Unity官方文档之“图形性能优化-批量draw call”的翻译,E文链接。
Draw Call Batching 批量Draw Call
To draw an object on the screen, the engine has to issue a draw call to the graphics API (e.g. OpenGL or Direct3D). Draw calls are often seen as expensive, with the graphics API doing significant work for every draw call, causing performance overhead on the CPU side. However this is not the case. The expensive part is the changing of the resources accessed by the GPU between the draw calls as it triggers a graphics driver validation each time. The emphasis should be on reducing the batch count, rather than the draw call count.
为了在屏幕上绘制一个物体,引擎需要发一个draw call给图形API(比如OpenGL或Direct3D)。对每个draw call,图形API要做可观的工作,所以通常认为draw call开销大,它消耗CPU性能。然而,这还不是关键,开销大的地方是draw call之间GPU变换访问资源,因为每个draw call都要触发一次图形驱动程序工作。降低批量数比draw call数更重要。
Unity uses static batching to address this. The goal of the static batching is to regroup as many meshes in less buffers to get better performance, rendering giant meshes instead of a lot of small meshes which is inefficient. Unity will only loop on the same resources to render different ranges of these resources. Effectively it executes a series of fast draw calls for each staticcally batched mesh.
Unity使用静态批处理方法来降低批量处理。静态处理的目的是重组许多网格来获取更好的性能,这样缓存更少,渲染大网格而不是很多低效的小网格。Unity仅仅循环处理相同资源来渲染这些资源的不同种类。针对每个静态调用批量过的网格,Unity高效执行连续的快速绘制调用。
Built-in batching support in Unity has significant benefit over simply combining geometry in the modeling tool (or using the CombineChildren script from the Standard Assets package). In Unity 5.0, there is only one build of the index buffer on start and then a draw call is submitted for each sub mesh of that big mesh for each visible sub mesh.
Unity支持的内置批量处理,比在建模工具中(或使用Standard Assets包中的CombineChildren脚本)简单的合并几何,有更好的效果。Unity 5.0中,开始时只构建一个索引缓存,然后针对大网格的所有可视子网格提交一次绘制调用。
Materials 材质
Only objects sharing the same material can be batched together. Therefore, if you want to achieve good batching, you need to share as many materials among different objects as possible.
只有共用相同材质的物体才可以一起批处理。因此,如果想获得好的批处理效果,你需要对不同物体尽可能多的共用相同的材质。
If you have two identical materials which differ only in textures, you can combine those textures into a single big texture - a process often called texture atlasing. Once textures are in the same atlas, you can use a single material instead.
如果两个独立的材质仅仅纹理不同,你可以合同两张纹理到一个大纹理上——这常常叫做打纹理图集。一旦纹理在同一个图集上,你就可以用一个材质来代替两个材质了。
If you need to access shared material properties from the scripts, then it is important to note that modifying Renderer.material will create a copy of the material. Instead, you should use Renderer.sharedMaterial to keep material shared.
如果你需要在脚本中访问共享材质的属性,请注意修改Randerer.material会创建材质的一份拷贝,你应该用Renderer.sharedMaterial来保持材质共享。
Dynamic Batching 动态批量
Unity can automatically batch moving objects into the same draw call if they share the same material and fulfill other criteria. Dynamic batching is done automatically and does not require any additional effort on your side.
Unity可以自动批量处理移动的物体到相同draw call,如果他们共享相同的材质并且遵守其它标准。动态批处理是自动完成的,你不需要做任何事。
Tips:小提示:
- Batching dynamic objects has certain overhead per vertex, so batching is applied only to meshes containing less than 900 vertex attributes in total.
- 批处理动态物体在每个顶点有一定开销,所以批处理只对总共包含小于900个顶点的网格进行。
- If your shader is using Vertex Position, Normal and single UV, then you can batch up to 300 verts; whereas if your shader is using Vertex Position, Normal, UV0, UV1 and Tangent, then only 180 verts.
- Please note: attribute count limit might be changed in future
- 如果你的着色器使用顶点位置、法线和UV值,那么最多可以批处理300个顶点;如果你的着色器使用了顶点位置、法线、UV0、UV1和切线,那么只能处理180个顶点。
- 请注意:属性的数量限制将来可能变化。
- Generally, objects should be using the same transform scale.
- 一般的,物体应该使用相同的缩放。
- The exception is non-uniform scaled objects; if several objects all have different non-uniform scale then they can still be batched.
