We often scan papers to convert them into images. We have various tools available online to enhance those images to make them brighter and remove any shading in those images. What if we can do that shading removal manually? We could just load any image as a gray-scale image into our code and obtain a output within seconds without the help of any app.
我们经常扫描纸张以将其转换为图像。 我们在线提供了各种工具来增强这些图像,使其更亮并消除这些图像中的任何阴影。 如果我们可以手动删除阴影怎么办? 我们可以将任何图像作为灰度图像加载到我们的代码中,无需任何应用程序即可在几秒钟内获得输出。
This can be achieved using basic Numpy manipulations and a few open CV functions. To explain the process the following image is used which is clicked from a phone.
这可以使用基本的Numpy操作和一些打开的CV函数来实现。 为了说明该过程,使用了以下图像,可从电话中单击该图像。
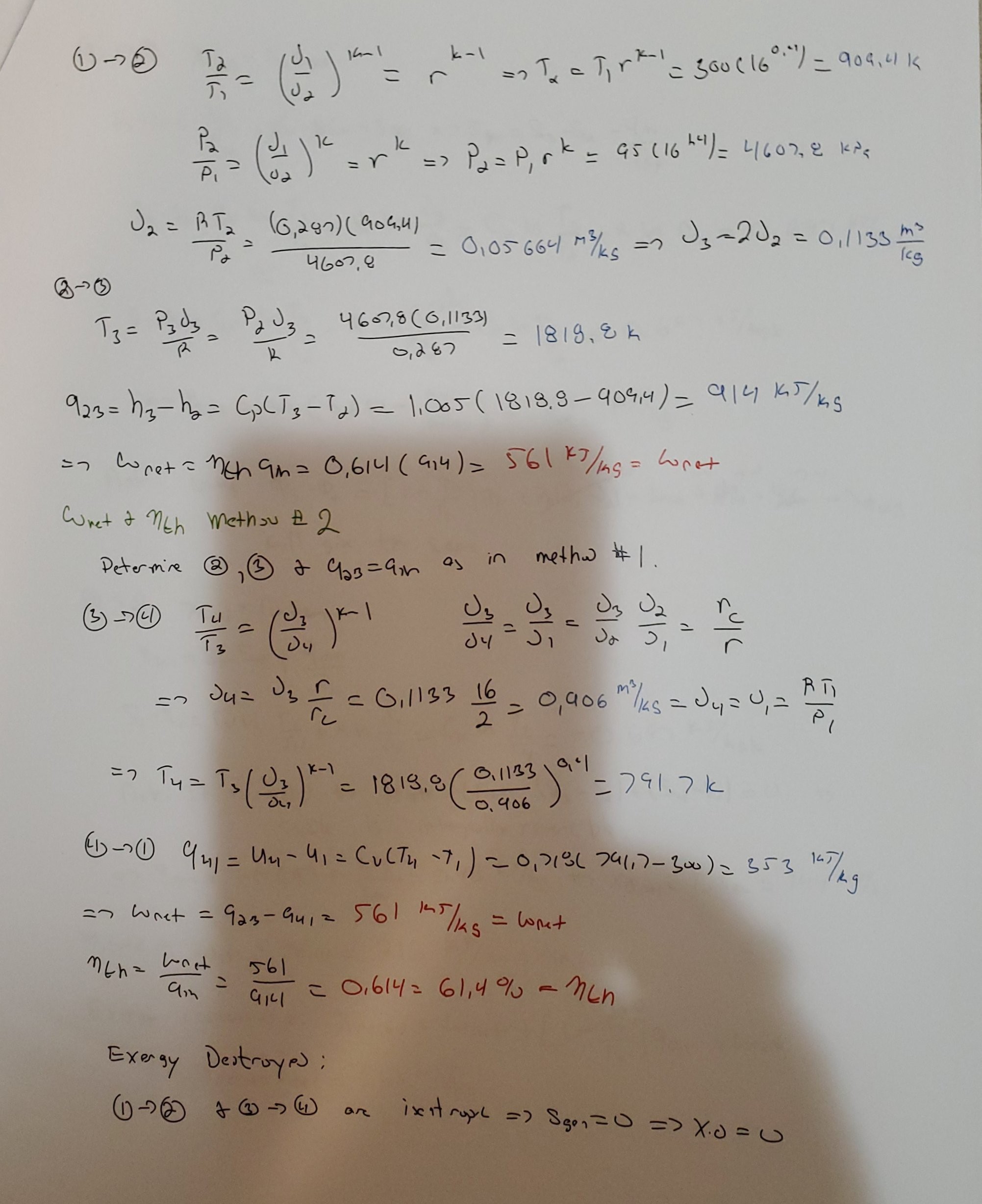
There is a definite shading which needs to be removed. Let’s get started.
