react mvvm
总览 (Overview)
Apple’s introduction of SwiftUI, alongside the rising popularity of Flutter, Jetpack Compose, and React/React Native(particularly with hooks), is an indicator of the future of front-end development: a functional and declarative style of programming supplanting the traditional stateful and object-oriented one.
苹果公司推出的SwiftUI ,以及Flutter , Jetpack Compose和React / React Native (尤其是带有钩子 )的日益流行, 标志着前端开发的未来:一种功能性和 声明性的编程风格 ,取代了传统的有状态和面向对象的 。
The guiding principle behind these frameworks is relatively simple and elegant: all views can be modeled as a function of some state.
这些框架背后的指导原则相对简单而优雅: 可以将所有视图建模为某个状态的函数 。
Take, for example, React: all (class-based) React components must implement a render()
function that describes what the component should look like. A component can also maintain some notion of state - for example, a button might keep track of how many times it's been clicked so it can display that number to the user. Whenever the state of a component changes, the magic of React takes over and the component is re-rendered—the render()
function is automatically called again and the component is visually updated (the return value of a render()
can depend on state). This is precisely the reason for the framework's name: views react to changes in state. 🤯
以React为例,所有(基于类的)React组件都必须实现一个render()
函数,该函数描述该组件的外观。 组件还可以保持某种状态概念-例如,按钮可以跟踪其被单击的次数,以便可以向用户显示该数字。 每当组件的状态发生变化时,React的魔力就会接管并重新渲染组件-会自动再次调用render()
函数,并以可视方式更新组件( render()
的返回值取决于状态) )。 这正是使用框架名称的原因: 视图对state的变化做出React 。 🤯
In iOS development, this behavior is traditionally implemented using a paradigm known as functional reactive programming. Libraries such as ReactiveSwift and RxSwift define a standard interface for events that views may want to react to, such as button taps, incoming network data, or a change in the user’s location. They rely heavily on the Observer pattern, in which views and/or view controllers observe/subscribe to observables and are “notified” appropriately whenever some event of interest occurs. The following pseudocode describes this process:
在iOS开发中,通常使用称为功能React式编程的范例来实现此行为。 诸如ReactiveSwift和RxSwift之类的库为视图可能要响应的事件定义了标准接口,例如按钮点击,传入的网络数据或用户位置的更改。 它们严重依赖于观察者模式 ,在该模式中,视图和/或视图控制器观察 / 订阅 可观察对象,并在发生某些感兴趣的事件时适当地“通知”它们。 以下伪代码描述了此过程:
class Observable<T> {
// Array of callbacks with a single generic parameter
private let subscribeBlocks = Array<T -> Void>()
func subscribe(block: T -> Void) {
self.subscribeBlocks.append(block)
}
/// Notify all observers
func notify(with event: T) {
for block in subscribeBlocks {
block(T) // Execute the callback
}
}
}// Suppose we have an observable bound to a UISwitch and we are
// broadcasting the event the user switches it on or off.
let switchObservable = Observable<Bool>()// Subscribing looks like…
switchObservable.subscribe { isOn in
if isOn {
updateViewSomeWay()
} else {
updateViewSomeOtherWay()
}
}// Notifying looks like…
switchObservable.notify(true)
Again, once you think about how many kinds of UI events there are (especially the fact that they are almost always generated asynchronously), you can begin to understand why the reactive paradigm is so powerful for front-end development. Over many years, the combination of reactive programming with other architecture and design patterns led to the birth of the MVVM Architectural Pattern. Though my experience is limited (I’ve only worked at 3 different companies), I claim that MVVM is the most widely-used and accepted architecture in industry right now for iOS development.
同样,一旦您考虑了有多少种UI事件(尤其是它们几乎总是异步生成的事实),您就可以开始理解为什么响应式范例对于前端开发如此强大。 多年以来,React式编程与其他架构和设计模式的结合导致了MVVM Architecture Pattern的诞生。 尽管我的经验有限(我只在3家不同的公司工作过),但我声称MVVM是目前iOS开发中业界使用最广泛和接受程度最高的体系结构。
MVVM stands for Model-View-ViewModel. I won’t bore you with the details, but the critical idea of MVVM is that every View Controller owns a View Model that is responsible for handling all business logic. The View Model generates events that the View Controller subscribes to, resulting in an effective decoupling of logic.
