1.大纲
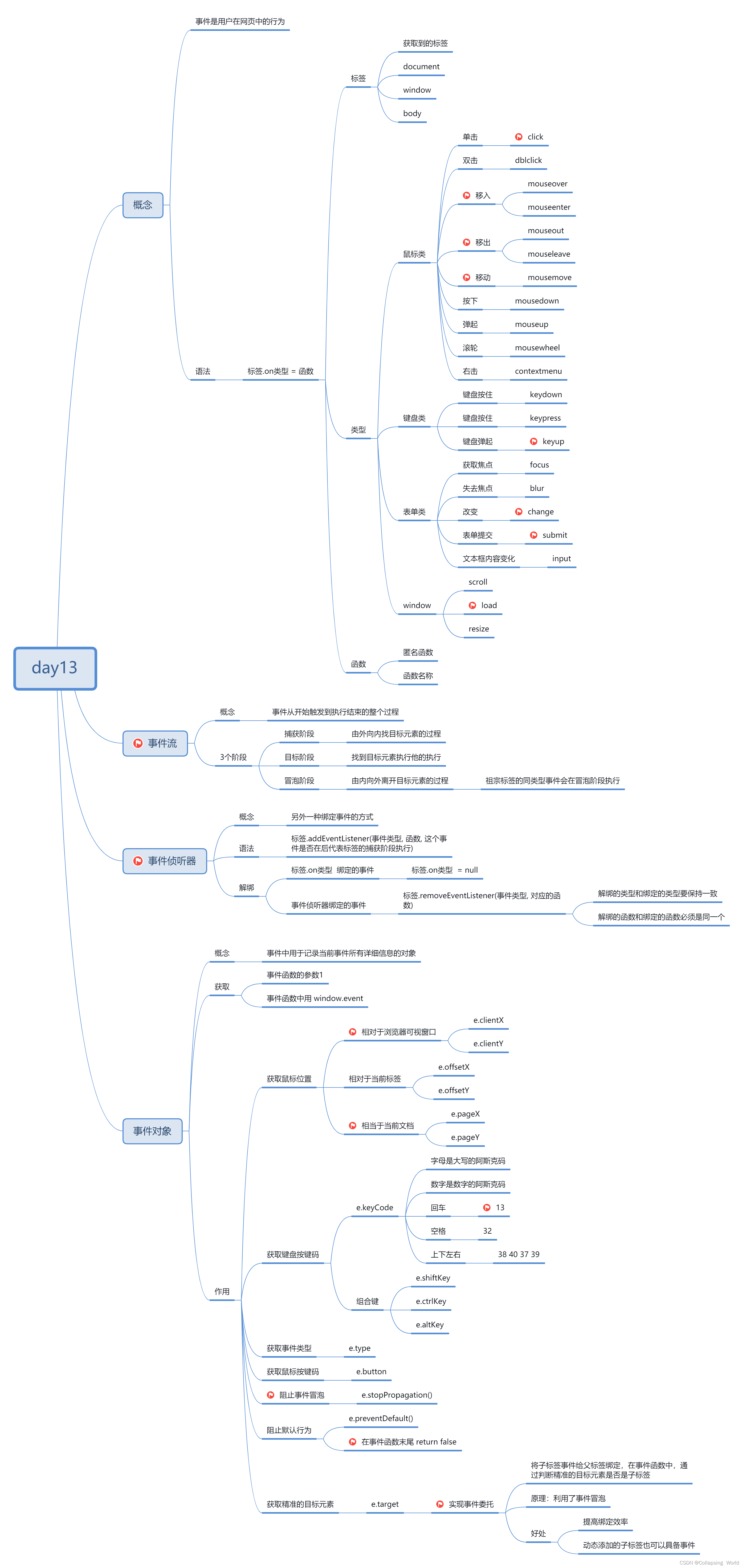
2.QQ菜单展开和折叠
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<link rel="stylesheet" href="./font/iconfont.css">
<style>
ul,ol{
list-style: none;
}
ul,ol,h2{
padding: 0;
margin: 0;
}
h2{
font-size: 18px;
line-height: 28px;
font-weight: normal;
}
h2 i.iconfont{
font-size: 12px;
position: relative;
top: -2px;
}
ul{
width: 200px;
border: 1px solid #ccc;
padding-left: 20px;
box-sizing: border-box;
}
ol li{
padding-left: 26px;
line-height: 30px;
}
ol{
display: none;
}
li{
cursor: pointer;
}
</style>
</head>
<body>
<ul>
<li>
<h2>
<i class="iconfont icon-xiangyoujiantou"></i>
<span>我的好友</span>
</h2>
<ol>
<li>张三</li>
<li>李四</li>
<li>王五</li>
</ol>
</li>
<li>
<h2>
<i class="iconfont icon-xiangyoujiantou"></i>
<span>我的同学</span>
</h2>
<ol>
<li>张大三</li>
<li>李大四</li>
<li>王大五</li>
</ol>
</li>
<li>
<h2>
<i class="iconfont icon-xiangyoujiantou"></i>
<span>黑名单</span>
</h2>
<ol>
<li>张小三</li>
<li>李小四</li>
<li>王小五</li>
</ol>
</li>
</ul>
</body>
<script>
// 行为:点击h2标签
// 效果:让对应的ol显示
var h2s = document.querySelectorAll('h2')
// 定义变量 - 假设当前是展开状态
var isOpen = false
// 遍历
for (var a = 0; a < h2s.length; a++) {
// 添加事件
h2s[a].onclick = function() {
// 判断自己是展开还是收回
// if (this.firstElementChild.className === 'iconfont icon-xiajiantou') {
// if (getComputedStyle(this.nextElementSibling).display === 'block') {
if (isOpen) {
// 自己是展开状态 - 自己就要收回
for (var b = 0; b < h2s.length; b++) {
h2s[b].nextElementSibling.style.display = 'none'
h2s[b].firstElementChild.className = 'iconfont icon-xiangyoujiantou'
}
isOpen = false
} else { // 自己是收回状态
// 将所有的都收回
for (var b = 0; b < h2s.length; b++) {
h2s[b].nextElementSibling.style.display = 'none'
h2s[b].firstElementChild.className = 'iconfont icon-xiangyoujiantou'
}
// 让自己的下一个标签显示
this.nextElementSibling.style.display = 'block'
// 让自己的第一个子标签切换类名
this.firstElementChild.className = 'iconfont icon-xiajiantou'
isOpen = true
}
}
}
// 获取所有ol中的li
var lis = document.querySelectorAll('ol li')
for (var a = 0; a < lis.length; a++) {
lis[a].onclick = function() {
for (var b = 0; b < lis.length; b++) {
lis[b].style.backgroundColor = 'transparent'
}
this.style.backgroundColor = '#eee'
}
}
</script>
</html>
3.秒表
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div class="box">
<span class="minute">00</span>
<b>:</b>
<span class="second">00</span>
