ios入门
In the first part of this series, we learned how to implement UIView
animation. In this part, we’ll see how to create an animation from an image’s sequence in iOS.
在本系列的第一部分中 ,我们学习了如何实现UIView
动画。 在这一部分中,我们将看到如何在iOS中根据图像序列创建动画。
We’ll begin with animating a loading-circle sequence’s images using only UIKit build-in functions. Next, we’ll see how to implement the Lottie library to make this same kind of animation (the singing bird) but much more elegantly and easily — while also providing for better performance. Finally, we’ll do a comparison of the various methods and tools that can produce this same kind of animation.
我们将从仅使用UIKit内置函数对加载循环序列的图像进行动画处理开始。 接下来,我们将看到如何实现Lottie库来制作相同类型的动画(唱歌的鸟),但更优雅,更轻松—同时还提供更好的性能。 最后,我们将比较可以产生这种动画的各种方法和工具。
So here’s the desired result we should have at the end of this tutorial:
因此,这是在本教程结尾处应有的预期结果:
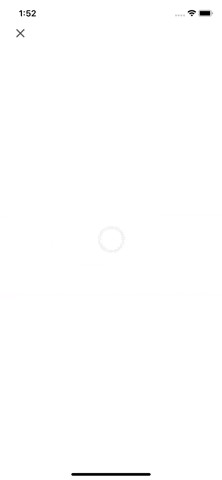
This tutorial is developing from the previous tutorial. You can download that project here. But you can follow along with this tutorial even if you’re starting from scratch with a project. Just keep in mind this story is on a separate view controller and has has a function, Go Back, enabling one to dismiss and go back to previous (first) view controller.
本教程是在上一教程的基础上开发的。 您可以在此处下载该项目。 但是,即使您从头开始创建一个项目,也可以按照本教程进行操作。 请记住,这个故事是在单独的视图控制器上,并且具有“返回”功能,可以使它退出并返回到上一个(第一个)视图控制器。
设置视图 (Set Up the View)
Now we have to set up the initial view components:
现在我们必须设置初始视图组件:
As you may have noticed, we inserted a UIImageView
into the center of the view and added a back button at the top left. Now we don’t have much to see here since we only have a back button to go back to the previous page. In viewDidAppear
