swift 导入
Postman is a simple tool to create and fire API requests. In this article, I will be sharing how we can capture Swift URLRequests fired from our iOS application and import them into Postman. This technique will be helpful to share requests among teammates during development. As a bonus, I will also showcase how to intercept Firebase HTTP requests.
Postman是创建和触发API请求的简单工具。 在本文中,我将分享如何捕获从我们的iOS应用程序触发的Swift URLRequest并将其导入Postman。 在开发过程中,此技术将有助于在队友之间共享请求。 另外,我还将展示如何拦截Firebase HTTP请求。
In your Podfile
, add pod 'URLRequest-cURL'
.
在您的Podfile
,添加Podfile
pod 'URLRequest-cURL'
。
This is a cocoapod library that attempts to re-engineer the components that were used to construct the URLRequest
object.
这是一个cocoapod库,它试图重新设计用于构造URLRequest
对象的组件。
The usage is pretty simple. Call the getter variable cURL
(applied through extension) to get the formatted cURL
command in String
:
用法很简单。 调用getter变量cURL
(通过扩展名应用)以在String
获取格式化的cURL
命令:
// let request = URLRequest()
print(request.cURL)
Here’s an example of what the String
value may look like. Don’t be surprised to see additional headers attached to your requests. Try printing the cURL command onto the Xcode console:
这是String
值可能看起来的示例。 看到附加到您的请求的标头不要感到惊讶。 尝试将cURL命令打印到Xcode控制台上:
curl -X POST 'https://www.anonymousapi.com/checksPassword?apikey=XXYYZZ' -H 'Accept-Language: en' -H 'Content-Length: 91' -H 'Content-Type: application/json' -d '{
"email" : "alpha2@gmail.com",
"returnSecureToken" : true,
"password" : "password"
}'
You can import the cURL
Command into Postman by importing it as raw text:
您可以通过将cURL
命令作为原始文本导入到Postman中:
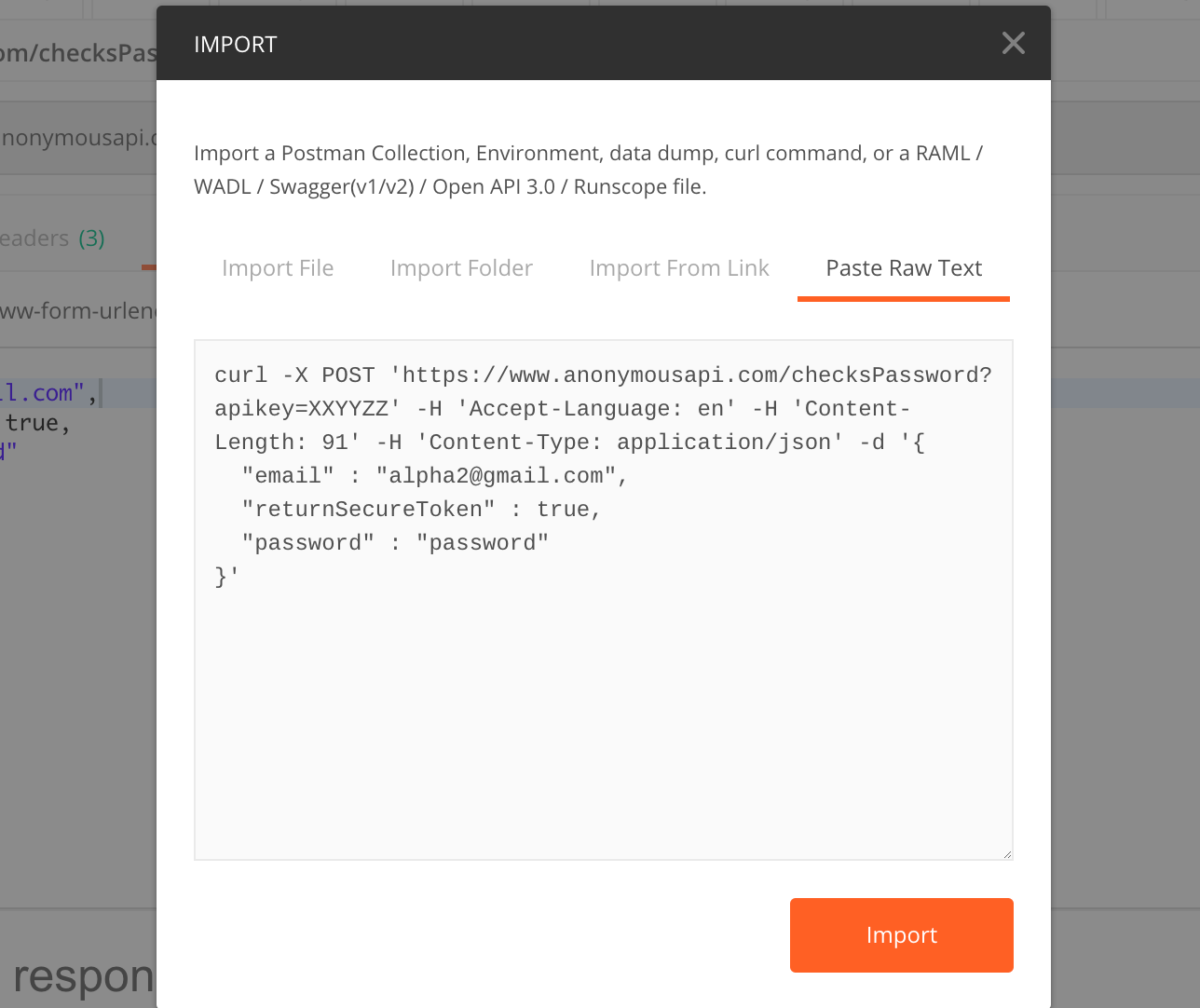
After importing, you can view all the header and payload-body values in Postman. This becomes useful for developers trying to debug API issues.
