lesson5画表情包
1.课堂笔记
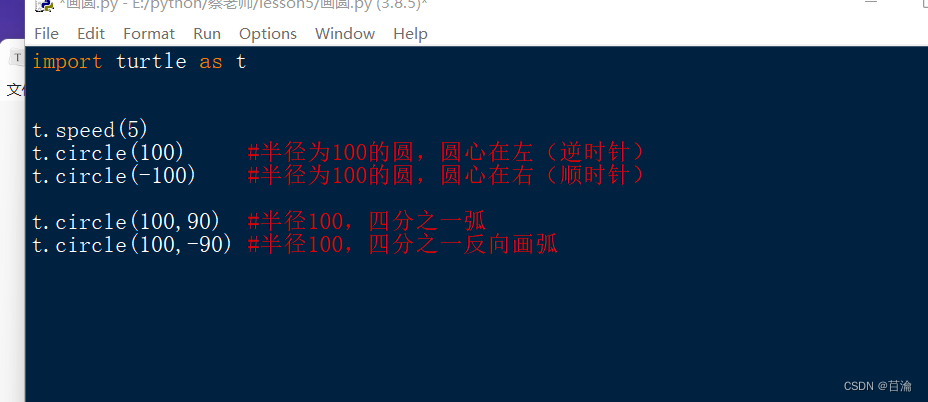
2.知识点
t.setheading(0)
t.circle(200)
t.circle(a,b)
3.课后作业
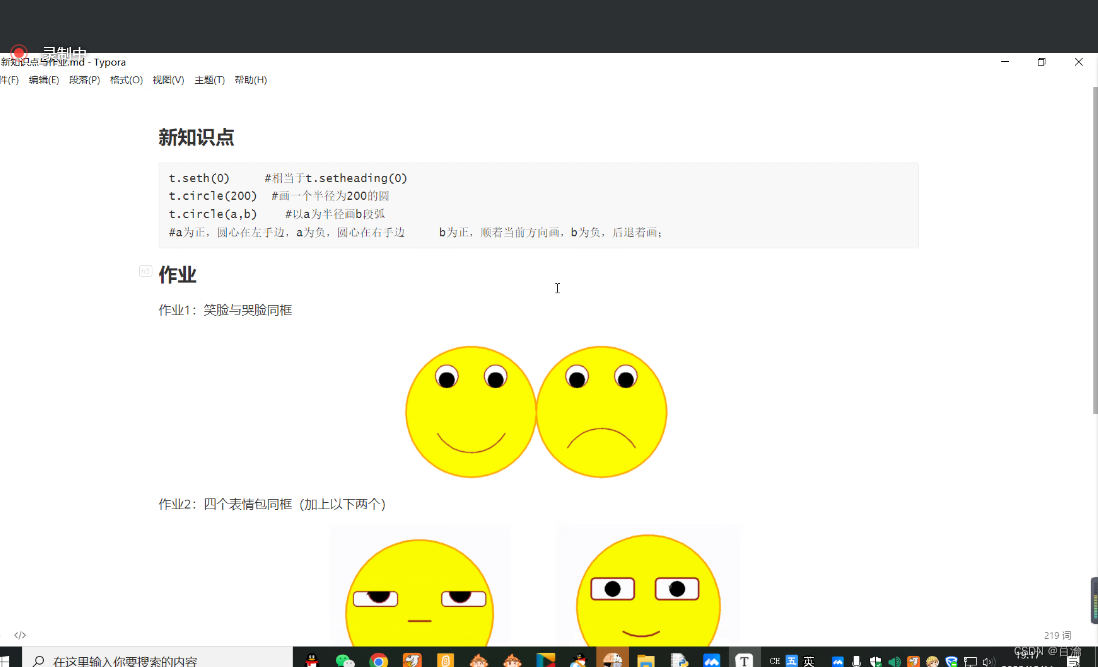
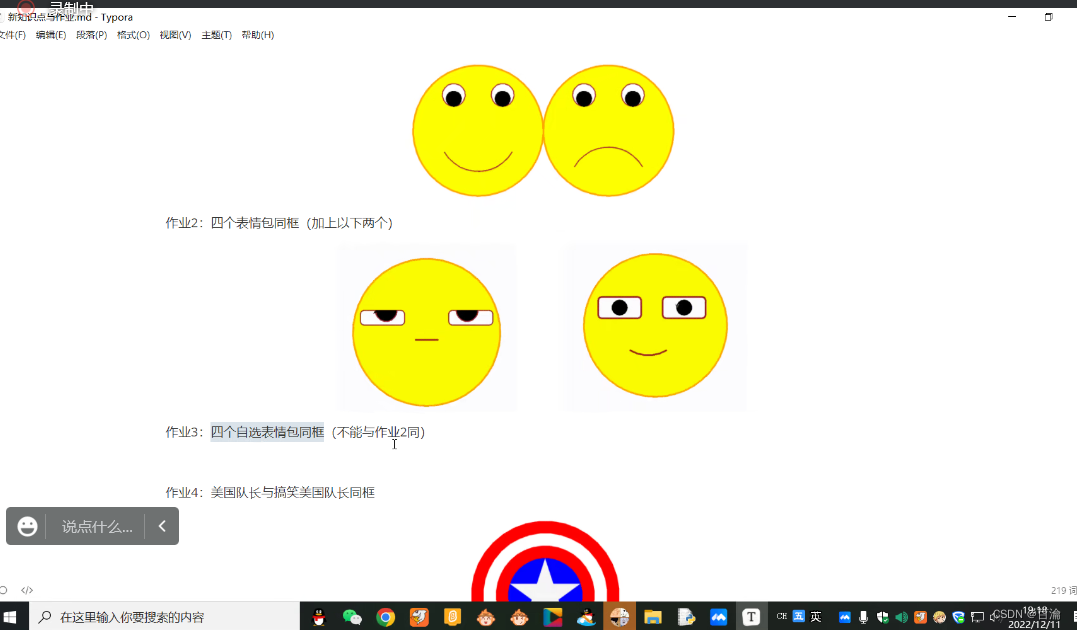
1.作业一(笑脸,哭脸)
import turtle as t
def face(x,y):
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("orange","yellow")
t.pensize(2)
t.begin_fill()
t.circle(200)
t.end_fill()
def eye(x,y):
t.pensize(3)
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("brown","white")
t.begin_fill()
t.circle(40)
t.end_fill()
t.color("black","black")
t.begin_fill()
t.circle(25)
t.end_fill()
def mouth(x,y,z):
if z == 0:
t.pensize(3)
t.setheading(-60)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(100,120)
if z == 1:
t.pensize(3)
t.setheading(-120)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(100,-120)
def all_face(x,y,z):
face(x,y)
eye(x-80,y+250)
eye(x+80,y+250)
mouth(x-80,y+110,z)
t.hideturtle()
t.speed(0)
all_face(-200,0,0)
all_face(-200,-400,1)
all_face(200,0,1)
all_face(200,-400,0)
'''
face(200,-200)
face(-200,-200)
eye(120,50)
eye(280,50)
eye(-120,50)
eye(-280,50)
mouth(120,-90,1)
mouth(-285,-80,0)
'''
2.作业二(四个表情包)
import turtle as t
def face(x,y):
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("orange","yellow")
t.pensize(2)
t.begin_fill()
t.circle(200)
t.end_fill()
def eye(x,y):
t.pensize(3)
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("brown","white")
t.begin_fill()
t.circle(40)
t.end_fill()
t.color("black","black")
t.begin_fill()
t.circle(25)
t.end_fill()
def mouth(x,y,z):
if z == 0:
t.pensize(3)
t.setheading(-60)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(100,120)
if z == 1:
t.pensize(3)
t.setheading(-120)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(100,-120)
def eye_2(x,y):
t.pensize(3)
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("brown","white")
t.begin_fill()
for i in range(2):
t.forward(100)
t.circle(7,90)
t.forward(50)
t.circle(7,90)
t.end_fill()
t.penup()
t.goto(x+45,y+10)
t.pendown()
t.color("black","black")
t.begin_fill()
t.circle(20)
t.end_fill()
def mouth_2(x,y):
t.setheading(-30)
