A couple of weeks ago, I was just about ready to release Caer, a Computer Vision library in Python to be publically available on PyPi, when I decided to send it to a friend in Alberta to tink around with it.
几周前,当我决定将它发送给艾伯塔省的一位朋友进行修改时,我正准备发布Caer (Python中的计算机视觉库)在PyPi上公开可用。
A few days later, I find that he’s still figuring out how to get it to work on his machine.
几天后,我发现他仍在弄清楚如何使其在他的机器上运行。
After dozens of hours, I finally found out why — Caer implemented code from the previous versions of other Python packages that simply weren’t available in their newer releases.
几十个小时后,我终于找到了原因-Caer实现了其他Python软件包以前版本中的代码,而这些代码在新版本中根本不可用。
Despite having those packages installed, my friend wasn’t able to run Caer for the reasons mentioned above.
尽管已安装了这些软件包,但由于上述原因,我的朋友仍无法运行Caer。
Issues like this aren’t solely specific to Python packages. You could face something similar when moving locally-built code into production and not have it work because of different operating systems.
诸如此类的问题并不仅仅针对Python软件包。 当将本地构建的代码移入生产环境时,由于操作系统不同,您可能会遇到类似的情况。
But, what if there was a way to mitigate this issue of portability?
但是,如果有减轻这种可移植性问题的方法怎么办?
Yes, Docker!
是的,Docker!
Before we talk about Docker, you need to understand the intuition behind a container.
在讨论Docker之前,您需要了解容器背后的直觉。
货柜 (Containers)
A container is an entire runtime environment: an application with all its dependencies, libraries, binaries, and configuration files needed to run it, bundled up into one package.
容器是整个运行时环境:一个应用程序及其运行所需的所有依赖项,库,二进制文件和配置文件,捆绑在一个程序包中。
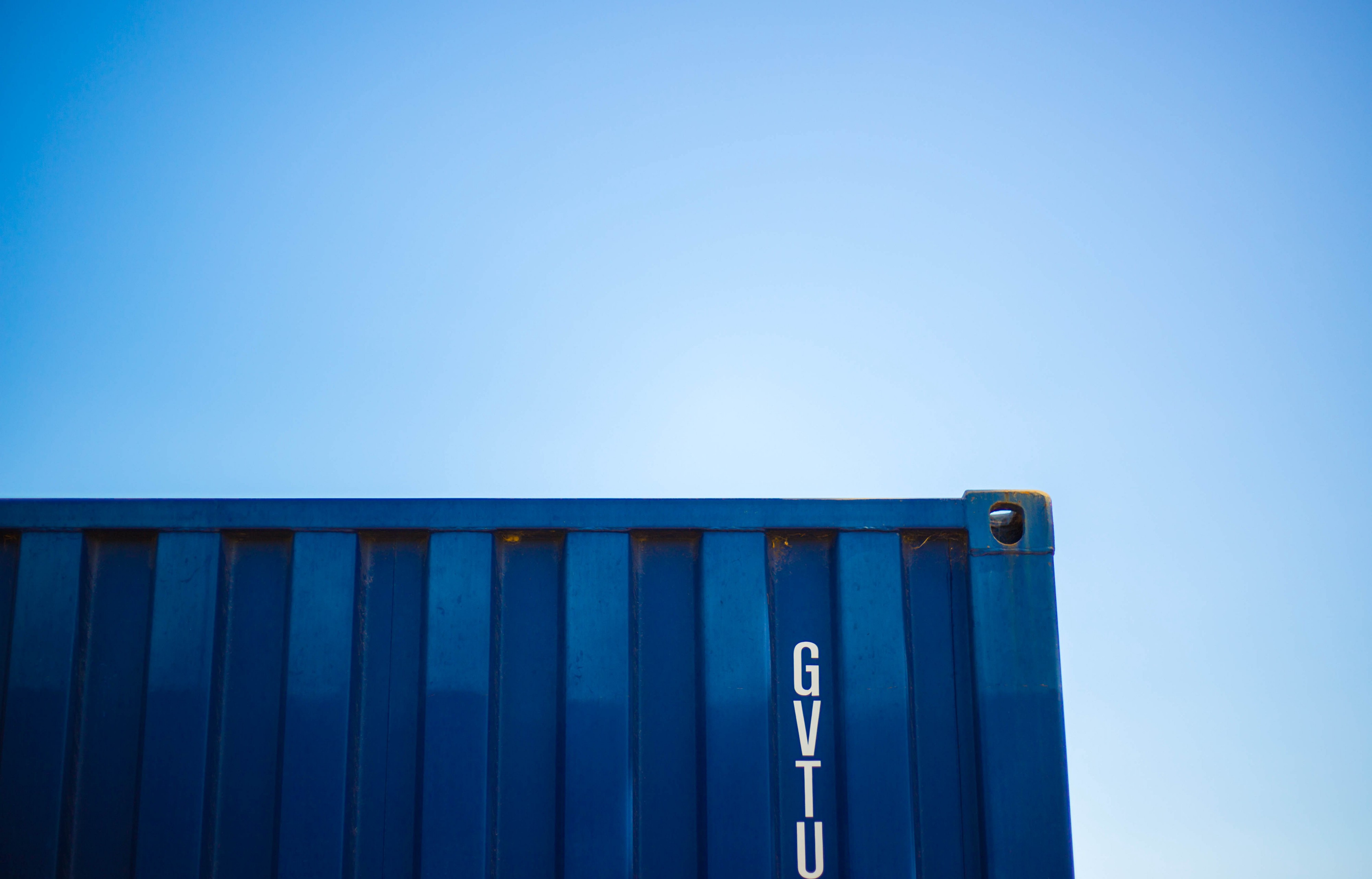
Containerization abstracts away differences in OS distributions, application dependencies and underlying infrastructure.