, we call the animateSequenceImages
function, but right now it’s empty.
您可能已经注意到,我们将UIImageView
插入到视图的中心,并在左上方添加了后退按钮。 现在我们没有什么可看的了,因为我们只有一个返回按钮可以返回上一页。 在viewDidAppear
,我们调用animateSequenceImages
函数,但现在它是空的。
使用UIKit对图像序列进行动画处理 (Animating an Image Sequence Using UIKit)
Firstly, we’ll create the loading animation. So we should have an image’s sequence as assets provided to this animation. We need to create a reference folder of images, like this, in Xcode:
首先,我们将创建加载动画。 因此,我们应该将图像的序列作为提供给此动画的资产。 我们需要在Xcode中创建一个图像的参考文件夹,如下所示:
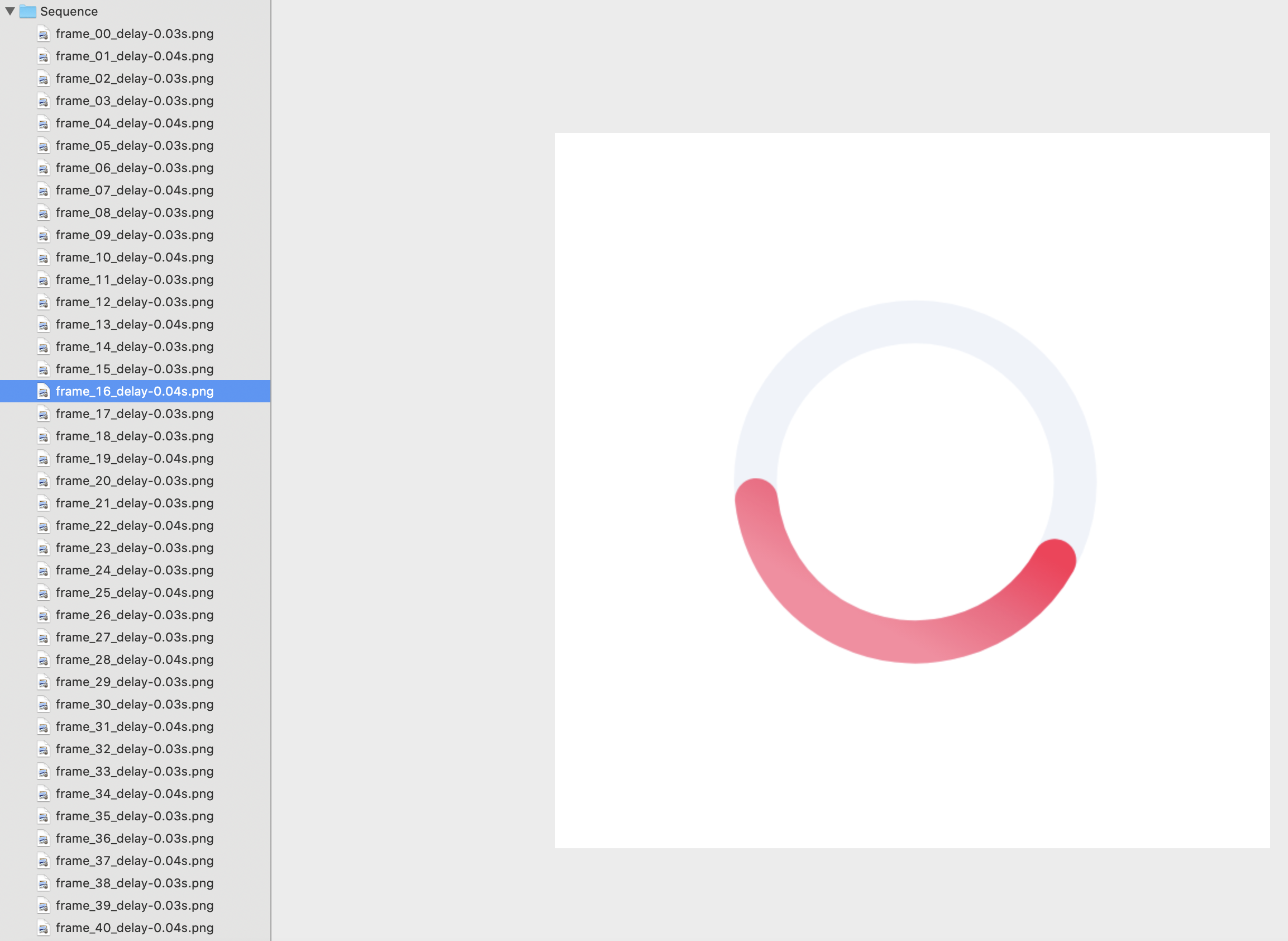
You can download the project via the link at the end of this story. Or you can download a GIF file you found on Google and split it into an image sequence. If you do so, I suggest using ezgif to split that GIF file.
您可以通过此故事末尾的链接下载项目。 或者,您可以下载在Google上找到的GIF文件,然后将其拆分为图像序列。 如果这样做,建议您使用ezgif拆分该GIF文件。
Cool! We had the assets — now animate it:
凉! 我们拥有资产-现在对其进行动画处理:
It’s pretty straightforward:
这很简单:
Feed the
UIImageView
with all of theUIImage
assets through theanimationImages
variable.通过
animationImages
变量向UIImageView
提供所有UIImage
资源。Assign the animation duration (
animationDuration = 5
).分配动画持续时间(
animationDuration = 5
)。We don’t repeat the animation (
animationRepeatCount = 0
).我们不重复动画(
animationRepeatCount = 0
)。Finally, start the animation (
sequenceImageView.startAnimating()
).最后,开始动画(
sequenceImageView.startAnimating()
)。
Actually, we’d like to fade out this loading view while it’s animating — that’s why we make the animating duration five seconds — but we fade it out after just two seconds.
