Description
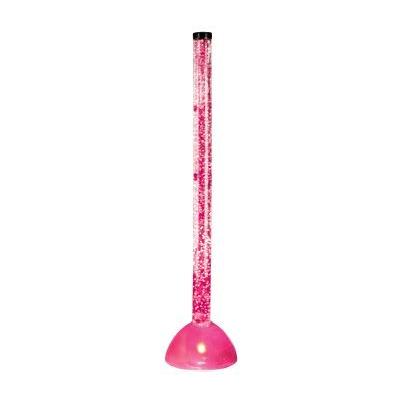
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
Output
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
题目大意:
给出长度为n的序列,每次只能交换相邻的两个元素,问至少要交换几次才使得该序列为递增序列。
解题思路:
一看就是冒泡,交换一次记录一次就可以了
但是n的范围达到50W,冒泡O(n^2)的复杂度铁定超时(即使有7000ms,其实这是一个陷阱)
直接用快排又不符合题目的要求(相邻元素交换),快排是建立在二分的基础上的,操作次数肯定比在所要求的规则下的交换次数要更少
那么该怎么处理?
其实这题题目已经给出提示了:Ultra-QuickSort
特殊的快排,能和快排Quicksort相媲美的就是归并排序Mergesort了,O(nlogn)
但是用归并排序并不是为了求交换次数,而是为了求序列的 逆序数(学过《线代》的同学应该很熟悉了)
一个乱序序列的 逆序数 = 在只允许相邻两个元素交换的条件下,得到有序序列的交换次数
关于归并排序具体实现请看这篇文章:
递归算法学习---归并排序
例如例子的
9 1 0 5 4
由于要把它排列为上升序列,上升序列的有序就是 后面的元素比前面的元素大
而对于序列9 1 0 5 4
9后面却有4个比9小的元素,因此9的逆序数为4
1后面只有1个比1小的元素0,因此1的逆序数为1
0后面不存在比他小的元素,因此0的逆序数为0
5后面存在1个比他小的元素4, 因此5的逆序数为1
4是序列的最后元素,逆序数为0
因此序列9 1 0 5 4的逆序数 t=4+1+0+1+0 = 6 ,恰恰就是冒泡的交换次数
PS:注意保存逆序数的变量t,必须要用__int64定义,int和long都是无法保存的。。。。会导致WA。 以前的long long 在现在的VC编译器已经无法编译了。
注意__int64类型的输出必须使用指定的c格式输出,printf(“%I64d”,t);
cout是无法输出__int64类型的
序列数组s[]用int就足够了,每个元素都是小于10E而已
#include <iostream>
#include <algorithm>
#include <cstring>
using namespace std;
const int inf=1000000000; //10E
__int64 cnt;
/*归并排序
左边小左边,左边++;右边小取右边,右边++*/
template<typename T>
void merge(T array[], int low, int mid, int high)
{
int k;
int len_L = mid - low + 1;
int len_R = high - mid;
T * left = new T[ len_L + 2 ];
T * right = new T[ len_R + 2 ];
int i,j;
for( i = 1; i <= len_L; i++ ) // 将mid左侧的数据拷贝到left数组中
left[i] = array[low + i - 1];
left[len_L + 1] = inf; //设置无穷上界,避免比较大小时越界
for( j = 1; j <= len_R ; j++ ) //将mid右侧的数据拷贝到right数组中
right[j] = array[mid + j];
right[len_R + 1] = inf; //设置无穷上界,避免比较大小时越界
i=j=1;
for( k = low; k <= high; )
if( left[i] <= right[j] )
array[k++] = left[i++];
else
{
array[k++] = right[j++];
cnt += len_L - i + 1; //计算逆序数
}
delete left;
delete right;
}
template<typename T>
void merge_sort(T array[], unsigned int first, unsigned int last)
{
int mid = 0;
if(first<last)
{
mid = ( first + last ) / 2;
//mid = (first & last) + ((first ^ last) >> 1);
merge_sort(array, first, mid);
merge_sort(array, mid+1,last);
merge(array,first,mid,last);
}
}
int main()
{
int N, i;
while( cin >> N && N )
{
int *a = new int[N + 1];
for( i = 1;i <= N; i++ )
cin >> a[i];
cnt = 0;
merge_sort( a, 1, N );
//for( i = 0; i < N; i++ )
// cout<< a[i] << ' ';
cout << cnt << endl;
delete a;
}
return 0;
}