赋值运算符重载:
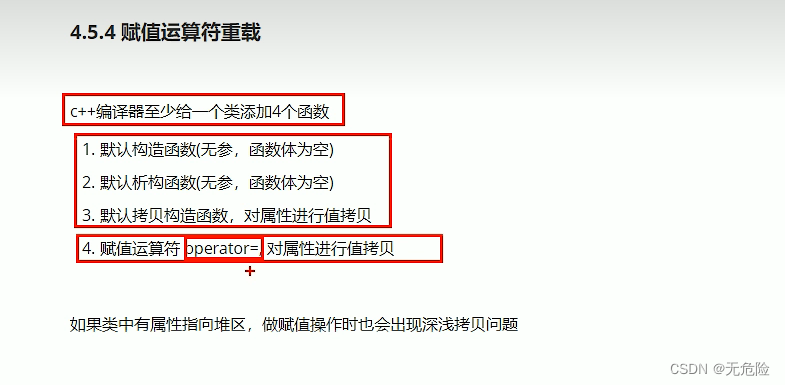
浅克隆导致堆区内存重复释放,程序崩溃
#include<iostream>
#include<string>
using namespace std;
class Person
{
public:
Person()
{
}
Person(int age)
{
m_Age=new int(age);
}
~Person()
{
if (m_Age != NULL)
{
delete m_Age;
m_Age = NULL;
}
}
int *m_Age;
};
void test01()
{
Person p(19);
Person p1(20);
p1 = p;
cout << "p年龄为" <<* p.m_Age << endl;
cout << "p1年龄为" << *p1.m_Age << endl;
}
int main()
{
test01();
}
可以用 深克隆解决
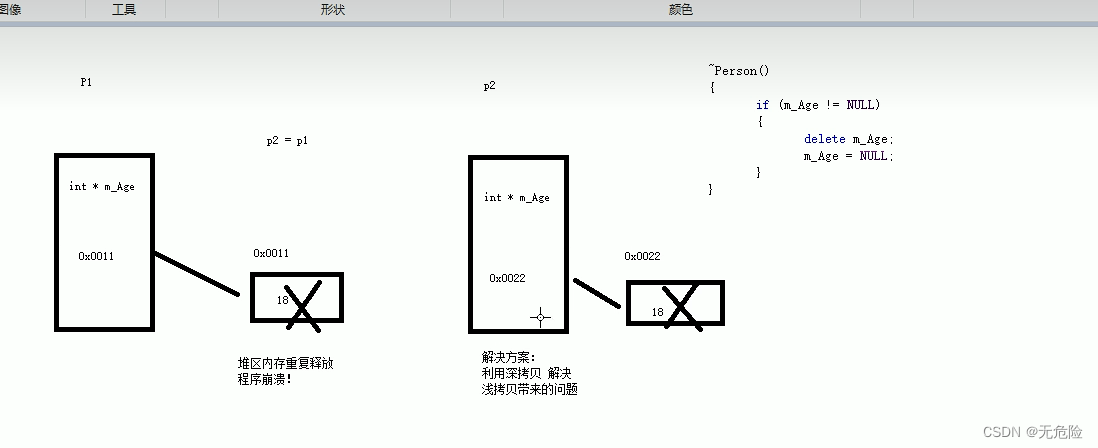
#include<iostream>
#include<string>
using namespace std;
class Person
{
public:
Person()
{
}
Person(int age)
{
m_Age=new int(age);
}
~Person()
{//释放堆区
if (m_Age != NULL)
{
delete m_Age;
m_Age = NULL;
}
}
Person& operator=(Person& p)
{
//编译器只提供浅拷贝
//m_Age=p.m_Age;
//应该判断是否有属性在堆区,如果有先释放干净,在深拷贝
if (m_Age != NULL)
{
delete m_Age;
m_Age = NULL;
}
//深拷贝
m_Age = new int(*p.m_Age);
//返回对象本身
return *this;
}
int *m_Age;
};
void test01()
{
Person p0(19);
Person p1(20);
Person p2(5);
p2 = p1 = p0;
cout << "p0年龄为" <<* p0.m_Age << endl;
cout << "p1年龄为" << *p1.m_Age << endl;
cout << "p3年龄为" << *p2.m_Age << endl;
}
int main()
{
test01();
}
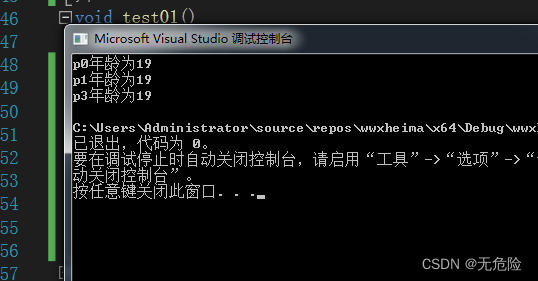