实际上,我们希望在动画加载时淡出该加载视图-这就是为什么我们将动画持续时间设置为5秒钟的原因-但我们仅在两秒钟后将其淡出。
Here’s the result so far:
到目前为止的结果是:
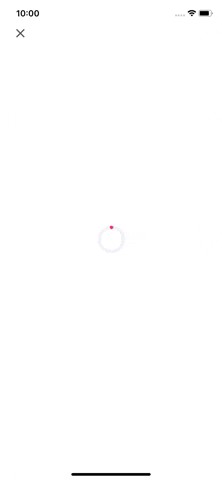
用洛蒂动画 (Animating With Lottie)
Lottie is an open-source framework originally created by Airbnb:
Lottie是最初由Airbnb创建的开源框架:
“Lottie is an iOS, Android, and React Native library that renders After Effects animations in real time, allowing apps to use animations as easily as they use static images.” — Lottie’s official site
“ Lottie是一个iOS,Android和React Native库,可实时渲染After Effects动画,使应用程序可以像使用静态图像一样轻松地使用动画。” — Lottie的官方网站
Firstly, we need to install lottie-ios
in our project. Lottie supports CocoaPods, Carthage, and Swift Package Manager. You can consult the details in their GitHub repo.
首先,我们需要在项目中安装lottie-ios
。 Lottie支持CocoaPods,Carthage和Swift Package Manager。 您可以在其GitHub存储库中查阅详细信息。
Secondly, we need to find the asset for Lottie. LottieFiles is a great resources to search for animating assets. Download any free JSON you found here, and name it bird.json
. Drag and drop this file into your current project. An animating JSON file for Lottie is like a PNG for a UIImageView
.
其次,我们需要找到洛蒂的资产。 LottieFiles是搜索动画资产的绝佳资源。 下载您在此处找到的所有免费JSON,并将其命名为bird.json
。 将此文件拖放到当前项目中。 Lottie的动画JSON文件就像UIImageView
的PNG。
Then, we set up the Lottie view (AnimationView
) in our view controller. Actually, using AnimationView
is very similar to an ordinary UIImageView
. We declare the variable:
然后,我们在视图控制器中设置Lottie视图( AnimationView
)。 实际上,使用AnimationView
与普通的UIImageView
非常相似。 我们声明变量:
private var giftView: AnimationView!
Now we can initialize this view in the setupUI()
function:
现在我们可以在setupUI()
函数中初始化此视图:
giftView = AnimationView(name: "bird")
giftView.frame = self.view.frame
giftView.contentMode = .scaleAspectFit
giftView.alpha = 0
giftView.loopMode = .autoReverse
view.addSubview(giftView)
AnimationView(name: “bird”)
is to tell the Lottie framework to look for the file bird.json
in the main bundle. We can notice that besides loopMode
, everything else is exactly similar to an UIImageView
. loopMode
tells us that the animation will be played forward and backward until stopped.
AnimationView(name: “bird”)
用于告诉Lottie框架在主捆绑包中查找文件bird.json
。 我们可以注意到,除了loopMode
之外,其他所有内容都与UIImageView
完全相似。 loopMode
告诉我们动画将向前和向后播放,直到停止。
Now we want to trigger the singing-bird animation when the loading fades out. We’ll call animateGift()
at the completion of animateSequenceImages()
in viewDidAppear
:
现在,我们要在加载消失时触发鸟鸣动画。 我们将在viewDidAppear
的animateSequenceImages()
完成时调用animateGift()
:
animateSequenceImages {
self.animateGift()
}
Inside animateGift()
, we fade in the AnimationView
:
在animateGift()
内部,我们在AnimationView
淡入淡出:
The play()
function starts the animation of AnimationView
. Here’s our final result:
play()
函数启动AnimationView的AnimationView
。 这是我们的最终结果:
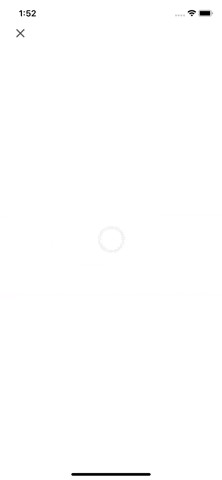
比较不同形式的动画 (Comparing Different Forms of Animation)
Actually, there’s many ways to embed a movielike animation like this into a view controller.
实际上,有很多方法可以将类似电影的动画嵌入到视图控制器中。
Besides Lottie and the image-sequence method we just did, we can also use a GIF, a video, or even code it using a Bézier path and/or a combination of UIView
’s animation functions. There are many reasons why designers and developers are starting to prefer Lottie animations over other formats. Let’s compare Lottie to the other options you have.
除了我们刚刚完成的Lottie和图像序列方法之外,我们还可以使用GIF,视频,甚至可以使用Bézier路径和/或UIView
动画功能的组合对其进行编码。 有许多原因使设计师和开发人员开始偏爱Lottie动画而不是其他格式。 让我们将Lottie与您拥有的其他选项进行比较。
PNG序列图像 (PNG-sequence image)
One of the biggest drawbacks of PNG-sequence graphics is they’re rather large in size, which can make them something of a hassle to export and manipulate on different interfaces for designers.
