SushiSwap是从UniswapV2-core、 UniswapV2-periphery分叉而来,加了MasterChef合约、SushiToken等合约。下面介绍,使用truffle将Sushiswap部署到ganache。
1、下载Sushiswap-core
地址:https://github.com/sushiswap/sushiswap
下载之后,解压,将文件夹名称sushiswap-master 改成 sushiswap。
2、新建WETH.sol
参考cn.etherscan.com里的WETH合约,在sushiswap/contracts目录下,新建一个WETH.sol文件,内容如下:
//WETH.sol (去掉了末尾的注释)
/**
*Submitted for verification at Etherscan.io on 2017-12-12
*/
// Copyright (C) 2015, 2016, 2017 Dapphub
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <http://www.gnu.org/licenses/>.
pragma solidity 0.6.12;
contract WETH {
string public name = "Wrapped Ether";
string public symbol = "WETH";
uint8 public decimals = 18;
event Approval(address indexed src, address indexed guy, uint wad);
event Transfer(address indexed src, address indexed dst, uint wad);
event Deposit(address indexed dst, uint wad);
event Withdrawal(address indexed src, uint wad);
mapping (address => uint) public balanceOf;
mapping (address => mapping (address => uint)) public allowance;
receive() external payable {
deposit();
}
function deposit() public payable {
balanceOf[msg.sender] += msg.value;
emit Deposit(msg.sender, msg.value);
}
function withdraw(uint wad) public {
require(balanceOf[msg.sender] >= wad);
balanceOf[msg.sender] -= wad;
msg.sender.transfer(wad);
emit Withdrawal(msg.sender, wad);
}
function totalSupply() public view returns (uint) {
return address(this).balance;
}
function approve(address guy, uint wad) public returns (bool) {
allowance[msg.sender][guy] = wad;
emit Approval(msg.sender, guy, wad);
return true;
}
function transfer(address dst, uint wad) public returns (bool) {
return transferFrom(msg.sender, dst, wad);
}
function transferFrom(address src, address dst, uint wad)
public
returns (bool)
{
require(balanceOf[src] >= wad);
if (src != msg.sender && allowance[src][msg.sender] != uint(-1)) {
require(allowance[src][msg.sender] >= wad);
allowance[src][msg.sender] -= wad;
}
balanceOf[src] -= wad;
balanceOf[dst] += wad;
emit Transfer(src, dst, wad);
return true;
}
}
3、屏蔽不常用的接口
由于Solidity单个智能合约编译之后的字节码体积不能超过24KB,所以单个.sol文件文件最好不要超过1000行,避免出现编译失败情况。
可以通过注释掉.sol文件里不常用的函数或者接口,减少.sol编译之后的字节码体积,使其小于24KB。
a) 注释掉sushiswap/contracts/uniswap2/UniswapV2Router02.sol里的4个函数:
// 注释掉UniswapV2Router02.sol这4个函数,以减少编译体积
//function _swapSupportingFeeOnTransferTokens()
//function swapExactTokensForTokensSupportingFeeOnTransferTokens()
//function swapExactETHForTokensSupportingFeeOnTransferTokens()
//function swapExactTokensForETHSupportingFeeOnTransferTokens()
即屏蔽UniswapV2Router02.sol的第319行~第401行;
b) 注释掉sushiswap/contracts/uniswap2/interface/IUniswapV2Router02.sol里的3个函数:
// 注释掉IUniswapV2Router02.sol这3个函数,以减少编译体积
