桶的触发器
using UnityEngine;
public class EggCollider : MonoBehaviour
{
void OnTriggerEnter(Collider co)
{
if (co.tag == "Egg")
{
ObjectPool.PushEgg(co.gameObject);
Camera.main.GetComponent<GetEgg>().Clearing(false);
}
}
}
蛋的移动
using UnityEngine;
public class EggMove : MonoBehaviour
{
Vector3 pos;
void Update()
{
pos = transform.position;
pos.y -= Time.deltaTime * GetEgg.eggMoveSpeed;
transform.position = pos;
}
}
对象池?
using System.Collections.Generic;
using UnityEngine;
public class ObjectPool : MonoBehaviour
{
static Queue<GameObject> pool = new Queue<GameObject>();
static GameObject egg;
static Vector3 _position;
static Quaternion _rotation;
static Transform tran;
void Awake()
{
tran = transform;
egg = Resources.Load("GetEgg/Egg") as GameObject;
_position = egg.transform.position;
_rotation = egg.transform.rotation;
}
public static void GetObject()//得到一个对象
{
GameObject _egg = null;
if (pool.Count >= 5)
{
_egg = pool.Dequeue();
}
else
{
_egg = Instantiate(egg);
_egg.AddComponent<EggMove>();
_egg.transform.SetParent(tran);
pool.Enqueue(_egg);
}
GetEgg.eggMoveSpeed += 0.2f;
_egg.transform.position = new Vector3(Random.Range(-14, 14), 0, -20);
_egg.SetActive(true);
}
public static void PushEgg(GameObject obj)//回收一个对象
{
obj.transform.position = _position;
obj.transform.rotation = _rotation;
obj.SetActive(false);
if (pool.Contains(obj))
return;
pool.Enqueue(obj);
}
}
地面上的触发器
using UnityEngine;
public class PlaneCollider : MonoBehaviour
{
void OnTriggerEnter(Collider co)
{
if (co.tag == "Egg")
{
ObjectPool.PushEgg(co.gameObject);
Camera.main.GetComponent<GetEgg>().Clearing(true);
}
}
}
计时器
看我上一篇文章
http://blog.csdn.net/u013452440/article/details/64441095
其他。。。
using UnityEngine;
using UnityEngine.UI;
using System.Collections.Generic;
public class GetEgg : MonoBehaviour
{
GameObject player;
int score = 0;
int maxScore;
int minScore;
int lose = 0;
Text showScore;
Text showLose;
GameObject showClearing;
float nextTime;
float oriSpeed;
float shift;
public static float eggMoveSpeed;
GameObject panelScore;
List<int> scoreList = new List<int>();
List<int> timeList = new List<int>();
public List<GameObject> obj = new List<GameObject>();
GameObject scoreView;
void Start()
{
scoreView = GameObject.Find("Scroll View");
for (int index = 0; index < scoreView.transform.GetChild(0).GetChild(0).childCount; index++)
{
obj.Add(scoreView.transform.GetChild(0).GetChild(0).GetChild(index).gameObject);
}
player = GameObject.Find("Player");
player.AddComponent<EggCollider>();
panelScore = Resources.Load("GetEgg/ScorePanel") as GameObject;
showScore = GameObject.Find("ShowScore").GetComponent<Text>();
showLose = GameObject.Find("ShowLose").GetComponent<Text>();
showClearing = GameObject.Find("ShowClearing");
showScore.text = "Score:0";
showLose.text = "Lose:0";
Button reset = GameObject.Find("ResetButton").GetComponent<Button>();
