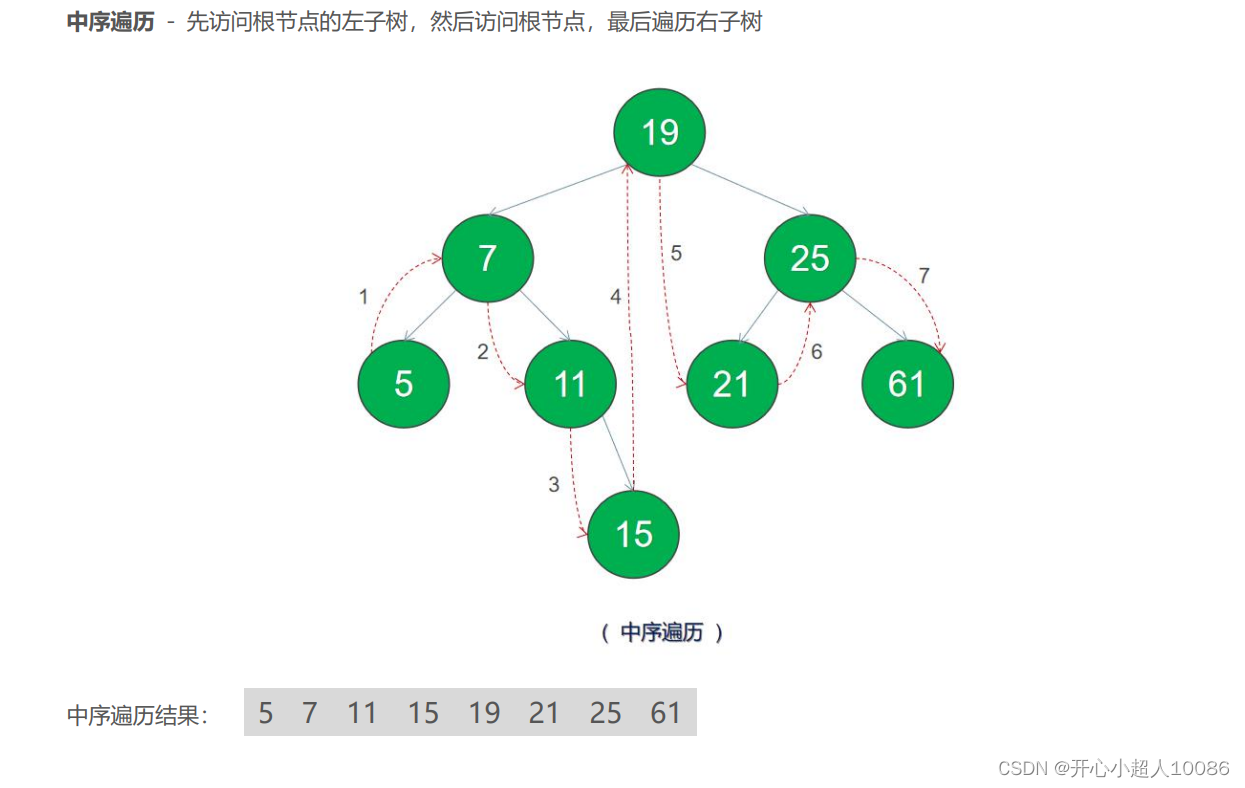
4.中序遍历----借助栈
void PreOrder2(Btree* root)
{
Bnode* cur = root;
Bnode cur1;
if(root == NULL)
return ;
SqStack stack;
InitStack(stack);
while(!(IsEmpty(stack))|| cur!=NULL)
{
while(cur!=NULL)
{
PushStack(stack,*cur);
cur = cur->lchild;
}
PopStack(stack,cur1);
printf("-%d-",cur1.data);
if(cur1.rchild!=NULL)
cur = cur1.rchild;
}
DestroyStack(stack);
}
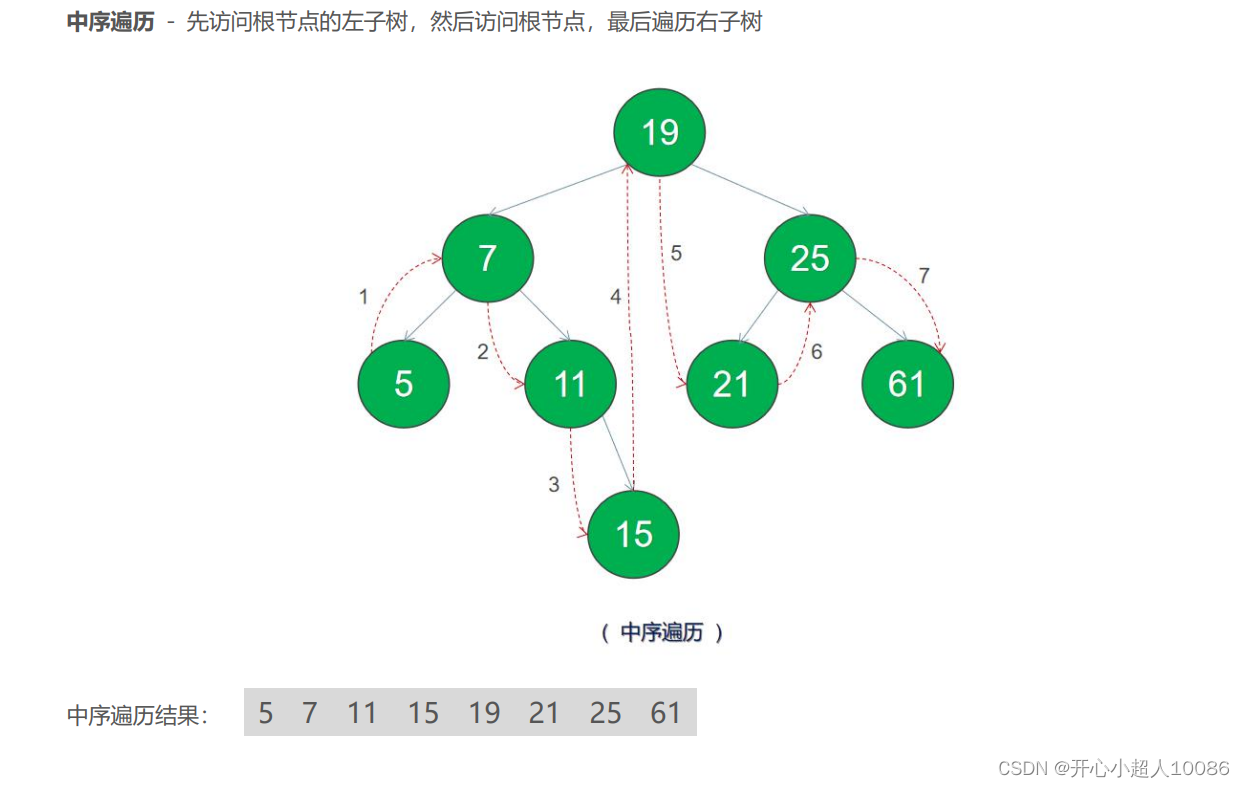
5.结点上树
bool InsertBtree(Btree** root, Bnode* node) {
Bnode* tmp = NULL;
Bnode* parent = NULL;
if (!node) {
return false;
}
else {
node->lchild = NULL;
node->rchild = NULL;
}
if (*root) {
tmp = *root;
}
else {
*root = node;
return true;
}
while (tmp != NULL) {
parent = tmp;
if (isLess(node->data, tmp->data)) {
tmp = tmp->lchild;
}
else {
tmp = tmp->rchild;
}
}
if (isLess(node->data, parent->data)) {
parent->lchild = node;
}
else {
parent->rchild = node;
}
return true;
}
6.删除树结点
Btree* DeleteNode(Btree* root, int key, Bnode*& deletedNode) {