//function swapExactTokensForTokensSupportingFeeOnTransferTokens()
//function swapExactETHForTokensSupportingFeeOnTransferTokens()
//function swapExactTokensForETHSupportingFeeOnTransferTokens()
即屏蔽IUniswapV2Router02.sol的第25行~第45行。
4、编写部署脚本
在sushiswap/contracts目录,新建一个2_deploy_contracts.js文件,内容如下:
// sushiswap/contracts/2_deploy_contracts.js
const Factory = artifacts.require('uniswapv2/UniswapV2Factory.sol');
const Router = artifacts.require('uniswapv2/UniswapV2Router02.sol');
const WETH = artifacts.require('WETH.sol');
const MockERC20 = artifacts.require('MockERC20.sol');
const SushiToken = artifacts.require('SushiToken.sol');
const MasterChef = artifacts.require('MasterChef.sol');
const SushiBar = artifacts.require('SuShiBar.sol');
const SushiMaker = artifacts.require('SushiMaker.sol');
const Migrator = artifacts.require('Migrator.sol');
const web3_utils = require('web3-utils');
module.exports = async function(deployer, _network, address) {
const [admin, _] = address;
await deployer.deploy(WETH);
const weth = await WETH.deployed();
const tokenA = await MockERC20.new('Token A', 'TKA', web3_utils.toWei('1000'));
const tokenB = await MockERC20.new('Token B', 'TKB', web3_utils.toWei('1000'));
await deployer.deploy(Factory, admin);
const factory = await Factory.deployed();
await factory.createPair(weth.address, tokenA.address);
await factory.createPair(weth.address, tokenB.address);
await deployer.deploy(Router, factory.address, weth.address);
const router = await Router.deployed();
await deployer.deploy(SushiToken);
const sushiToken = await SushiToken.deployed();
await deployer.deploy(
MasterChef,
sushiToken.address,
admin,
web3_utils.toWei('100'),
1,
10
);
const masterChef = await MasterChef.deployed();
await sushiToken.transferOwnership(masterChef.address);
await deployer.deploy(SushiBar, sushiToken.address);
const sushiBar = await SushiBar.deployed();
await deployer.deploy(
SushiMaker,
factory.address,
sushiBar.address,
sushiToken.address,
weth.address
);
const sushiMaker = await SushiMaker.deployed();
await factory.setFeeTo(sushiMaker.address);
await deployer.deploy(
Migrator,
masterChef.address,
'0x5C69bEe701ef814a2B6a3EDD4B1652CB9cc5aA6f',
factory.address,
1
);
};
5、部署Sushiswap-core
a) 设置ganache的IP:127.0.0.1,端口为8545,HardFork选中Istanbul。
由于UniswapV2的工厂合约、路由合约都使用的是Istanbul类型的硬分叉,所以,在ganache设置HARDFORK为Istanbul,然后重启ganache,如图(1)所示:
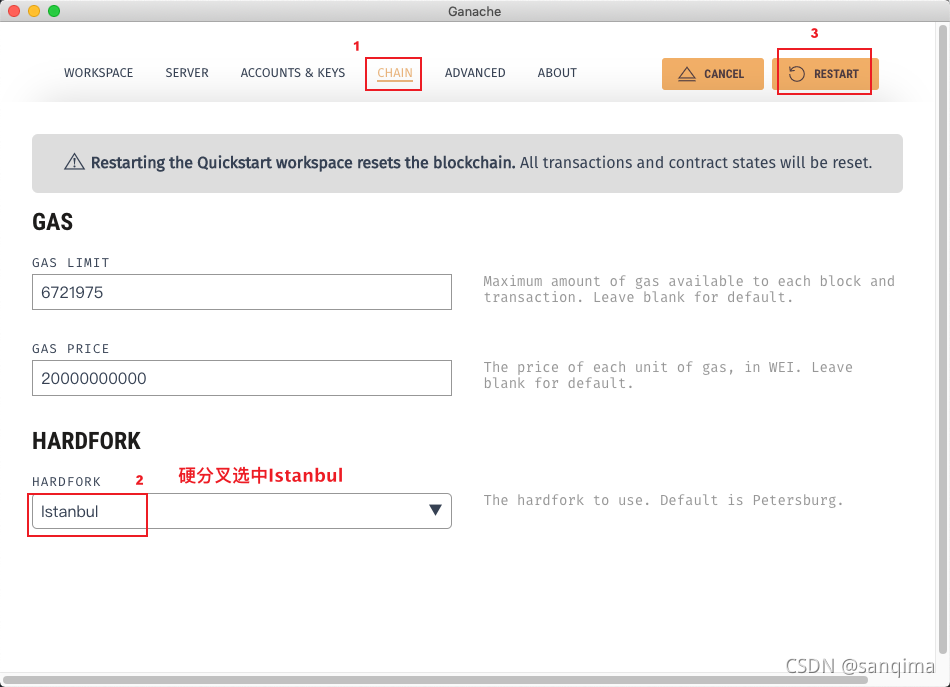
b) 打开一个黑框框终端,依次输入如下命令:
## 进入工程目录
cd sushiswap
## 进入truffle控制台
truffle console
## 编译智能合约
compile
## 部署sushiswap
migrate -f 2 --to 2
效果如图(2)、图(3)所示:
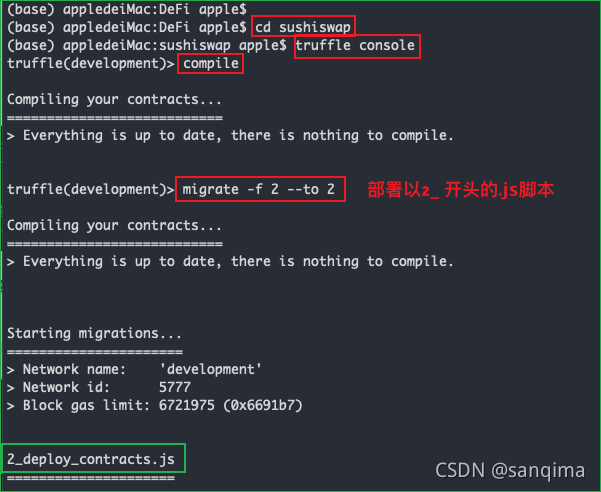
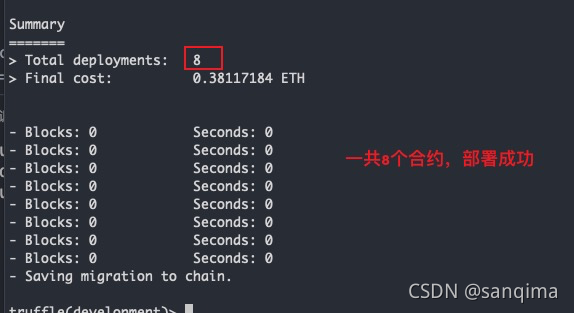