import cv2
import matplotlib.pyplot as plt
import numpy as np
def show(image):
plt.imshow(image)
plt.axis('off')
plt.show()
def imread(image):
image = cv2.imread(image)
image = cv2.cvtColor(image,cv2.COLOR_BGR2RGB)
return image
image = imread('jianghe.jpeg')
show(image)
print(image.shape)
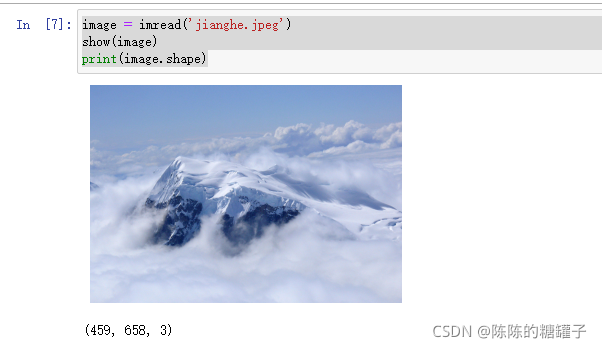
width = 150
high = 150
image = cv2.resize(image,(width,high))
show(image)
print(image.shape)
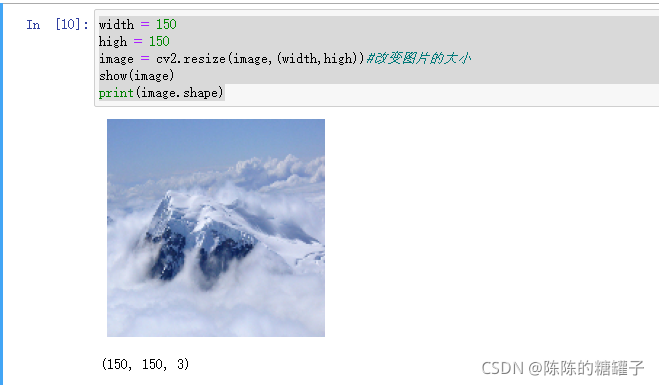
image = imread('jianghe.jpeg')
width = 80
high = int(image.shape[0]*width/image.shape[1])
image = cv2.resize(image,(width,high))
show(image)
print(image.shape)
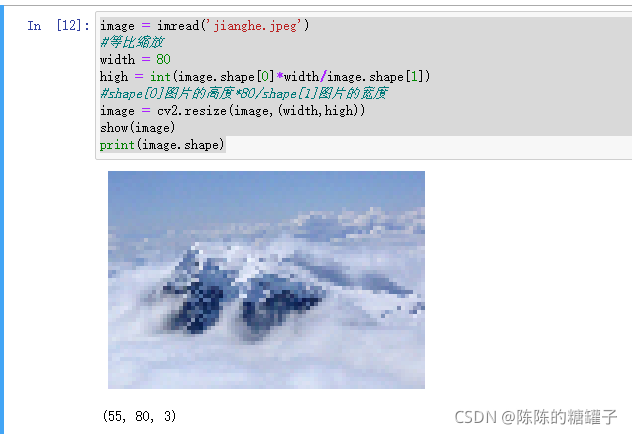
image = imread('jianghe.jpeg')
width = 150
high = 150
image = cv2.resize(image,(width,high), interpolation=cv2.INTER_NEAREST)
show(image)
print(image.shape)
