1.新建图片处理工具
- 新建包,命名:edu.qau.utils
- 新建类,命名:ImageUtils
2.新建类getPath,获取文件路径
public static String getPath(Context context, Uri uri) {
String path = null;
Cursor cursor = context.getContentResolver()
.query(uri, new String[]{MediaStore.Images.Media.DATA},
null, null, null);
if (cursor != null) {
cursor.moveToFirst();
int columnIndex = cursor
.getColumnIndex(MediaStore.Images.Media.DATA);
path = cursor.getString(columnIndex);
cursor.close();
}
return path;
}
3.在MainActicity中,限制图片上传数量
public void addImg(View view) {
if (count < 9) {
dialog.show();
} else {
Toast.makeText(this, "最多上传九张", Toast.LENGTH_LONG).show();
}
}
4.新建ImageBean
- 新建包,命名:edu.qau.bean
- 新建类,命名:Image
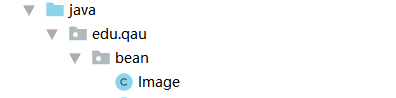
5.为Image添加成员属性并封装
package edu.qau.bean;
public class Image {
private String path;
private String name;
private int flag;
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getFlag() {
return flag;
}
public void setFlag(int flag) {
this.flag = flag;
}
}
6.在MainActicity中,实例化添加Image数组
private ArrayList<Image> list;
list = new ArrayList<Image>();
7.在MainActicity中,提交图片
public void submit(View view) {
int netType = NetWorkUtils.getAPNType(this);
if (netType == 0) {
Toast.makeText(this, "无法连接到网络", Toast.LENGTH_LONG).show();
} else {
String temp = talkEditText.getText().toString();
Talk talk = new Talk();
talk.setTalk(temp);
talk.setList(list);
Gson gson = new Gson();
MultipartBody.Builder builder = new MultipartBody.Builder();
builder.addFormDataPart("json", gson.toJson(talk));
for (int i = 0; i < list.size(); i++) {
final Image image = list.get(i);
if (netType == 1) {
File uploadFile = new File(image.getPath());
builder.addFormDataPart("File" + i, uploadFile.getName(), RequestBody.create(JPEG, uploadFile));
} else {
RequestBody os = new RequestBody() {
@Nullable
@Override
public MediaType contentType() {
return JPEG;
}
@Override
public void writeTo(@NotNull BufferedSink sink) throws IOException {
ByteArrayOutputStream byteArrayOutputStream = ImageUtils.compressImage(image.getPath(), 300);
sink.write(byteArrayOutputStream.toByteArray());
byteArrayOutputStream.close();
byteArrayOutputStream.reset();
byteArrayOutputStream = null;
}
};
builder.addPart(Headers.of("Content-Disposition", "form-data; name=\"file" + i + "\""), os);
}
}
8.MainActicity源码
package edu.qau.phone;
import android.Manifest;
import android.annotation.TargetApi;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.content.res.Configuration;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.os.Message;
import android.os.SystemClock;
import android.provider.MediaStore;
import android.support.annotation.NonNull;
import android.support.v4.content.FileProvider;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import com.google.gson.Gson;
import com.orhanobut.dialogplus.DialogPlus;
import com.orhanobut.dialogplus.OnItemClickListener;
import com.squareup.picasso.Picasso;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import edu.qau.bean.Image;
import edu.qau.bean.Talk;
import edu.qau.utils.ImageUtils;
import edu.qau.utils.NetWorkUtils;
import edu.qau.utils.OkHttpUtils;
import okhttp3.Call;
import okhttp3.Headers;
import okhttp3.MediaType;
import okhttp3.MultipartBody;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import okio.BufferedSink;
public class MainActivity extends Activity {
public static final int TAKE = 1;
public static final int PICK = 2;
private LayoutInflater inflater;
private DialogPlus dialog;
private File camera;
private File file;
private Uri uri;
private int count = 0;
private LinearLayout group;
private ArrayList<Image> list;
private EditText talkEditText;
private MediaType JPEG = MediaType.parse("image/jpeg");
private Handler handler;
private ImageView image;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
}
public void init() {
list = new ArrayList<Image>();
inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
talkEditText = findViewById(R.id.talk);
group = findViewById(R.id.preview_group);
DialogAdapter adapter = new DialogAdapter();
dialog = DialogPlus.newDialog(this).setAdapter(adapter)
.setContentBackgroundResource(R.color.dialog_bg)
.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(@NonNull DialogPlus dialog, @NonNull Object item,
@NonNull View view, int position) {
if (position == 1) {
dialog.dismiss();
take();
} else if (position == 0) {
pick();
} else {
dialog.dismiss();
}
}
}).setExpanded(false).create();
handler = new Handler(getMainLooper(), new Handler.Callback() {
@Override
public boolean handleMessage(Message msg) {
if (msg.what == 1) {
Toast.makeText(MainActivity.this, "上传成功", Toast.LENGTH_LONG).show();
} else if (msg.what == 2) {
Toast.makeText(MainActivity.this, "上传失败", Toast.LENGTH_LONG).show();
}
return false;
}
});
File dcim = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DCIM);
if (!dcim.exists()) {
dcim.mkdir();
}
camera = new File(dcim, "camera");
if (!camera.exists()) {
camera.mkdir();
}
}
private void takePic() {
file = new File(camera, SystemClock.elapsedRealtime() + ".jpg");
if (file.exists()) {
file.delete();
}
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
uri = FileProvider.getUriForFile(this, "edu.qau.phone.fileprovider", file);
} else {
uri = Uri.fromFile(file);
}
intent.putExtra(MediaStore.EXTRA_OUTPUT, uri);
startActivityForResult(intent, TAKE);
