elasticache
I have spent the last 18 month learning and using Redis. Redis is an awesome technology and it is made easier to use in the cloud with Amazon ElastiCache for Redis. I use Redis as more than just a cache, even though it is a great choice when you need to accelerate access to resources like files or database records. I use it as a resilient extension of my program memory, it includes implementation of common data structures like lists, sets and sorted sets. Because of its extreme performance and data type support, Redis is great for scenarios such as stream analysis and atomic increments. It is very easy to use Redis when you need to quickly aggregate data or deduplicate incoming records. But enough about Redis, by leveraging Serverless technologies like AWS Lambda and AppSync we can create applications that use Redis cheaply and quickly. In this blog post I will go through a practical example of Serverless technology together with Amazon ElastiCache for Redis.
最近18个月,我一直在学习和使用Redis 。 Redis是一项了不起的技术, Amazon ElastiCache for Redis使它更易于在云中使用。 尽管您需要加快对文件或数据库记录等资源的访问,这是一个不错的选择,但我不仅将Redis用作缓存。 我将其用作程序存储器的弹性扩展,它包括常见数据结构(如列表,集合和排序集合)的实现。 由于Redis具有出色的性能和数据类型支持,因此非常适合诸如流分析和原子增量之类的方案。 当您需要快速聚合数据或对传入记录进行重复数据删除时,使用Redis非常容易。 但是对于Redis而言,已经足够了,通过利用AWS Lambda和AppSync等无服务器技术,我们可以创建廉价,快速使用Redis的应用程序。 在此博客文章中,我将结合Amazon ElastiCache for Redis一起探讨无服务器技术的实际示例。
问题 (The problem)
I have been working on some projects recently where I used Redis (backed by DynamoDB) as an object store and a simple aggregation mechanism for processing realtime data streams. During the process I had to tackle a couple of challenges:
我最近一直在从事一些项目,在这些项目中,我使用Redis(由DynamoDB支持)作为对象存储和一种用于处理实时数据流的简单聚合机制。 在此过程中,我不得不应对一些挑战:
- I needed Public access to my Redis cluster over HTTP. Redis is using a TCP based protocol to communicate with clients and ElastiCache is configured by default inside an AWS VPC. With that in mind, if I wanted to access my Redis cluster from a mobile app or a browser I would have had to configure public access to the Redis port from my VPC. Also, by using HTTP in my client, I save myself from having to install and maintain local Redis libraries. 我需要通过HTTP对Redis集群进行公共访问。 Redis使用基于TCP的协议与客户端进行通信,并且默认情况下,在AWS VPC内配置了ElastiCache。 考虑到这一点,如果我想从移动应用程序或浏览器访问Redis群集,则必须配置从VPC对Redis端口的公共访问。 另外,通过在客户端中使用HTTP,我无需安装和维护本地Redis库。
I wanted to use Redis commands to access data instead of trying to create new abstractions on top of a perfectly good syntax.
我想使用Redis命令来访问数据,而不是尝试在完美语法的基础上创建新的抽象。
- I wanted the option to authorize the clients that connect to my Redis clusters from an untrusted source (e.g. the Internet). 我希望该选项授权从不受信任的来源(例如Internet)连接到我的Redis集群的客户端。
It also goes without saying that as a Cloud developer I expect to pay as little as possible and spend the least amount of time on setting up and installing my application.
毋庸置疑,作为一名云开发人员,我希望支付尽可能少的钱,并花费最少的时间来设置和安装我的应用程序。
解决方案 (The solution)
As you can see in the image, the solution ended up pretty simple. Let’s look at the pieces:
如您在图像中所见,解决方案最终变得非常简单。 让我们来看看碎片:
AWS AppSync — AppSync makes it easy to expose data sources as GraphQL endpoints, it also supports several authorization methods. The AppSync team recently announced Direct Lambda Resolvers reducing the number of steps it takes to map Lambda Functions to AppSync endpoints.
