截图
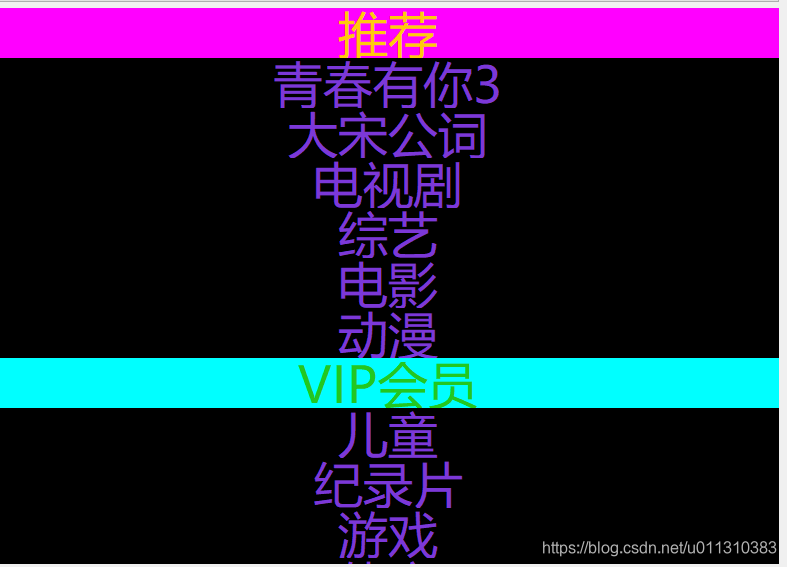
NavWidget.h
#ifndef NAVWIDGET_H
#define NAVWIDGET_H
#include <QWidget>
#include <QVBoxLayout>
#include <QPushButton>
#include <QPainter>
#include <QMouseEvent>
class NavWidget : public QWidget
{
Q_OBJECT
Q_PROPERTY(int currentIndex MEMBER m_CurrentIndex READ currentIndex WRITE setCurrentIndex)
Q_PROPERTY(QColor backgroundColor MEMBER m_CurrentBackgroundColor READ backgroundColor WRITE setBackgroundColor)
Q_PROPERTY(QColor textColor MEMBER m_TextColor READ textColor WRITE setTextColor)
Q_PROPERTY(QColor currentTextColor MEMBER m_CurrentTextColor READ currentTextColor WRITE setCurrentTextColor)
Q_PROPERTY(QColor currentBackgroundColor MEMBER m_CurrentBackgroundColor READ currentBackgroundColor WRITE setCurrentBackgroundColor)
Q_PROPERTY(QColor hoverTextColor MEMBER m_HoverTextColor READ hoverTextColor WRITE setHoverTextColor)
Q_PROPERTY(QColor hoverBackgroundColor MEMBER m_HoverBackgroundColor READ hoverBackgroundColor WRITE setHoverBackgroundColor)
Q_PROPERTY(int pixelSize MEMBER m_PixelSize READ pixelSize WRITE setPixelSize)
Q_PROPERTY(QString family MEMBER m_Family READ family WRITE setFamily)
public:
explicit NavWidget(QWidget *parent = nullptr);
int currentIndex() const;
QColor textColor() const;
QColor backgroundColor() const;
QColor currentTextColor() const;
QColor currentBackgroundColor() const;
QColor hoverTextColor() const;
QColor hoverBackgroundColor() const;
int pixelSize() const;
QString family() const;
void append(const QString str);
bool empty() const;
void removeAt(int i);
public slots:
void setCurrentIndex(int index);
void setBackgroundColor(QColor color);
void setTextColor(QColor color);
void setCurrentTextColor(QColor color);
void setCurrentBackgroundColor(QColor color);
void setHoverTextColor(QColor color);
void setHoverBackgroundColor(QColor color);
void setPixelSize(int size);
void setFamily(QString family);
signals:
void currentIndexChanged(int index);
protected:
void paintEvent(QPaintEvent *event);
void mousePressEvent(QMouseEvent *event);
void mouseMoveEvent(QMouseEvent *event);
private:
QStringList m_Item;
int m_HoverIndex;
QFont m_Font;
int m_OldIndex;
private:
int m_CurrentIndex;
QColor m_BackgroundColor;
QColor m_TextColor;
QColor m_CurrentBackgroundColor;
QColor m_CurrentTextColor;
QColor m_HoverTextColor;
QColor m_HoverBackgroundColor;
int m_PixelSize;
QString m_Family;
};
#endif
NavWidget.cpp
#include "navwidget.h"
NavWidget::NavWidget(QWidget *parent) : QWidget(parent)
{
m_CurrentIndex = 0;
m_HoverIndex = 0;
m_OldIndex = 0;
m_TextColor = QColor(255, 255, 255);
m_BackgroundColor = QColor(0, 0, 0);
m_CurrentTextColor = QColor(0, 255, 0);
m_CurrentBackgroundColor = QColor(0, 0, 0);
m_HoverTextColor = QColor(0, 255, 255);
m_HoverBackgroundColor = QColor(0, 0, 0);
m_PixelSize = 16;
m_Family = "宋体";
m_Font.setPixelSize(m_PixelSize);
m_Font.setFamily(m_Family);
m_Font.setWeight(QFont::Normal);
setMouseTracking(true);
}
int NavWidget::currentIndex() const
{
return m_CurrentIndex;