t.pensize(3)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(100,60)
def eye_3(x,y):
t.pensize(3)
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("brown","white")
t.begin_fill()
for i in range(2):
t.forward(110)
t.circle(7,90)
t.forward(30)
t.circle(7,90)
t.end_fill()
t.penup()
t.goto(x+35,y+45)
t.pendown()
t.setheading(-90)
t.color("black","black")
t.begin_fill()
t.circle(20,180)
t.end_fill()
def mouth_3(x,y):
t.pensize(3)
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.forward(100)
def all_face(x,y,z):
face(x,y)
eye(x-80,y+250)
eye(x+80,y+250)
mouth(x-80,y+110,z)
def all_face_2(x,y):
face(x,y)
eye_2(x-140,y+250)
eye_2(x+50,y+250)
mouth_2(x-45,y+80)
def all_face_3(x,y):
face(x,y)
eye_3(x-140,y+250)
eye_3(x+50,y+250)
mouth_3(x-45,y+80)
t.hideturtle()
t.speed(0)
all_face_3(-200,0,)
all_face(-200,-400,0)
all_face(200,0,1)
all_face_2(200,-400,)
3.作业三(小猪佩奇)
import turtle as t
t.pensize(4)
t.hideturtle()
t.colormode(255)
t.color((255,155,192),"pink")
t.setup(840,500)
t.speed(0)
t.pu()
t.goto(-100,100)
t.pd()
t.seth(-30)
t.begin_fill()
a=0.4
for i in range(120):
if 0<=i<30 or 60<=i<90:
a=a+0.08
t.lt(3)
t.fd(a)
else:
a=a-0.08
t.lt(3)
t.fd(a)
t.end_fill()
t.pu()
t.seth(90)
t.fd(25)
t.seth(0)
t.fd(10)
t.pd()
t.pencolor(255,155,192)
t.seth(10)
t.begin_fill()
t.circle(5)
t.color(160,82,45)
t.end_fill()
t.pu()
t.seth(0)
t.fd(20)
t.pd()
t.pencolor(255,155,192)
t.seth(10)
t.begin_fill()
t.circle(5)
t.color(160,82,45)
t.end_fill()
t.color((255,155,192),"pink")
t.pu()
t.seth(90)
t.fd(41)
t.seth(0)
t.fd(0)
t.pd()
t.begin_fill()
t.seth(180)
t.circle(300,-30)
t.circle(100,-60)
t.circle(80,-100)
t.circle(150,-20)
t.circle(60,-95)
t.seth(161)
t.circle(-300,15)
t.pu()
t.goto(-100,100)
t.pd()
t.seth(-30)
a=0.4
for i in range(60):
if 0<=i<30 or 60<=i<90:
a=a+0.08
t.lt(3)
t.fd(a)
else:
a=a-0.08
t.lt(3)
t.fd(a)
t.end_fill()
t.color((255,155,192),"pink")
t.pu()
t.seth(90)
t.fd(-7)
t.seth(0)
t.fd(70)
t.pd()
t.begin_fill()
t.seth(100)
t.circle(-50,50)
t.circle(-10,120)
t.circle(-50,54)
t.end_fill()
t.pu()
t.seth(90)
t.fd(-12)
t.seth(0)
t.fd(30)
t.pd()
t.begin_fill()