<b>.</b>
<span class="millisecond">00</span>
</div>
<button>重置</button>
<button>开始</button>
</body>
<script>
// 获取标签
var minuteSpan = document.querySelector('.minute')
var secondSpan = document.querySelector('.second')
var millisecondSpan = document.querySelector('.millisecond')
var resetBtn = document.querySelector('button')
var startBtn = document.querySelector('button+button')
// 开始按钮点击
var timer
var isZanting = true
startBtn.onclick = function() {
if (this.innerText === '开始' || this.innerText === '继续') {
isZanting = false
// 更换按钮文字
this.innerText = '暂停'
// 让毫秒开始跑起来
timer = setInterval(function() {
// 获取毫秒数字
var num = millisecondSpan.innerText - 0
// 数字自增
num++
// 限制最大值
if (num === 100) {
num = 0
// 让秒自增
var second = secondSpan.innerText - 0
second++
if (second === 60) {
second = 0
var minute = minuteSpan.innerText - 0
minuteSpan.innerText = minute < 10 ? '0' + ++minute : ++minute
}
secondSpan.innerText = second < 10 ? '0' + second : '' + second
}
if (num < 10) {
num = '0' + num
} else {
num = '' + num
}
// 设置数字到标签中
millisecondSpan.innerText = num
}, 10)
}
else {
clearInterval(timer)
this.innerText = '继续'
isZanting = true
}
}
resetBtn.onclick = function() {
if (isZanting) {
clearInterval(timer)
startBtn.innerText = '开始'
minuteSpan.innerText = secondSpan.innerText = millisecondSpan.innerText = '00'
}
}
</script>
</html>
4.星星评分
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<img src="./images/rank_3.gif" alt=""><img src="./images/rank_4.gif" alt="">
<img src="./images/rank_3.gif" alt=""><img src="./images/rank_4.gif" alt="">
<img src="./images/rank_3.gif" alt=""><img src="./images/rank_4.gif" alt="">
<img src="./images/rank_3.gif" alt=""><img src="./images/rank_4.gif" alt="">
<img src="./images/rank_3.gif" alt=""><img src="./images/rank_4.gif" alt="">
<hr>
<div>阿斯顿福建哈市劳动法哈开始累加大恒科技</div>
</body>
<script>
var imgs = document.querySelectorAll('img')
for (var a = 0; a < imgs.length; a++) {
(function(a) {
imgs[a].onmouseover = function() {
// 获取当前img的下标
// console.log(a);
// 循环前面所有的img
for (var b = 0; b <= a; b++) {
if (b % 2 === 0) {
imgs[b].setAttribute('src', './images/rank_1.gif')
} else {
imgs[b].setAttribute('src', './images/rank_2.gif')
}
}
// 循环后面所有的img
for (var b = a + 1; b < imgs.length; b++) {
if (b % 2 === 0) {
imgs[b].setAttribute('src', './images/rank_3.gif')