有一个明确的阴影需要删除。 让我们开始吧。
- Import the necessary packages into your environment. For easy display of images Jupyter Notebook is being used. 将必要的软件包导入您的环境。 为了易于显示图像,正在使用Jupyter Notebook。
import cv2
import numpy as np
import matplotlib.pyplot as plt
2. There are 2 things to note while performing the shading removal. As the image is a gray-scale image, if the image has a light background and darker objects, we have to perform max-filtering first, followed by min-filtering. If the image has a dark background and light objects, we can perform min-filtering first and then proceed with max-filtering.
2.删除阴影时,有两件事要注意。 由于图像是灰度图像,如果图像背景较浅且对象较暗,则必须先执行最大过滤,然后再执行最小过滤。 如果图像背景较暗且物体较亮,我们可以先执行最小过滤,然后再进行最大过滤。
So what exactly is max-filtering and min-filtering?
那么,最大过滤和最小过滤到底是什么?
3. Max-filtering: Let us assume we have an image I of a certain size. The algorithm that we write should go through the pixels of I one by one, and for each pixel (x,y) it must find the maximum gray value in a neighborhood (a window of size N x N) around that pixel, and write that maximum gray value in the corresponding pixel location (x,y) in A. The resulting image A is called a max-filtered image of input image I.
3. 最大滤波 :让我们假设我们有一定大小的图像I。 我们编写的算法应逐个遍历I的像素,并且对于每个像素(x,y),它必须找到该像素周围的邻域(大小为N x N的窗口)中的最大灰度值,并进行写操作A中相应像素位置(x,y)的最大灰度值。所得图像A称为输入图像I的最大滤波图像。
Lets implement this concept in code.
让我们在代码中实现这个概念。
- max_filtering() function takes in the input image and the window size N. max_filtering()函数接受输入图像和窗口大小N。
- It initially creates a ‘wall’ (pads with -1) around the input array which would help us when we iterate through edge pixels. 它最初在输入数组周围创建一个“墙”(带有-1的填充),当我们遍历边缘像素时会有所帮助。
- We then create a ‘temp’ variable to copy our calculated max values into it. 然后,我们创建一个“ temp”变量,将计算出的最大值复制到该变量中。
- We then iterate through the array and create a window around the current pixel of size N x N. 然后,我们遍历数组,并围绕当前像素大小N x N创建一个窗口。
- We then calculate the max value in that window using ‘amax()’ function and write that value in the temp array. 然后,我们使用“ amax()”函数在该窗口中计算最大值,并将该值写入temp数组。
- We the copy this temp array into the main array A and return this as the output. 我们将该临时数组复制到主数组A中,并将其作为输出返回。
- A is the max-filtered image of input I. A是输入I的最大滤波图像。
def max_filtering(N, I_temp):
wall = np.full((I_temp.shape[0]+(N//2)*2, I_temp.shape[1]+(N//2)*2), -1)
wall[(N//2):wall.shape[0]-(N//2), (N//2):wall.shape[1]-(N//2)] = I_temp.copy()
temp = np.full((I_temp.shape[0]+(N//2)*2, I_temp.shape[1]+(N//2)*2), -1)
for y in range(0,wall.shape[0]):
for x in range(0,wall.shape[1]):
if wall[y,x]!=-1:
window = wall[y-(N//2):y+(N//2)+1,x-(N//2):x+(N//2)+1]
num = np.amax(window)
temp[y,x] = num
A = temp[(N//2):wall.shape[0]-(N//2), (N//2):wall.shape[1]-(N//2)].copy()
return A
4. Min-filtering: This algorithm is exactly the same as max-filtering but instead of finding the maximum gray values in the neighborhood, we find the minimum values in the N x N neighborhood around that pixel, and write that minimum gray value in (x,y) in B. The resulting image B is called a min-filtered image of the image I.