导入后,您可以在Postman中查看所有标头和有效载荷主体值。 这对于尝试调试API问题的开发人员来说很有用。
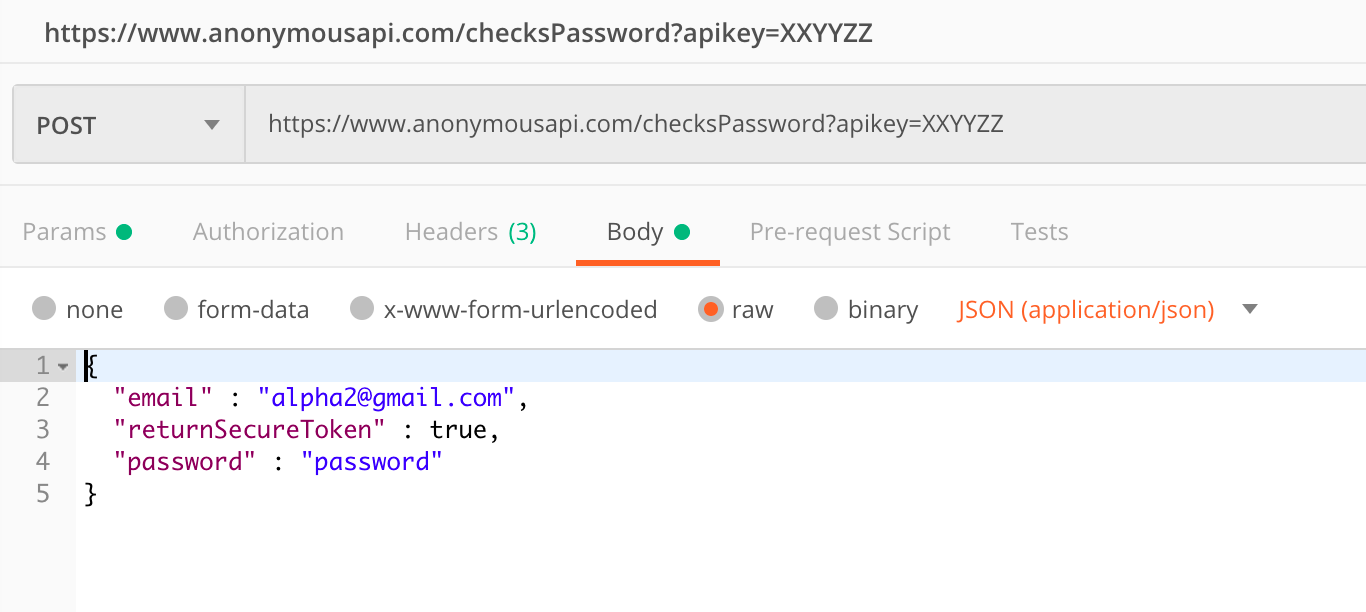
However, the scenarios above only work if we are able to get the instance of URLRequest
in our source codes. For projects that rely on API requests fired by third-party libraries, we will need another library to intercept requests at the URProtocol
level.
但是,仅当我们能够在源代码中获取URLRequest
实例时,以上方案才有效。 对于依赖第三方库触发的API请求的项目,我们将需要另一个库来拦截URProtocol
级别的请求。
Podfile:pod NetworkInterceptor (Podfile: pod NetworkInterceptor)
This library uses the URLRequest-cURL
pod as a dependency library. NetworkInterceptor creates and prioritizes a custom URLProtocol with the intention to intercept and inspect outgoing requests made by the iOS application. All you have to do is to register a RequestSniffer
object and initiate the setup:
该库使用URLRequest-cURL
pod作为依赖库。 NetworkInterceptor创建自定义URLProtocol并对其进行优先排序,以拦截和检查iOS应用程序发出的外发请求。 您要做的就是注册一个RequestSniffer
对象并启动设置:
让我们尝试一下 (Let’s try it out)
In this example, we will use Firebase Authentication to register new users in our app.
