Collection Views didn’t exactly make their way into SwiftUI during WWDC 2020, but that didn’t stop UICollectionView
from receiving some powerful new updates.
在WWDC 2020期间,Collection View并未完全进入SwiftUI,但这并未阻止UICollectionView
接收一些强大的新更新。
CompositionalLayouts
and DiffableDataSources
were introduced in iOS 13 CollectionViews
and brought more flexibility in constructing layouts and data sources for our UICollectionView
.
在iOS 13 CollectionViews
中引入了CompositionalLayouts
和DiffableDataSources
它们为UICollectionView
构造布局和数据源提供了更大的灵活性。
With iOS 14, there are three new additions to the CollectionView APIs for layouts, data sources, and cells — the three pillars of constructing collection views:
在iOS 14中,为布局,数据源和单元格的CollectionView API新增了三项功能-构建集合视图的三大Struts:
Lists
are a new addition toCompositionalLayouts
and bring aUITableView
-like appearance inCollectionViews
.Lists
是CompositionalLayouts
的新增功能,并在CollectionViews
带来UITableView
的外观。UICollectionView.CellRegistration
lets you configure cells in a completely different way using new Configuration APIs. Also, there’s a newUICollectionViewListCell
concrete class that provides cells with list-like content styling by default.UICollectionView.CellRegistration
使您可以使用新的Configuration API以完全不同的方式配置单元。 此外,还有一个新的UICollectionViewListCell
具体类,默认情况下为单元格提供类似列表的内容样式。- Diffable Data Source now includes Section Snapshots to allow more updating data on a per-section basis. This is useful in building Outlined Styled lists, a new hierarchical design introduced in iOS 14. 差异数据源现在包括分区快照,以允许在每个分区的基础上进行更多更新。 这对于构建大纲样式列表很有用,这是iOS 14中引入的新分层设计。
In the next few sections, we’ll discuss the first two additions. Let’s see how to construct layouts and cells in UICollectionView
in the new iOS 14 way.
在接下来的几节中,我们将讨论前两个补充。 让我们看看如何以新的iOS 14方式在UICollectionView
中构造布局和单元格。
iOS 14单元配置和注册 (iOS 14 Cell Configuration and Registration)
iOS 14 introduces a brand new Cell Configuration API for configuring our CollectionView and TableView cells.
iOS 14引入了全新的单元配置API,用于配置我们的CollectionView和TableView单元。
This means that we don’t need to set properties directly on the UITableViewCell
and UICollectionViewCell
like we did earlier.
这意味着我们不需要像前面那样直接在UITableViewCell
和UICollectionViewCell
上设置属性。
The new configuration API lets us use a content configuration to set the cell’s content and styling or update it based on different states:
使用新的配置API,我们可以使用内容配置来设置单元格的内容并根据不同的状态对其样式或进行更新:
For UICollectionViews
, we have a new UICollectionViewListCell
. We can directly fetch its default configuration in the following way:
对于UICollectionViews
,我们有一个新的UICollectionViewListCell
。 我们可以通过以下方式直接获取其默认配置:
var content = listCell.defaultContentConfiguration()
In doing so, we’re able to get rid of using cell identifiers and the obligatory if let
and guard let
statements we used to write to ensure that the cell was registered.
这样一来,我们就可以摆脱使用单元格标识符的使用,而不必使用我们过去用来确保单元格已注册的if let
和guard let
语句。
More importantly, we’re not directly accessing the cell’s label and image property anymore, which allows us to compose configurations that can be used across TableView
, CollectionView
, and custom cells.
更重要的是,我们不再直接访问单元格的label和image属性,这使我们能够构成可在TableView
, CollectionView
和自定义单元格中使用的配置。
Aside from contentConfiguration
, there’s also a backgroundConfiguration
that we can leverage to set background appearance properties and also a leadingSwipeActionsConfiguration
and trailingSwipeActionsConfiguration
to easily embed UITableView
-like swipe behavior directly in our UICollectionView
cell implementation.
除了contentConfiguration
,还有一个backgroundConfiguration
,我们可以利用这些设置背景外观属性,也是一个leadingSwipeActionsConfiguration
和trailingSwipeActionsConfiguration
轻松嵌入UITableView
在我们的直接般滑动操作UICollectionView
单元实现。
The new UICollectionView.CellRegistration
structure that we just created automatically takes care of cell registration when passed inside the dequeueConfiguredReusableCell
. You don’t need to register cells using identifiers anymore.
