函数式编程语言
All the cool kids know functional programming. When object-oriented programmers try to explore or learn it, functional concepts might seem impractical, especially if they never incorporated functional concepts in their day-to-day programming.
所有很酷的孩子都知道函数编程。 当面向对象的程序员尝试探索或学习它时,功能概念似乎不切实际,尤其是如果他们从未在日常编程中纳入功能概念。
When curiosity and fear-of-missing-out eventually override practicality, object-oriented programmers look to either:
当好奇心和对遗漏的恐惧最终超越了实用性时,面向对象的程序员会选择以下任一种:
- learn a new functional programming language from scratch or 从头开始学习一种新的函数式编程语言,或者
- learn from functional constructs in languages they are comfortable with. 从他们熟悉的语言的功能构造中学习。
Each path has its limitations. The first choice puts emphasis on the language rather than concepts and the second choice is messy due to the short-comings and caveats of loosely implemented functional features in general purpose languages.
每个路径都有其局限性。 第一种选择侧重于语言而不是概念,而第二种选择则是混乱的,这是由于通用语言中松散实现的功能特性的不足和警告。
There is no bad choice to get started on learning. However, there is a reprieve for you folks who are thinking of option 1 — Scheme
开始学习没有坏选择。 但是,正在考虑备选方案1的人们可以放宽心
什么是计划? (What is Scheme?)
Scheme, according to wikipedia, is “a minimalist dialect of the Lisp family of programming languages”. Lisp is an expression oriented language that existed decades before the first object oriented languages were invented. The Scheme dialect distills Lisp into the most basic constructs needed to execute a program.
根据维基百科,Scheme是“ Lisp编程语言家族的极简方言”。 Lisp是一种面向表达的语言,在发明第一种面向对象的语言之前已经存在了数十年。 Scheme方言将Lisp提炼为执行程序所需的最基本的构造。
构造和关键字 (Constructs and keywords)
Here is an example of a Scheme expression to add two numbers:
这是一个将两个数字相加的Scheme表达式的示例:
(+ 2 3)Output: 5
This will seem absurd at first. You would have expected the operation to look like 2 + 3 but this absurdity is also the simplicity of Scheme. You see, the “+” is not an operator, it is a function. The “+” function adds the inputs given to it. For you CompSci savvy people, this is the prefix notation as opposed to the infix “2+3” notation used commonly in modern languages.
起初这似乎是荒谬的。 您可能希望运算看起来像2 + 3,但是这种荒谬之处也是Scheme的简单性。 您会看到,“ +”不是运算符,而是一个函数。 “ +”功能将为其提供输入。 对于精通CompSci的人们,这是前缀表示法,与现代语言中常用的中缀“ 2 + 3”表示法相反。
These basic functional expressions can be nested within each other, forming new expressions. Here is another example:
这些基本功能表达式可以相互嵌套,形成新的表达式。 这是另一个示例:
(/ (* 10 2) (+ 2 3))Output:4 - divide the result of (* 10 2) with result of (+ 2 3) which is 20 divided by 5
With these expressions, we are already using function composition and first-order functions! Imagine the code you write to express a concept like this in JavaScript.
通过这些表达式,我们已经在使用函数组合和一阶函数! 想象一下您编写的代码来表达JavaScript中的类似概念。
Scheme has keywords, like all languages do. Here are some of the common ones used in the majority of the Scheme programs. The following examples don’t go into the finer details but they just highlight how it is used.
像所有语言一样,Scheme具有关键字。 以下是大多数Scheme程序中使用的一些常见程序。 下面的示例不涉及更详细的信息,而仅强调其用法。
define — for naming values; what one might call “user-defined functions”.
define —用于命名值; 所谓的“用户定义函数”。
(define (square x) (* x x))
(square 5)Output: 25
- creates a function named "square" and calls it with value 5
lambda — does the same thing as above but without it giving a name. Why we need such a feature will become apparent in a later example. Incidentally, this is where the lambda of Python, C# and other languages came from.
lambda —与上述相同,但未给出名称。 在后面的示例中,我们为什么需要这样的功能将变得显而易见。 顺便说一句,这就是Python,C#和其他语言的lambda的来源。
((lambda (x) (* x x)) 5)Output: 25
cond, if, and, or, not, else — conditional branching stuff
继续,如果有,和/或没有,则有条件分支的东西
(if (> 5 6) 4 (+ 7 1))Output: 8
- if 5 is greater than 6 then give 4 otherwise give (+ 7 1)
cons, car, cdr, list, ‘ (single quote) — these are used to create and produce lists. Lisp stands for List Processor after all, so lists are heavily used.
