We all hear a lot of good things about GraphQl and we all are aware of the benefits which we get using that. In short, we can say GraphQl as the query language for APIs at runtime for fulfilling those queries with the existing data. Its kind of selective querying of data. If we use graphQl combined with the Typescript it provides a better type safety with your graphQl queries, giving us end to end type safety. I will further deep dive into the Type Generation on Front End later in the next blog. I got a chance to get hands on to all these professionally and I would say it has so much of a learning curve that I still get to learn a lot of stuff everyday 🙂.
我们都听到了有关GraphQl的很多好消息,并且我们都知道使用它会带来的好处。 简而言之,我们可以说GraphQl作为运行时API的查询语言,用于使用现有数据来完成那些查询。 它是数据的选择性查询。 如果我们将graphQl与Typescript结合使用,它将为您的graphQl查询提供更好的类型安全性,从而提供端到端类型安全性。 稍后在下一个博客中,我将进一步深入探讨前端的Type Generation。 我有机会专业地掌握所有这些知识,我想说它的学习曲线是如此之多,以至于我每天仍然可以学习很多东西🙂。
So before continuing on the blog further, I want to call out one thing for the backend I am going with NodeJs, but GrapQL is a wrapper layer on top of our backend so we are free to go with creating our API’s in any other language.
因此,在继续浏览博客之前,我想为NodeJs后端做一件事,但是GrapQL是后端上的包装层,因此我们可以自由地使用任何其他语言来创建API。
So, just check for node installed in your machine by running — node -v
因此,只需运行以下命令即可检查计算机中是否已安装node -v
So the very first step would be itself a big one to Create an API in NodeJs and MongoDB this could be again a very big topic but will try to keep this not long
因此,第一步本身将是在NodeJs和MongoDB中创建API的重要一步,这可能再次成为一个非常大的话题,但将尝试保持很长时间
I personally feel Express is a really cool Node framework that’s designed to help JavaScript developers create servers really quickly on the fly.
我个人认为Express是一个非常酷的Node框架,旨在帮助JavaScript开发人员真正快速地创建服务器。
So let me put out the basic use case I am trying to solve which is the restaurant's list page
因此,让我提出我要解决的基本用例,这是餐厅的列表页面
It has the following steps -
它具有以下步骤-
Initialize the App —
npm install -g express-generator
初始化应用程序—
npm install -g express-generator
Installing Express —
npm install express --save
安装Express —
npm install express --save
- creating the index.js file and importing the express library and initializing the app to run - 创建index.js文件并导入Express库并初始化应用程序以运行-
var express = require("express");
var app = express();app.listen(3000, () => {
console.log("Server running on port 3000");
});
4. Let's create a simple API which would just return some cities name -
4.让我们创建一个简单的API,该API仅返回一些城市名称-
app.get("/url", (req, res, next) => {
res.json(["Bangalore","Varanasi","Mirzapur","Delhi","Bombay"]);
});
5. Let's run our App -
5.让我们运行我们的应用程序-
node index.js
node index.js
6. let's go the Browser
6.让我们去浏览器
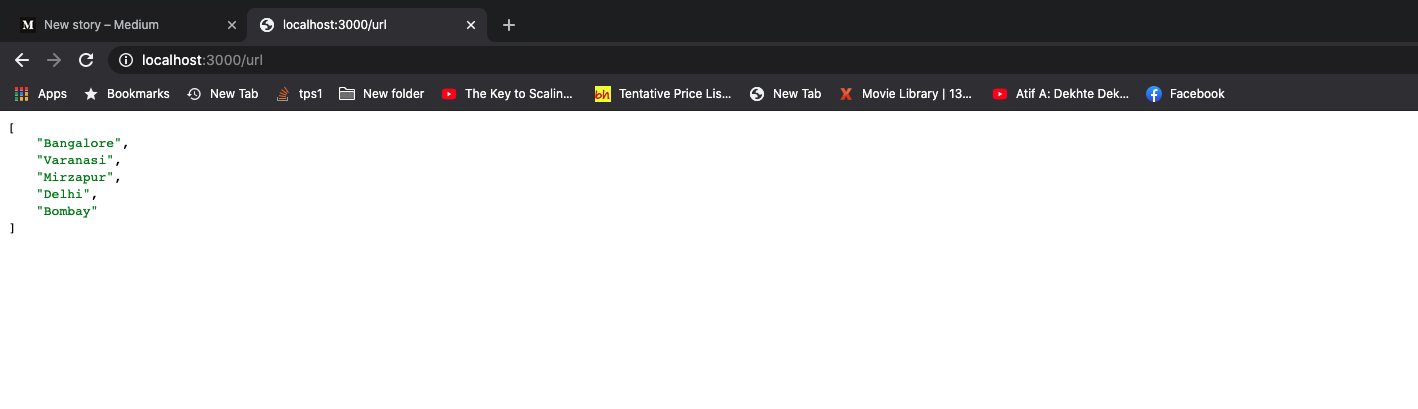
Yay! Was not that too quick and simple😅
好极了! 并不是那么简单快捷吗😅
Now let's See How can we add GraphQl layer on top of this but before adding that let's get to know few terms usually we encounter while using GraphQl
现在让我们看看如何在此之上添加GraphQl层,但是在添加之前,让我们了解使用GraphQl时通常遇到的几个术语
GraphQL Query
GraphQL查询
A query is a way that a client can read and manipulate data from the API. You can pass the type of an object and select which kind of fields you want to receive back. A simple query would be like the following:
查询是客户端可以从API读取和操作数据的一种方式。 您可以传递对象的类型,然后选择要接收的字段类型。 一个简单的查询如下所示:
query{
restaurants{
name,
address,
ratings,
averageRatings }
}
The result might be something like this —
结果可能是这样的-
{
"data": {
"restuarants": [{
"name": "Ksheer Sagar",
"address": "Bhelupur, Varanasi",
"averageRatings": "5",
"ratings": [{
"date": "25-12-2019",
"value": 5
},
{
"date": "25-02-2020",
"value": 5
}
]
},
{
"name": "Bikaner Wala",
"address": "Sunderpur, Varanasi",
"averageRatings": "4",
"ratings": [{
"date": "26-12-2019",
"value": 4
},
{
"date": "23-02-2020",
"value": 4
}
]
}
]
}
}
Now let's write some code to create Schema in NodeJs
现在让我们写一些代码在NodeJs中创建Schema
import express from 'express';
import graphqlHTTP from 'express-graphql';
import {
makeExecutableSchema
} from 'graphql-tools';
import { Restuarant } from 'schema/restuarant';const app: express.Application = express();
const port = 3000;let typeDefs: any = [`
type Restuarant {
name: String,
address: String,
ratings: Rate,
averageRating: Number,
restuarantID: ID!
}
type Query {
restuarants : [Restuarant]
}
type ratings {
value: Int!
date: Date!
}
type Mutation {
updateResturantRating(rating: Rate,restuarantID: ID!) : Restuarant
}
`];let resolvers = {
Query: {
fetchresturantsList: () => {
let restuarants = Restuarant.find({}, function(err, restuarants) {
if (err) {
return new Error('Internal server error');
}
return res.json(restuarants);
});
}
}
};
app.use(
'/graphql',
graphqlHTTP({
schema: makeExecutableSchema({
typeDefs,
resolvers
}),
graphiql: true
})
);
app.listen(port, () => console.log(`Node Graphql API listening on port ${port}!`));
Mongo Db Schema
Mongo Db模式
const mongoose = require('mongoose');
let restuarantSchema = mongoose.Schema({
name: {
type: String,
required: true
},
address: {
type: String,
required: true
},
ratings: {
type: Object,
required: true
},
averageRating: {
type: Number,
required: true
},
restuarantID: {
type:
auto: true
}
});
let Restuarant = module.exports = mongoose.model('Restuarant', restuarantSchema);
Basic Setup Of The Mongo DB -
Mongo DB的基本设置-
Steps
脚步
mongo
run this in Terminalmongo
在终端中运行To create and select a database :
use nodemongo
创建和选择数据库:
use nodemongo
db.createCollection('restuarants')
— will create a Collectiondb.createCollection('restuarants')
-将创建一个Collection- Let's insert Some records already mentioned above - 让我们插入一些上面已经提到的记录-
db.
"name": "Ksheer Sagar",
"address": "Bhelupur, Varanasi",
"averageRatings": "5",
"ratings": [{
"date": "25-12-2019",
"value": 5
},
{
"date": "25-02-2020",
"value": 5
}
]
});db.
"name": "Bikaner Wala",
"address": "Sunderpur, Varanasi",
"averageRatings": "4",
"ratings": [{
"date": "26-12-2019",
"value": 4
},
{
"date": "23-02-2020",
"value": 4
}
]
})
5. Verify the entered data- db.restuarants.find().pretty()
5.验证输入的数据db.restuarants.find().pretty()
Now Comes The Turn of Front End I really wanted to include the Frontend Part here itself but the blog would become long enough.
现在轮到前端了,我真的很想在其中包括前端部分,但是博客将变得足够长。
I would provide the short summary of the Front End part that I would cover, I will be using Typescript with ApolloClient in the Front End. I will use Apollo Codegen to generate Type definitions for the Schema in the Typescript.
我将提供有关前端部分的简短摘要,我将在前端将Typescript与ApolloClient一起使用。 我将使用Apollo Codegen为Typescript中的模式生成Type定义。