MVVM代表Model-View-ViewModel 。 我不会给您带来任何细节,但是MVVM的关键思想是每个View Controller都拥有一个负责处理所有业务逻辑的View Model。 视图模型生成视图控制器订阅的事件,从而导致逻辑的有效解耦。
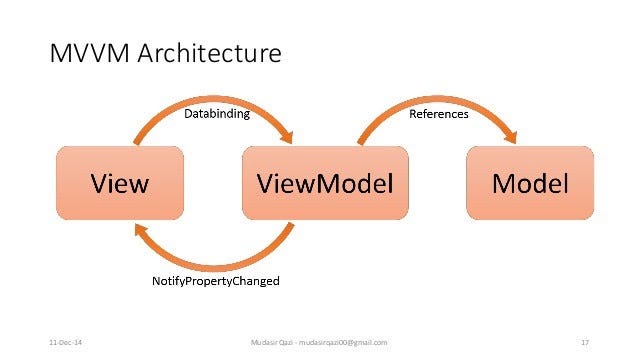
输入SwiftUI (Enter SwiftUI)
During WWDC 2019, Apple announced the development of a framework introducing a completely new way of building iOS apps: SwiftUI. It leverages many powerful features of Swift such as protocols, function builders, and implicit returns to facilitate a declarative style of building user interfaces, similar to how HTML looks. In many ways, it seems to mimic React; here’s a fun diagram I made loosely mapping React concepts to their SwiftUI counterparts:
在WWDC 2019期间,Apple宣布开发了一个框架,该框架引入了一种全新的构建iOS应用程序的方式: SwiftUI 。 它利用了Swift的许多强大功能,例如协议,函数构建器和隐式返回,以促进声明性的样式来构建用户界面,类似于HTML的外观。 在许多方面,它似乎模仿了React。 这是我制作的一个有趣的图,将React概念大致映射到其SwiftUI对应对象:

SwiftUI is a game-changer for iOS development in many ways, ditching stateful, object-oriented programming in favor of a declarative, functional, and reactive one. In addition, Apple has developed their own reactive framework called Combine that integrates seamlessly with SwiftUI.
SwiftUI在许多方面都改变了iOS开发的规则, 放弃了有状态的,面向对象的程序 ,而采用了声明性,功能性和React性程序 。 此外,苹果公司开发了自己的名为Combine的React框架,该框架与SwiftUI无缝集成。
To demonstrate, here’s how you’d properly add a “Hello, world!” label to the center of a view using the current iOS SDK (programmatically without constraints, because storyboards and Auto-layout are evil) vs. SwiftUI:
为了演示,这是您正确添加“ Hello,world!”的方法 使用当前iOS SDK(以编程方式没有限制,因为情节提要和自动布局很糟糕)将标签标注到视图的中心,而不是SwiftUI:
当前的iOS SDK (Current iOS SDK)
import UIKitclass HelloWorldViewController: UIViewController {
let label = UILabel()
override func viewDidLoad() {
super.viewDidLoad()
label.text = “Hello, world!”
view.addSubview(label)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
var labelFrame = CGRect.zero
labelFrame.size = label.intrinsicContentSize
labelFrame.origin.x = (view.bounds.width — labelFrame.width) / 2
labelFrame.origin.y = (view.bounds.height — labelFrame.height) / 2
label.frame = labelFrame
}
}
SwiftUI (SwiftUI)
import SwiftUIstruct HelloWorldView: View {
var body: some View {
Text(“Hello, world!”)
}
}
Of course, this is just one particular example where SwiftUI is clearly superior to the old way of doing things; I’ll discuss its shortcomings as I see them later. But you can easily see why it’s such a powerful framework, and I can even prove syllogistically that it’s better than React:
当然,这只是SwiftUI明显优于旧的做事方式的一个示例。 我将在稍后讨论它们的缺点。 但是您可以轻松地了解为什么它是如此强大的框架,而且我什至可以通过语言学证明它比React更好:
- React is a JavaScript framework. React是一个JavaScript框架。
- SwiftUI is not a JavaScript framework. SwiftUI不是JavaScript框架。
- SwiftUI is superior to React. SwiftUI优于React。
JavaScript bashing aside, I wanted an opportunity to compare both styles of programming side-by-side, so I set out to build…
除了JavaScript扑朔迷离之外,我希望有机会并排比较两种编程风格,因此我着手构建……
A cat fact generator app.
