Circle and Points
Time Limit: 5000MS | Memory Limit: 30000K | |
Total Submissions: 6119 | Accepted: 2149 | |
Case Time Limit: 2000MS |
Description
You are given N points in the xy-plane. You have a circle of radius one and move it on the xy-plane, so as to enclose as many of the points as possible. Find how many points can be simultaneously enclosed at the maximum. A point is considered enclosed by a circle when it is inside or on the circle.
Fig 1. Circle and Points
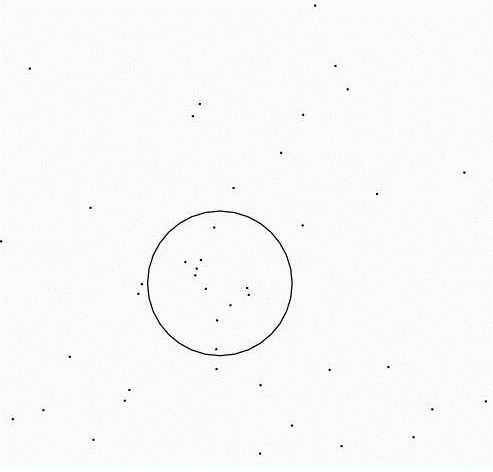
Fig 1. Circle and Points
Input
The input consists of a series of data sets, followed by a single line only containing a single character '0', which indicates the end of the input. Each data set begins with a line containing an integer N, which indicates the number of points in the data set. It is followed by N lines describing the coordinates of the points. Each of the N lines has two decimal fractions X and Y, describing the x- and y-coordinates of a point, respectively. They are given with five digits after the decimal point.
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0 <= Y <= 10.0. No two points are closer than 0.0001. No two points in a data set are approximately at a distance of 2.0. More precisely, for any two points in a data set, the distance d between the two never satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a data set are simultaneously very close to a single circle of radius one. More precisely, let P1, P2, and P3 be any three points in a data set, and d1, d2, and d3 the distances from an arbitrarily selected point in the xy-plane to each of them respectively. Then it never simultaneously holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0 <= Y <= 10.0. No two points are closer than 0.0001. No two points in a data set are approximately at a distance of 2.0. More precisely, for any two points in a data set, the distance d between the two never satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a data set are simultaneously very close to a single circle of radius one. More precisely, let P1, P2, and P3 be any three points in a data set, and d1, d2, and d3 the distances from an arbitrarily selected point in the xy-plane to each of them respectively. Then it never simultaneously holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
Output
For each data set, print a single line containing the maximum number of points in the data set that can be simultaneously enclosed by a circle of radius one. No other characters including leading and trailing spaces should be printed.
Sample Input
3 6.47634 7.69628 5.16828 4.79915 6.69533 6.20378 6 7.15296 4.08328 6.50827 2.69466 5.91219 3.86661 5.29853 4.16097 6.10838 3.46039 6.34060 2.41599 8 7.90650 4.01746 4.10998 4.18354 4.67289 4.01887 6.33885 4.28388 4.98106 3.82728 5.12379 5.16473 7.84664 4.67693 4.02776 3.87990 20 6.65128 5.47490 6.42743 6.26189 6.35864 4.61611 6.59020 4.54228 4.43967 5.70059 4.38226 5.70536 5.50755 6.18163 7.41971 6.13668 6.71936 3.04496 5.61832 4.23857 5.99424 4.29328 5.60961 4.32998 6.82242 5.79683 5.44693 3.82724 6.70906 3.65736 7.89087 5.68000 6.23300 4.59530 5.92401 4.92329 6.24168 3.81389 6.22671 3.62210 0
Sample Output
2 5 5 11
Source
题意:给定一些点,求单位圆可以覆盖的最多点数
题解:可知必定有2个或2个以上的点在圆上,则枚举2个点作圆上的点,然后求出一个方向的圆心,再枚举一次所有点是否在圆上,O(N^3)的做法就是这样。至于为什么只需求一个方向的圆心,可以画个图出来假象一下,另一个方向的圆必定可以向原方向移动,直至另外2个点落在圆上,所以这样就不必要重复枚举另外一个方向了。
至于O(N^2 logN)算法,是枚举以每一个点为圆心,作一个单位圆,然后求和其他点为圆心的单位圆的相交弧,这相当于一个角度范围,然后用类似扫描线的方法加入每个相交信息就可以了,注意,可以直接忽略角度超过了范围的考虑,因为总会有一个圆使这段角度不超过范围~
上面的
O(N^2 logN)算法依赖于2个单位圆如果相交,则在相交部分内任意选一点作为圆心
作单位圆都可以包含这2个圆心,换言之只需要看成对于被枚举的圆的圆弧上任取一点作为圆心作单位圆都可以包含这2个圆心,这就是要求相交弧的原因了~
O(N^3)
#include<stdio.h>
#include<math.h>
#define eps 1e-8
struct point{
double x,y;
}p[308],circle,vec;
int MAX(int x,int y){ return x>y?x:y; }
double dis(struct point p1,struct point p2)
{
return sqrt((p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y));
}
void get_circle(struct point p1,struct point p2)
{
double len=sqrt(1-dis(p1,p2)*dis(p1,p2)/4.0);
vec.x=p1.y-p2.y;
vec.y=p2.x-p1.x;
circle.x=(p1.x+p2.x)/2.0+vec.x*len/sqrt(vec.x*vec.x+vec.y*vec.y);
circle.y=(p1.y+p2.y)/2.0+vec.y*len/sqrt(vec.x*vec.x+vec.y*vec.y);
}
int main()
{
int i,j,k,n,res,cou;
while(scanf("%d",&n),n)
{
for(i=0;i<n;i++) scanf("%lf%lf",&p[i].x,&p[i].y);
res=1;
for(i=0;i<n;i++)
{
for(j=i+1;j<n;j++)
{
if(dis(p[i],p[j])>2.0) continue;
get_circle(p[i],p[j]);
for(cou=k=0;k<n;k++) if(dis(circle,p[k])<1.0+eps) cou++;
res=MAX(res,cou);
}
}
printf("%d\n",res);
}
return 0;
}
#include<stdio.h>
#include<stdlib.h>
#include<math.h>
#define eps 1e-8
struct point{
double x,y;
}p[308],circle,vec;
struct line{
double angle;
int in;
}l[308];
int MAX(int x,int y){ return x>y?x:y; }
double dis(struct point p1,struct point p2)
{
return sqrt((p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y));
}
int cmp(const void *a,const void *b)
{
struct line c=*(struct line *)a;
struct line d=*(struct line *)b;
if(c.angle==d.angle) return c.in>d.in?-1:1;
return c.angle<d.angle?-1:1;
}
int main()
{
int i,j,n,res,cou,all;
double angle,delta;
while(scanf("%d",&n),n)
{
for(i=0;i<n;i++) scanf("%lf%lf",&p[i].x,&p[i].y);
res=1;
for(i=0;i<n;i++)
{
for(all=j=0;j<n;j++)
{
if(i==j) continue;
if(dis(p[i],p[j])>2.0) continue;
angle=atan2(p[j].y-p[i].y,p[j].x-p[i].x);
delta=acos(dis(p[j],p[i])/2.0);
l[all].angle=angle-delta; l[all++].in=1;
l[all].angle=angle+delta; l[all++].in=0;
}
qsort(l,all,sizeof(l[0]),cmp);
for(cou=1,j=0;j<all;j++)
{
if(l[j].in) cou++;
else cou--;
res=MAX(res,cou);
}
}
printf("%d\n",res);
}
return 0;
}