4. 最小过滤 :此算法与最大过滤完全相同,但是我们没有找到附近的最大灰度值,而是找到了该像素周围N x N附近的最小值,并将该最小灰度值写入B中的(x,y)。所得的图像B称为图像I的经过最小滤波的图像。
Lets code this function.
让我们对该函数进行编码。
def min_filtering(N, A):
wall_min = np.full((A.shape[0]+(N//2)*2, A.shape[1]+(N//2)*2), 300)
wall_min[(N//2):wall_min.shape[0]-(N//2), (N//2):wall_min.shape[1]-(N//2)] = A.copy()
temp_min = np.full((A.shape[0]+(N//2)*2, A.shape[1]+(N//2)*2), 300)
for y in range(0,wall_min.shape[0]):
for x in range(0,wall_min.shape[1]):
if wall_min[y,x]!=300:
window_min = wall_min[y-(N//2):y+(N//2)+1,x-(N//2):x+(N//2)+1]
num_min = np.amin(window_min)
temp_min[y,x] = num_min
B = temp_min[(N//2):wall_min.shape[0]-(N//2), (N//2):wall_min.shape[1]-(N//2)].copy()
return B
5. So, if a image has a lighter background, we want to perform max-filtering first which will give us a enhanced background and pass that max-filtered image into the min-filtering function which will take care of the actual content enhancement.
5.因此,如果图像的背景较浅,我们要先执行最大过滤,这将为我们提供增强的背景,并将该最大过滤后的图像传递到最小过滤功能中,该功能将负责实际的内容增强。
6. So, after performing the min-max filtering the values we obtain are not in the range of 0–255. So, we have to normalize the final array obtained using the background subtraction method which is subtracting the min-max filtered image with the original image to obtain the final image with the shading removed.
