c语言中指针的指针
While learning C/C++, you may have run over the term ‘Pointers’, and frequently heard that it is an idea difficult to comprehend. Pointers are very useful and powerful concept of C/C++ and, it isn’t that hard to comprehend. This article will introduce you with the real power of pointers in C.
在学习C / C ++时,您可能遇到过“指针”一词,并且经常听说这是一个很难理解的想法。 指针是C / C ++的非常有用且功能强大的概念,并且不难理解。 本文将向您介绍C语言中指针的真正功能。
指针简介 (Introduction To Pointer)
A pointer is a variable that contains a memory address of another variable. A pointer variable is declared to some types like other variable, so that it will work only with data of given type. Just as integer variable can hold integer data only, pointer variable can point to one specific data type(integer pointer can only hold the address of integer data). Pointer can have any name that is legal to any other variables but it is always preceded by asterisk(*) operator.
指针是一个变量,其中包含另一个变量的内存地址。 像其他变量一样,将指针变量声明为某些类型,因此它仅适用于给定类型的数据。 就像整数变量只能保存整数数据一样,指针变量也可以指向一种特定的数据类型(整数指针只能保存整数数据的地址)。 指针可以具有对任何其他变量合法的任何名称,但始终以asterisk(*)运算符开头。
指针的用途 (Uses of Pointer)
Pointer is used is Dynamic Memory Allocation.
使用的指针是动态内存分配。
- Pointer can be used to return multiple value from functions. 指针可用于从函数返回多个值。
- Pointer can be used to access the element of array. 指针可用于访问数组的元素。
- Pointer increase the execution speed of program. 指针提高了程序的执行速度。
- Pointer are used to create complex data structure. 指针用于创建复杂的数据结构。
指针声明 (Pointer Declaration)
句法: (Syntax:)
Data_type *Var;
例: (Example:)
int *x;
float *y;
char *z;
In the above example, ‘x’ is an integer pointer which can hold the address of integer data. Similarly, ‘y’ is a float pointer which can hold the address of float data, and ‘z’ is a character pointer which can hold the address of character data.
在上面的示例中,“ x”是一个整数指针,可以保存整数数据的地址。 同样,“ y”是一个浮点指针,可以保存浮点数据的地址,而“ z”是一个字符指针,可以保存字符数据的地址。
通过示例理解: (Understanding With Example:)
#include<stdio.h>
#include<stdio.h>
int main(){
int x; //Var Declaration
int *p; //Pointer Declaration
x = 69; //Var Initialization
p = &x; //Pointer Initialization
printf("Address is %d \n",&x);
printf("Address is %d \n",p);
printf("Value is %d \n",*p);
printf("Value is %d",x);
return 0;
}Output:Address is 59084068
Address is 59084068
Value is 69
Value is 69
让我们来说明一下 (Let’s Illustrate It)
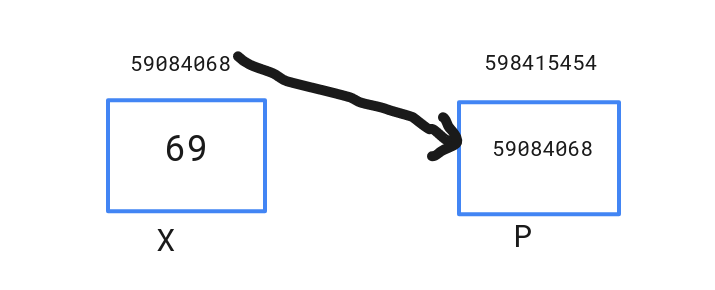
‘x’ is an integer variable and it’s value is 69. The variable p is pointer variable. p = &x assigns address of ‘x’ to ‘p’. ‘p’ is a pointer to ‘x’. We can use ‘p’ to access the address and value of ‘x’.’p’ is noting but variable which store the memory address of ‘x’. *p is used to display the value stored at a memory location pointed by pointer variable ‘p’. OH!!! Crap So Confusing….