在此示例中 ,我们将使用Firebase身份验证在我们的应用程序中注册新用户。
1.设置NetworkInterceptor (1. Set up NetworkInterceptor)
Use the following pods in the Podfile:
在Podfile中使用以下Pod:
pod 'NetworkInterceptor'
pod 'NetworkInterceptor'
pod 'Firebase/Auth'
pod 'Firebase/Auth'
2.设置NetworkInterceptor (2. Set up NetworkInterceptor)
Set up the NetworkInterceptor
codes as we did above.
像上面一样设置NetworkInterceptor
代码。
3.使用这些凭据使用FirebaseAuth创建新用户 (3. Use these credentials to create a new user using FirebaseAuth)
email:
abcstrawberry@gmail.com
电子邮件:
abcstrawberry@gmail.com
password:
password1
密码:
password1
import FirebaseAuthAuth.auth().createUser(withEmail: email, password: password) { [weak self] (authResult, error) in //implement post registration logic
}
4.检查控制台中的cURL命令 (4. Check console for cURL command)
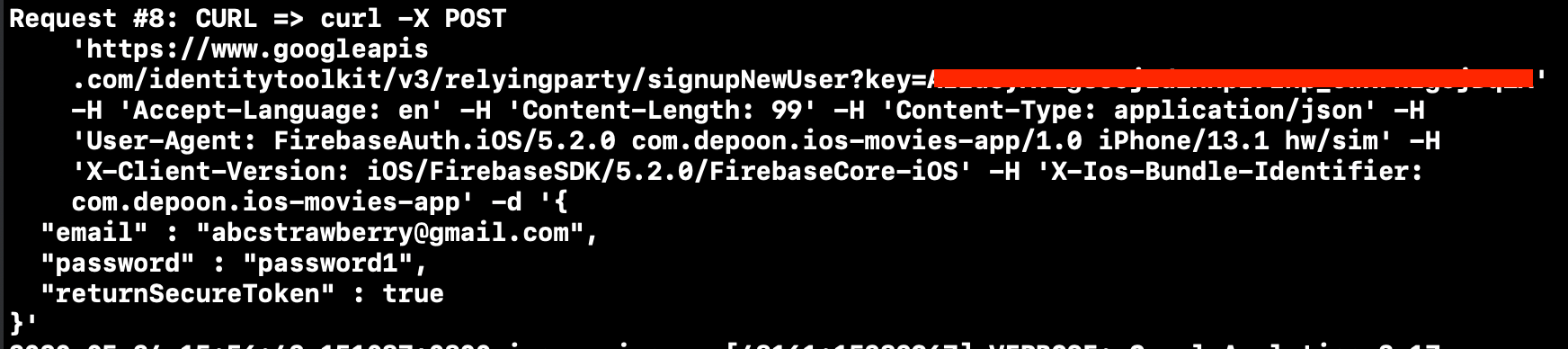
The cURL
command for the create user
API request appears in the Xcode console. You can simply copy it and import it into Postman. Note that the API checks the payload against the Content-Length
header value. You will need to modify the Content-Length
value if you are using different email/password values.
用于create user
API请求的cURL
命令出现在Xcode控制台中。 您可以简单地将其复制并导入到Postman中。 请注意,API会根据Content-Length
标头值检查有效负载。 如果您使用其他电子邮件/密码值,则需要修改Content-Length
值。
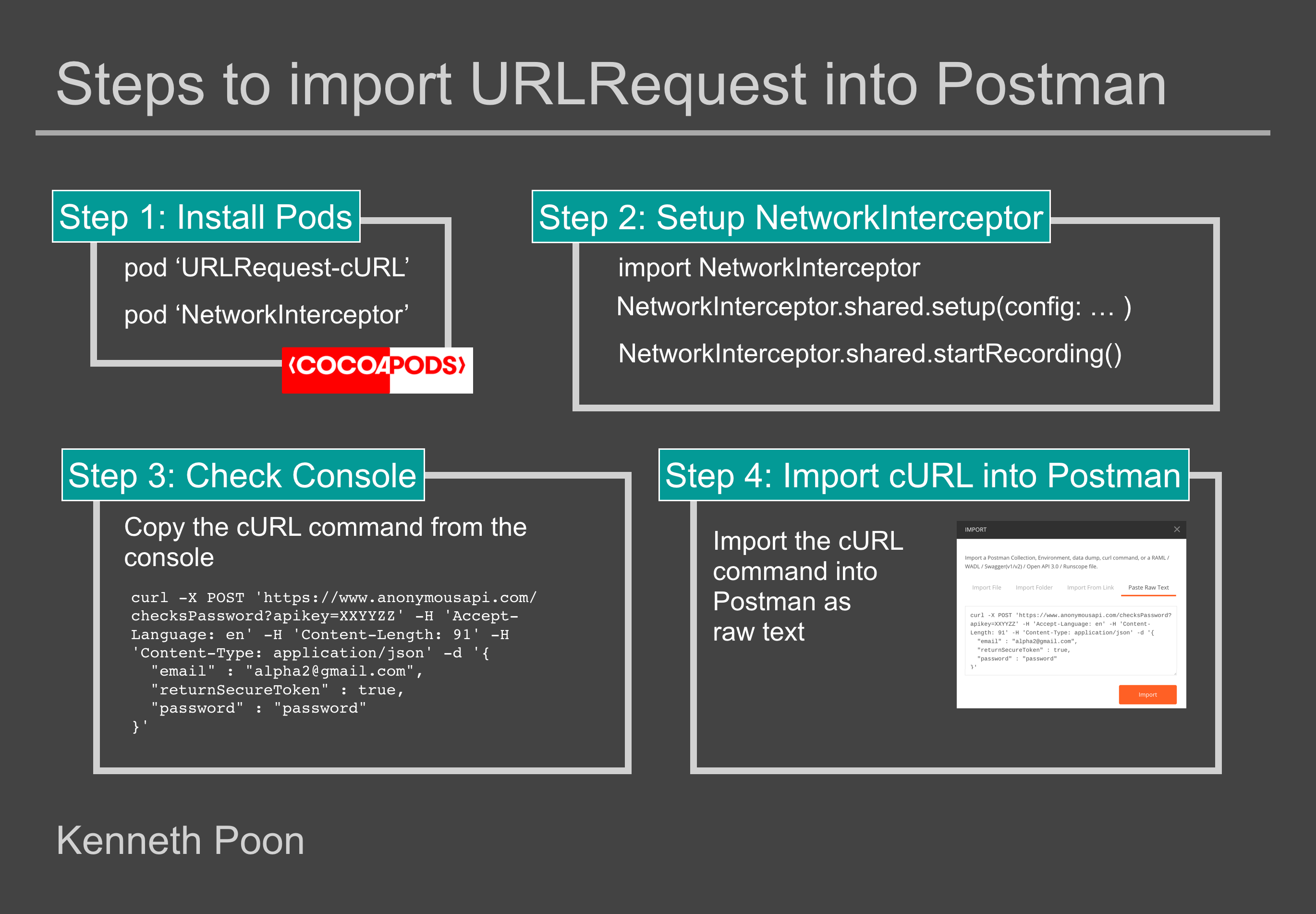
我以前在哪里可以看到此NetworkInterceptor库? (Where Have I Seen This NetworkInterceptor Library Before?)
NetworkInterceptor was featured in one of my previous articles.
我以前的一篇文章中介绍了NetworkInterceptor。