AWS AppSync-通过 AppSync,可以轻松将数据源公开为GraphQL端点,它还支持多种授权方法 。 AppSync团队最近宣布了Direct Lambda解析器,减少了将Lambda函数映射到AppSync端点所需的步骤。
AWS Lambda — AWS Lambda needs no introduction. It is a Serverless Functions service that manages all the aspects of running code in the cloud.
AWS Lambda-无需介绍。 它是一项无服务器功能服务,可管理云中运行代码的所有方面。
Amazon ElastiCahe — Amazon ElastiCache is fully managed in-memory datastore that supports the Memcached and Redis open source engines.
Amazon ElastiCahe — Amazon ElastiCache是完全托管的内存中数据存储,支持Memcached和Redis开源引擎。
Honorable mention: AWS Serverless Application Model (SAM) — I’ve spent some quality time with SAM recently automating my deployment scripts and after a bit of a learning-curve I was able create a repeatable deployment process that helped me build and test my code.
荣誉奖: AWS无服务器应用程序模型 (SAM)—最近,我花了一些时间在SAM上,使我的部署脚本实现了自动化,经过一些学习后,我能够创建一个可重复的部署过程,以帮助我构建和测试代码。
这个怎么运作 (How it works)
To expose Redis commands through AppSync we will need a schema (you will deploy it as part of your SAM template later on).
要通过AppSync公开Redis命令,我们需要一个架构(稍后将其部署为SAM模板的一部分)。
We are using a Mutation to send an array of commands (technically an array of arrays) to the Lambda Function where they parsed and executed inside a Redis pipeline.
我们正在使用Mutation向Lambda函数发送命令数组(技术上是数组数组),在Lambda函数中它们在Redis管道中进行解析和执行。
We are using a Query to read data from Redis (in pratice we are not testing whether the Redis commands passed to the Query are mutative or not).
我们正在使用查询从Redis读取数据(实际上,我们不测试传递给查询的Redis命令是否是可变的)。
type Mutation {
# Send an array of Redis commands and parameters to be executed together
putRedis(Command: [[String!]]): String
}
type Query {
# Send a single Redis command to fetch and array of items back
getRedis(Command: [String!]): [String]
}
schema {
query: Query
mutation: Mutation
}
Next, we map the Mutation and Query to our Lambda function by creating a Resolver for our Mutation and Query. Note that the resolvers are identical with the exception of the type of call to the Lambda function.
接下来,通过为突变和查询创建解析器 ,将突变和查询映射到Lambda函数。 请注意,解析器与Lambda函数的调用类型相同。
{
"version": "2017-02-28",
"operation": "Invoke",
"payload": {
"query": "RedisSet",
"context": $utils.toJson($context)
}
}
{
"version": "2017-02-28",
"operation": "Invoke",
"payload": {
"query": "RedisGet",
"context": $utils.toJson($context)
}
}
def lambda_handler(event, context):
#Check whether we are reading or writing
commandType = event["query"]
retVal = []
#Connect to Redis
r = get_redis_client()
if commandType == "RedisSet":
#If this is a mutation command, we expect an array of arrays, each containing a Redis command and paramters
pipe = r.pipeline()
#Create a Redis pipeline
for cmd in event["context"]["arguments"]["Command"]:
#Add commands into the pipeline
sCMD = makeArgStr(cmd)
pipe.execute_command(sCMD)
#Execute the set of commands
retVal = formatRedisReply(pipe.execute())
if commandType == "RedisGet":
#If this is a query, execute the Redis command and return the result(s)
sCMD = makeArgStr(event["context"]["arguments"]["Command"])
retVal = formatRedisReply(r.execute_command(sCMD))
#Close the Redis connection
r.close()
return retVal
放在一起 (Putting it all together)
This section will walk you through downloading, installing and testing this solution.