t.seth(100)
t.circle(-50,50)
t.circle(-10,120)
t.circle(-50,56)
t.end_fill()
t.color((255,155,192),"white")
t.pu()
t.seth(90)
t.fd(-20)
t.seth(0)
t.fd(-95)
t.pd()
t.begin_fill()
t.circle(15)
t.end_fill()
t.color("black")
t.pu()
t.seth(90)
t.fd(12)
t.seth(0)
t.fd(-3)
t.pd()
t.begin_fill()
t.circle(3)
t.end_fill()
t.color((255,155,192),"white")
t.pu()
t.seth(90)
t.fd(-25)
t.seth(0)
t.fd(40)
t.pd()
t.begin_fill()
t.circle(15)
t.end_fill()
t.color("black")
t.pu()
t.seth(90)
t.fd(12)
t.seth(0)
t.fd(-3)
t.pd()
t.begin_fill()
t.circle(3)
t.end_fill()
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(-95)
t.seth(0)
t.fd(65)
t.pd()
t.begin_fill()
t.circle(30)
t.end_fill()
t.color(239,69,19)
t.pu()
t.seth(90)
t.fd(15)
t.seth(0)
t.fd(-100)
t.pd()
t.seth(-80)
t.circle(30,40)
t.circle(40,80)
t.color("red",(255,99,71))
t.pu()
t.seth(90)
t.fd(-20)
t.seth(0)
t.fd(-78)
t.pd()
t.begin_fill()
t.seth(-130)
t.circle(100,10)
t.circle(300,30)
t.seth(0)
t.fd(230)
t.seth(90)
t.circle(300,30)
t.circle(100,3)
t.color((255,155,192),(255,100,100))
t.seth(-135)
t.circle(-80,63)
t.circle(-150,24)
t.end_fill()
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(-40)
t.seth(0)
t.fd(-27)
t.pd()
t.seth(-160)
t.circle(300,15)
t.pu()
t.seth(90)
t.fd(15)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-10)
t.circle(-20,90)
t.pu()
t.seth(90)
t.fd(30)
t.seth(0)
t.fd(237)
t.pd()
t.seth(-20)
t.circle(-300,15)
t.pu()
t.seth(90)
t.fd(20)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-170)
t.circle(20,90)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(-75)
t.seth(0)
t.fd(-180)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(40)
t.seth(0)
t.fd(90)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(4)
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(70)
t.seth(0)
t.fd(95)
t.pd()
t.seth(0)
t.circle(70,20)
t.circle(10,330)
t.circle(70,30)
4.作业四(美国队长)
import turtle as t
def a(x,y):
t.pensize(30)
t.setheading(90)
t.penup()
t.goto(x,y)
t.pendown()
t.color("red")
t.circle(200)
t.penup()
t.goto(x-55,y)
t.pendown()
t.begin_fill()
t.color("red","blue")
t.circle(140)
