Java中的集合
集合类概述
为什么出现集合类?
我们学习的是面向对象的编程语言,面向对象的编程语言对事物的描述都是通过对象体现的,
为了方便对多个对象进行操作,我们就必须把这多个对象进行存储,而要想存储多个对象,就不能
是基本的变量了,应该是一个容器类型的变量。
回顾我们学习过的知识,有哪些容器类型的呢?
数组,StringBuilder
首先说StringBuilder,它的结果是一个字符串,不一定满足我们的需求
数组的长度
而数组的长度固定,不能适应变化的需求,在这种情况下Java提供集合类给我们使用
由此可见集合类的长度是可变的。
集合类的特点:长度可变。
Collection:是单列集合的顶层接口。
Collection表示一组对象,这些对象也称为collection的元素。
一些colloection允许有重复的元素,而另一些则是无序的。
JDK 不提供此接口的任何实现:它提供更加具体的子接口(Set,List)
创建Collection集合的对,我们采用的是多态的方式,使用的是具体的ArrayList类
因为这个类是最常用的集合类
ArrayList()
Collection<E>:
<E>:是一种特殊的数据类型,泛型,这里我们会使用就可以了
如何使用?
在出现E的地方使用引用数据类型替换即可’、
举例Collection<String>/Collection<Student>
集合类体系结构

Collection与迭代器
Collection集合概述:
是单例集合的顶层接口,Collection表示一组对象,这些对象也称为collection的元素
JDK不提供此接口的任何直接实现:它提供更具体的子接口(Set和List)实现。
创建Collection集合对象
多态的方式
具体类的实现类ArrayList()
添加元素
add(E e)
public class CollectionDemo {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
c.add("hello");
c.add("world");
c.add("java");
System.out.println(c);
}
}
Collection集合的成员方法
public class CollectionDemo {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
1. boolean add(E e):添加元素
System.out.println("add:"+c.add("hello"));
c.add("world");
c.add("java");
2. boolean remove(Object o):从集合中移除元素
3. void clear():清空集合中的元素
4. boolean contains(Object o):判断集合中是否存在指定的元素
5. boolean isEmpty():判断集合是否为空
System.out.println("isEmpty:"+c.isEmpty());
6. int size():集合的长度,也就是集合中元素的个数
System.out.println("siez:"+c.size());
System.out.println(c);
}
}
Collection集合的遍历
Collection集合的遍历
在Collection中的iterator()方法中,
Iterator<E> iterator():返回的是一个collection的元素上进行迭代的迭代器
通过集合对象调用iterator()方法得到迭代器对象,他是Iterator<E>的实现类对象
Iterator:
E next();:返回迭代的下一个元素
boolean hasNext():如果有元素则可以迭代,返回true
void remove():
public class CollectionDemo {
public static void main(String[] args) {
Collection<String> c = new ArrayList<String>();
c.add("hello");
c.add("world");
c.add("java");
Iterator<String> it = c.iterator();
while (it.hasNext()){
String s = it.next();
System.out.println(s);
}
}
}
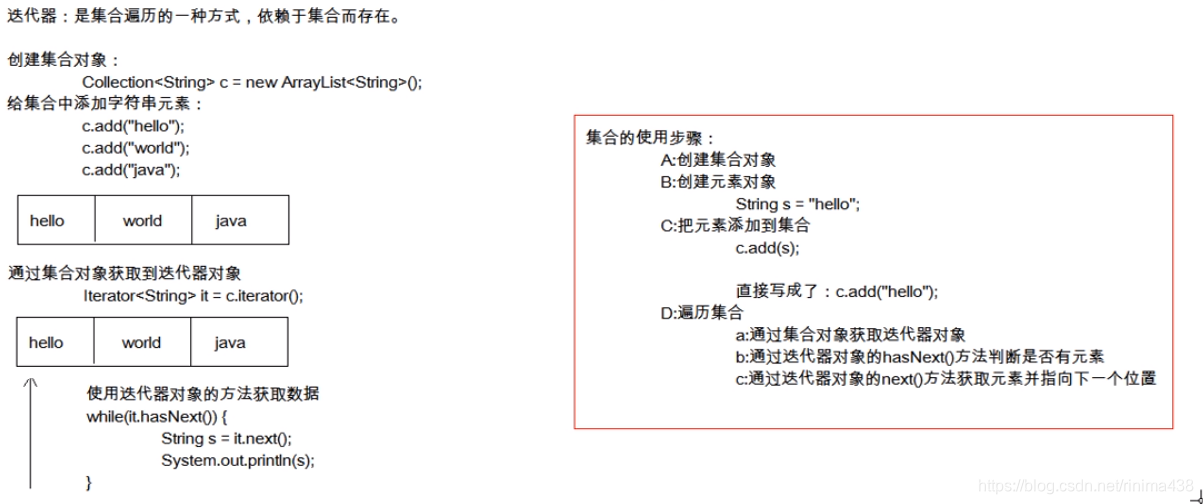
Collection集合的练习
需求:Collection集合存储自定义对象并遍历
自定义学生类:给出成员变量name和age,遍历集合的时候,在控制台输出对象的成员变量值
package com.scy11;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class CollectionDemo {
public static void main(String[] args) {
Collection<Student> c = new ArrayList<Student>();
Student s1 = new Student("林青霞",20);
Student s2 = new Student("张曼玉",35);
Student s3 = new Student("王祖贤",50);
c.add(s1);
c.add(s2);
c.add(s3);
Iterator<Student> it = c.iterator();
while (it.hasNext()){
Student s = it.next();
System.out.println(s.getName()+"---"+s.getAge());
}
}
}
List
List:
有序的 collection(也称为序列)。此接口的用户可以对列表中每个元素的插入位置进行精确
地控制。用户可以根据元素的整数索引(在列表中的位置)访问元素,并搜索列表中的元素。
与 set 不同,列表通常允许重复的元素。
List集合特点:
a:有序(存储和取出的元素的顺序一致)
b:存储的元素可以重复
public class ListDemo {
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("hello");
list.add("world");
list.add("java");
list.add("world");
Iterator<String> it = list.iterator();
while (it.hasNext()){
String s = it.next();
System.out.println(s);
}
}
}
List集合的遍历
List集合的遍历:
a:迭代器;
b:普通for循环遍历
List<String> list = new ArrayList<String>();
list.add("hello");
list.add("world");
list.add("java");
list.add("world");
for (int i=0;i<list.size();i++){
String s = list.get(i)
System.out.println(s);
}
List集合的练习
需求:List集合存储自定义对象并遍历
提示:自定义一个学生类,给出成员变量name和age,遍历集合的时候,在控制台输出学生对象的
成员变量值(用两种方式遍历)
public class ListDemo {
public static void main(String[] args) {
List<Student> list = new ArrayList<Student>();
Student s1 = new Student("林青霞",20);
Student s2 = new Student("张曼玉",10);
Student s3 = new Student("王祖贤",25);
list.add(s1);
list.add(s2);
list.add(s3);
Iterator<Student> it = list.iterator();
while (it.hasNext()){
Student s = it.next();
System.out.println(s.getName()+"---"+s.getAge());
}
System.out.println("-------------------");
for (int i=0;i<list.size(<