本节将引导您完成下载,安装和测试此解决方案的过程。
Requirements* Git installed * AWS CLI installed and configured with Administrator permission.* SAM CLI — Install the SAM CLI* Python 3.8.x is not required to run the sample but will be needed to make changes in the Lambda function
要求 * Git的安装 * AWS CLI 安装和使用管理员权限配置* SAM CLI - 安装SAM CLI * 的Python 3.8.x不需要运行示例,但将需要作出lambda函数的变化
Downloading and installingClone this git repo locally and go to the project directory
下载并在本地安装此git repo并转到项目目录
$ git clone https://github.com/nirmash/appsync-redis-api.git
Cloning into 'appsync-redis-api'...
remote: Enumerating objects: 39, done.
remote: Counting objects: 100% (39/39), done.
remote: Compressing objects: 100% (20/20), done.
remote: Total 39 (delta 19), reused 37 (delta 17), pack-reused 0
Unpacking objects: 100% (39/39), done.
$ cd appsync-redis-api/
Build the SAM deployment package
生成SAM部署程序包
$ sam build
Building function 'RedisExecuteCommand'
Running PythonPipBuilder:ResolveDependencies
Running PythonPipBuilder:CopySourceBuild SucceededBuilt Artifacts : .aws-sam/build
Built Template : .aws-sam/build/template.yamlCommands you can use next=========================
[*] Invoke Function: sam local invoke
[*] Deploy: sam deploy --guided
Deploy the application.Note: The SAM template will create a new AppSync API, a Lambda Function and an Amazon ElastiCache cluster.
部署应用程序。 注意: SAM模板将创建一个新的AppSync API,一个Lambda函数和一个Amazon ElastiCache集群。
$ sam deploy --guided
When prompted, select a stack name (the name of the CloudFormation stack that you will use to delete the application later). Your preferred AWS Region and the name for the AppSync API to be created.Note: You will need the AppSync API name later to obtain the API ID.
出现提示时,选择堆栈名称(以后将用于删除应用程序的CloudFormation堆栈的名称)。 您首选的AWS区域和要创建的AppSync API的名称。 注意:您稍后将需要AppSync API名称来获取API ID。
Configuring SAM deploy
====================== Looking for samconfig.toml : Not found
Setting default arguments for 'sam deploy' =========================================
Stack Name [sam-app]: redisAppSyncApi
AWS Region [us-east-1]: us-west-2
Parameter RedisGraphQLApiName [GraphQL-Api]: RedisAppSyncApi
Follow the rest of the prompts. SAM will create a CloudFormation template and deploy the application components, this will take a few minutes.
按照其余提示进行操作。 SAM将创建一个CloudFormation模板并部署应用程序组件,这将需要几分钟。
... Deployment status will show here ...CloudFormation outputs from deployed stack
--------------------------------------------------------------------Outputs
--------------------------------------------------------------------Key RedisExecuteCommand
Description -
Value arn:aws:lambda:us-west-2:..:redisAppSyncApiRedis
Key RedisQueryAPI
Description -
Value arn:aws:appsync:us-west-2:apis/xxxxxxxxxxxxx
--------------------------------------------------------------------
Validating and testingOnce the deployment is completed you will see the below output. We will then obtain the API Key for the App Sync API and execute some Redis commands against it.To obtain the API Key you will need the API Id. You will do that by calling the aws cli.
验证和测试部署完成后,您将看到以下输出。 然后我们将获取App Sync API的API密钥并对其执行一些Redis命令。要获取API密钥,您将需要API ID。 您可以通过调用aws cli来实现。
$ aws appsync list-graphql-apis
Copy the apiId from the command output. To obtain the API Key, call another aws cli command. Also, copy the value of the GRAPHQL uri endpoint for later use.
从命令输出中复制apiId 。 要获取API密钥,请调用另一个aws cli命令。 另外,复制GRAPHQL uri endpoin t的值以备后用。
$ aws appsync list-api-keys --api-id <your api Id>
Copy the id value from the command output. You now have what you need to test your new API. The image below shows the steps in a terminal window.
