react钩子
React hooks have been around for some time now, yet many React developers are not actively using them. I see two primary reasons behind this. The first reason is that many React developers are already involved in a large project, which requires a significant effort to migrate the entire codebase. The other reason is the familiarity with React Classes. With the experience, it feels more comfortable to keep using Classes.
React挂钩已经存在了一段时间,但是许多React开发人员并未积极使用它们。 我看到这背后的两个主要原因。 第一个原因是许多React开发人员已经参与了一个大型项目,这需要付出巨大的努力才能迁移整个代码库。 另一个原因是对React类的熟悉。 有了这些经验,继续使用Classes会感到更自在。
In this article, we’ll look into five reasons why you should consider React Hooks.
在本文中,我们将研究为什么应该考虑使用React Hooks的五个原因。
1.当功能组件增长时,不必将其重构为类组件 (1. You don’t have to refactor a functional component into a class component when it grows)
Usually, there are times when a React component starts with a functional component, which only depends on the props and later evolves into a Class component with having a state. Changing from a functional component to a class component requires a little bit of refactoring, depending on how complex the component is.
通常,有时React组件从功能组件开始,而功能组件仅取决于道具,后来演变为具有状态的Class组件。 从功能组件更改为类组件需要一些重构,具体取决于组件的复杂程度。
With React Hooks, since functional components have the capability of tapping into the state, the refactoring effort will be minimal. Let’s consider the below example, a dumb component that shows a label with a count.
使用React Hooks,由于功能组件具有进入状态的能力,因此重构工作将最少。 让我们考虑以下示例,这是一个哑巴组件,它显示带有计数的标签。
Let’s say we need to increment the count with mouse clicks and let’s assume this only affects this particular component. As the first step, we need to introduce the state to our component. Let’s take a look at how we would do that with a class-based approach.
假设我们需要通过单击鼠标来增加计数,并假设这仅影响此特定组件。 第一步,我们需要将状态引入组件。 让我们看一下如何使用基于类的方法来做到这一点。
The same component will look like the following if we use Hooks.
如果使用挂钩,则相同的组件将如下所示。
2.您不必再担心“这个”了(2. You don’t have to worry about “this” anymore)
Classes confuse both people and machines
类会使人和机器混淆
The above sentence is from React documentation. One of the reasons for this confusion is this
keyword. If you are familiar with JavaScript, you know that this
in JavaScript doesn’t work exactly like in other languages. When it comes to React Hooks, you don’t have to worry about this