It was created for the purpose of intercepting SSL-pinned HTTPS requests. However, I soon discovered it to be a very useful tool to debug API-related issues. In fact, many of my teammates and a handful of my readers said that it helped them a lot during development.
创建它是为了拦截SSL固定的HTTPS请求。 但是,我很快发现它是调试与API相关的问题的非常有用的工具。 实际上,我的许多队友和少数读者说,这在开发过程中对他们有很大帮助。
有什么我可以帮助改进的吗? (Is There Anything I Can Help to Improve Upon?)
Yes. One of my current issues is generating the cURL
command for Firebase Analytics event log requests. Knowing the exact cURL
of Firebase Analytics would allow me to build a mock server for XCUITest. Feel free to create a pull request on GitHub to help me out.
是。 我当前的问题之一是为Firebase Analytics事件日志请求生成cURL
命令。 知道Firebase Analytics的确切cURL
可以让我为XCUITest构建一个模拟服务器。 随时在GitHub上创建拉取请求以帮助我。
结论 (Conclusion)
This article was inspired by my good friend Rizwan Ahmed of Oh my Swift. In his piece, he showcased how anyone can create example URLSession Swift codes using Postman. Both his article and mine show different ways of using Postman in iOS development.
这篇文章的灵感来自我的好朋友Rizwan Ahmed,来自《 我的雨燕》 。 在他的文章中 ,他展示了任何人如何使用Postman创建示例URLSession Swift代码。 他的文章和我的文章都展示了在iOS开发中使用Postman的不同方式。
Feel free to drop NetworkInterceptor
and URLRequest-cURL
into your iOS Xcode projects and let me know if this is helpful. Thanks for reading.
随时将NetworkInterceptor
和URLRequest-cURL
放入您的iOS Xcode项目中,并告诉我这是否有帮助。 谢谢阅读。
翻译自: https://medium.com/better-programming/how-to-import-swift-urlrequests-into-postman-ef6c65c20834
swift 导入