缺点,汽车,cdr,列表,'(单引号) —这些用于创建和产生列表。 Lisp毕竟代表列表处理器,因此列表被大量使用。
(list 1 2 3 4)
Output: (1 2 3 4)'(1 2 3 4)
Output: (1 2 3 4)
- the single quote is a lisp shorthand for list
do, map, filter — looping and recursion. Note that functional languages don’t really use loops like iterative languages do. Recursion is preferred instead.
做,映射,过滤 -循环和递归。 请注意,功能语言并不像迭代语言那样真正使用循环。 首选递归。
(map (lambda (x) (* x x)) '(1 2 3 4 5))Output: (1 4 9 16 25)
- the lambda from before, executed on every item in (1 2 3 4 5)
There are more subtle lexicons, but as we see here, the minimalism of the language is quite evident. The examples specifically avoided iterative programming concepts like variable assignments because they are rarely used.
还有更多微妙的词典,但是正如我们在此处看到的那样,该语言的极简主义非常明显。 这些示例特别避免了诸如变量赋值之类的迭代编程概念,因为它们很少使用。
Also, these programs tend to include a lot of parenthesis (). For some, it makes the program look ugly but with the right IDE, it won’t be a problem. Which lead us to ..
而且,这些程序往往包含很多括号()。 对于某些人来说,它使程序看起来很丑陋,但是如果使用了正确的IDE,就不会有问题。 导致我们..
开发设置 (Development Setup)
Playing with a language involves writing code and that code has to run somewhere. Where do you get IDE and compilers for it?
使用某种语言进行游戏涉及编写代码,并且该代码必须在某个地方运行。 您从哪里获得IDE和编译器?
The simplest way to get started is Dr Racket. Racket is a language of its own (another dialect of Lisp) but it supports the Scheme compiler as a subset and provides rich auto-formatting, static code analysis, and debugging features out-of-the-box.
最简单的入门方法是Racket博士 。 Racket是一种自己的语言(Lisp的另一种方言),但是它支持Scheme编译器作为子集,并提供了现成的丰富的自动格式化,静态代码分析和调试功能。
In order to set Scheme as the preferred language, type the following in the workspace and either click on Run from the options bar or press Ctrl+r.
为了将Scheme设置为首选语言,请在工作区中键入以下内容,然后从选项栏中单击Run或按Ctrl + r。
#lang scheme
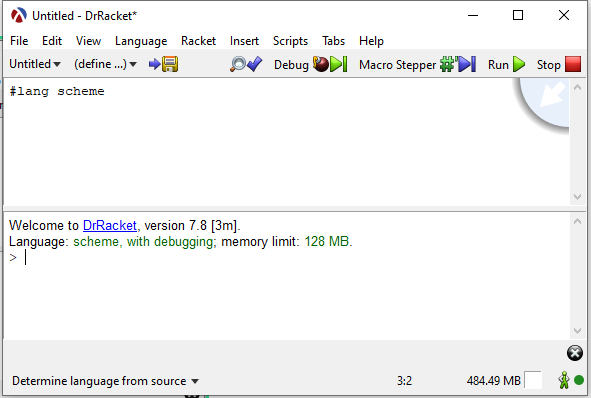
Et Violà! You have an IDE to run Scheme. Let’s test this by running the last example from above to see the output. If you choose to type the code, pressing enter after keywords would neatly auto-indent the program.