reset.GetComponentInChildren<Text>().text = "重试";
reset.onClick.AddListener(delegate { ResetGame(); });
Button exit = GameObject.Find("ExitButton").GetComponent<Button>();
exit.GetComponentInChildren<Text>().text = "Exit";
exit.onClick.AddListener(delegate { ExitGame(); });
Button sv = GameObject.Find("ScoreViewButton").GetComponent<Button>();
sv.GetComponentInChildren<Text>().text = "排行榜";
sv.onClick.AddListener(delegate { ScoreListState(); });
showClearing.SetActive(false);
scoreView.SetActive(false);
ResetGame();
}
float moveSpeed;
Vector3 move;
float minX = -14f;
float maxX = 14f;
void Update()
{
move.x += Input.GetAxis("Horizontal") * Time.deltaTime * moveSpeed;
if (move.x < minX)
move.x = maxX;
else if (move.x > maxX)
move.x = minX;
player.transform.position = move;
if (nextTime < oriSpeed)
nextTime += Time.deltaTime;
else
{
nextTime -= oriSpeed;
oriSpeed *= shift;
if (oriSpeed < 0.5f)
oriSpeed = 0.5f;
else if (oriSpeed > 3f)
oriSpeed = 3f;
ObjectPool.GetObject();
}
}
public void Clearing(bool isLose)
{
if (isLose)
{
showLose.text = "Lose:" + ++lose;
if (lose >= 10)
{
if (score > maxScore)
{
maxScore = score;
showClearing.GetComponentInChildren<Text>().text = "不错哟,你又进步了";
}
else if (score < minScore)
{
minScore = score;
showClearing.GetComponentInChildren<Text>().text = "又偷懒了吧你";
}
else
{
showClearing.GetComponentInChildren<Text>().text = "怎么又挂了,我想静静...";
}
scoreList.Add(score);
timeList.Add(Camera.main.GetComponent<TimeCount>().CurrentSecond);
Time.timeScale = 0;
showClearing.SetActive(true);
}
}
else
showScore.text = "Score:" + ++score;
}
void ResetGame()
{
lose = 0;
score = 0;
showLose.text = "Lose:" + lose;
showScore.text = "Score:" + score;
eggMoveSpeed = 10;
shift = 0.98f;
nextTime = 0;
oriSpeed = 2;
moveSpeed = 12;
move = new Vector3(0, -30f, -20f);
showClearing.SetActive(false);
Camera.main.GetComponent<TimeCount>().ResetTime();
Transform tran = GameObject.Find("ObjectPool").transform;
for (int index = 0; index < tran.childCount; index++)
tran.GetChild(index).gameObject.SetActive(false);
Time.timeScale = 1;
}
void ExitGame()
{
Application.Quit();
}
bool isOpen = true;
void ScoreListState()
{
if (isOpen)
{
Time.timeScale = 0;
scoreList.Sort();
Vector2 pos = new Vector2(0, 0);
int shift = -35;
for (int count = 0; count < 10; count++)
{
pos.y += shift;
int mIndex = scoreList.Count - count - 1;
obj[count].transform.GetChild(0).GetComponentInChildren<Text>().text = (count + 1).ToString();
if (count < scoreList.Count)
{
obj[count].SetActive(true);
obj[count].transform.GetChild(1).GetComponentInChildren<Text>().text = timeList[mIndex] + "秒钟接了" + scoreList[mIndex] + "个!";
}
else
obj[count].SetActive(false);
}
}
else
{
Time.timeScale = 1;
}
scoreView.SetActive(isOpen);
isOpen = !isOpen;
}
}
结果
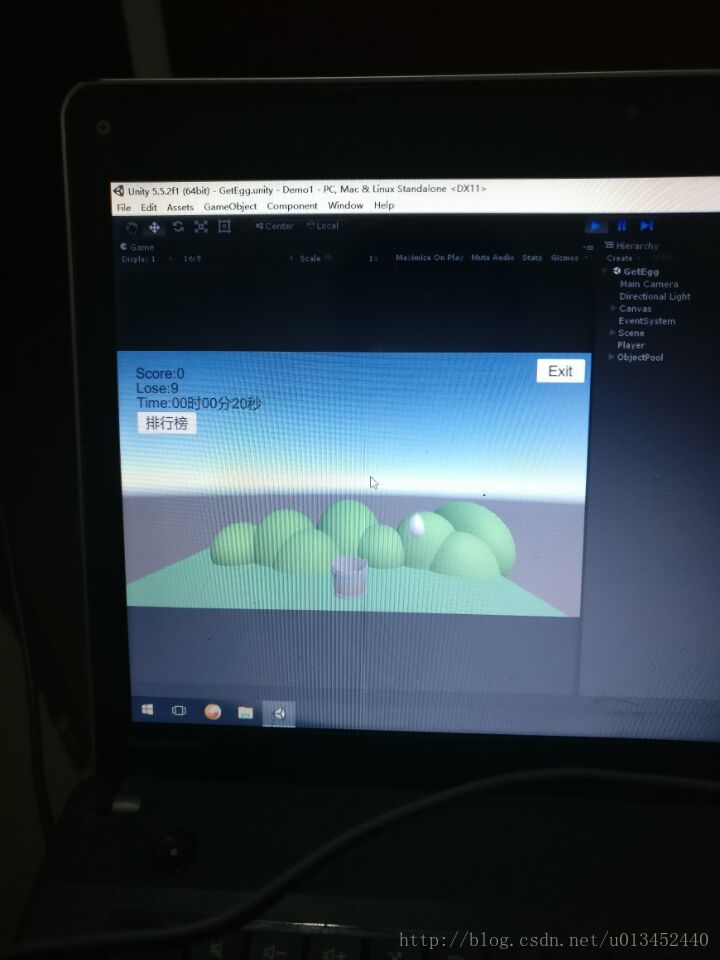