猫事实发生器应用程序。
RxCatFact (RxCatFact)
I stumbled upon the fun cat fact API at https://catfact.ninja a while ago, but never had the opportunity to build a toy app around it. I started by whipping up a quick Figma prototype:
不久前,我在https://catfact.ninja偶然发现了有趣的猫事实API,但从未有机会围绕它构建玩具应用程序。 我首先制作了一个快速的Figma原型:

Here are the key features to implement:
以下是要实现的关键功能:
Tapping the “Generate!” Button causes:
轻按“生成!” 按钮原因:
- The background to fade into another random gorgeous gradient. 背景逐渐淡入另一个随机的华丽渐变中。
- The cat emoji to change to another random cat emoji. 猫表情符号更改为另一个随机猫表情符号。
- A call to the cat fact API, with the fetched fact displayed in the center box. While we wait for the network call to complete, the contents of the box are replaced with a loading indicator. 调用cat fact API,并在中心框中显示获取的事实。 在我们等待网络呼叫完成的同时,包装箱中的物品被装载指示器代替。
The back button in the bottom left goes to the previous fact.
左下角的后退按钮转到上一个事实。
- It should be disabled when the app starts, and when there are no more facts to go back to. 当应用启动时,并且没有其他事实可回溯时,应禁用它。
The share button in the bottom right opens a share sheet that allows the user to share the currently displayed fact.
右下方的共享按钮将打开一个共享表,该共享表允许用户共享当前显示的事实。
- It should be disabled when the app starts. 应用启动时应将其禁用。
The middle box will be empty when the app starts.
应用启动时,中间的框将为空。
You can check out the project’s repo here.
您可以在此处查看项目的仓库。
I implemented the app twice — once in the “old way” using MVVM and the framework RxSwift, and once in SwiftUI. If you actually build the app and view it on a simulator/device, you’ll see I embedded each inside a TabView. You can swap back and forth between the two. Here’s a screenshot from the completed app:
我实施了两次应用程序- 一次使用MVVM和框架RxSwift以“旧方式”实现,一次在SwiftUI中实现 。 如果您实际构建该应用程序并在模拟器/设备上查看它,您会看到我将它们嵌入TabView中。 您可以在两者之间来回交换。 这是完成的应用程序的屏幕截图:

Feel free to explore the repo to see both implementations; I tried to mirror them as much as possible. You can find all of the relevant MVVM files in the “RxSwift” folder and the SwiftUI files in the “SwiftUI” folder. Rather than explain all of the code in unnecessary detail, I thought I’d finish this README by discussing what I think are the real benefits and drawbacks of SwiftUI in its current state.
随意浏览回购以查看两个实现; 我试图尽可能地反映它们。 您可以在“ RxSwift ”文件夹中找到所有相关的MVVM文件,并在“ SwiftUI ”文件夹中找到SwiftUI文件。 我认为不必结束所有自述代码,而是通过讨论我认为SwiftUI当前状态的真正优点和缺点来完成本自述文件。
结论:烦人与好 (Conclusion: Annoying vs Good)
I kept a list of things I found both annoying and good while I worked on this, and rather than list each point in detail I’ve grouped them to form overarching themes and concepts I’d like to discuss. I’ll start with the annoying bits so we can end on a good note. 😄😄
我整理了一份工作过程中发现的烦人和好事情的清单,而不是详细列出每个要点,而是将它们分组以形成我要讨论的总体主题和概念。 我将从烦人的内容开始,以便我们以一个良好的音调结束。 😄😄
烦人的 (Annoying)