我们刚刚创建的新UICollectionView.CellRegistration
结构在传递到dequeueConfiguredReusableCell
内时会自动处理单元格注册。 您不再需要使用标识符来注册单元格。
iOS 14 CollectionViews的新列表布局 (iOS 14 New List Layouts for CollectionViews)
Taking a cue from the UICollectionViewListCell
we discussed in the previous section, we now have a new List Layout built on top of compositional layouts.
从我们在上一节中讨论过的UICollectionViewListCell
一个提示,现在我们有了一个在合成布局之上构建的新的List Layout。
All it takes to quickly set up this layout is passing in the UICollectionViewListConfiguration
, which could be set as grouped
, insetGrouped
, sideBar
, and sideBarPlain
in addition to the following plain appearance:
所有需要快速建立这种布局是通过在UICollectionViewListConfiguration
,这可以被设置为grouped
, insetGrouped
, sideBar
和sideBarPlain
除了以下朴实无华的外表:
The good thing about the new Lists
support in UICollectionView
is that it provides out-of-the-box support for self-sizing cells.
UICollectionView
新的Lists
支持的UICollectionView
在于,它为自定义大小的单元格提供了开箱即用的支持。
We can also create lists on a per-section basis by passing in the NSCollectionSectionLayout.list
within the compositional layout.
我们也可以通过在合成布局中传入NSCollectionSectionLayout.list
按节创建列表。
Customizing header
and footer
for lists in UICollectionView
is a bit different from what we did in UITableView
.
自定义UICollectionView
列表的header
和footer
与我们在UITableView
中UICollectionView
的有些不同。
You’d need to invoke the headerMode
or footerMode
on the list configuration in the following way:
您需要通过以下方式在列表配置上调用headerMode
或footerMode
:
config.headerMode = .supplementary
config.footerMode = .supplementary
Subsequently, you’d need to provide the view by invoking supplementaryViewProvider
on the Diffable Data Source, wherein you can set the dequeueConfiguredReusableSupplementary
function and pass in the header configuration.
随后,你需要通过调用以提供视图supplementaryViewProvider
上Diffable数据源,其特征在于可以设置dequeueConfiguredReusableSupplementary
函数并传递在头标配置。
Now let’s combine the new features above (Cell Configuration API, List Layout, and Registrations).
现在,让我们结合上面的新功能(单元配置API,列表布局和注册)。
We’ve set up a simple UICollectionView
in our UIViewController
, as shown below:
我们在UIViewController
设置了一个简单的UICollectionView
,如下所示:
Upon running the application in a simulator, you’d get the following output:
在模拟器中运行该应用程序后,您将获得以下输出:
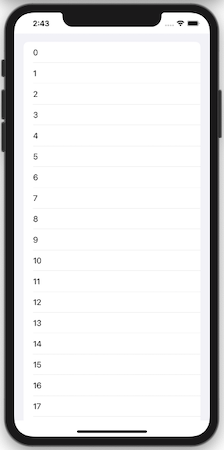
结论 (Conclusion)
To sum up:
总结一下:
iOS 14 introduced a new
CellRegistration
technique that doesn’t require registration using cell identifiers.iOS 14引入了新的
CellRegistration
技术,该技术不需要使用单元格标识符进行注册。A new configuration API encapsulates the cell’s content and background view properties.
UICollectionViewListCell
provides us with a default configuration that can be set and updated based on states.新的配置API封装了单元格的内容和背景视图属性。
UICollectionViewListCell
为我们提供了默认配置,可以根据状态进行设置和更新。- Customizations such as swipe actions and setting accessories can now be done directly when setting the cell configuration, thereby encouraging us to keep a single source of truth. 现在,可以在设置单元格配置时直接进行自定义操作,例如滑动动作和设置附件,从而鼓励我们保留唯一的事实来源。
The new lists for
UICollectionView
are aUITableView
lookalike and can be composed easily by specifying the layout and configuration (it can be on a per-section basis too) with appropriate header and footers.UICollectionView
的新列表UICollectionView
UITableView
,可以通过使用适当的页眉和页脚指定布局和配置(也可以基于每个部分)来轻松组成。
We managed to quickly combine the things we’ve learned to build a simple UICollectionView
in a declarative form. It’s time to say goodbye to cell item identifiers.
我们设法快速地将我们学到的东西结合起来,以声明的形式构建一个简单的UICollectionView
。 现在该告别单元格项目标识符了。
In the next article, we’ll look at what’s new in Diffable Data Sources for iOS 14. Stay tuned and thanks for reading.
在下一篇文章中,我们将研究iOS 14的可扩散数据源中的新增功能。敬请关注,并感谢您的阅读。
翻译自: https://medium.com/better-programming/whats-new-in-ios-14s-uicollectionview-3c02b63f7a0f