'x'是一个整数变量,其值为69。变量p是指针变量。 p =&x将地址“ x”分配给“ p”。 “ p”是指向“ x”的指针。 我们可以使用'p'来访问'x'的地址和值。'p'只是在存储变量'x'的变量。 * p用于显示指针变量“ p”指向的存储位置中存储的值。 哦!!! 真是令人困惑...
解引用运算符 (Dereference Operator)
The dereference operator (*) is a prefix operator that can be used with any pointer variable, like *p. This returns the value of the variable pointed at by that pointer.
解引用运算符(*)是前缀运算符,可以与任何指针变量(例如* p)一起使用。 这将返回该指针指向的变量的值。
地址运算符 (Address Operator)
The operator ‘&’ is known as address operator. This returns the address of variable. We have already used this in Scanf.
运算符“&”称为地址运算符。 这将返回变量的地址。 我们已经在Scanf中使用了它。
初始化指针 (Initializing Pointer)
Address of variable can be assigned to pointer variable. Address of pointer can be also stored in another pointer. Let’s see how to initialize pointer.
变量的地址可以分配给指针变量。 指针的地址也可以存储在另一个指针中。 让我们看看如何初始化指针。
int *p = &x;ORint *p; int *p = &x;ORint *p;
p = &x;
p = &x;
错误指针 (Bad Pointer)
With great power comes great responsibility. Pointers can make your system crash and corrupt memory locations if not handled properly. When a pointer variable is declared, it does not have any value(address) inside it. The pointer is uninitialized or bad pointer or wild pointer. You must assign some address to it. Sometimes it can lead to serious run time error.
强大的力量带来巨大的责任。 如果处理不当,指针会使系统崩溃并损坏内存位置。 声明指针变量时,其内部没有任何值(地址)。 指针未初始化或错误的指针或野生指针。 您必须为其分配一些地址。 有时会导致严重的运行时错误。
空指针 (Void Pointer)
A void pointer is also known as Generic pointer. Void pointer is an special type of pointer which can point to any data type. Typecasting is used to change void to any other data type.
空指针也称为通用指针。 无效指针是一种特殊的指针类型,可以指向任何数据类型。 类型转换用于将void更改为任何其他数据类型。
句法: (Syntax:)
Void *Var=;
空指针 (Null Pointer)
A null pointer is a type of pointer which points to nothing. We can define null pointer using constant NULL. A null pointer always contains value 0
空指针是一种不指向任何内容的指针。 我们可以使用常量NULL定义空指针。 空指针始终包含值0
指针数组 (Array of Pointers)
Like array of variables, we can create an array of pointers too. An array of pointer can be declared as: type *var[size];
像变量数组一样,我们也可以创建一个指针数组。 指针数组可以声明为:type * var [size];
例 (Example)
int *ptr[5];
This declare an array of 5 pointers, each points to a address of an integer data. It can be initialized as:
这声明了一个由5个指针组成的数组,每个指针都指向一个整数数据的地址。 可以将其初始化为:
int u=1,v=2,x=10,y=20,z=30int ptr[0] = &u;
int ptr[1] = &v;
int ptr[2] = &w;
int ptr[3] = &x;
int ptr[4] = &y;
双指针 (Double Pointer)
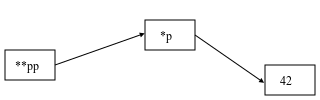
A pointer to a pointer is called a double pointer.
指向指针的指针称为双指针。
例 (Example)
#include<stdio.h>
int main()
{
int num = 69;
int *p1;
int **p2; // double pointer
p1 = #
p2 = &p1;
printf("Address of num = %d\n", p1);
printf("Address of p1 = %d\n", p2);
}
指针和数组 (Pointer and Array)
Arrays and pointers are highly linked in C/C++. Fully understanding the relationship between the two probably requires several days of study and experimentation, but it is well worth the effort.
