自转、跳动、闪烁、旋转等动态效果
loadDynamicBillboardByEntity (viewer: Cesium.Viewer, options: TBillboardOptions) {
const { image, position } = options
let imageWidth = 0
const imageWidthMin = 0
const imageWidthMax = 50
viewer?.entities.add({
position: position.toCartesian3(),
billboard: {
image: image,
width: new Cesium.CallbackProperty(() => {
return imageWidth
}, false),
verticalOrigin: Cesium.VerticalOrigin.BOTTOM,
heightReference: Cesium.HeightReference.RELATIVE_TO_GROUND,
},
})
viewer.scene.preRender.addEventListener(function (scene, time) {
const elapsedSeconds = time.secondsOfDay
imageWidth = Math.abs(Math.sin(elapsedSeconds)) * (imageWidthMax - imageWidthMin) + imageWidthMin
})
}
dynamicBillboardByPrimitive (viewer: Cesium.Viewer) {
const scene = viewer.scene
const clock = viewer.clock
const billboards = scene.primitives.add(new Cesium.BillboardCollection())
const imageWidth = 30
const imageHeight = 40
const billboard = billboards.add({
position: Cesium.Cartesian3.fromDegrees(70, 70, 10000),
image: '/public/bg_billboard.png',
scale: 0.5,
color: new Cesium.Color(1.0, 1.0, 1.0, 1.0),
rotation: 0.0,
imageSubRegion: new Cesium.BoundingRectangle(0, 0, imageWidth, imageHeight),
})
clock.multiplier = 1
scene.preRender.addEventListener(function (scene, time) {
const elapsedSeconds = time.secondsOfDay
const newLongitude = -75.59777 + 0.01 * elapsedSeconds
billboard.position = Cesium.Cartesian3.fromDegrees(newLongitude, 40.03883)
const alpha = Math.abs(Math.sin(elapsedSeconds))
billboard.color = new Cesium.Color(1.0, 1.0, 1.0, alpha)
billboard.rotation = elapsedSeconds * 0.1
billboard.scale = 1.0 + 0.5 * Math.sin(elapsedSeconds)
billboard.width = Math.abs(Math.sin(elapsedSeconds)) * imageWidth
})
}
加载GIF
import SuperGif from './libgif.js'
loadGifByEntity (viewer: Cesium.Viewer, options: TBillboardOptions) {
console.log('billboard loadGif', options)
const { image, position } = options
if (image) {
const gifDiv = document.createElement('div')
const gifImg = document.createElement('img')
gifImg.setAttribute('rel:animated_src', image)
gifImg.setAttribute('rel:auto_play', '1')
gifDiv.appendChild(gifImg)
const superGif = new SuperGif({ gif: gifImg })
superGif.load(function () {
viewer.entities.add({
position: position.toCartesian3(),
billboard: {
image: new Cesium.CallbackProperty(() => {
return superGif.get_canvas().toDataURL('image/png')
}, false),
scale: 0.1,
},
})
})
}
}
import SuperGif from './libgif.js'
loadGifByPrimitive (viewer: Cesium.Viewer) {
const url = '/public/img.gif'
const gifDiv = document.createElement('div')
const gifImg = document.createElement('img')
gifImg.setAttribute('rel:animated_src', url)
gifImg.setAttribute('rel:auto_play', '1')
gifDiv.appendChild(gifImg)
const superGif = new SuperGif({ gif: gifImg })
superGif.load(() => {
const scene = viewer.scene
const clock = viewer.clock
clock.multiplier = 1.5
const billboards = scene.primitives.add(new Cesium.BillboardCollection())
const billboard = billboards.add({
position: Cesium.Cartesian3.fromDegrees(30, 70, 1000000),
image: url,
})
let imageIndex = 0
const imgLength = superGif.get_length()
scene.preRender.addEventListener(function (scene, time) {
imageIndex = (time.secondsOfDay) % imgLength
superGif.move_to(imageIndex)
billboard.image = superGif.get_canvas().toDataURL('image/png')
})
})
}
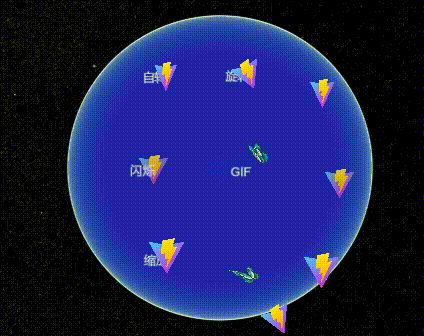