at all. This is good for beginners as well as experienced developers.
上面的句子来自React文档。 造成这种混乱的原因之一是this
关键字。 如果你熟悉JavaScript,你知道, this
在JavaScript中不工作完全像在其他语言。 当谈到React鱼钩,你不必担心this
的。 这对初学者和经验丰富的开发人员都是有益的。
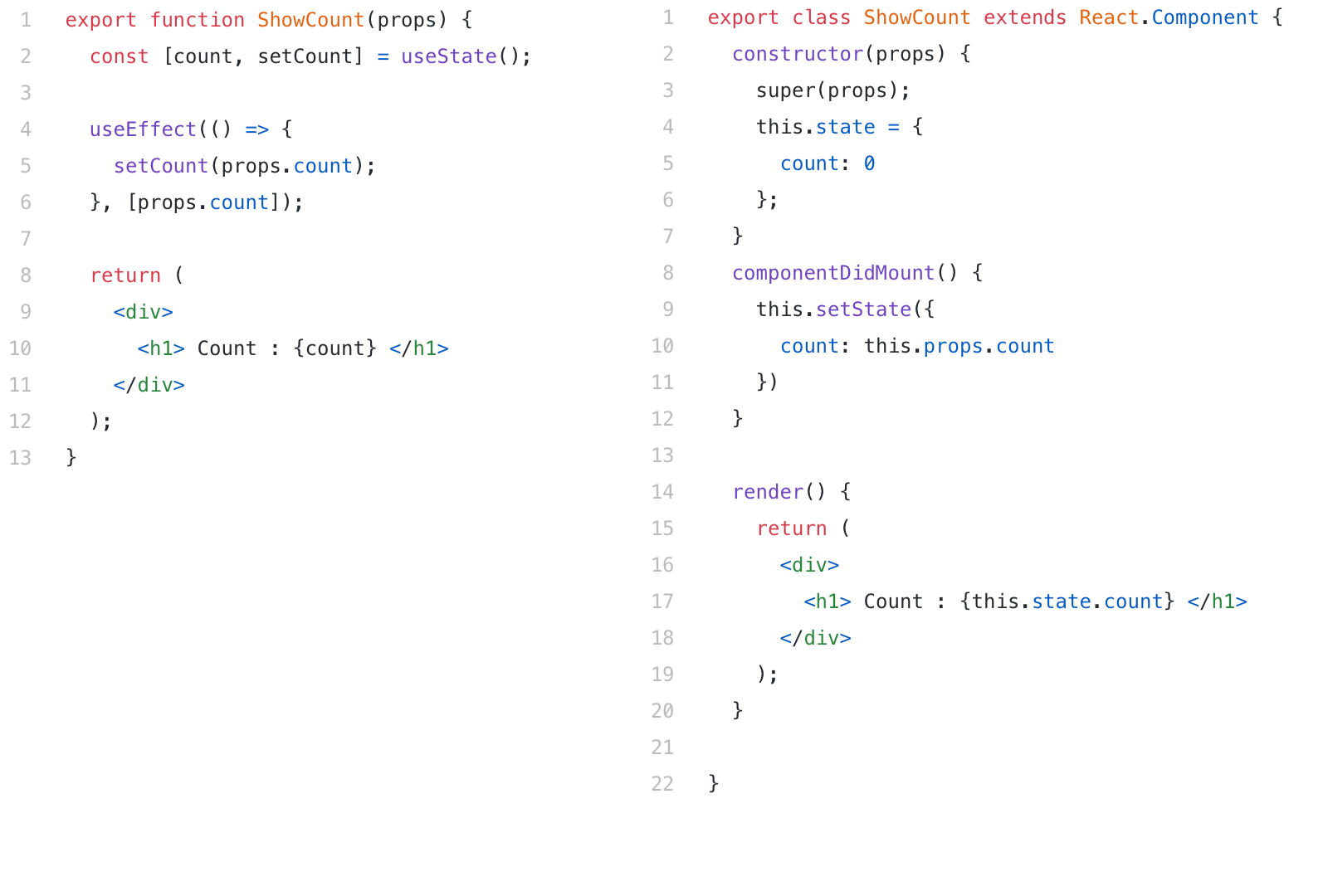
According to the above example, you can see that we no longer have to use “this
” while accessing the state. This makes it less confusing for everyone.
根据上面的示例,您可以看到访问状态时我们不再需要使用“ this
”。 这使得每个人都不会感到困惑。
3.不再有方法绑定 (3. No more method bindings)
Now for the above same ShowCount component let’s introduce a method to update the count of the state when the user clicks on the label.
现在,对于上述相同的ShowCount组件,让我们介绍一种在用户单击标签时更新状态计数的方法。
We have introduced handleClickEvent
method. To use it, first, we have to bind it to this
of the Component.
我们引入了handleClickEvent
方法。 要使用它,首先,我们必须将其绑定到this
组件。
this.handleClickEvent = this.handleClickEvent.bind(this);
We have to do this because the execution context is different when the method gets executed. For a beginner developer, this might be a bit hard to understand.
我们必须这样做,因为执行方法时执行上下文是不同的。 对于初学者来说,这可能有点难以理解。
Instead of binding all the methods, there are some syntax proposals where you can get around this. For example, we can rewrite the function to an arrow function.
除了绑定所有方法之外,还有一些语法建议可以解决此问题。 例如,我们可以将函数重写为箭头函数。
handleClickEvent = () => {
this.setState({count: this.state.count + 1});
}
Let’s see how we can implement the same functionality with Hooks.
让我们看看如何使用Hooks实现相同的功能。
As you can see, we have only added the function. Also, you might notice that when we use the event handler, we have removed this
in the template.
如您所见,我们仅添加了该功能。 此外,您可能会注意到,当我们使用事件处理程序,我们已经移除this
模板。
onClick={ handleClickEvent }
4.更容易将逻辑与UI分离,从而使两者都更可重用 (4. Easier to decouple logic from UI, making both more reusable)
Using hooks, logic and UI are easier to separate. No need for HOC or render props. Hooks do it elegantly with less boilerplate and more intuitive compositions of UI and logic.
使用钩子,逻辑和UI更容易分离。 无需HOC或渲染道具。 钩子用更少的样板和更直观的UI和逻辑组成来优雅地完成它。
This “elegant separation” is especially crucial when sharing components using tools and platforms like Bit (Github) as each (independently shared) component is much easier to understand, maintain, and reuse across different apps.