} else {
imgs[b].setAttribute('src', './images/rank_4.gif')
}
}
}
})(a)
}
</script>
</html>
5.全选反选案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div>
<input type="checkbox">全选
<input type="checkbox">全不选
<input type="checkbox">反选
</div>
<hr>
<p>
<input type="checkbox">吃饭
<input type="checkbox">睡觉
<input type="checkbox">做作业
<input type="checkbox">写代码
<input type="checkbox">打豆豆
<input type="checkbox">玩游戏
<input type="checkbox">听音乐
<input type="checkbox">谈恋爱
</p>
</body>
<script>
var btns = document.querySelectorAll('div input')
console.log(btns);
var ipts = document.querySelectorAll('p input')
btns[0].onchange = function() {
// 判断自己是否是选中
// console.log( this.checked );
if (this.checked) {
// 让一个复选框选中,就将他的checked赋值为true
for (var a = 0; a < ipts.length; a++) {
console.log(666);
ipts[a].checked = true
}
}
}
btns[1].onchange = function() {
if (this.checked) {
// 让一个复选框选中,就将他的checked赋值为true
for (var a = 0; a < ipts.length; a++) {
ipts[a].checked = false
}
}
}
btns[2].onchange = function() {
for (var a = 0; a < ipts.length; a++) {
ipts[a].checked = !ipts[a].checked
}
}
</script>
</html>
6.拖拽移动案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<style>
.box{
width: 100px;
height: 100px;
background-color: #00f;
position: absolute;
left: 0;
top: 0;
}
</style>
<body>
<div class="box"></div>
<div style="height: 2000px;"></div>
</body>
<script>
// 拖拽 = 按下事件配合移动事件
var box = document.querySelector('.box')
box.onmousedown = function(e) {
var x1 = e.offsetX
var y1 = e.offsetY
// 嵌套一个鼠标移动事件
document.onmousemove = function(e) {
// 让div重新计算left和top
// 移动过程中,需要获取鼠标在浏览器中坐标
var x = e.clientX
var y = e.clientY
// div的left = 鼠标位置 - div宽度 / 2
// var divLeft = x - box.offsetWidth / 2
// var divTop = y - box.offsetHeight/ 2
var divLeft = x - x1
var divTop = y - y1
// 限制left和top的最小值和最大值
if (divLeft < 0) {
divLeft = 0
}
if (divTop < 0) {
divTop = 0
}
if (divLeft > document.documentElement.clientWidth - box.offsetWidth) {
divLeft = document.documentElement.clientWidth - box.offsetWidth
}
if (divTop > document.documentElement.clientHeight - box.offsetHeight) {
divTop = document.documentElement.clientHeight - box.offsetHeight
}
// 设置div的left和top样式
box.style.left = divLeft + 'px'
box.style.top = divTop + 'px'
}
}
// 松开鼠标 - 解绑移动事件
window.onmouseup = function() {
document.onmousemove = null
}
</script>
</html>