Tensorflow实现直线拟合
代码:
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
tf.compat.v1.disable_eager_execution()
rng = np.random
learning_rate = 0.02
training_epoch = 10000
display_step = 50
train_X = np.asarray([3.3,4.4,5.5,6.71,6.93,4.618,9.779,6.182,7.59,2.167,7.042,10.791,5.313,7.997,5.654,9.27,3.1])
train_Y = np.asarray([1.7,2.76,2.09,3.19,1.694,1.573,3.366,2.596,2.53,1.2,2.827,3.465,1.65,2.904,2.42,2.94,1.3])
n_samples_x = train_X.shape[0]
X = tf.compat.v1.placeholder(tf.float32)
Y = tf.compat.v1.placeholder(tf.float32)
W = tf.Variable(rng.random(),name= 'Weight')
b = tf.Variable(rng.random(),name= 'bias')
pred = tf.add(tf.multiply(X,W),b)
cost = tf.reduce_sum(tf.pow(pred - Y,2))/(2*n_samples_x)
optimiziers = tf.compat.v1.train.GradientDescentOptimizer(learning_rate).minimize(cost)
init = tf.compat.v1.global_variables_initializer()
with tf.compat.v1.Session() as sess:
sess.run(init)
for epochs in range(training_epoch):
for (x,y) in zip(train_X,train_Y):
sess.run(optimiziers,feed_dict={X:x,Y:y})
if (epochs + 1)%display_step == 0:
c = sess.run(cost,feed_dict={X:train_X,Y:train_Y})
print('epochs:{:},cost:{:.9f},w:{:.3f},b:{:.3f}'.format(epochs,c,sess.run(W),sess.run(b)))
print("Optimization Finished!")
train_cost = sess.run(cost,feed_dict={X:train_X,Y:train_Y})
Ww = sess.run(W)
Bb = sess.run(b)
print("Final cost:{:.9f},Final W:{:.3f},Final b:{:.3f}".format(train_cost,Ww,Bb))
plt.plot(train_X,train_Y,'ro',label = 'Origin data')
plt.plot(train_X,Ww*train_X+Bb,'b',label = 'Fitted Line')
plt.legend()
plt.show()
结果:
Optimization Finished!
Final cost:0.079473563,Final W:0.250,Final b:0.784
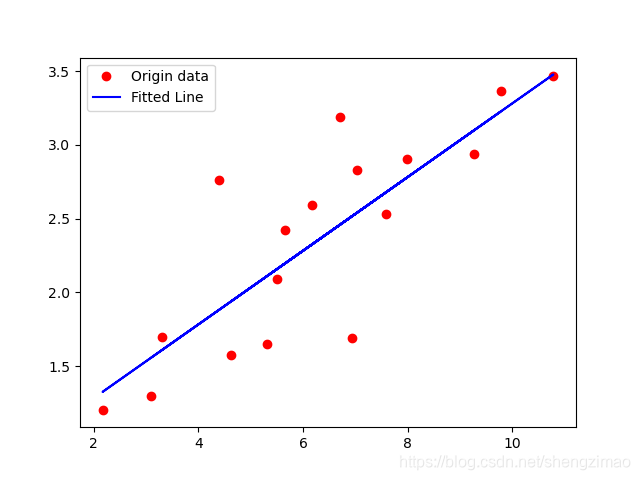