Welcome to The JS Bifrost, your pathway to a rock-solid foundation for a God-level JavaScript. Here is the next up in the series that throws light on memoization - let’s do it the dynamic programming way.
欢迎来到JS Bifrost,这是通往神级JavaScript的坚实基础的途径。 这是该系列中的下一篇文章,介绍了记忆化-让我们以动态编程的方式进行。
In computing, memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. - sources: Wikipedia.
在计算中 ,记忆是一种优化技术,主要用于通过存储昂贵的函数调用的结果并在再次出现相同的输入时返回缓存的结果来加速计算机程序 。 -资料来源:维基百科。
In a simpler words, Memoization is nothing but caching or storing the results in a memory.
用简单的话来说,记忆化只是将结果缓存或存储在内存中。
没有记忆 (Without Memoization)
Fibonacci is the best example to illustrate the memoization concept. Let’s create the function which finds out Fibonacci of 10.
斐波那契是说明记忆概念的最佳例子。 让我们创建一个找出10的斐波那契的函数。
This function is suitable for smaller values of ’n’. As you increase the value of ’n’, the performance decreases.
此函数适用于较小的“ n”值。 随着增加“ n”的值,性能会降低。
As you see in the logs below, to find out the Fibonacci of 10, the function is called 177 times. This is because the fibo() function is getting called multiple times to compute the same result for the same value.
如您在下面的日志中看到的,要找出10的斐波那契,该函数被调用177次。 这是因为fibo()函数被多次调用以针对相同的值计算相同的结果。
Consider, if value of ‘n’ is 4, then it’ll calculate fibo(3) and fibo(2), then fibo(3) calculate fibo(2) and fibo(1) and so on. I first thought, if ’n’ is 4 it’ll calculate only fibo(4), fibo(3), fibo(2) and fibo(1) with time complexity O(n) but nay !! The real time complexity for this is O(2^n).Let’s deep dive and check how to optimize this using memoization.
考虑一下,如果'n'的值为4,则它将计算fibo(3)和fibo(2),然后fibo(3)计算fibo(2)和fibo(1),依此类推。 我首先想到的是,如果“ n”为4,它将只计算具有时间复杂度O(n)的fibo(4),fibo(3),fibo(2)和fibo(1),但是不! 实时复杂度为O(2 ^ n)。 让我们深入研究并检查如何使用备忘录来优化此设置。
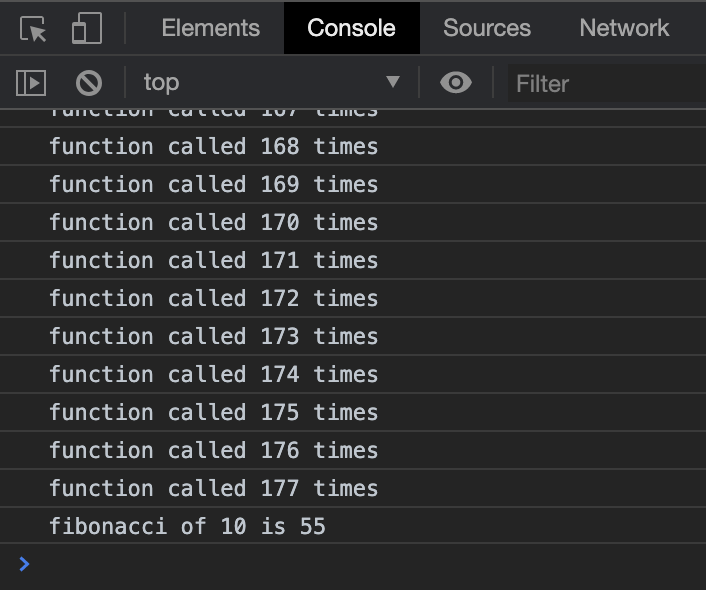
随着回忆 (With Memoization)
The problem of re-computing the same result for the same value can be resolved if the function remembers the result that it had computed previously.