维奥拉! 您有一个IDE可以运行Scheme。 让我们通过从上面运行最后一个示例来查看输出,以进行测试。 如果您选择键入代码,则在关键字后按Enter键将使程序自动缩进。
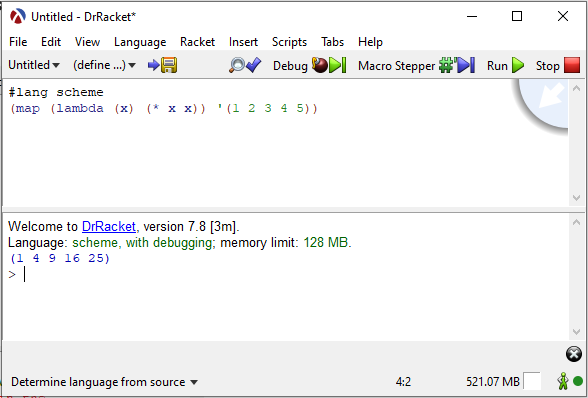
样例程序 (Sample Program)
Let’s expand on what we know so far and write a simple program to calculate the factorial of a given number.
让我们扩展到目前为止的知识,并编写一个简单的程序来计算给定数字的阶乘。
The factorial of a number N is defined by this mathematical expression below:
N的阶乘由以下数学表达式定义:
Factorial(N) = N * (N-1) * (N-2) * …. * 1
Expressed in a different mathematical formula, we can say
用不同的数学公式表示,我们可以说
Factorial(N) = N * Factorial(N-1) ; when N is not 1
= 1 ; when N is 1
Here is the code to calculate the factorial in Scheme. The program defines a factorial function and uses it to calculate the factorial of number 5.
这是在Scheme中计算阶乘的代码。 该程序定义了阶乘函数,并使用它来计算数字5的阶乘。
#lang scheme(define (factorial N)
(if (= N 1)
1
(* N (factorial (- N 1)))))(factorial 5)
Notice similarity of the mathematical expression and the corresponding expression in Scheme.
注意Scheme中数学表达式和相应表达式的相似性。
下一步 (Next Steps)
If you are sold on using Scheme to learn functional programming, I encourage you to get the book “Structure and Implementation of Computer Programs”, otherwise known as the SICP book, to continue learning. Another popular recommendation is “How to Design Programs”.
如果您被出售使用Scheme学习功能编程,我建议您拿本书“ 计算机程序的结构和实现 ”(也称为SICP书)继续学习。 另一个流行的建议是“ 如何设计程序 ”。
Dev tip — it would be sufficient to go over the first three sections of the SICP book to learn 90% of practical functional programming including mutability, higher order functions, streams (which is what Observables in RxJS are about), lambdas, closures, and much more. Personally, I found the last two sections of SICP to be a bit arcane for a web applications programmer like myself, but it might interest other species of programmers.
开发技巧-只需阅读SICP本书的前三部分,就可以学习90%的实用函数式编程,包括可变性,高阶函数,流(这是RxJS中的Observables所涉及的),lambda,闭包和多得多。 就我个人而言,我发现SICP的后两个部分对于像我这样的Web应用程序程序员来说有点不可思议,但是它可能会让其他种类的程序员感兴趣。
Scheme is great for students to get comfortable with functional programming but due to its minimal nature, it doesn’t scale for very large projects. However, learning Scheme will open the doors to more advanced Lisp dialects that come with pre-implemented libraries for common utilities. The DrRacket IDE supports Racket and Common Lisp, which are other powerful dialects of Lisp. The macros in Common Lisp for instance, are impossible to imitate with other languages. Java programmers could leverage learning Clojure to integrate functional programming into existing projects.
Scheme非常适合让学生熟悉函数式编程,但由于它的性质极小,因此无法用于大型项目。 但是,“学习计划”将为更高级的Lisp方言打开大门,这些方言带有预实现的公用程序库。 DrRacket IDE支持Racket和Common Lisp,这是Lisp的其他强大方言。 例如,Common Lisp中的宏无法与其他语言一起模仿。 Java程序员可以利用学习Clojure将功能性编程集成到现有项目中。
翻译自: https://medium.com/swlh/the-easiest-language-to-learn-functional-programming-5cc5751a7cf0
函数式编程语言