<html>
<head></head>
<body>
<canvas class="canvas" style="background:#fff"></canvas>
<script type ="text/javascript">
var S = {
start: function(){
Draw.init();
var dots = this.createPoint(this.Rectangle(30,20));
Draw.loop(function(){
for(var i = 0; i < dots.length;i++){
dots[i].render();
}
});
},
Rectangle: function(w,h){
var gap = 13, points = [],width = gap * w,height = gap * h;
for(var y = 0; y < height ; y+=gap){
for(var x = 0; x < width ; x+=gap){
points.push(new Point({
x:x,
y:y,
}));
}
}
return {points:points,w:width,h:height};
},
createPoint: function(p){
var dots = [];
var size = p.points.length,s = 0;
for(var n = 0; n < size; n++){
dots.push(new Dot(0,0));
}
while(size --){
dots[s].fillD(new Point({
x:p.points[s].x +5,
y:p.points[s].y +5
}));
s++;
}
return dots;
},
};
Point = function(args){
this.x = args.x;
this.y = args.y;
this.z = args.z;
};
Dot = function(x,y){
this.p = new Point({
x:x,
y:y,
z:5
});
this.e = 0.05;
this.d = [];
};
Dot.prototype = {
distance: function(){
var dx = this.p.x - this.d[0].x,
dy = this.p.y - this.d[0].y,
dl = Math.sqrt(dx * dx + dy * dy),
e = this.e * dl;
if(dl > 1){
this.p.x -= dx / dl * e;
this.p.y -= dy / dl * e;
}
else {
this.p.x = this.p.x;
this.p.y = this.p.y;
}
},
fillD: function(p){
this.d.push(p);
},
render: function(){
this.distance();
Draw._draw(this.p);
}
};
Draw =(function(){
var canvas,context,loopfn,requestFrame = window.requestAnimationRequest ||
window.webkitRequestAnimationRequest ||
window.mozRequestAnimationRequest ||
window.oRequestAnimationRequest ||
window.msRequestAnimationRequest ||
function(callback){
window.setTimeout(callback,1000/60);
};
return {
init: function(){
canvas = document.querySelector('.canvas');
context = canvas.getContext('2d');
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
},
clearFrame: function(){
context.clearRect(0,0,canvas.width,canvas.height);
},
_draw: function(p){
context.fillStyle = '#000';
context.beginPath();
context.arc(p.x,p.y,p.z,0,2 * Math.PI);
context.closePath();
context.fill();
},
loop:function(fn){
loopfn = !loopfn?fn:loopfn;
this.clearFrame();
loopfn();
requestFrame.call(window,this.loop.bind(this));
}
}
}()) ;
S.start();
</script>
</body>
</html>
散点形成矩形
最新推荐文章于 2021-10-27 09:51:14 发布
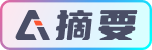