using System;
using System.Collections.Generic;
using System.IO;
class DijkstraAlgorithm
{
private int numVertices;
private int[,] graph;
private int[] distance;
private bool[] shortestPathTreeSet;
public DijkstraAlgorithm(int numVertices)
{
this.numVertices = numVertices;
graph = new int[numVertices, numVertices];
distance = new int[numVertices];
shortestPathTreeSet = new bool[numVertices];
}
public void ReadGraphFromFile(string filePath)
{
string[] lines = File.ReadAllLines(filePath);
foreach (string line in lines)
{
string[] values = line.Split(' ');
int source = int.Parse(values[0]);
int destination = int.Parse(values[1]);
int weight = int.Parse(values[2]);
graph[source, destination] = weight;
}
}
public void FindShortestPath(int source)
{
for (int i = 0; i < numVertices; i++)
{
distance[i] = int.MaxValue;
shortestPathTreeSet[i] = false;
}
distance[source] = 0;
for (int count = 0; count < numVertices - 1; count++)
{
int u = MinimumDistance(distance, shortestPathTreeSet);
shortestPathTreeSet[u] = true;
for (int v = 0; v < numVertices; v++)
{
if (!shortestPathTreeSet[v] && graph[u, v] != 0 && distance[u] != int.MaxValue &&
distance[u] + graph[u, v] < distance[v])
{
distance[v] = distance[u] + graph[u, v];
}
}
}
PrintSolution(distance, source);
}
private int MinimumDistance(int[] distance, bool[] shortestPathTreeSet)
{
int min = int.MaxValue, minIndex = -1;
for (int v = 0; v < numVertices; v++)
{
if (!shortestPathTreeSet[v] && distance[v] <= min)
{
min = distance[v];
minIndex = v;
}
}
return minIndex;
}
private void PrintSolution(int[] distance, int source)
{
Console.WriteLine("Shortest Path from {0}:", source);
Console.WriteLine("Vertex \t Distance \t Path");
for (int v = 0; v < numVertices; v++)
{
if (distance[v] == int.MaxValue)
{
Console.WriteLine(v + " \t\t NULL \t\t NULL");
}
else
{
Console.WriteLine(v + " \t\t " + distance[v] + " \t\t " + GetPath(v));
}
}
}
private string GetPath(int vertex)
{
string path = vertex.ToString();
int currentVertex = vertex;
while (currentVertex != 0)
{
for (int v = 0; v < numVertices; v++)
{
if (graph[v, currentVertex] != 0 && distance[v] + graph[v, currentVertex] == distance[currentVertex])
{
path = v + " -> " + path;
currentVertex = v;
break;
}
}
}
return path;
}
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the number of vertices:");
int numVertices = int.Parse(Console.ReadLine());
DijkstraAlgorithm dijkstra = new DijkstraAlgorithm(numVertices);
Console.WriteLine("Enter the file path of the graph data:");
string filePath = Console.ReadLine();
dijkstra.ReadGraphFromFile(filePath);
Console.WriteLine("Enter the source vertex:");
int source = int.Parse(Console.ReadLine());
dijkstra.FindShortestPath(source);
}
}
GIS算法——C#迪杰斯特拉算法
于 2023-12-23 16:12:17 首次发布
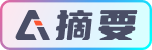