PNG序列图形的最大缺点之一是它们的尺寸很大,这使它们难以为设计者在不同的界面上导出和操作。
Lottie files, in comparison, are extremely small and conveniently sized, consequently having a quick download speed and keeping your site or app operations as smooth as possible.
相比之下,Lottie文件非常小,而且大小方便,因此下载速度很快,并且可以使您的网站或应用程序运行尽可能顺畅。
GIF (GIF)
While smaller than PNG graphics, GIFs are still a pretty space-occupying format, being in average double in size than a simple Lottie animation.
尽管比PNG图形小,但GIF仍然是相当占空间的格式,其大小平均比简单的Lottie动画大一倍。
GIFs are also typically scaled at a fixed size. This means you can’t modify them to better fit to scale on a different interface than where they were originally created. Lottie animations give you the space you need to adjust them to large-scale solutions as well as to smaller-scale screens.
GIF通常也按固定大小缩放。 这意味着您不能修改它们以使其更适合在与最初创建它们不同的界面上缩放。 Lottie动画为您提供了将它们调整为适用于大型解决方案以及较小尺寸屏幕的空间。
视频 (Video)
Regarding a simple and short animation, video content comes up quite often. For video content, particularly live-action stuff, you need to arrange for a host of resources, including props, actors, and locations. Animations are relatively simpler and cheaper alternatives that still get the job done.
关于简单而简短的动画,视频内容经常出现。 对于视频内容,特别是实况转播,您需要安排大量资源,包括道具,演员和位置。 动画是相对简单且便宜的替代方案,但仍然可以完成工作。
In term of coding, handling a video is much more of a hassle than an AnimationView
(which is as simple as a UIImageView
). Not to mention: A good animation keeps your visitor engaged and doesn’t compel them to turn it off.
在编码方面,处理视频比AnimationView
(就像UIImageView
一样简单)要麻烦得多。 更不用说:一个好的动画可以吸引您的访客,并不会迫使他们将其关闭。
代码动画 (Code animation)
App-development teams want to streamline the development process and make it as cost- or resource-effective as possible.
应用程序开发团队希望简化开发过程,并使其尽可能具有成本效益或资源效益。
Writing animation in code can be a time- and skill-intensive process, not to mention how easy it is to encounter a host of problems while writing the code. Instead of dedicating valued resources to either outsourcing the project or hiring skilled staff, why not use an alternative animation tool in the form of Lottie that is intuitive, scalable, and compatible with multiple platforms?
用代码编写动画可能是一个时间和技能密集的过程,更不用说在编写代码时遇到许多问题是多么容易了。 为什么不使用宝贵的资源来外包项目或雇用熟练的员工,为什么不使用直观,可扩展且兼容多个平台的Lottie形式的替代动画工具?
从这往哪儿走 (Where to Go From Here)
You can download the project here.
您可以在此处下载项目。
In this article, we learned how to create movielike animation by using PNG-sequence images and Lottie. We also discovered a great resources for Lottie animations: LottieFiles. And we saw why Lottie outperforms all of the other animation forms when it comes to short animations.
在本文中,我们学习了如何使用PNG序列图像和Lottie创建类似电影的动画。 我们还发现了很多有关Lottie动画的资源: LottieFiles 。 我们了解了为什么在短动画方面,Lottie的表现优于所有其他动画形式。
We finished the second part of the series. In the next part, we’ll see how to animate the transition between view controllers. Here’s the final result of our three-part series:
我们完成了该系列的第二部分。 在下一部分中,我们将看到如何为视图控制器之间的过渡设置动画。 这是我们的三部分系列的最终结果:
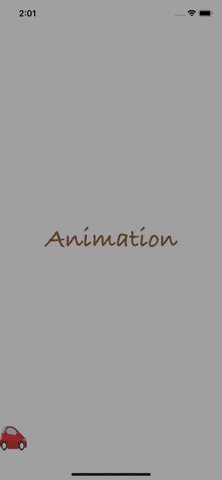
翻译自: https://medium.com/better-programming/getting-start-with-ios-animation-part-2-65f5bcd0c087
ios入门