More and more mobile applications are utilizing power WebView to customize the user experience on runtime. And it is all the more important to track users’ activities on the WebView.
越来越多的移动应用程序正在利用强大的WebView来定制运行时的用户体验。 在WebView上跟踪用户的活动变得更加重要。
However, Firebase analytics’s SDK doesn’t support sending events from WebView pages of the mobile app. So to use Analytics in WebView, its events must be forwarded to native code before they can be sent to analytics.
但是,Firebase Analytics的SDK不支持从移动应用程序的WebView页面发送事件。 因此,要在WebView中使用Analytics(分析),必须先将其事件转发到本机代码,然后再将其发送到Analytics(分析)。
So, First, we have to pass the events from WebView to native code. Second, fetch the passed events in the native environment and send it to Firebase Analytics.
因此,首先,我们必须将事件从WebView传递到本机代码。 其次,在本地环境中获取传递的事件,然后将其发送到Firebase Analytics。
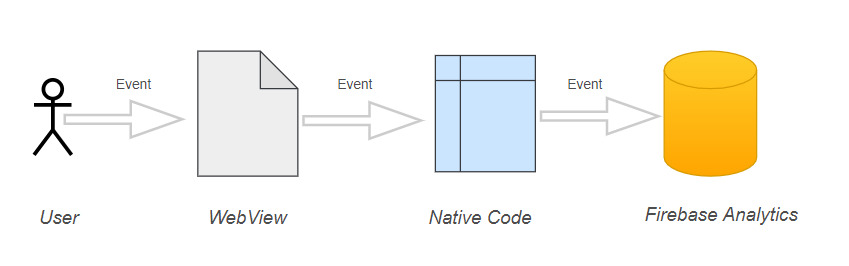
In this guide, I will show you the codes required to log WebView events to firebase analytics. And then I will touch on some of the example events to send from WebView to Firebase at the end of the article.
在本指南中,我将向您展示将WebView事件记录到Firebase分析所需的代码。 然后,在文章结尾,我将介绍一些示例事件,这些事件将从WebView发送到Firebase。
If you are not familiar with event tracking or are new to the analytics world here is a good beginner course on analytics to start with. And this course for more advanced analytics training.
使用Javascript事件处理程序从WebView发送事件 (Send Events from WebView Using Javascript Event Handler)
The following code creates a JavaScript function that can forward the WebView events to native code.
以下代码创建一个JavaScript函数,该函数可以将WebView事件转发到本机代码。
function logEvent(name, params) {
if (!name) {
return;
}
if (window.AnalyticsWebInterface) {
// Call Android interface
window.AnalyticsWebInterface.logEvent(name, JSON.stringify(params));
} else if (window.webkit
&& window.webkit.messageHandlers
&& window.webkit.messageHandlers.firebase) {
// Call iOS interface
var message = {
command: 'logEvent',
name: name,
parameters: params
};
window.webkit.messageHandlers.firebase.postMessage(message);
} else {
// No Android or iOS interface found
console.log("No native APIs found.");
}
}
function setUserProperty(name, value) {
if (!name || !value) {
return;
}
if (window.AnalyticsWebInterface) {
// Call Android interface
window.AnalyticsWebInterface.setUserProperty(name, value);
} else if (window.webkit
&& window.webkit.messageHandlers
&& window.webkit.messageHandlers.firebase) {
// Call iOS interface
var message = {
command: 'setUserProperty',
name: name,
value: value
};
window.webkit.messageHandlers.firebase.postMessage(message);
} else {
// No Android or iOS interface found
console.log("No native APIs found.");
}
}
The above code is all we need to handle the events for both iOS and Android. So it is all we need on WebView side. However, on the native side, we have to fetch the passed events in iOS and Android separately.
上面的代码是我们处理iOS和Android事件所需的全部内容。 因此,这是我们在WebView方面所需的全部。 但是,在本机方面,我们必须分别在iOS和Android中获取传递的事件。
以本机代码从Webview接收事件 (Receive Events from Webview in Native Code)
Next, we have to update the native code for both iOS and Android to collect the events from WebView and pass it to the firebase SDK. Here is how to do it.
接下来,我们必须更新iOS和Android的本机代码,以从WebView收集事件并将其传递给firebase SDK。 这是怎么做。
对于Android (For Android)
To invoke native Android code from JavaScript, implement a class with methods marked @JavaScriptInterface:
要从JavaScript调用本地Android代码,请使用标记为@JavaScriptInterface的方法实现一个类:
public class AnalyticsWebInterface {
public static final String TAG = "AnalyticsWebInterface";
private FirebaseAnalytics mAnalytics;
public AnalyticsWebInterface(Context context) {
mAnalytics = FirebaseAnalytics.getInstance(context);
}
@JavascriptInterface
public void logEvent(String name, String jsonParams) {
LOGD("logEvent:" + name);
mAnalytics.logEvent(name, bundleFromJson(jsonParams));
}
@JavascriptInterface
public void setUserProperty(String name, String value) {
LOGD("setUserProperty:" + name);
mAnalytics.setUserProperty(name, value);
}
private void LOGD(String message) {
// Only log on debug builds, for privacy
if (BuildConfig.DEBUG) {
Log.d(TAG, message);
}
}
private Bundle bundleFromJson(String json) {
// ...