数组和指针在C / C ++中高度链接。 完全了解两者之间的关系可能需要几天的研究和实验,但是值得付出努力。
Array name itself is an pointer. It points to the address of 0th element of an array. 1th element of array can be expressed as array name + 1 or simply as &array[1]. Similarly 2nd can be expressed as array name + 2 or as &array[2].
数组名称本身就是一个指针。 它指向数组第0个元素的地址。 数组的第1个元素可以表示为数组名+ 1,也可以简单表示为&array [1]。 类似地,第二可以表示为数组名+ 2或&array [2]。
例 (Example)
#include<stdio.h>
int main()
{
int arr[5] = {1,2,3,4,5};
printf("Addr of 0th is %d \n",arr);
printf("Addr of 1th is %d \n",arr+1);
printf("Addr of 2th is %d \n",arr+2);
printf("Addr of 3th is %d \n",arr+3);
printf("Addr of 5th is %d \n",arr+4);
}
将指针传递给函数 (Passing Pointer to Function)
A pointer can be passed to a function as an argument. When we pass a pointer as an argument instead of a variable then the address of the variable is passed instead of the value. So any change made by the function using the pointer is permanently made at the address of passed variable.This mechanism is known as Call by Reference or Call by Address.
可以将指针作为参数传递给函数。 当我们传递指针作为参数而不是变量时,则传递变量的地址而不是值。 因此,函数使用指针所做的任何更改都将在传递的变量的地址处永久进行。此机制称为按引用调用或按地址调用。
例 (Example)
#include <stdio.h>void swap(int*, int*); //Swap function declarationint main()
{
int x, y;printf("Enter the value of x and y\n");
scanf("%d%d",&x,&y);printf("Before Swapping\nx = %d\ny = %d\n", x, y);swap(&x, &y);printf("After Swapping\nx = %d\ny = %d\n", x, y);return 0;
}
void swap(int *a, int *b)
{
int temp; temp = *b;
*b = *a;
*a = temp;
}
指针和字符串 (Pointer and String)
A String is a sequence of characters stored in an array and arrays are highly connected with pointers, we can say strings and pointers are also highly connected to each other.
字符串是存储在数组中的一系列字符,数组与指针高度相关,可以说字符串和指针也高度相关。
例 (Example)
*char text[10] = "HelloBabe";
As string is a sequence of characters stored in an array, string variable is also a pointer to a first character of the string. Address of first char of string can be accessed as variable name(text). Similarly address of second char can be accessed as array name + 1.
由于string是存储在数组中的一系列字符,因此string变量也是指向该字符串第一个字符的指针。 字符串的第一个字符的地址可以作为变量名(文本)访问。 同样,第二个字符的地址可以作为数组名+ 1访问。
范例程序 (Example Programs)
1. C程序使用指针更改常量整数的值。 (1. C program to change the value of constant integer using pointers.)
#include <stdio.h>int main()
{ const int a=60;int *p;
p=&a;
printf("Before changing value of a: %d",a);
//assign value using pointer
*p=69;
printf("\nAfter changing value of a: %d",a); return 0;
}
2. C程序演示双指针示例 (2. C program to demonstrate example of double pointer)
#include <stdio.h>int main()
{int a; int *p1; int **p2;
p1=&a;
p2=&p1;
a=100;
//access the value of a using p1 and p2
printf("\nValue of a (using p1): %d",*p1);
printf("\nValue of a (using p2): %d",**p2);
//change the value of a using p1
*p1=200;
printf("\nValue of a: %d",*p1);
//change the value of a using p2
**p2=200;
printf("\nValue of a: %d",**p2);return 0;
}
Phew! Yeah, we’re finished. We completed learning basic of pointers. This article is done, but you shouldn’t be done with pointers. Play with them. Best Wishes.
! 是的,我们完成了。 我们完成了指针的学习基础。 本文已完成,但您不应使用指针。 和他们一起玩。 最好的祝愿。
翻译自: https://medium.com/@41414141/what-the-heck-is-pointer-in-c-c987378349a5
c语言中指针的指针