1. SwiftUI还太年轻。 (1. SwiftUI is still too young.)
Any new framework is bound to have its share of kinks and flaws, and SwiftUI is not exempt. While building this app, I ran into many minor inconveniences, the kind that give rise to thoughts like “Why?” and “ew Gross” (with a capital G) when you eventually fix them. They include:
任何新框架都必将有其类似之处和缺陷,SwiftUI也不例外。 在构建此应用程序时,我遇到了许多小麻烦,这种麻烦引起了诸如“ 为什么? ”和“ ew Gross ”(大写G)(最终修复它们时)。 它们包括:
- Modifier order matters. 修饰符顺序很重要。
- Absolute positioning can be difficult. 绝对定位可能很困难。
- You can’t declare constants in Function Builders. 您不能在Function Builders中声明常量。
The
EnvironmentObject
check happens at run-time and can crash your app.EnvironmentObject
检查在运行时发生,并且可能使您的应用程序崩溃。- Transition animations only work on View add/removal. 过渡动画仅适用于View添加/删除。
These kind of problems introduce two main roadblocks to a good development experience: unnecessary technicalities, and ugly workarounds. I’ll elaborate on two of the above points just so you can see what I mean.
这些问题为良好的开发体验引入了两个主要障碍: 不必要的技术和丑陋的解决方法 。 我将详细说明以上两点,以便您了解我的意思。
不必要的技术性:修饰符顺序很重要。 (An unnecessary technicality: Modifier order matters.)
One of the most notable ways SwiftUI exhibits its functional style is through the way you modify a view’s properties. For example, consider the following code snippet:
SwiftUI展示其功能样式的最著名方式之一是通过修改视图属性的方式。 例如,考虑以下代码片段:
// SwiftUI
Rectangle()
.foregroundColor(.blue)
.cornerRadius(10)
.opacity(0.5)// UIKit
let view = UIView()
view.backgroundColor = .blue
view.layer.cornerRadius = 10
view.alpha = 0.5
Each modifier function returns a new instance of that View with the modification applied (and since Views are value-type structs, this isn’t terribly inefficient). However, absurdly, the order in which you apply modifiers can affect the state of the finalized view. Again, consider the following code snippet:
每个修改器函数都返回一个应用了修改的View的新实例(并且由于Views是值类型的结构,因此效率不是很高)。 但是,荒谬的是, 应用修饰符的顺序会影响最终视图的状态 。 同样,请考虑以下代码片段:
VStack {
Button(“Hello, world!”) { }
.frame(width: 100, height: 100)
.background(Color.red) Button(“Hello, world!”) { }
.background(Color.red)
.frame(width: 100, height: 100)
}
The buttons merely have the modifier order swapped. So what do you think this will look like? Given the title of this grievance you’ll probably guess that they’ll look different, and you would be correct! Here’s what you’ll get:
这些按钮仅交换了修改器顺序。 那么您认为这会是什么样子? 给定这个委屈的标题,您可能会猜测它们的外观会有所不同,您是正确的 ! 这是您将得到的:

Technicalities like this can really make any framework or language annoying to work with (think of the difference between the double equals and triple equals in JavaScript) because you need to remember them! Modifier order really should not matter in order to make the SwiftUI development experience as simple, clean, and honestly sensible, as possible. Hopefully edge cases like these are fixed in the future.
像这样的技术真的会使任何框架或语言都变得令人讨厌 (想想JavaScript中的double equals和Triple equals之间的区别),因为您需要记住它们! 修改顺序实际上并不重要,以使SwiftUI开发体验尽可能简单,干净和诚实。 希望将来会修复此类边缘情况。
丑陋的解决方法:过渡动画仅在视图添加/删除上起作用。 (An ugly workaround: Transition animations only work on view add/removal.)