当使用诸如Bit ( Github )之类的工具和平台共享组件时,这种“优雅的分离”尤其重要,因为每个(独立共享的)组件在不同应用程序之间都更易于理解,维护和重用。
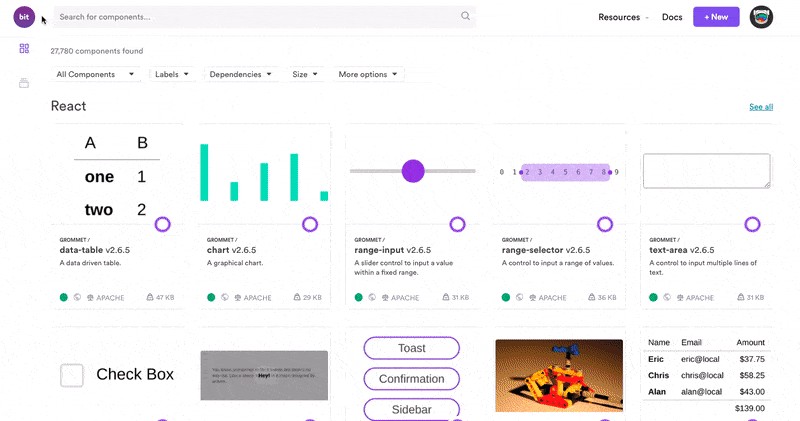
5.将相关逻辑放在同一位置(5. Keep related logic in the same place)
Complex components become hard to understand
复杂的组件变得难以理解
With the class-based approach, we have different life cycle methods such as componentDidMount
and componentDidUpdate
etc. Let's consider a situation where subscribing to services A and B in componentDidMount
and unsubscribing them on componentWillUnmount
. With time, there will be many logics included in both life cycle methods, and it will be hard to keep track of which part of mounting is related in unmounting.
随着基于类的方法,我们有不同的生命周期方法,如componentDidMount
和componentDidUpdate
等让我们考虑的情况下在订阅服务A和B componentDidMount
和取消他们componentWillUnmount
。 随着时间的流逝,两种生命周期方法中都会包含许多逻辑,并且很难跟踪卸载过程中与安装相关的部分。
To demonstrate this, let's create an RxJs based service to get the count. We will use this service to update the count in ShowCount example. Note that we will be removing the handleClickEvent
as we no longer need to update the component on click events.
为了演示这一点,让我们创建一个基于RxJs的服务来获取计数。 我们将使用此服务来更新ShowCount示例中的计数。 请注意,由于不再需要在点击事件中更新组件,因此我们将删除handleClickEvent
。
You can see that inside the useEffect
we have included subscribing as well as corresponding unsubscribing logic. Similarly, if we need to introduce more service subscriptions or unrelated logics, we can add multiple useEffect
blocks to logically separate different concerns.
您可以看到在useEffect
内部,我们包括了订阅以及相应的取消订阅逻辑。 同样,如果我们需要引入更多的服务订阅或不相关的逻辑,则可以添加多个useEffect
块在逻辑上分离不同的关注点。
6.在组件之间共享状态逻辑(6. Sharing stateful logic between components)
With the class-based approach, it is hard to share stateful logic between components. Consider two components where both have to fetch, sort, and display data from two different data sources. Even though both components have the same functionality, it is hard to share the logic because these components have different sources and states.
使用基于类的方法,很难在组件之间共享状态逻辑。 考虑两个组件,这两个组件都必须从两个不同的数据源获取,排序和显示数据。 即使两个组件具有相同的功能,也很难共享逻辑,因为这些组件具有不同的源和状态。
While we can use render props and higher-order components to solve this, it will also introduce additional cost as we have to restructure our components, which will eventually make it harder to follow.
虽然我们可以使用渲染道具和高阶组件来解决此问题,但由于我们必须重新构造组件,这还会引入额外的成本,最终将使后续操作变得更加困难。
React Hooks提供什么? (What React Hooks offers?)
With Custom React Hooks you can extract these reusable stateful logics and test them separately.
使用Custom React Hooks,您可以提取这些可重用的状态逻辑并分别进行测试。
We can extract a custom hook from the ShowCount example.
我们可以从ShowCount示例中提取自定义钩子。
Using the above custom hook, we can rewrite the ShowCount component as follows. Notice that we have to pass the data source to the custom hook as a parameter.
使用上面的自定义钩子,我们可以如下重写ShowCount组件。 注意,我们必须将数据源作为参数传递给自定义钩子。
Note that we invoke
getCounts
in a parent component rather than inShowCount
component. OtherwiseserviceSubject
will have a new value each time it runsShowCount
and we wouldn’t get the result we expect.请注意,我们在父组件而不是
ShowCount
组件中调用getCounts
。 否则,serviceSubject
每次运行ShowCount
时都将具有一个新值,而我们将无法获得预期的结果。
结论 (Conclusion)
While there are many reasons to switch to React Hooks, I have mentioned the most compelling reasons which made me change to React Hooks. If you look at the official documentation, you will see that there are many interesting functionalities with React Hooks. Please let me know in the comments about your journey to React Hooks.
切换到React Hooks的原因有很多,但我已经提到了最令人信服的原因,这些使我改变为React Hooks。 如果看官方文档,您会发现React Hooks有很多有趣的功能。 请在您对React Hooks之旅的评论中让我知道。
You can find the completed source code here.
您可以在此处找到完整的源代码。
学到更多 (Learn More)
翻译自: https://blog.bitsrc.io/6-reasons-to-use-react-hooks-instead-of-classes-7e3ee745fe04
react钩子