t.end_fill()
def star(x,y):
t.setheading(0)
t.penup()
t.goto(x,y)
t.pendown()
t.color("white","white")
t.begin_fill()
for i in range(5):
t.forward(208)
t.right(144)
t.end_fill()
def mian(x,y):
a(x,y)
star(x-300,y+32)
def hand(x,y):
t.pensize(4)
t.right(90)
t.penup()
t.goto(x+400,y)
t.pendown()
t.color("gray","gray")
t.begin_fill()
t.fd(20)
t.right(90)
t.fd(78)
t.right(90)
t.fd(20)
t.right(90)
t.fd(78)
t.end_fill()
t.color("black","black")
t.right(90)
t.penup()
t.fd(24)
t.pendown()
t.left(25)
t.circle(70,50)
t.circle(-10,180)
t.left(5)
t.circle(-120,20)
t.seth(0)
t.fd(50)
t.circle(-5,100)
t.fd(74)
t.seth(180)
t.fd(82)
t.right(90)
t.fd(80)
t.hideturtle()
t.hideturtle()
t.speed(0)
mian(-100,0)
a(400,0)
hand(-250,25)
5.作业五
import turtle as t
def face(x,y):
t.pensize(2)
t.penup()
t.goto(x,y)
t.pendown()
t.color("yellow","yellow")
t.begin_fill()
t.circle(200)
t.end_fill()
def mouth(x,y):
t.pensize(15)
t.seth(-90)
t.penup()
t.goto(x,y)
t.pendown()
t.color("black")
t.circle(170,180)
def eye(x,y):
t.pensize(55)
t.seth(130)
t.penup()
t.goto(x,y)
t.pendown()
t.color("white")
t.circle(70,100)
t.pensize(20)
t.penup()
t.goto(x-110,y)
t.pendown()
t.color("black")
t.circle(10)
def all_face(x,y):
face(x,y)
mouth(x-240,y+190)
eye(x-90,y+260)
eye(x+75,y+260)
def mouth_2(x,y):
t.pensize(2)
t.seth(-90)
t.penup()
t.goto(x,y)
t.pendown()
t.begin_fill()
t.color("black","white")
t.circle(100,180)
t.left(90)
t.forward(200)
t.end_fill()
t.seth(-90)
t.penup()
t.goto(x+30,y)
t.pendown()
t.begin_fill()
t.color("red","red")
t.circle(70,180)
t.end_fill()
def all_face_2(x,y):
face(x,y)
mouth_2(x+55,y-200)
eye(x+120,y-80)
eye(x+295,y-80)
t.pensize(4)
t.penup()
t.goto(400,0)
t.pendown()
t.color("gray","gray")
t.begin_fill()
t.fd(20)
t.right(90)
t.fd(78)
t.right(90)
t.fd(20)
t.right(90)
t.fd(78)
t.end_fill()
t.color("black","black")
t.right(90)
t.penup()
t.fd(24)
t.pendown()
t.left(25)
t.circle(70,50)
t.circle(-10,180)
t.left(5)
t.circle(-120,20)
t.seth(0)
t.fd(50)
t.circle(-5,100)
t.fd(74)
t.seth(180)
t.fd(82)
t.right(90)
t.fd(80)
t.hideturtle()
t.penup()
t.pensize(2)
t.goto(200,-2)