SwiftUI has greatly improved the ease of adding animations to views. In the following snippet, the excerpts of code do roughly the same thing:
SwiftUI大大提高了向视图添加动画的便利性。 在以下代码段中,代码摘录执行的操作大致相同:
// SwiftUI
Rectangle()
.scaleEffect(isLarge ? 1.5 : 1)
.animation(.easeInOut)// UIKit
let animations: () -> Void
if isLarge {
animations = {
viewToAnimate.transform = CGAffineTransform(scaleX: 1.5, y: 1.5)
}
} else {
animations = {
viewToAnimate.transform = .identity
}
}UIView.animate(
duration: 0.5,
delay: 0.0,
options: .curveEaseInOut,
animations: animations,
completion: nil
)
Pretty neat right? However, there is another subclass of animations called transitions. These occur when you want to animate a view from one state to another; for example, suppose you want to fade the background color of a view to red. In UIKit, this might look like:
很整洁吧? 但是,还有另一个动画子类,称为transitions 。 当您想要将视图从一种状态动画化为另一种状态时,就会发生这种情况。 例如,假设您想将视图的背景色淡化为红色。 在UIKit中,这可能类似于:
UIView.transition(
with: viewToTransition,
duration: 0.5,
options: [.curveEaseInOut, .transitionCrossDissolve],
animations: {
viewToTransition.backgroundColor = .red
},
completion: nil
)
You can achieve the same effect in SwiftUI using the transition(.opacity)
modifier on a View. However, the fading animation only ever occurs if the View is being added or removed from the View hierarchy. In other words, without any workaround, you cannot use the transition
modifier to fade a view's background color from one to another because the final state of the transition does not involve the View getting removed from the screen.
您可以在View上使用transition(.opacity)
修饰符在SwiftUI中实现相同的效果。 但是,仅当在View层次结构中添加或删除View时,才会发生淡入淡出动画。 换句话说,如果没有任何解决方法,则不能使用transition
修饰符将视图的背景颜色从一种淡入另一种, 因为过渡的最终状态不涉及将视图从屏幕上移开 。
This was an issue for me because I wanted to both fade the background between different gradients and the cat facts from one to another. After a bit of StackOverflow googling I found a workaround — to convince SwiftUI that the View being animated to was a completely different one. You can see this in CatFactView.swift
:
这对我来说是个问题,因为我既想淡化不同渐变之间的背景,又要使猫咪之间的猫咪事实淡化。 经过一番StackOverflow的搜索之后,我找到了一种解决方法 -让SwiftUI确信要动画化的View是完全不同的视图。 您可以在CatFactView.swift
看到这CatFactView.swift
:
GradientBackground(colors: outputs.backgroundColors)
.edgesIgnoringSafeArea(.top)
.transition(.opacity)
.id(outputs.backgroundColors.hashValue)
The key is the id
modifier. By assigning the view a new and unique ID value based on the hash of the background colors, SwiftUI is convinced that the same view is actually a different view and the fade animation works perfectly. But you can't help that queasy feeling in your stomach generated by your body's instinctual disgust. Let me put it simply: this is gross.
关键是id
修饰符。 通过基于背景颜色的哈希值为视图分配新的唯一ID值,SwiftUI确信同一视图实际上是不同的视图,并且淡入淡出动画效果完美。 但是,您忍不住由于身体本能的厌恶而在胃部产生的那种不适感。 让我简单地说: 这很糟糕。
Until SwiftUI matures, workarounds like these will be needed to achieve seemingly trivial behaviors. They detract from the beautiful and concise code aesthetic that SwiftUI strives to promote. Again, hopefully new APIs and features will fix this in the future.
在SwiftUI成熟之前,将需要采取类似的变通方法来实现看似微不足道的行为。 它们损害了SwiftUI努力推广的美观简洁的代码美感。 同样,希望新的API和功能将来能够解决此问题。
2. SwiftUI抽象了许多 iOS开发。 (2. SwiftUI abstracts a lot of iOS development away.)
This second and final annoying point comes from my perspective as someone that has helped teach iOS development to new computer science students for four years. As much as I hate storyboards and Auto-layout, I always start my lessons there because it’s simple, easy to use, intuitive, and allows you to get something working in a matter of minutes. But at least with storyboards, there is a foundation upon which you can segue (ha ha) into fundamental iOS concepts such as the View Controller lifecycle, the view hierarchy, the protocol-delegate pattern, etc.
从我的角度来看,第二个也是最后一个令人烦恼的观点是,四年来曾帮助新计算机科学专业的学生教授iOS开发的人。 虽然我讨厌故事板和自动布局,我总是开始我的教训有,因为它的简单,易用,直观,可以让你得到的东西在短短的几分钟内工作。 但是至少有了情节提要,您才能在此基础上了解( 基本)iOS基本概念,例如View Controller生命周期,视图层次结构,协议委托模式等。
After this experiment, I think I can say that SwiftUI outclasses even storyboards with regards to the ease and speed in which you can build an app. Furthermore, the stickler inside me is really happy that it’s pure programming rather than visual construction. However, the functional and declarative style of SwiftUI is so far from the frameworks and processes underlying UIKit and iOS apps in general (which are not going anywhere for a long time) that I worry for beginners.
