javascript递归
This blog will give an intro explanation of recursion in javascript.
该博客将介绍javascript中的递归解释。
Recursion is the act of calling a function on itself. This allows you to recurse, or iterate over the parameter passed in while holding values in memory for each iteration. Once the function has finished calling, each stacked value will be reduced into one. There are other ways to iterate over data, however recursion can be useful when there is a repeatable pattern that can be executed.
递归是对自身调用函数的行为。 这使您可以递归或迭代传入的参数,同时将每次迭代的值保存在内存中。 函数完成调用后,每个堆叠的值将减少为一个。 还有其他方法可以遍历数据,但是当存在可以执行的可重复模式时,递归可能很有用。
Recursion can seem dangerous because of the possibility of your function to infinitely call itself, but keeping these three points below in mind, hopefully you can avoid this.
由于函数可以无限调用自身,因此递归似乎很危险,但是请记住以下三点,希望您可以避免这种情况。
递归需要3个简单的部分: (Recursion needs 3 simple parts:)
- Call itself within 在内部称呼自己
- Recursive case 递归案例
- Base case / Return statement 基本案例/退货声明
概念: (Concept:)
The idea behind recursion is to evaluate a return statement by calling the function on itself. Each function call will allow you to go deeper into your parameter. In the function below we’re going to be adding elements in an array of whole numbers to return a number which is the sum of all the elements in the array.
递归的思想是通过调用函数本身来评估return语句。 每个函数调用都可以让您更深入地了解参数。 在下面的函数中,我们将在整数数组中添加元素,以返回一个数字,该数字是数组中所有元素的总和。
// Recursive function
function recursionSum(collection){
// Base case
if(collection.length === 0){
return 0;
}
// Recursive case
const currentNumber = collection[0];
const shortenArray = collection.slice(1);
// Recursive call
return currentNumber + recursionSum(shortenArray);
}
recursionSum([1, 2, 3]); // returns 6
确定目的地并制定计划 (Deciding the Destination and Making a Plan)
在递归中遍历参数时,您一次只关注一层。 (When iterating over a parameter in recursion you focus on one layer at a time.)
When this function is called, the argument will be an array of numbers, therefore, we will focus on one number at a time while iterating. The output of the function will be the sum of all numbers in the given array. Keeping your expected output in mind will help you understand how to plan.
调用此函数时,参数将是一个数字数组,因此,我们将在迭代时一次关注一个数字。 函数的输出将是给定数组中所有数字的总和。 牢记预期的输出结果将帮助您了解如何进行计划。
递归案例和函数调用-“ The Trail” (Recursive Case and Function Call — “The Trail”)
递归情况由函数将在每次迭代中执行的动作组成。 最后一步是返回函数调用。 (A recursive case is comprised of the action the function will take on each iteration. The last step will be to return the function call.)

Each time the recursive function is called, the return is stored in memory leaving a trail of values. The example function returns a trail of numbers and uses the addition operator to add together value. In order for the function to stop calling itself and in this example add the values together, a base case is needed.
每次调用递归函数时,返回值都会存储在内存中,并留下一连串的值。 示例函数返回数字尾迹,并使用加法运算符将值相加。 为了使函数停止自行调用,并在此示例中将这些值加在一起,需要一个基本情况。
基本案例-“回报” (Base Case — “The Return”)
一个基本案例包括一个条件,该条件告诉您的函数何时停止递归并返回其值到链上 (A base case is comprised of a condition that tells your function when to stop recursion and return its value back up the chain)
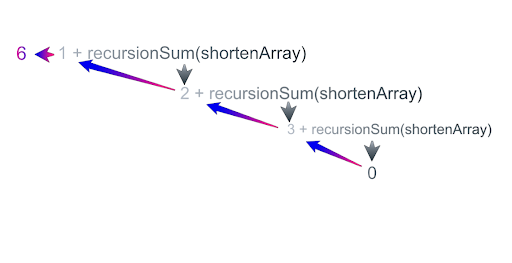
Once the function stops calling itself, the recursive stacks will collapse, returning each value up the chain. In the case of the above function, the function will stop once it reaches the last element of the array. The condition will be set to return once the length of the array is zero. The value will be set to return zero to preserve the true sum once all values are added together.
一旦函数停止调用自身,递归堆栈将崩溃,并沿链返回每个值。 对于上述函数,一旦到达数组的最后一个元素,该函数将停止。 一旦数组的长度为零,该条件将被设置为返回。 一旦将所有值加在一起,该值将设置为返回零以保留真实的总和。
Depending on your conditional, you’ll need to adjust your function so that the base case can be reached. In this case, the condition that needs to be met is recursing to the last element in the array. The approach taken in the function is to use the slice method to preserve the first element in memory. Specifically this also changes the first element in the array going further into the recursive call. The new array is passed into the recursive call, with a decreasing length at each call until the final conditional is met.
根据您的条件,您需要调整功能,以便可以达到基本情况。 在这种情况下,需要满足的条件将递归到数组中的最后一个元素。 该函数采用的方法是使用切片方法将第一个元素保留在内存中。 具体来说,这还将更改数组中的第一个元素,使其进一步进入递归调用。 新数组传递到递归调用中,每次调用的长度减小,直到满足最终条件为止。
And that’s all there is to it! I hope this clarified a bit on how recursion isn’t so bad and in some cases a very unique way tackle problems.
这就是全部! 我希望这可以弄清楚递归不是那么糟糕,并且在某些情况下以一种非常独特的方式解决问题。
翻译自: https://medium.com/@bri.skinner02/explaining-recursion-in-javascript-9168e4aba8f2
javascript递归