- 例外是非统一缩放的物体:如果几个物体用不同的非统一缩放,那么它们可以批处理。【译注:这里的意思是,如果一个缩放(1,1,1)的物体和一个缩放(2,2,2)的物体不可以批处理;但是,缩放(1,2,1)的物体和缩放(1,3,1)的物体可以批处理】
- Using different material instances - even if they are essentially the same - will make objects not batched together.
- Objects with lightmaps have additional renderer parameter: lightmap index and offset/scale into the lightmap. So generally dynamic lightmapped objects should point to exactly the same lightmap location to be batched.
- Multi-pass shaders will break batching. Almost all unity shaders supports several lights in forward rendering, effectively doing additional pass for them. The draw calls for “additional per-pixel lights” will not be batched.
- Objects that receive real-time shadows will not be batched.
- 使用不同材质的实例,即使他们本质上是相同的,但是物体不批处理到一起。含有lightmaps的物体有附加的渲染参数:lightmap索引和偏移/缩放,所以,一般来说,为了批处理动态lightmap的物体,应该严格的指向相同的lightmap部位。
- 多通道着色器会破坏批处理,几乎所有unity着色器在前向渲染时都支持几个灯光,并且为它们添加高效的附加通道。“附加的逐像素灯光”的draw call不会被批处理。
- 接受实时阴影的物体也不会被批处理。
Static Batching 静态批处理
Static batching, on the other hand, allows the engine to reduce draw calls for geometry of any size (provided it does not move and shares the same material). Static batching is significantly more efficient than dynamic batching. You should choose static batching as it will require less CPU power.
在另一方面,静态批处理允许引擎为任何尺寸的几何降低draw call(如果它不移动,并且共用相同材质)。静态批处理比动态批处理效果好得多,因为它需要消耗更少的CPU,所以你应该选择静态批处理。
In order to take advantage of static batching, you need explicitly specify that certain objects are static and will not move, rotate or scale in the game. To do so, you can mark objects as static using the Static checkbox in the Inspector:
为了利用静态批处理,你应该显式指定某些物体是静态的,并且它不移动、旋转或缩放。你可以在检视器(Inspector)中使用Static checkbox来标明物体是静态的。
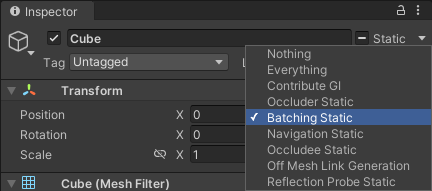
Using static batching will require additional memory for storing the combined geometry. If several objects shared the same geometry before static batching, then a copy of geometry will be created for each object, either in the Editor or at runtime. This might not always be a good idea - sometimes you will have to sacrifice rendering performance by avoiding static batching for some objects to keep a smaller memory footprint. For example, marking trees as static in a dense forest level can have serious memory impact.
使用静态批处理需要额外的内存来存储合并的几何。如果几个物体在批处理前共用相同的几何,那么在编辑器中或运行时,对每个物体都会创建一份几何的拷贝。批处理不一定是好的,有时候对有些物体,你需要牺牲渲染性能来避免批处理,以减少内存消耗。比如,把一片浓密森林中的每一棵树标注为静态的,会消耗很大的内存。
Static batching does not reduce batches instead of draw calls. Their number stays the same, but they are a lot faster with static batching.
静态批处理降低批次,而不是draw call数,draw call还是那么多,但是使用静态批处理可以获得更快的效果。
Other batching tips 其它批处理提示
Currently, only Mesh Renderers and Particle Systems are batched. This means that skinned meshes, cloth, trail renderers and other types of rendering components arenot batched.
目前,只有Mesh.Renderers和粒子系统使用批处理,蒙皮网格、衣服、尾迹渲染和其它类型的渲染组件并没有批处理。
Semitransparent shaders most often require objects to be rendered in back-to-front order for transparency to work. Unity first orders objects in this order, and then tries to batch them - but because the order must be strictly satisfied, this often means less batching can be achieved than with opaque objects.
半透明shader为了透明效果的需要,常常要求物体以由后向前的顺序渲染。Unity首先要求物体以这种顺序,然后试着批处理它们,但是因为顺序被严格限制了,所以,不透明物体比透明物体会获得更好的批处理效果。
Some parts of Unity’s rendering do not have batching implemented yet; for example rendering shadow casters, camera’s depth textures or GUI will not do batching.
Unity的有些渲染还没实现批处理,比如渲染阴影投射、相机的深度纹理或者GUI将不会做批处理。
原文: http://blog.csdn.net/ynnmnm/article/details/44650801