如果函数记住先前已计算的结果,则可以解决针对相同值重新计算相同结果的问题。
See in the above example, we have created one “cache” variable in the self-executing anonymous function which returns an inner function f(). When f() is returned, its closure allows it to continue to access the “cache” object, which stores all of its previous results.
参见上面的示例,我们在自执行匿名函数中创建了一个“ cache”变量,该变量返回一个内部函数f()。 返回f()时,其关闭使其可以继续访问存储所有先前结果的“缓存”对象。
If the function executes with any value of ’n’, it will first check its results in the cache at line no. 6. If it exists, then it will return the results from the cache. Otherwise, it will execute the original Fibonacci function in the else part.
如果函数以“ n”的任何值执行,它将首先在高速缓存的第n行检查其结果。 6.如果存在,则它将从缓存中返回结果。 否则,它将在else部分中执行原始的Fibonacci函数。
As you see in the logs below, to find out the Fibonacci of 10, the function is called only 19 times. Because the function f() will execute only once for any value of ’n’ and time complexity with this approach is O(n), not O(2^n). Awesome right :)
如您在下面的日志中看到的,要找出10的斐波那契,该函数仅被调用19次 。 因为函数f()对于任何'n'值仅执行一次,并且此方法的时间复杂度为O(n),而不是O(2 ^ n)。 很棒的权利:)

Another example of memoization that finds the sum of ’n’ numbers where the function consistently receives the same arguments.
记忆的另一个示例是在函数始终接收相同参数的情况下,查找“ n”个数字的总和。

As you see in the above example and in the console logs, when it invokes the function for the first time to find out the sum of ’n’ number, it calculates and stores its results into the cache.
如您在上面的示例和控制台日志中所看到的,当它第一次调用该函数以查找'n'的总和时,它将计算其结果并将其存储到缓存中。
Later when it receives the same argument, it returns the result from the cache instead of re-computing the same, which saves the time and improves the performance.
稍后,当它接收到相同的参数时,它将从缓存中返回结果,而不是重新计算该结果,从而节省了时间并提高了性能。
何时以及如何使用记忆 (When and How to use memoization)
- Use memoization when the application performs heavy, time-consuming tasks, therefore storing the result might help you to save some processing time. 当应用程序执行繁重且耗时的任务时,请使用备忘录,因此存储结果可能有助于您节省一些处理时间。
- Use it when the function receives the same arguments multiple times, which calculates the same results each time. With memoization, it saves time from re-calculating it. 当函数多次接收相同的参数时,使用它,每次计算相同的结果。 使用备忘录,可以节省重新计算的时间。
- Try to divide big functions into the smaller ones to achieve the benefits of memoization. 尝试将大功能划分为较小的功能,以实现记忆化的好处。
- Don’t do memoization if you don’t see increase in the performance after implementing it, this will save some memory space too. 如果在实现后没有看到性能提升,请不要做备忘录,这也会节省一些内存空间。
结论 (Conclusion)
With memoization, we stop functions being called multiple times to calculate the same results for the same values and improves the performance by saving the time from re-calculating it over and over again.
有了备忘录,我们就停止了多次调用函数以针对相同的值计算相同的结果,并通过节省一次又一次地重新计算它的时间来提高性能。
Those who can not remember the past are condemned to repeat it. - George Santayana.
那些不记得过去的人会被谴责重复 过去 。 -乔治·桑塔亚娜(George Santayana)。
Stick around for more from our ‘The JS Bifrost’ Series.
请继续关注我们 的“ JS Bifrost” 系列。
资源资源 (Resources)
https://www.sitepoint.com/implementing-memoization-in-javascript/
https://www.sitepoint.com/implementing-memoization-in-javascript/
https://www.freecodecamp.org/news/understanding-memoize-in-javascript-51d07d19430e/
https://www.freecodecamp.org/news/understanding-memoize-in-javascript-51d07d19430e/
翻译自: https://medium.com/globant/the-js-bifrost-memoization-it-is-65f890f14308