6.因此,执行最小-最大滤波后,我们获得的值不在0-255的范围内。 因此,我们必须归一化使用背景减法获得的最终阵列,该方法将原始图像减去最小-最大滤波图像,以获得去除阴影的最终图像。
#B is the filtered image and I is the original image
def background_subtraction(I, B):
O = I - B
norm_img = cv2.normalize(O, None, 0,255, norm_type=cv2.NORM_MINMAX)
return norm_img
7. The variable N which is the window size for filtering is to be altered for the size of the particles or content in your image. For the test image the size N=20 was chosen. The final output image after being enhanced looks like:
7.变量N(用于过滤的窗口大小)将根据图像中粒子或内容的大小进行更改。 对于测试图像,选择大小N = 20。 增强后的最终输出图像如下所示:
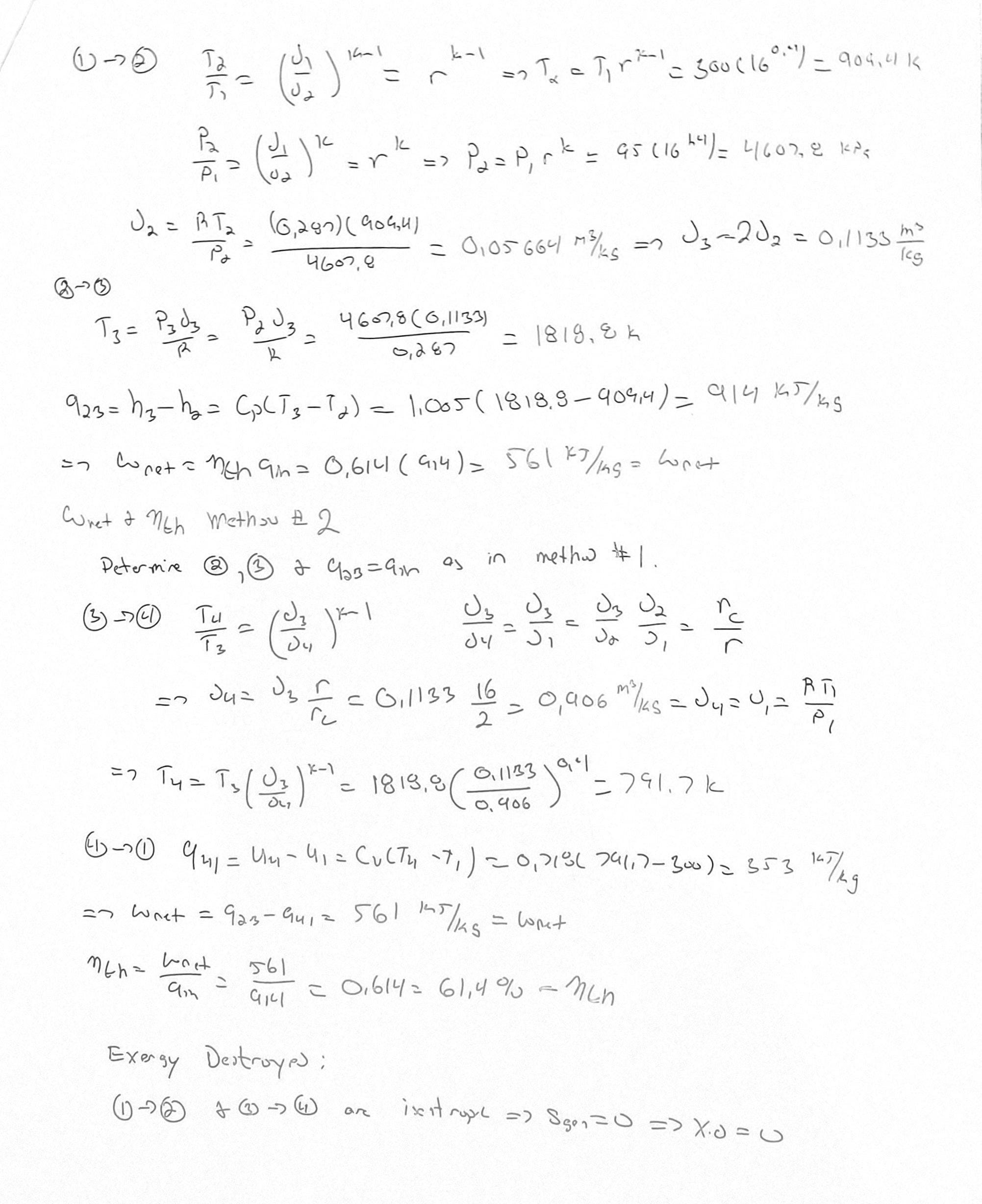
The output image is more enhanced than the original image. The code implemented is a humble attempt to manually implement some of the pre-existing functions in open CV to enhance images. The entire notebook with the images can be found in the Github link.
输出图像比原始图像更加增强。 所实现的代码是在开放CV中手动实现一些预先存在的功能以增强图像的谦虚尝试。 带有图像的整个笔记本可以在Github链接中找到。
翻译自: https://medium.com/@kavyamusty/enhancing-gray-scale-images-using-numpy-open-cv-9e6234a4d10d