}
public void pickPic() {
Intent intent = new Intent(Intent.ACTION_PICK);
intent.setData(MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(intent, PICK);
}
@TargetApi(23)
public void take() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (checkSelfPermission(Manifest.permission_group.STORAGE) == PackageManager.PERMISSION_GRANTED) {
takePic();
} else {
requestPermissions(new String[]{
Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
1);
}
} else takePic();
}
@TargetApi(23)
private void pick() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (checkSelfPermission(Manifest.permission_group.STORAGE) == PackageManager.PERMISSION_GRANTED) {
pickPic();
} else {
requestPermissions(new String[]{
Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE}, 2);
}
} else {
pickPic();
}
}
private ImageView createImageView() {
ImageView iv = new ImageView(this);
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(
getResources().getDimensionPixelOffset(R.dimen.preview_width),
getResources().getDimensionPixelOffset(R.dimen.preview_height));
layoutParams.setMargins(
0,
0,
getResources().getDimensionPixelOffset(R.dimen.preview_margin_right),
0);
iv.setLayoutParams(layoutParams);
return iv;
}
private boolean checkPermissionAllGranted(int[] grantResults) {
boolean granted = true;
for (int i = 0; i < grantResults.length; i++) {
if (grantResults[i] != PackageManager.PERMISSION_GRANTED) {
granted = false;
break;
}
}
return granted;
}
public void submit(View view) {
int netType = NetWorkUtils.getAPNType(this);
if (netType == 0) {
Toast.makeText(this, "无法连接到网络", Toast.LENGTH_LONG).show();
} else {
String temp = talkEditText.getText().toString();
Talk talk = new Talk();
talk.setTalk(temp);
talk.setList(list);
Gson gson = new Gson();
MultipartBody.Builder builder = new MultipartBody.Builder();
builder.addFormDataPart("json", gson.toJson(talk));
for (int i = 0; i < list.size(); i++) {
final Image image = list.get(i);
if (netType == 1) {
File uploadFile = new File(image.getPath());
builder.addFormDataPart("File" + i, uploadFile.getName(), RequestBody.create(JPEG, uploadFile));
} else {
RequestBody os = new RequestBody() {
@Nullable
@Override
public MediaType contentType() {
return JPEG;
}
@Override
public void writeTo(@NotNull BufferedSink sink) throws IOException {
ByteArrayOutputStream byteArrayOutputStream = ImageUtils.compressImage(image.getPath(), 300);
sink.write(byteArrayOutputStream.toByteArray());
byteArrayOutputStream.close();
byteArrayOutputStream.reset();
byteArrayOutputStream = null;
}
};
builder.addPart(Headers.of("Content-Disposition", "form-data; name=\"file" + i + "\""), os);
}
}
final Request request = new Request.Builder().url("http://192.168.43.146.8080/Server/file")
.post(builder.build()).build();
OkHttpUtils.CLIENT.newCall(request).enqueue(new okhttp3.Callback() {
@Override
public void onFailure(@NotNull Call call, @NotNull IOException e) {
Toast.makeText(MainActivity.this, "访问网络失败", Toast.LENGTH_LONG).show();
}
@Override
public void onResponse(@NotNull Call call, @NotNull Response response) throws IOException {
String result = response.body().string();
try {
JSONObject json = new JSONObject(result);
if ("success".equals(json.getString("result"))) {
handler.sendEmptyMessage(1);
} else {
handler.sendEmptyMessage(2);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
}
public void addImg(View view) {
if (count < 9) {
dialog.show();
} else {
Toast.makeText(this, "最多上传九张", Toast.LENGTH_LONG).show();
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (resultCode == Activity.RESULT_OK) {
count++;
image = createImageView();
group.addView(image, count - 1);
switch (requestCode) {
case TAKE:
Picasso.get()
.load(file)
.resizeDimen(R.dimen.preview_width, R.dimen.preview_height)
.placeholder(R.drawable.w)
.error(R.drawable.e)
.centerCrop()
.into(image);
Image imageFromTake = new Image();
imageFromTake.setName(file.getName());
imageFromTake.setPath(file.getAbsolutePath());
imageFromTake.setFlag(TAKE);
list.add(imageFromTake);
break;
case PICK:
Uri uri = data.getData();
String path = ImageUtils.getPath(this, uri);
if (path != null) {
file = new File(path);
Picasso.get()
.load(file)
.resizeDimen(R.dimen.preview_width, R.dimen.preview_height)
.centerCrop()
.into(image);
Image imageFromPick = new Image();
imageFromPick.setName(file.getName());
imageFromPick.setPath(file.getAbsolutePath());
imageFromPick.setFlag(TAKE);
list.add(imageFromPick);
}
break;
}
}
}
@Override
public void onConfigurationChanged(Configuration newConfig) {
super.onConfigurationChanged(newConfig);
}
@TargetApi(23)
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
switch (requestCode) {
case 1:
if (checkPermissionAllGranted(grantResults)) {
takePic();
}
break;
case 2:
if (checkPermissionAllGranted(grantResults)) {
pickPic();
}
break;
}
}
private class DialogAdapter extends BaseAdapter {
@Override
public int getCount() {
return 3;
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view;
if (position == 0 || position == 1) {
view = inflater.inflate(R.layout.dialog_item, parent, false);
} else {
view = inflater.inflate(R.layout.dialog_cancel, parent, false);
}
TextView tv = view.findViewById(R.id.dialog_item);
tv.setText(position == 0 ? "从相册选择" : position == 1 ? "拍照" : "取消");
return view;
}
}
}