Problem 3: Shift Cipher
Write a C++ program which encrypts and decrypts a sequence of input characters using an
algorithm adapted from the Vignѐre cipher as described below.
We first consider the algorithm
to encrypt/decrypt a single character
:
To encrypt (decrypt) a letter
c
(within the alphabet A-Z or a-z) with a shift of
k
positions:
1. Let
x
be
c
's position in the alphabet (0 based), e.g., position of
B
is 1 and
position of
g
is 6.
2. For encryption, calculate
y
=
x
+
k
modulo 26;
for decryption, calculate
y
=
x
−
k
modulo 26.
3. Let
w
be the letter corresponding to position
y
in the alphabet. If
c
is in
uppercase, the encrypted (decrypted) letter is
w
in lowercase; otherwise, the
encrypted (decrypted) letter is
w
in uppercase.
A character which is not within the alphabet A-Z or a-z will remain unchanged under
encryption or decryption.
Example
. Given letter
B
and
k
= 3, we have
x
= 1,
y
= 1 + 3 mod 26 = 4, and
w
=
E
. As
B
is in uppercase, the encrypted letter is
e
.
Now, to encrypt/decrypt a sequence of characters:
The number of positions,
k
, used to shift a character is determined by a key
V
of
n
characters. For example, if
V
is a 4-character key 'C', 'O', 'M', 'P', and their positions in the
alphabet is 2, 14, 12 and 15, respectively. To encrypt a sequence of characters, we shift the
first character by +2 positions, the second by +14, the third by +12, the fourth by +15 and
repeat the key, i.e., we shift the fifth character by +2, the sixth by +14, until we encrypt all the
characters in the input sequence.
Example
. Consider the input sequence of characters 'H','e','l','l','o','W','o','r','l','d' and a
4-character key 'C','O','M','P':
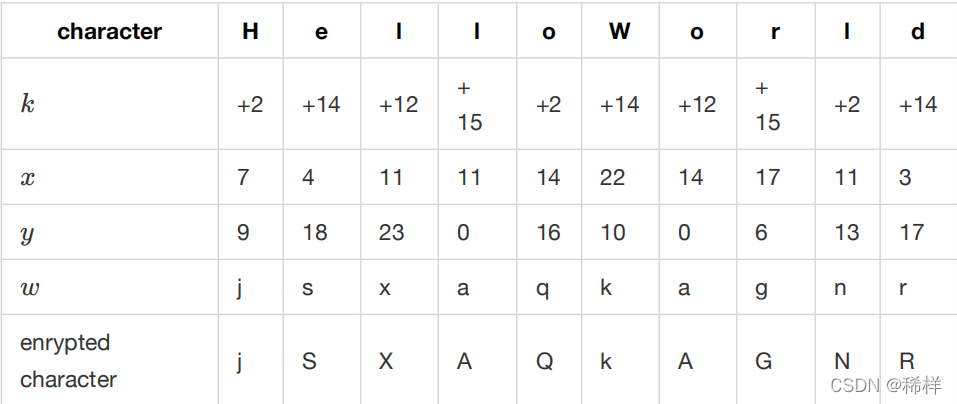
Note
: For decryption, we will shift by
−
k
instead of
k
.
Input:
• first line of input
s c
1
c
2
c
3
…
, where
◦
s
is either the character
e
for encryption, or the character
d
for
decryption
◦
c
1
c
2
c
3
…
is a sequence of space separated characters, ended by
!
, to be encrypted or decrypted
◦
you may assume that the input number of characters to encrypt or
decrypt (including
!
) is no greater than 50.
• second line of input
n v
1
v
2
…
v
n
, where
n
is the number of characters in the
key, and
v
1
,
v
2
,...,
v
n
are the characters in the key.
◦
you may assume that all characters in the key is in uppercase and the
number of characters in the key is at least one and no greater than
10.
Output:
• the encrypted/decrypted message ended by
!
. There should be no space
between two consecutive characters.
Requirement:
• You are provided with a template program
3.cpp
. Read the code in the template
carefully to see what have been provided for you.
• You can ONLY use the simple data types
char
,
bool
,
int
,
double
and
arrays. In other words, you are not allowed to use other data types or data
structures such as strings or STL containers (e.g., vectors), etc.
Sample Test Cases
User inputs are shown in
blue
.
3_1
e !
3 A B C
!
3_2
e a B c D e !
2 X Y
XzZbB!
3_3
d X z Z b B !
2 X Y
aBcDe!
3_4
e H e l l o E N G G 1 3 4 0 / C O M P 2 1 1 3 !
4 C O M P
jSXAQszvi1340/odod2113!
3_5
d f 3 O 3 B 3 I _ P Q R 3 _ Q K _ X 3 D I J K 3 V _ W B F 3 !
9 C O D E I S F U N
D3l3t3d_cod3_is_d3bugg3d_cod3!