经过这个实验,我想我可以说SwiftUI在构建应用程序的便捷性和速度方面甚至超越了故事板。 此外,我内心的粘滞者真的很高兴,因为它纯粹是编程而不是可视化的构造。 但是,SwiftUI的功能和声明式风格与UIKit和iOS应用程序的基础框架和流程(到目前为止已经很久没有使用)相去甚远了,我为初学者担心 。
I firmly believe that the fewer black boxes you can have while teaching coding, the better. I’m all too familiar with the slight frustration that appears on my students’ faces when I tell them, “Just trust me, it’ll work.” Of course, some black boxes are necessary — taken to an extreme, it would obviously be ridiculous to start a course on iOS development with understanding how operating systems work. But there are so many powerful features that SwiftUI relies on: function builders, protocols, UIKit interoperability, value-type semantics, that require a strong understanding of Swift and the way iOS apps already work to fully understand.
我坚信,教授编码的黑匣子越少越好。 当我告诉学生:“只要相信我,它就会起作用。”我对学生脸上的沮丧感到非常熟悉。 当然,有些黑匣子是必要的-太极端了,很明显荒谬的是,开始了解iOS的工作方式的iOS开发课程。 但是SwiftUI依赖许多强大的功能:函数生成器,协议,UIKit互操作性,值类型语义,这些都需要对Swift以及OS应用程序已经充分理解的方式有深入的了解 。
My biggest worry with SwiftUI is that it’s so easy that it provides a poor starting point for someone that really wants to get into iOS development long-term. I will end with the same lame concluding sentence that I have used in the previous section: hopefully, this changes in the future.
我对SwiftUI的最大担心是,它是如此简单 ,以至于它为真正想长期从事iOS开发的人提供了一个糟糕的起点。 我将以与上一节相同的la脚结论作为结尾:希望将来会有所改变。
好 (Good)
1.易于扩展并与UIKit互操作。 (1. Easy to extend and interoperate with UIKit.)
Onto the good parts! This first one is fairly short and sweet: it’s pretty easy to create adapters for UIKit components that you can add to your SwiftUI views. This is primarily achieved through the UIViewRepresentable
and UIViewControllerRepresentable
protocols. You can take a look at ActivityIndicator.swift
and ActivityViewController.swift
in the repo to see how this works. While Apple continues to work on porting UIKit views to SwiftUI, these protocols serve as a pretty good alternative in the meantime.
好的部分! 第一个很简短,也很贴切:为UIKit组件创建适配器很容易,您可以将其添加到SwiftUI视图中。 这主要是通过UIViewRepresentable
和UIViewControllerRepresentable
协议实现的。 您可以在回购中查看ActivityIndicator.swift
和ActivityViewController.swift
,以了解其工作原理。 尽管Apple继续致力于将UIKit视图移植到SwiftUI,但这些协议在此期间是一个很好的选择。
2.轻松促进强大的体系结构和组织良好的代码库。 (2. Easily facilitates a powerful architecture and well-organized codebase.)
One of the most notorious questions for any iOS developer is: “What architecture should I use?” Apple’s answer to that is MVC, which stands for Model-View-Controller. The idea is to separate raw data (models) from the actual user-facing views. The two interact through the controller object, which is responsible for reading and updating models and telling views what to display. The problem that arises from this architecture is also called MVC, or Massive View Controller. Since the view controllers have so much work to handle, you end up shoving all of your logic (business, data formatting, view updating) inside them and end up with these files that are thousands of lines long. Not very scalable or easy to work with.
