In a world were SwiftUI gets all the talk when it comes to building UI for iOS apps, UIKit got some surprising shout outs during the WWDC 2020, one of which was the “Modern cell configuration” presentation, where we saw some great updates to UITableViewCell coming our way, starting iOS 14. If you’ve already worked with the new “appearance states” of UINavigationBar and UITabbar in iOS 13, this should feel like a seamless transition because Apple seems to bring the same approach to UITableViewCell as well.
在世界上,SwiftUI在为iOS应用程序构建UI方面广为人知,在WWDC 2020期间,UIKit发出了令人惊讶的喊叫声,其中之一就是“现代单元配置”演示文稿,其中我们看到了UITableViewCell的一些重大更新首先,从iOS 14开始。如果您已经在iOS 13中使用过UINavigationBar和UITabbar的新“外观状态”,那应该感觉像是无缝过渡,因为Apple似乎也为UITableViewCell带来了相同的方法。
构型 (Configurations)
UITableViewCell in iOS 14 comes with Configurations. Apple defines a configuration as “the appearance of a view for a specific state”. If you’ve worked with complex tableview cells, there is a pretty good chance that you stumbled upon some lagging behavior at one point or another. As per Tyler Fox, one of the UIKit team engineers, configurations are lightweight, more efficient and they should help smoothing the scrolling animation and eliminate previous laggy behaviour. One of the reasons for that is that configurations are value types, so they are not expensive to create.
iOS 14中的UITableViewCell带有Configurations 。 Apple将配置定义为“特定状态的视图外观”。 如果您使用的是复杂的表格单元格,则很有可能偶然发现某一点或另一点的滞后行为。 根据UIKit团队工程师之一的Tyler Fox的说法,配置轻巧,效率更高,它们应有助于平滑滚动动画并消除以前的缓慢行为。 这样做的原因之一是配置是值类型,因此创建它们并不昂贵。
Each configuration can be implemented based on a state with the help of UICellConfigurationState
. By default, UICellConfigurationState
has 13 states that you can play with to take your UITableViewCell
or UICollectionViewCell
game to the next level. Some are inherited from UIViewConfigurationState
(which can be used for configuring states for your header and footer), but you can also add your own custom states.
可以借助UICellConfigurationState
基于状态来实现每个配置。 默认情况下, UICellConfigurationState
具有13种状态,您可以使用这些状态将UITableViewCell
或UICollectionViewCell
游戏提升到一个新的水平。 有些继承自UIViewConfigurationState
(可用于配置页眉和页脚的状态),但是您也可以添加自己的自定义状态。
To be able to set up our configurations we will have to use the brand new updateConfiguration(using state: UICellConfigurationState)
function on UITableViewCell. This method will be called before the cell is displayed and after any configuration state changes. You can also enforce its execution by calling setNeedsUpdateConfiguration()
on your cell.
为了能够设置我们的配置,我们将必须在UITableViewCell上updateConfiguration(using state: UICellConfigurationState)
全新的updateConfiguration(using state: UICellConfigurationState)
函数。 在显示单元之前以及任何配置状态更改之后,将调用此方法。 您还可以通过在单元格上调用setNeedsUpdateConfiguration()
来强制执行它。
In our cell class, we will override this function and start updating our configurations in 3 easy steps:
在我们的单元格类中,我们将覆盖此功能,并通过3个简单的步骤开始更新配置:
override func updateConfiguration(using state: UICellConfigurationState) {
// start with a fresh configuration
var content = self.defaultContentConfiguration().updated(for: state)
// define it dependent of the configuration state
if state.isSelected {
content.text = "Selected"
content.imageProperties.tintColor = .green
content.image = UIImage(systemName: "checkmark")
} else {
content.text = "Unselected"
content.textProperties.color = .darkGray
content.image = UIImage(systemName: "applelogo")
}
// apply it to your cell
self.contentConfiguration = content
}
Always start with a fresh configuration. For content configuration, you can do this by calling the cell’s
defaultContentConfiguration()
function. What callingupdated(for: state)
does is asking for the default configuration for the specific state.始终以全新的配置开始。 对于内容配置,可以通过调用单元格的
defaultContentConfiguration()
函数来实现。 调用updated(for: state)
所做的是要求特定状态的默认配置。Configure each state according to your preference. For our scope, just configuring the
isSelected
state should be enough to understand how configurations work. Obviously, you should go for aswitch
if you will work with more than just these 2 states.根据您的喜好配置每个状态。 对于我们的范围,仅配置
isSelected
状态应该足以了解配置如何工作。 显然,如果您要处理的不仅仅是这两种状态,就应该进行switch
。- Apply your new configuration to the cell for rendering. 将新配置应用于单元以进行渲染。
As you can see we are not modifying the cell itself but rather a new configuration state that we later apply to the cell to render.
