// testattachedtype.h
#ifndef TESTATTACHEDTYPE_H
#define TESTATTACHEDTYPE_H
#include <qstring.h>
#include <qobject.h>
#include <qqml.h> // QML_HAS_ATTACHED_PROPERTIES需要头文件
/* 附加类,在.qml中使用时,相当于单件类,可以直接用类名.成员名来使用
* 附件类,可以附加到多个组件中
方法如下:
1.定义MessageBoardAttachedType,然后在里面声明需要直接调用的属性(此属性即为qml文件中需要直接使用的字段)
2.定义MessageBoard,里面只需要有定义一个静态的qmlAttachedProperties()函数返回MessageBoardAttachedType即可,
然后在该类外面使用语句QML_DECLARE_TYPEINFO(MessageBoard,QML_HAS_ATTACHED_PROPERTIES) 声明为附加类
3.在main函数中注册附加类和组件类都为qml组件
4. 声明一个组件类MyMessage,在.qml文件中注册为组件,然后将组件类的对象指针传给qmlAttachedPropertiesObject()函数,即使得
附加类MessageBoard附加到MyMessage组件上,在.qml中MyMessage组件上可以直接使用MessageBoard来调用成员
5.只要将组件类通过传参形式传给qmlAttachedPropertiesObject(),就可以使得MessageBoard成为它的附加类,也即MessageBoard附加类可以在多个组件中使用
6. .qml文件相应的组件中可以直接使用MessageBoard来取得它的成员变量
*/
class MessageBoardAttachedType : public QObject
{
Q_OBJECT
Q_PROPERTY(QString goodMsg READ GoodMsg WRITE SetGoodMsg)
Q_PROPERTY(int msgCnt READ MsgCnt WRITE SetMsgCnt)
public:
MessageBoardAttachedType(QObject *parent = Q_NULLPTR) : QObject(parent) {}
QString GoodMsg(){return mGoodMsg;}
void SetGoodMsg(const QString &msg){mGoodMsg = msg;}
int MsgCnt(){return mMsgCnt;}
void SetMsgCnt(int cnt){mMsgCnt = cnt;}
private:
QString mGoodMsg;
int mMsgCnt;
};
/*
Then the attaching type, MessageBoard, must declare a qmlAttachedProperties() method that
returns an instance of the attached object type as implemented by MessageBoardAttachedType.
Additionally, Message board must be declared as an attached type through the QML_DECLARE_TYPEINFO() macro:
*/
class MessageBoard : public QObject
{
Q_OBJECT
public:
// qml引擎会调用本接口来附加一个附加类的实例到被附加类上(通过传参具体化,也即qmlAttachedPropertiesObject()函数传的参数里面)
/*
The QML engine invokes this method in order to attach an instance of the attached object type to the
attachee specified by the object parameter. It is customary, though not strictly required,
for this method implementation to parent the returned instance to object in order to prevent memory leaks.
*/
static MessageBoardAttachedType *qmlAttachedProperties(QObject *object)
{
return new MessageBoardAttachedType(object);
}
};
QML_DECLARE_TYPEINFO(MessageBoard,QML_HAS_ATTACHED_PROPERTIES) // 声明MessageBoard为附加类型
// 组件类MyMessage
class MyMessage : public QObject
{
Q_OBJECT
Q_PROPERTY(QString msg READ Msg WRITE SetMsg NOTIFY NotifyMsgChange)
public:
MyMessage(QObject *parent = Q_NULLPTR) : QObject(parent){}
QString Msg(){return mMsg;}
void SetMsg(const QString &msg)
{
if(msg == mMsg)
return;
mMsg = msg;
emit NotifyMsgChange();
}
signals:
void NotifyMsgChange();
private:
QString mMsg;
};
// 组件类MyGoodMassageAlsoUseAttachedType
class MyGoodMassageAlsoUseAttachedType : public QObject
{
Q_OBJECT
Q_PROPERTY(QString goodMsg READ Msg WRITE SetMsg )
public:
MyGoodMassageAlsoUseAttachedType(QObject *parent = Q_NULLPTR) : QObject(parent){}
QString Msg(){return mGoodMsg;}
void SetMsg(const QString &msg)
{
mGoodMsg = msg;
}
signals:
void NotifyMsgChange();
private:
QString mGoodMsg;
};
#endif // TESTATTACHEDTYPE_H
// main.cpp
// 在main函数中注册组件类和附加类为qml的组件类
// 注册附加类为qml组件
qmlRegisterType<MyMessage>("TestAttachedType",1,0,"MyMessage");
qmlRegisterType<MessageBoardAttachedType>();
qmlRegisterType<MessageBoard>("TestAttachedType",1,0,"MessageBoard");
qmlRegisterType<MyGoodMassageAlsoUseAttachedType>("TestAttachedType",1,0,"MyGoodMassageAlsoUseAttachedType");
// 附加类用在MyMessage组件中
QQmlComponent cmp2(&engine,"qrc:/TestAttachedType.qml");
MyMessage *pMsg = qobject_cast<MyMessage*>(cmp2.create()); // 取得qml组件相应的类
// 通过MyMessage类的对象指针pMsg传给qmlAttachedPropertiesObject,即可以使得MessageBoard为MyMessage的附加类
MessageBoardAttachedType *pMsgAttachedType =
qobject_cast<MessageBoardAttachedType*>(qmlAttachedPropertiesObject<MessageBoard>(pMsg));
qDebug()<<"msg:"<<pMsg->Msg()<<" goodMsg:"<<pMsgAttachedType->GoodMsg()<<" msgCnt:"<<pMsgAttachedType->MsgCnt();
// 通过MyGoodMassageAlsoUseAttachedType类的对象指针pMsgType传给qmlAttachedPropertiesObject,
// 即可以使得MessageBoard为MyGoodMassageAlsoUseAttachedType的附加类
QQmlComponent cmp3(&engine,"qrc:/TestAttachedTypeAlsoUseOther.qml");
MyGoodMassageAlsoUseAttachedType *pMsgType = qobject_cast<MyGoodMassageAlsoUseAttachedType*>(cmp3.create());
MessageBoardAttachedType *pMsgAlsoAttachedType =
qobject_cast<MessageBoardAttachedType*>(qmlAttachedPropertiesObject<MessageBoard>(pMsgType));
qDebug()<<"MygoodMsg:"<<pMsgAlsoAttachedType->GoodMsg()<<" goodMsg:"<<pMsgAlsoAttachedType->GoodMsg()<<" msgCnt:"<<pMsgAlsoAttachedType->MsgCnt();
// TestAttachedType.qml MyMessage组件
import QtQuick 2.0
import TestAttachedType 1.0
MyMessage
{
msg : "你很靓仔"
MessageBoard.goodMsg : "今天天气很好" // 可以直接使用附加类MessageBoard来取得成员变量
MessageBoard.msgCnt : 1
}
// TestAttachedTypeAlsoUseOther.qml MyGoodMassageAlsoUseAttachedType组件
import QtQuick 2.0
import TestAttachedType 1.0
MyGoodMassageAlsoUseAttachedType
{
goodMsg : "今天阳光灿烂"
MessageBoard.goodMsg :"今天是个好日子" // 可以直接使用附加类MessageBoard来取得成员变量
MessageBoard.msgCnt : 1
}
qml创建附加类
于 2024-03-20 10:50:42 首次发布
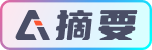