对于任何iOS开发人员而言,最臭名昭著的问题之一是:“我应该使用哪种架构?” 苹果公司的答案是MVC ,它代表Model-View-Controller。 这个想法是将原始数据(模型)与面向用户的实际视图分开。 两者通过控制器对象进行交互,该控制器对象负责读取和更新模型以及告诉视图要显示的内容。 这种体系结构引起的问题也称为MVC或Massive View Controller 。 由于视图控制器需要处理大量工作,因此最终将所有逻辑(业务,数据格式,视图更新)推到了其中,最终得到了数千行的文件。 伸缩性不是很好,也不容易使用。
Over the years, a number of alternative architectures have risen in popularity: the aforementioned MVVM, VIPER, and Clean Swift are some examples. I always found it odd that though all of these are far better than MVC, Apple never changed their tutorials or guides to endorse a different architecture. SwiftUI solves this problem and makes the development process much easier. Now, developers are strongly persuaded through the actual framework itself to adopt a reactive architecture where state drives views.
多年以来,许多替代体系结构越来越流行:上述MVVM, VIPER和Clean Swift就是其中的一些示例。 我总是感到奇怪,尽管所有这些都比MVC更好,但是Apple从未更改其教程或指南以支持不同的体系结构。 SwiftUI解决了这个问题,并使开发过程更加轻松。 现在,开发人员已被完全说服他们通过实际框架本身采用了状态驱动视图的React式架构 。
3.很快。 (3. It’s Swift.)
Pun intended. I want this point to end my post, because it really is the most promising thing I see about SwiftUI. The iOS development process is far from perfect. It has taken me an extremely long time (4+ years) to become very comfortable with UIKit and the intricate processes that underlie any iOS app.
双关语意。 我希望这一点结束我的文章,因为这确实是我对SwiftUI看到的最有希望的事情 。 iOS开发过程远非完美。 我花了很长时间(4年以上)来熟悉UIKit和任何iOS应用程序基础的复杂流程。
But there were many instances during the development of RxCatFact that I was astounded by how fast and easy it was to code with SwiftUI. Things that would normally take many lines to code were achievable in a single one (animation by far is the most impressive). In addition, there are many things that suck in UIKit (like working with attributed strings) that have been greatly improved in SwiftUI.
但是在RxCatFact的开发过程中,有很多实例让我为使用SwiftUI编写代码的速度和便捷感到惊讶。 通常只需一行就可以完成很多事情的代码 (到目前为止,动画是最令人印象深刻的)。 此外,在UIKit中还有很多吸引人的东西(例如使用属性字符串),而SwiftUI中已大大改善了这些东西。
Out of the many programming languages I’ve used, Swift has a special place in my heart because I strongly resonate with its mission to make code readable and succinct and to make development easy and enjoyable. It’s incredibly satisfying to write a gorgeous line in Swift that you couldn’t in other languages. It’s a compiled and statically typed language, meaning you have a powerful compiler that double-checks your code, and you (usually) don’t have to worry about run-time crashes caused by silly errors.
在我使用过的许多编程语言中,Swift在我心中占有特殊的位置,因为我强烈共鸣它的使命,即使代码可读性和简洁性并使开发变得轻松愉快 。 在Swift中写出您用其他语言无法做到的华丽线条令人难以置信。 它是一种编译后的静态类型语言,这意味着您拥有一个功能强大的编译器,它会仔细检查您的代码,并且(通常)您不必担心由于愚蠢的错误而导致的运行时崩溃。
SwiftUI brings this philosophy to the entire process of iOS development, and as much as I hate change and relearning things, it is an incredibly exciting and promising framework. Though it is still very immature, if it ends up working it could really revolutionize what it means to be an iOS developer. But until then, I’ll be happy with my good ol’ MVVM, UIKit, and object-oriented programming.
SwiftUI将这一理念带入了iOS开发的整个过程,尽管我讨厌更改和再学习,但它却是一个令人难以置信的令人兴奋且充满希望的框架。 尽管它还很不成熟,但是如果最终成功了,它可能会彻底改变成为iOS开发人员的意义。 但是直到那时,我都会对我出色的MVVM,UIKit和面向对象的编程感到满意。
Thanks for reading, and remember: Cats do not think that they are little people. They think that we are big cats. This influences their behavior in many ways. Me-wow!
感谢您的阅读,并记住: 猫并不认为他们是小人物。 他们认为我们是大猫。 这从许多方面影响他们的行为。 哇!
翻译自: https://medium.com/@kevintan_35360/swiftui-vs-the-reactive-mvvm-ios-architecture-17d403848ac7
react mvvm