}
}
Once you have created the native interface, register it with your WebView so that it is visible to JavaScript code running in the WebView:
创建本机接口后,请在WebView中注册它,以便WebView中运行JavaScript代码可以看到它:
// Only add the JavaScriptInterface on API version JELLY_BEAN_MR1 and above, due to
// security concerns, see link below for more information:
// https://developer.android.com/reference/android/webkit/WebView.html#addJavascriptInterface(java.lang.Object,%20java.lang.String)
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR1) {
mWebView.addJavascriptInterface(
new AnalyticsWebInterface(this), AnalyticsWebInterface.TAG);
} else {
Log.w(TAG, "Not adding JavaScriptInterface, API Version: " + Build.VERSION.SDK_INT);
}
Here is an example MainActivity.java to get you an idea about the class implementation.
这是一个示例MainActivity.java ,可让您了解类的实现。
对于iOS (For iOS)
To invoke native iOS code from JavaScript, create a message handler class conforming to the WKScriptMessageHandler
protocol. You can make Google Analytics calls inside the userContentController:didReceiveScriptMessage:
callback:
要从JavaScript调用本机iOS代码,请创建符合WKScriptMessageHandler
协议的消息处理程序类。 您可以在userContentController:didReceiveScriptMessage:
回调内部进行Google Analytics(分析)调用:
func userContentController(_ userContentController: WKUserContentController,
didReceive message: WKScriptMessage) {
guard let body = message.body as? [String: Any] else { return }
guard let command = body["command"] as? String else { return }
guard let name = body["name"] as? String else { return }
if command == "setUserProperty" {
guard let value = body["value"] as? String else { return }
Analytics.setUserProperty(value, forName: name)
} else if command == "logEvent" {
guard let params = body["parameters"] as? [String: NSObject] else { return }
Analytics.logEvent(name, parameters: params)
}
}
Finally, add the message handler to the webview’s user content controller:
最后,将消息处理程序添加到Webview的用户内容控制器中:
self.webView.configuration.userContentController.add(self, name: "firebase")
Here is an example viewController.swift file to get you the implementation idea. And see here is the objective-C code
这是一个示例viewController.swift文件,以帮助您实现实现。 看看这里是目标C代码
将事件从WebView发送到本机代码的示例代码 (Example code to send events from WebView to native code)
Consider the example events and parameters depicted in the below image that we will be triggered from WebView to pass to native code.
考虑下图所示的示例事件和参数,我们将从WebView触发事件并将其传递给本机代码。
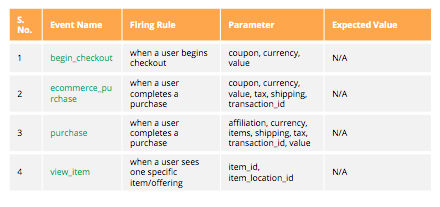
1-发送“ begin_checkout”事件 (1- Send “begin_checkout” event)
logEvent("begin_checkout", {
"coupon":"someCoupon",
"currency":"AED",
"value":100
});
2-发送“电子商务购买”事件 (2- Send “ecommerce_purchase” Event)
logEvent("ecommerce_purchase", {
"coupon":"someCoupon",
"currency":"AED",
"value":100,
"tax":10,
"shipping":10,
"transaction_id":orderId
});
3-发送“购买”事件 (3- Send “purchase” Event)
logEvent("purchase", {
"affiliation":"someAffiliation",
"currency":"AED",
"value":100,
"items":items,
"tax":10,
"shipping":10,
"transaction_id":orderId
});
4-发送“ view_item”事件 (4- Send “view_item” Event)
logEvent("view_item", {
"item_id":itemId,
"item_location_id":itemLocaitonId
});
结论 (Conclusion)
Firebase Analytics is a powerful analytics tool from Google to track and target users’ activities on mobile. However, it’s SDK doesn’t support sending events from WebView of the mobile. Therefore we have to pass the events from WebView to native code and send them from there.
Firebase Analytics是Google提供的功能强大的分析工具,可以跟踪和定位用户在移动设备上的活动。 但是,它的SDK不支持从手机的WebView发送事件。 因此,我们必须将事件从WebView传递到本机代码,然后从那里发送。
类似阅读 (Similar Read)
翻译自: https://medium.com/swlh/send-events-from-webview-to-firebase-analytics-28d278f0bf4d