}
void NavWidget::setCurrentIndex(int index)
{
m_CurrentIndex = index;
}
QColor NavWidget::backgroundColor() const
{
return m_BackgroundColor;
}
void NavWidget::setBackgroundColor(QColor color)
{
m_BackgroundColor = color;
}
QColor NavWidget::textColor() const
{
return m_TextColor;
}
void NavWidget::setTextColor(QColor color)
{
m_TextColor = color;
}
QColor NavWidget::currentTextColor() const
{
return m_CurrentTextColor;
}
void NavWidget::setCurrentTextColor(QColor color)
{
m_CurrentTextColor = color;
}
QColor NavWidget::currentBackgroundColor() const
{
return m_CurrentBackgroundColor;
}
void NavWidget::setCurrentBackgroundColor(QColor color)
{
m_CurrentBackgroundColor = color;
}
QColor NavWidget::hoverTextColor() const
{
return m_HoverTextColor;
}
void NavWidget::setHoverTextColor(QColor color)
{
m_HoverTextColor = color;
}
QColor NavWidget::hoverBackgroundColor() const
{
return m_HoverBackgroundColor;
}
void NavWidget::setHoverBackgroundColor(QColor color)
{
m_HoverBackgroundColor = color;
}
int NavWidget::pixelSize() const
{
return m_PixelSize;
}
void NavWidget::setPixelSize(int size)
{
m_PixelSize = size;
m_Font.setPixelSize(size);
repaint();
}
QString NavWidget::family() const
{
return m_Family;
}
void NavWidget::setFamily(QString family)
{
m_Family = family;
m_Font.setFamily(family);
repaint();
}
void NavWidget::append(const QString str)
{
m_Item.append(str);
}
bool NavWidget::empty() const
{
return m_Item.empty();
}
void NavWidget::removeAt(int i)
{
m_Item.removeAt(i);
}
void NavWidget::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event)
QPainter painter(this);
painter.fillRect(0, 0, width(), height(), QBrush(m_BackgroundColor));
painter.setFont(m_Font);
painter.setPen(m_TextColor);
for (int i = 0; i < m_Item.count(); i++) {
painter.drawText(0, m_PixelSize * i, width(), m_PixelSize, Qt::AlignCenter, m_Item.at(i));
}
painter.setPen(m_CurrentTextColor);
painter.fillRect(0, m_PixelSize * m_CurrentIndex, width(), m_PixelSize, QBrush(m_CurrentBackgroundColor));
if (m_CurrentIndex < m_Item.count())
painter.drawText(0, m_PixelSize * m_CurrentIndex, width(), m_PixelSize, Qt::AlignCenter, m_Item.at(m_CurrentIndex));
painter.setPen(m_HoverTextColor);
painter.fillRect(0, m_PixelSize * m_HoverIndex, width(), m_PixelSize, QBrush(m_HoverBackgroundColor));
if (m_HoverIndex < m_Item.count())
painter.drawText(0, m_PixelSize * m_HoverIndex, width(), m_PixelSize, Qt::AlignCenter, m_Item.at(m_HoverIndex));
}
void NavWidget::mousePressEvent(QMouseEvent *event)
{
Q_UNUSED(event)
m_CurrentIndex = event->pos().y() / m_PixelSize;
if (m_Item.count() > 0 && m_CurrentIndex >= m_Item.count())
m_CurrentIndex = m_Item.count() - 1;
if (m_OldIndex != m_CurrentIndex) {
m_OldIndex = m_CurrentIndex;
currentIndexChanged(m_CurrentIndex);
}
repaint();
}
void NavWidget::mouseMoveEvent(QMouseEvent *event)
{
Q_UNUSED(event)
m_HoverIndex = event->pos().y() / m_PixelSize;
if (m_Item.count() > 0 && m_HoverIndex >= m_Item.count())
m_HoverIndex = m_Item.count() - 1;
repaint();
}