从命令输出中复制id值。 现在,您具有测试新API所需的功能。 下图显示了终端窗口中的步骤。
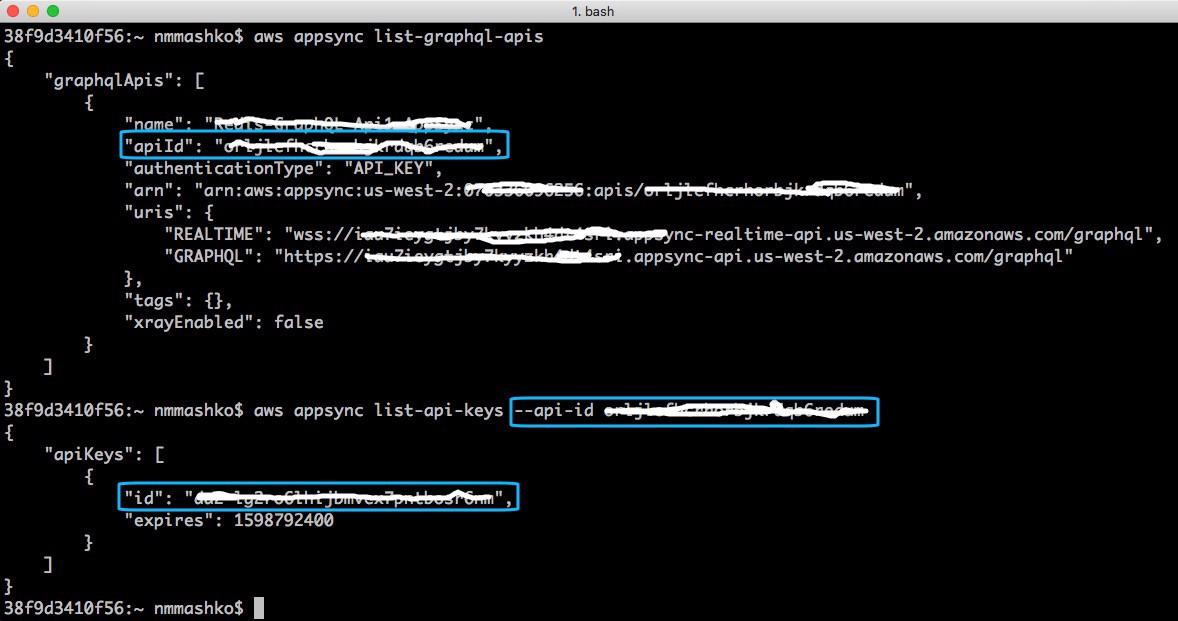
To make testing easier (and because it’s cool…) this repo includes a simple HTML test client that attempts to emulate the redis-cli experience. To get it to work, just double-click the index.html file in the root directory of the repo. Paste the GraphQL uri endpoint and API key you saved earlier into the HTML form and hit the connect button, once connected, `>>` will appear in the cli text area. You can now use some Redis commands to communicate with ElastiCache for Redis!
为了使测试更容易(并且因为它很酷……),此仓库包含一个简单HTML测试客户端,该客户端尝试模仿redis-cli体验。 要使其正常工作,只需双击存储库根目录中的index.html文件。 将您之前保存的GraphQL uri端点和API密钥粘贴到HTML表单中,然后单击连接按钮,一旦连接,“ >>”将出现在cli文本区域。 现在,您可以使用一些Redis命令与ElastiCache for Redis通信!

Cleaning upYou can use the AWS cloud formation CLI to remove the cloud components you deployed with SAM.
清理您可以使用AWS云形成CLI删除通过SAM部署的云组件。
aws cloudformation delete-stack --stack-name <Stack Name you entered earlier>
Note: This call is asynchronous so don’t be alarmed when it completes immediately. You can check the AWS Console CloudFormation screen for the stack deletion status.
注意:此调用是异步的,因此立即完成时不会发出警报。 您可以在AWS Console CloudFormation屏幕上查看堆栈删除状态。
Summary By adding AppSync and Lambda functions to ElastiCache for Redis, we can make it easy and cheap to connect clients to Redis directly and greatly simplify setup and ongoing maintenance.
总结通过将AppSync和Lambda函数添加到ElastiCache for Redis中,我们可以使将客户端直接连接到Redis变得容易且便宜,并大大简化了设置和日常维护。
elasticache