如您所见,我们不是在修改单元格本身,而是在稍后将其应用于要渲染的单元格的新配置状态。
There are 2 types of configurations:
有两种配置类型:
Content configurations. As the name suggests, content configurations can help you manipulate the content of the cell like image, text, secondary text, layout metrics and behaviors.
内容配置 。 顾名思义,内容配置可以帮助您操纵单元格的内容,例如图像,文本,辅助文本,布局指标和行为。
Background configurations can help with the manipulation of background color, visual effect, stroke, insets and corner radius. All cells will inherit a default background configuration even if we don’t specify one.
背景配置可以帮助操纵背景颜色,视觉效果,笔触,插图和拐角半径。 即使不指定,所有单元都将继承默认的后台配置。
Now let’s see how we can update the background of our cell as well. You probably guessed it already, with the same 3 steps:
现在,让我们看看如何更新单元格的背景。 您可能已经猜到了,只需执行以下3个步骤:
override func updateConfiguration(using state: UICellConfigurationState) {
var content = self.defaultContentConfiguration().updated(for: state)
// start with the default configuration for a list grouped cell style
var background = UIBackgroundConfiguration.listGroupedCell()
// define it dependent of the configuration state
if state.isSelected {
content.text = "Selected"
content.imageProperties.tintColor = .green
content.image = UIImage(systemName: "checkmark")
background.backgroundColor = .systemGray6
} else {
content.text = "Unselected"
content.textProperties.color = .darkGray
content.image = UIImage(systemName: "applelogo")
background.backgroundColor = .white
}
// apply it to the cell
self.backgroundConfiguration = background
self.contentConfiguration = content
}
Start with a new configuration. Here we use a new way of getting a configuration, by calling a default configuration for a preferred style. To do that we used
UIBackgroundConfiguration
which will give us the default iOS background configuration for thelistGroupedCell()
style. If we would want to do this for the content configuration as well we could useUIListContentConfiguration
.从新配置开始。 在这里,我们通过调用首选样式的默认配置来使用一种获取配置的新方法。 为此,我们使用了
UIBackgroundConfiguration
,它将为我们提供listGroupedCell()
样式的默认iOS背景配置。 如果我们也想对内容配置执行此操作,则可以使用UIListContentConfiguration
。- Define each state. 定义每个状态。
- Apply the configuration to the cell for rendering. 将配置应用于单元以进行渲染。
Now let’s see the result:
现在让我们看一下结果:
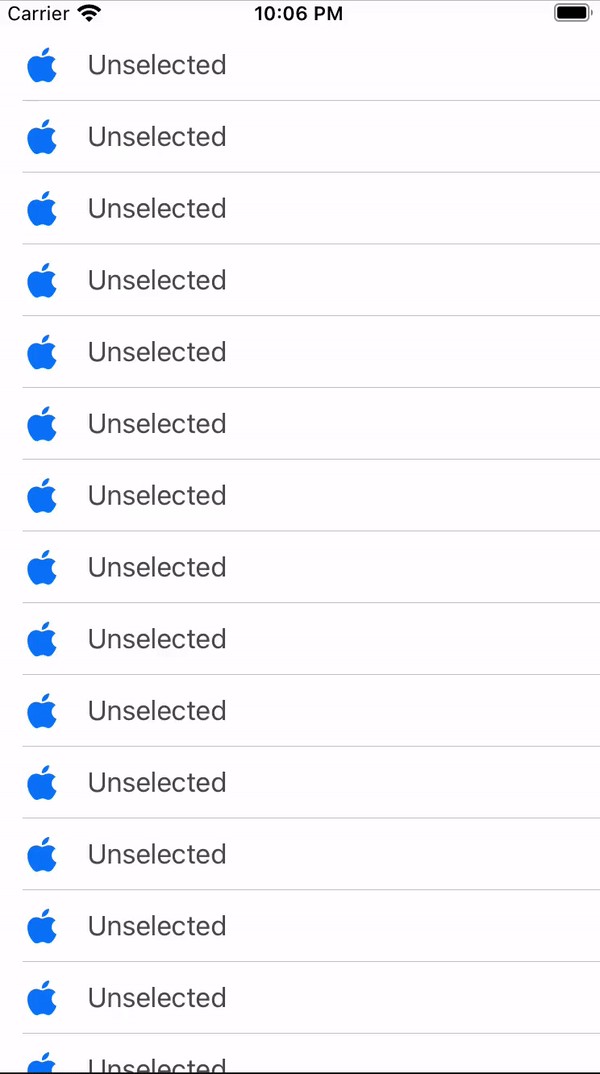
