平时练习三
1. 应用JavaScript编写留言功能,在文本中输入文字提交后,在下方进行显示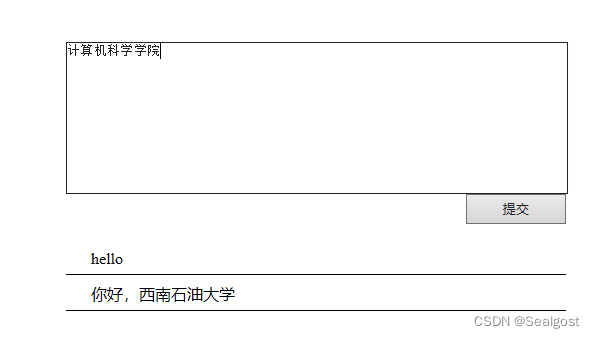
提示:可将下方内容以列表体现,提交时动态创建列表的项。可使用以下两种方式之一的方法:
1) 使用CreateElenment动态创建li标签及li中的文本
2) 在列表标签ul或者ol对象上设置InnerHtml
列表对象.innerHTML += “
- 文本内容
- ”
代码:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>E3.1</title> </head> <body> <div> <textarea id="input"></textarea> <button id="submit">提交</button> <ul id="content"> <li id="firstLi">hello</li> <li>你好,西南石油大学</li> </ul> </div> </body> </html> <script type="text/javascript"> var button=document.getElementById("submit"); button.onclick=function(){ var UL=document.getElementById("content"); var message=document.getElementById("input"); var LI=document.createElement("li"); LI.innerHTML=message.value; UL.appendChild(LI); message.value=""; } </script> <style> body{ position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } #input{ width: 800px; height: 200px; } #submit{ width: 100px; height: 40px; float: right; } #content li{ font-size: 22px; list-style: none; height: 40px; border-bottom: 2px solid black; padding-left: 10px; padding-top: 12px; } #firstLi{ margin-top: 80px; } </style>
2. 设计一个选项卡功能,当鼠标移动至某一选项卡时出现切换。
提示:- 选项卡可采用三个行内元素,为选中背景色#000,选中背景色#aaa
- 选项卡内容可采用三个不同列表
- 针对选项卡和选项内容定义两组不同样式。选项卡未选中背景色#000,选中背景色#aaa;文本颜色#fff;;选项内容未选中不显示display:none,选中显示display:block。
- 通过JavaScript动态设置样式,页面对象.classname=“class样式选择器”
如mydiv. className = “selectSpanStyle” - 鼠标移至选项卡设置onmouseenter事件
如:mySpan.onmouseenter = function(){
…………
}
代码:
<!DOCTYPE htjl> <htjl lang="en"> <head> <jeta charset="UTF-8"> <title>E3.2</title> </head> <body> <div id="navigation"> <ul> <li>热图排行</li> <li>美图速递</li> <li>前沿科技</li> </ul> </div> <div id="content"> <section class="bulk" > <div class="news">座谈会</div> <div class="news">高压线不能碰</div> <div class="news">全国高中2019年秋季起分步实施新课程使用新教材</div> <div class="news">个税</div> </section> <section class="bulk" > <div class="news">鹅教官、羊陪练......这所中学养的动物成了“网红”</div> <div class="news">最伤身体的10个生活习惯,一定要避开</div> <div class="news">12岁孩子带着父亲去西藏 吃住行自己拿主意</div> <div class="news">16岁男孩暑假干了俩月工地,练出满身肌肉,只为赚学费</div> </section> <section class="bulk" > <div class="news">科技新闻标题01</div> <div class="news">科技新闻标题02</div> <div class="news">科技新闻标题03</div> <div class="news">科技新闻标题04</div> </section> </div> </body> </htjl> <script> window.onload=function () { var Nav=document.getElementById('navigation').getElementsByTagName('li'); var Bulk=document.getElementById('content').getElementsByClassName('bulk'); for(var i=0;i<Nav.length;i++){ Nav[i].index=i; Nav[i].onmouseenter =function () { for(var i=0;i<Nav.length;i++){ Bulk[i].style.display="none"; } Bulk[this.index].style.display="block" } for(var m=1;m<Nav.length;m++){ Bulk[m].style.display="none"; } } }; </script> <style> body{ position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } ul{ width: 450px; height: 30px; margin: 0; padding: 0; list-style: none; } li{ width: 150px; height: 30px; float: left; background-color:#000; color:#FFF; font-size: 18px; text-align: center; } li:hover{ background-color: #aaa; } .news{ background-color:#FFF; border-bottom: 2px dotted #aaa; padding-left: 10px; padding-top: 5px; padding-bottom: 5px; } </style>
3. 设计如下表单,并进行数据验证。
提示:- 输入元素取值可通过var name =document.getElementsById(“元素id”).value;
- 判断长度name.length
- 是否英文字符开头
- 首字母是大小写字符,获取输入的字符对应的编码
var keycode=name.charCodeAt(0);
if(keycode <65||( keycode >90&& keycode <97)|| keycode >122){
不是英文字符
}
代码:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>E3.3</title> </head> <body> <div class="form"> <div class="title">注册</div> <div class="content"> <input class="userName" id="userName" placeholder="请输入您的用户名"> <div class="reminder"> <p class="sunReminder" id="sunReminder01">用户名长度必须为6到18位!</a> <p class="sunReminder" id="sunReminder02">用户名必须以英文字母开头!</a> </div> <input class="password" id="password" placeholder="请输入您的密码"> <div class="reminder"> <p class="sunReminder" id="sunReminder03">密码长度不能小于6位!</a> </div> <button id ="btn">提交</button> </div> </div> </body> </html> <script > window.onload=function () { var bton=document.getElementById("btn"); var sunReminder01=document.getElementById("sunReminder01"); var sunReminder02=document.getElementById("sunReminder02"); var sunReminder03=document.getElementById("sunReminder03"); bton.onclick=function(){ sunReminder01.setAttribute('style','display:none'); sunReminder02.setAttribute('style','display:none'); sunReminder03.setAttribute('style','display:none'); var userName=document.getElementById("userName").value; var password=document.getElementById("password").value; if(userName.length>18 || userName.length<6){ sunReminder01.setAttribute('style','display:block'); } if(password.length<6){ sunReminder03.setAttribute('style','display:block'); } var keycode=userName.charCodeAt(0); if(keycode <65||( keycode >90&& keycode <97)|| keycode >122){ sunReminder02.setAttribute('style','display:block'); } } } </script> <style> body{ position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } .form{ width: 450px; } .title { padding: 10px; text-align: center; background-color: #0099cc; text-align: center; color: #fff; font: 25px bold; } .content{ background-color: #00ccff; height: 300px; padding-left: 70px; padding-top: 30px; } .userName{ height: 35px; width: 300px; padding-left: 10px; } .password{ height: 35px; width: 300px; padding-left: 10px; } .reminder{ padding-top: 5px; padding-bottom: 5px; margin: 0px; height: 40px; } .sunReminder{ font-size: 15px; color:red; display:none; } #btn{ height: 40px; width: 300px; background-color:#0099cc; color:#fff; font-size: 20px; } </style>