if (root == NULL)return NULL;
if (root->data > key)
{
root->lchild = DeleteNode(root->lchild, key, deletedNode);
return root;
}
if (root->data < key)
{
root->rchild = DeleteNode(root->rchild, key, deletedNode);
return root;
}
deletedNode = root;
if (root->lchild == NULL && root->rchild == NULL)return NULL;
if (root->lchild == NULL && root->rchild != NULL)return root->rchild;
if (root->lchild != NULL && root->rchild == NULL)return root->lchild;
int val = findMax(root->lchild);
root->data = val;
root->lchild = DeleteNode(root->lchild, val, deletedNode);
return root;
}
7.1查找结点(递归)
Bnode* QueryByRec(Btree* root, ElemType e) {
if (isEqual(root->data, e) || NULL == root) {
return root;
}
else if (isLess(e, root->data)) {
return QueryByRec(root->lchild, e);
}
else {
return QueryByRec(root->rchild, e);
}
}
7.2查找结点(非递归)
Bnode* QueryByLoop(Bnode* root, int e) {
while (NULL != root && !isEqual(root->data, e)) {
if (isLess(e, root->data)) {
root = root->lchild;
}
else {
root = root->rchild;
}
}
return root;
}
8.入口函数
int main(void)
{
int test[]={19, 7, 25, 5, 11, 15, 21, 61};
Bnode * root=NULL, *node =NULL;
node = new Bnode;
node->data = test[0];
InsertBtree(&root, node);
for(int i=1;i<sizeof(test)/sizeof(test[0]);i++)
{
node = new Bnode;
node->data = test[i];
if(InsertBtree(&root, node))
{
printf("节点 %d 插入成功\n", node->data);
}
}
printf("前序遍历结果: \n");
PreOrder(root);
printf("\n");
system("pause");
printf("删除节点 15\n");
Bnode *deletedNode = NULL;
root = DeleteNode(root, 15, deletedNode);
printf("二叉搜索树删除节点 15, %s\n", deletedNode?"删除成功":"删除不成功,节点不存在");
if(deletedNode) delete deletedNode;
printf("删除后,再次前序遍历结果: \n");
PreOrder2(root);
printf("\n");
Bnode * node1 = QueryByLoop(root, 20);
printf("搜索二叉搜索树,节点 20 %s\n", node1?"存在":"不存在");
Bnode * node2 = QueryByLoop(root, 21);
printf("搜索二叉搜索树,节点 21 %s\n", node2?"存在":"不存在");
system("pause");
return 0;
}
9.测试结果
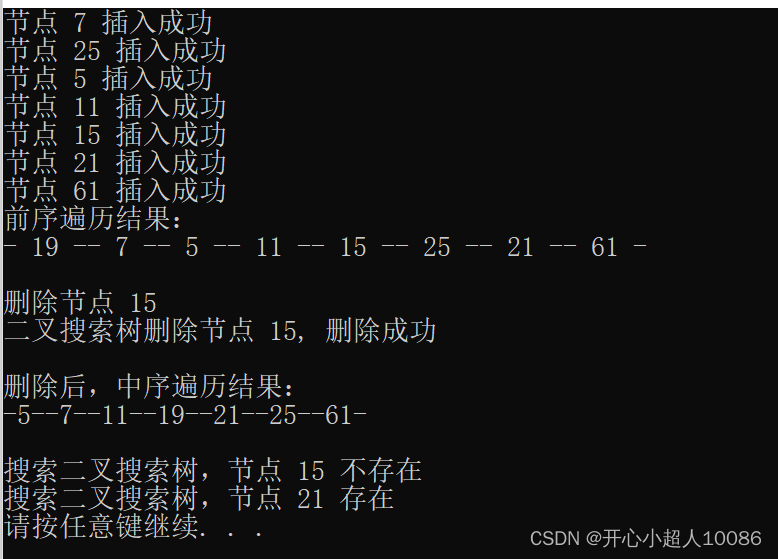