t.showturtle()
t.pendown()
t.begin_fill()
t.seth(0)
t.fd(195)
t.right(90)
t.fd(74)
t.right(90)
t.fd(195)
t.right(90)
t.fd(74)
t.end_fill()
t.pensize(4)
t.hideturtle()
t.colormode(255)
t.color((255,155,192),"pink")
t.setup(840,500)
t.speed(0)
t.pu()
t.goto(-100,100)
t.pd()
t.seth(-30)
t.begin_fill()
a=0.4
for i in range(120):
if 0<=i<30 or 60<=i<90:
a=a+0.08
t.lt(3)
t.fd(a)
else:
a=a-0.08
t.lt(3)
t.fd(a)
t.end_fill()
t.pu()
t.seth(90)
t.fd(25)
t.seth(0)
t.fd(10)
t.pd()
t.pencolor(255,155,192)
t.speed(0)
t.seth(10)
t.begin_fill()
t.circle(5)
t.color(160,82,45)
t.end_fill()
t.pu()
t.seth(0)
t.fd(20)
t.pd()
t.speed(0)
t.pencolor(255,155,192)
t.seth(10)
t.begin_fill()
t.circle(5)
t.color(160,82,45)
t.end_fill()
t.color((255,155,192),"pink")
t.pu()
t.seth(90)
t.fd(41)
t.seth(0)
t.fd(0)
t.pd()
t.begin_fill()
t.seth(180)
t.circle(300,-30)
t.circle(100,-60)
t.circle(80,-100)
t.circle(150,-20)
t.circle(60,-95)
t.seth(161)
t.circle(-300,15)
t.pu()
t.goto(-100,100)
t.pd()
t.seth(-30)
a=0.4
for i in range(60):
if 0<=i<30 or 60<=i<90:
a=a+0.08
t.lt(3)
t.fd(a)
else:
a=a-0.08
t.lt(3)
t.fd(a)
t.end_fill()
t.color((255,155,192),"pink")
t.pu()
t.seth(90)
t.fd(-7)
t.seth(0)
t.fd(70)
t.pd()
t.begin_fill()
t.seth(100)
t.circle(-50,50)
t.circle(-10,120)
t.circle(-50,54)
t.end_fill()
t.pu()
t.seth(90)
t.fd(-12)
t.seth(0)
t.fd(30)
t.pd()
t.begin_fill()
t.seth(100)
t.circle(-50,50)
t.circle(-10,120)
t.circle(-50,56)
t.end_fill()
t.color((255,155,192),"white")
t.pu()
t.seth(90)
t.fd(-20)
t.seth(0)
t.fd(-95)
t.pd()
t.begin_fill()
t.circle(15)
t.end_fill()
t.color("black")
t.pu()
t.seth(90)
t.fd(12)
t.seth(0)
t.fd(-3)
t.pd()
t.begin_fill()
t.circle(3)
t.end_fill()
t.color((255,155,192),"white")
t.pu()
t.seth(90)
t.fd(-25)
t.seth(0)
t.fd(40)
t.pd()
t.begin_fill()
t.circle(15)
t.end_fill()
t.color("black")
t.pu()
t.seth(90)
t.fd(12)
t.seth(0)
t.fd(-3)
t.pd()
t.begin_fill()
t.circle(3)
t.end_fill()
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(-95)
t.seth(0)
t.fd(65)
t.pd()
t.begin_fill()
t.circle(30)
t.end_fill()
t.color(239,69,19)
t.pu()
t.seth(90)
t.fd(15)
t.seth(0)
