#include<GL/glut.h>
#include<stdio.h>
#include<stdlib.h>
#define LEFT_EDGE 1
#define RIGHT_EDGE 2
#define BOTTOM_EDGE 4
#define TOP_EDGE 8
void LineGL(int x0,int y0,int x1,int y1)
{
glBegin(GL_LINES);
glColor3f(1.0f,0.0f,0.0f); glVertex2f(x0,y0);
glColor3f(0.0f,1.0f,0.0f); glVertex2f(x1,y1); //虽然两个点的颜色设置不同,但是最终线段颜色是绿色
glEnd(); //因为glShadeModel(GL_FLAT)设置为最后一个顶点的颜色
} //决定整个图元的颜色。
struct Rectangle
{
float xmin,xmax,ymin,ymax;
};
Rectangle rect;
int x0,y0,x1,y1;
int CompCode(int x,int y,Rectangle rect)
{
int code=0x00; //此处是二进制
if(y<rect.ymin)
code=code|4;
if(y>rect.ymax)
code=code|8;
if(x>rect.xmax)
code=code|2;
if(x<rect.xmin)
code=code|1;
return code;
}
int cohensutherlandlineclip(Rectangle rect,int &x0,int &y0,int &x1,int &y1)
{
int accept,done;
float x,y;
accept=0;
done=0;
int total=0,flag=0; //total与flag是标志
int code0,code1,codeout;
code0=CompCode(x0,y0,rect);
printf("code0=%d\n",code0);
code1=CompCode(x1,y1,rect);
printf("code1=%d\n",code1);
do{
if(!(code0|code1)) //code0&code1=0000B,线段完全在窗口内部,全部显示
{
accept=1;
done=1;
}
else if(code0 & code1)
{
done=1;flag=1;
}
else
{
if(code0!=0)
codeout=code0;
else
codeout=code1;
if(codeout&LEFT_EDGE)
{
y=y0+(y1-y0)*(rect.xmin-x0)/(x1-x0);
x=(float)rect.xmin;
}
else if(codeout&RIGHT_EDGE)
{
y=y0+(y1-y0)*(rect.xmax-x0)/(x1-x0);
x=(float)rect.xmax;
}
else if(codeout&BOTTOM_EDGE)
{
x=x0+(x1-x0)*(rect.ymin-y0)/(y1-y0);
y=(float)rect.ymin;
OpenGL编码剪裁算法Cohen-Surtherland算法
最新推荐文章于 2024-09-24 11:05:42 发布
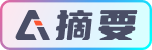