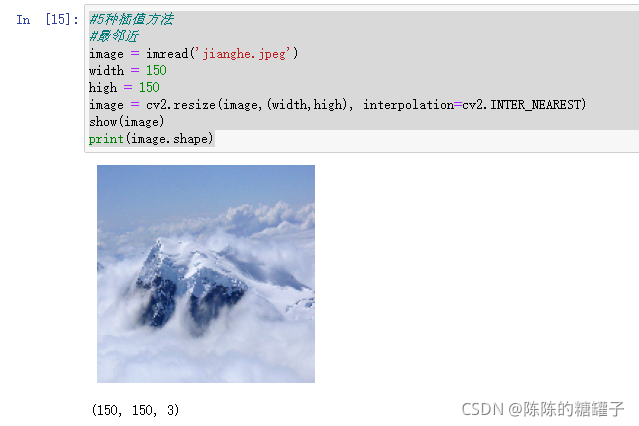
image = imread('jianghe.jpeg')
width = 150
high = 150
image = cv2.resize(image,(width,high), interpolation=cv2.INTER_LINEAR)
show(image)
print(image.shape)
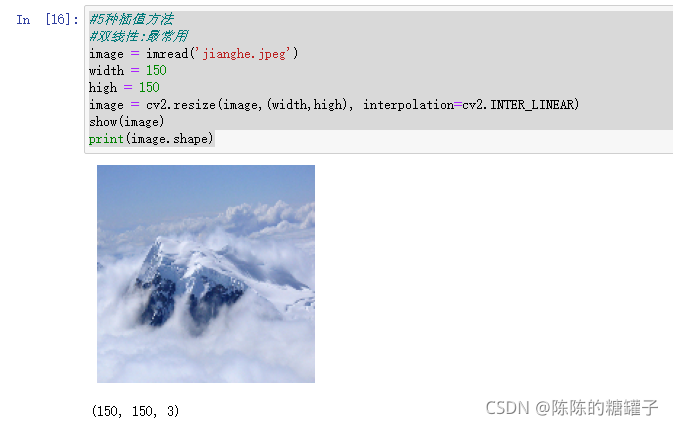
image = imread('jianghe.jpeg')
width = 150
high = 150
image = cv2.resize(image,(width,high), interpolation=cv2.INTER_AREA)
show(image)
print(image.shape)
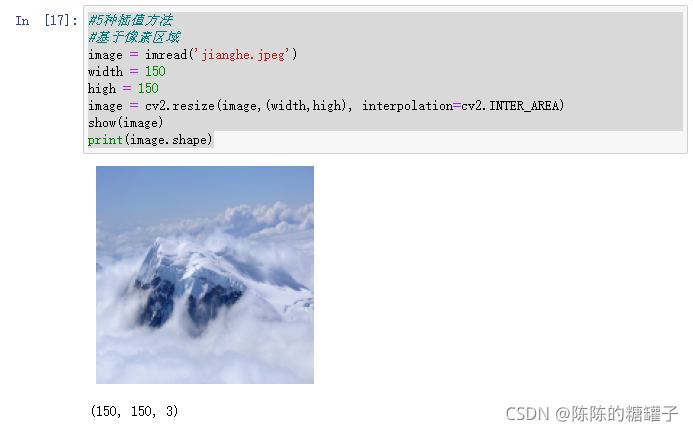
image = imread('jianghe.jpeg')
width = 150
high = 150
image = cv2.resize(image,(width,high), interpolation=cv2.INTER_CUBIC)
show(image)
print(image.shape)
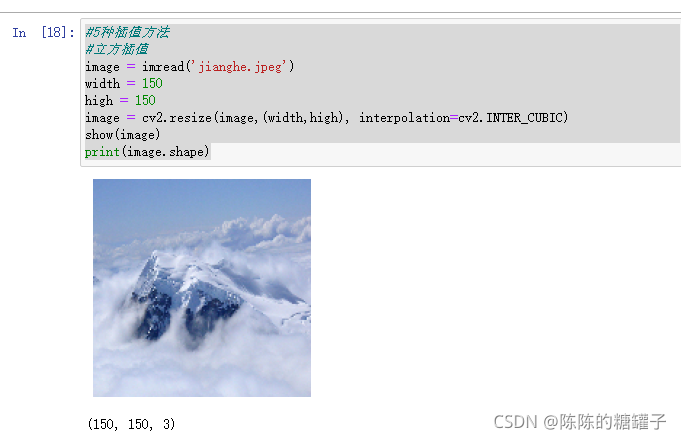
image = imread('jianghe.jpeg')
width = 150
high = 150
image = cv2.resize(image,(width,high), interpolation=cv2.INTER_LANCZOS4)
show(image)
print(image.shape)
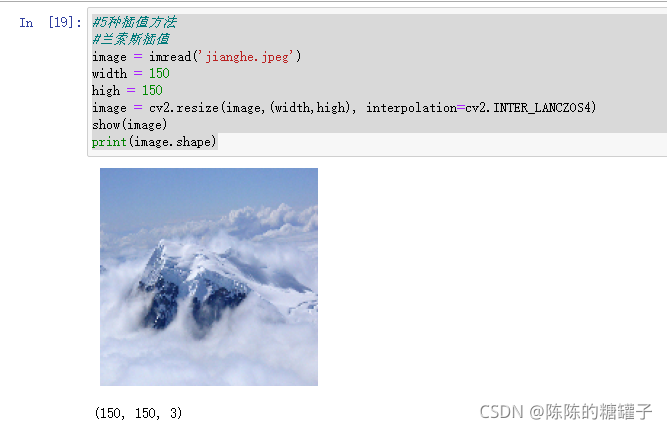