Each cell is now configured according to its state and updates automatically once the state changes.
现在,每个单元根据其状态进行配置,并在状态更改后自动更新。
One thing to notice here is the image of the cell. We change its tint color on the isSelected
state, but we don’t mention it for the other state and it still changes back its color. Because we start with a fresh configuration, the color is not being persisted through both states, it just uses the default one that came with the defaultContentConfiguration()
method.
这里要注意的一件事是细胞的图像。 我们在isSelected
状态下更改其颜色颜色,但在其他状态下未提及该颜色,但它仍会变回其颜色。 因为我们从全新的配置开始,所以颜色不会在两种状态下都保持defaultContentConfiguration()
,因此它仅使用defaultContentConfiguration()
方法随附的默认颜色。
It is important to know that applying a background configuration will override any changes you make to the backgroundColor
and backgroundView
properties of the cell and set them to nil
. The same behavior applies the other way around, so if you set the backgroundColor
property on a cell, the background configuration will become nil
重要的是要知道,应用背景配置将覆盖您对单元格的backgroundColor
和backgroundView
属性所做的任何更改,并将它们设置为nil
。 相同的行为反之亦然,因此,如果在单元格上设置backgroundColor
属性,则背景配置将变为nil
This is also the case for content configuration. It will override your imageView
, textLabel
and detailTextLabel
properties and the other way around.
内容配置也是如此。 这将覆盖你imageView
, textLabel
和detailTextLabel
性质和周围的其他方法。
All the mentioned properties that will be overriden both by background configurations or content configurations will be deprecated in future releases.
在后台版本或内容配置中将覆盖的所有上述属性将在以后的版本中弃用。
结语 (Wrapping up)
The purpose of this article was to show how we should change our mindset for using UITableViewCell in iOS 14. It seems that, by making this type of improvements to it, Apple is trying to send a message that UIKit will still be around for a while.
本文的目的是展示如何改变在iOS 14中使用UITableViewCell的思维方式。似乎,通过对此类型的改进,Apple试图发送一条消息,表明UIKit仍然存在一段时间。 。
接下来要去哪里: (Where to go next:)
For a more in depth presentation of UITableViewCell in iOS 14, check out the WWDC 2020 “Modern Cell Configuration” video: https://developer.apple.com/videos/play/wwdc2020/10027/
有关iOS 14中UITableViewCell的更深入的介绍,请查看WWDC 2020“现代单元配置”视频: https : //developer.apple.com/videos/play/wwdc2020/10027/
翻译自: https://medium.com/@dragos.rotaru9/uitableviewcell-in-ios-14-ef19e877319f