t.fd(-100)
t.pd()
t.seth(-80)
t.circle(30,40)
t.circle(40,80)
t.color("red",(255,99,71))
t.pu()
t.seth(90)
t.fd(-20)
t.seth(0)
t.fd(-78)
t.pd()
t.begin_fill()
t.seth(-130)
t.circle(100,10)
t.circle(300,30)
t.seth(0)
t.fd(230)
t.seth(90)
t.circle(300,30)
t.circle(100,3)
t.color((255,155,192),(255,100,100))
t.seth(-135)
t.circle(-80,63)
t.circle(-150,24)
t.end_fill()
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(-40)
t.seth(0)
t.fd(-27)
t.pd()
t.seth(-160)
t.circle(300,15)
t.pu()
t.seth(90)
t.fd(15)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-10)
t.circle(-20,90)
t.pu()
t.seth(90)
t.fd(30)
t.seth(0)
t.fd(237)
t.pd()
t.seth(-20)
t.circle(-300,15)
t.pu()
t.seth(90)
t.fd(20)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-170)
t.circle(20,90)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(-75)
t.seth(0)
t.fd(-180)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(40)
t.seth(0)
t.fd(90)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(4)
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(70)
t.seth(0)
t.fd(95)
t.pd()
t.seth(0)
t.circle(70,20)
t.circle(10,330)
t.circle(70,30)
t.speed(0)
t.hideturtle()
all_face(-250,100)
all_face_2(-600,0)
t.end_fill()
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(-40)
t.seth(0)
t.fd(-27)
t.pd()
t.seth(-160)
t.circle(300,15)
t.pu()
t.seth(90)
t.fd(15)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-10)
t.circle(-20,90)
t.pu()
t.seth(90)
t.fd(30)
t.seth(0)
t.fd(237)
t.pd()
t.seth(-20)
t.circle(-300,15)
t.pu()
t.seth(90)
t.fd(20)
t.seth(0)
t.fd(0)
t.pd()
t.seth(-170)
t.circle(20,90)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(-75)
t.seth(0)
t.fd(-180)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(10)
t.color((240,128,128))
t.pu()
t.seth(90)
t.fd(40)
t.seth(0)
t.fd(90)
t.pd()
t.seth(-90)
t.fd(40)
t.seth(-180)
t.color("black")
t.pensize(15)
t.fd(20)
t.pensize(4)
t.color((255,155,192))
t.pu()
t.seth(90)
t.fd(70)
t.seth(0)
t.fd(95)
t.pd()
t.seth(0)
t.circle(70,20)
t.circle(10,330)
t.circle(70,30)
t.speed(0)
t.hideturtle()
all_face(-250,100)
all_face_2(-600,0)