#include<stdio.h>
#include<stdlib.h>
typedef int Item;
typedef struct avl_node* AVL;
struct avl_node
{
Item val;
struct avl_node* left;
struct avl_node* right;
int height;
};
static void terminate(const char* message);
int Max(int a, int b);
int height(AVL root);
AVL single_rotate_with_left(AVL root);
AVL single_rotate_with_right(AVL root);
AVL double_rotate_with_left(AVL root);
AVL double_rotate_with_right(AVL root);
AVL insert(AVL root, int n);
//错误处理函数
static void terminate(const char* message)
{
printf("%s\n", message);
exit(EXIT_FAILURE);
}
//求两者中较大的
int Max(int a, int b)
{
return a > b ? a : b;
}
//求树高
int height(AVL root)
{
if (root==NULL)
{
return -1;
}
else
{
return root->height;
}
}
//单左旋
//对root的右儿子的右子树进行一次插入
AVL single_rotate_with_left(AVL root)
{
AVL new_root;
new_root = root->right;
root->right = new_root->left;
new_root->left = root;
root->height = Max(height(root->left), height(root->right)) + 1;
new_root->height = Max(root->height, height(new_root->right) + 1);
return new_root;
}
//单右旋
//对root的左儿子的左子树进行一次插入
AVL single_rotate_with_right(AVL root)
{
AVL new_root;
new_root = root->left;
root->left = new_root->right;
new_root->right = root;
root->height = Max(height(root->left), height(root->right)) + 1;
new_root->height = Max(height(new_root->left), root->height) + 1;
return new_root;
}
//双左旋
//对root的右儿子的左子树进行一次插入
AVL double_rotate_with_left(AVL root)
{
root->left = single_rotate_with_right(root->right);
return single_rotate_with_left(root);
}
//双右旋
//对root的左儿子的右子树进行一次插入
AVL double_rotate_with_right(AVL root)
{
root->left = single_rotate_with_left(root->left);
return single_rotate_with_right(root);
}
//插入元素n
AVL insert(AVL root, Item n)
{
if (root==NULL)
{
root = malloc(sizeof(struct avl_node));
if (root==NULL)
{
terminate("Error in insert: create a root failed in malloc.");
}
else
{
root->val = n;
root->left = NULL;
root->right = NULL;
root->height = 0;
}
}
else if (n < root->val)
{
root->left = insert(root->left, n);
if (height(root->left) - height(root->right) == 2)
{
if (n < root->left->val)
{
root = single_rotate_with_right(root);
}
else
{
root = double_rotate_with_right(root);
}
}
}
else if (n > root->val)
{
root->right = insert(root->right, n);
if (height(root->right) - height(root->left) == 2)
{
if (n > root->right->val)
{
root = single_rotate_with_left(root);
}
else
{
root = double_rotate_with_left(root);
}
}
}
else
{
printf("n is in the AVL tree already.");
}
root->height = Max(height(root->left), height(root->right)) + 1;
return root;
}
AVL树(平衡二叉树)
最新推荐文章于 2024-09-11 01:30:06 发布
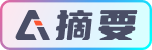