容器化消除了OS分布,应用程序依赖项和基础架构中的差异。
容器就像VM,但体积更小 (Containers are like VMs, but way smaller)
With virtualization, these containers are called Virtual Machines. These include the operating system in addition to the application. A server running three virtual machines may have three different operating systems running on top of it.
通过虚拟化,这些容器称为虚拟机。 除应用程序外,这些还包括操作系统。 运行三个虚拟机的服务器可能在其顶部运行三个不同的操作系统。
Imagine how bulky this can get.
想象一下,这可能会带来多大的麻烦。
In contrast, you can have the same server run 3 containerized applications with Docker that all run the same operating system. The parts of the operating system are shared are read-only, while a container has a mount (a way to access the container) for writing.
相反,您可以让同一台服务器运行3个带有Docker的容器化应用程序,而这些应用程序都运行相同的操作系统。 操作系统的各个部分是只读的,而容器具有用于写入的安装座(一种访问容器的方式)。
While VMs may be several gigabytes in size, a container may be just a few megabytes in size.
尽管VM的大小可能为数GB,但容器的大小可能仅为几MB。
容器的魔力 (The magic of containers)
When applications are containerized, only the operating system is virtualized, as opposed to hardware (in the case of VMs). Instead of provisioning hardware, a virtual OS is provisioned to the application, enabling you to run multiple applications and set resource-limitations as you please.
将应用程序容器化后,与硬件(对于VM)相比,仅对操作系统进行虚拟化。 无需配置硬件,而是为应用程序配置了虚拟OS,使您可以运行多个应用程序并根据需要设置资源限制。
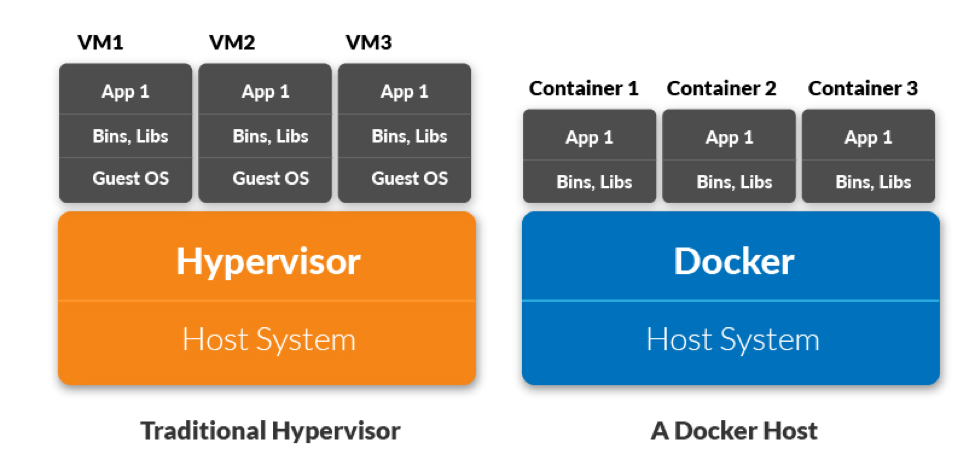
啊,Docker! (Ah, Docker!)
Docker is a containerization tool used that developers use to spin up isolated, reproducible environments for their applications.
Docker是一种容器化工具,开发人员可使用它为应用程序启动隔离的,可复制的环境。
It has a fast development process, it is easy to use, works the same on local machines, dev, staging and production servers, and is extremely scalable.
它具有快速的开发过程,易于使用,可在本地计算机,开发人员,暂存和生产服务器上相同地工作,并且具有极高的可伸缩性。
Docker, in fact, drove the shift to the containerization of applications, and is, to no surprise, the most powerful player in the market today.
实际上,Docker推动了向应用程序容器化的转变,毫无疑问,Docker是当今市场上最强大的参与者。
安装 (Installation)
Windows or MacOS: Install Docker Desktop
Windows或MacOS:安装Docker Desktop
Linux: Install Docker and Docker Compose
Linux:安装Docker和Docker Compose
If you are on Linux, you’ll need to run your commands as root or add the user to the Docker group:
如果您使用的是Linux,则需要以root用户身份运行命令或将用户添加到Docker组:
sudo usermod -aG docker $(thatsme)
创建一个Dockerfile (Creating a Dockerfile)
In Python, to containerize an application, you will need to pack it as a Docker Image. To generate one, you need to define a Dockerfile. This name of the file is simply DOCKERFILE (no extension)
在Python中,要将应用程序容器化,您需要将其打包为Docker Image。 要生成一个文件,您需要定义一个Dockerfile。 该文件的名称就是DOCKERFILE (无扩展名)
# Defining a base image (Python 3 in our case)
FROM python:3# Adding a Python script to be run
ADD hello_world_script.py /# If our script uses the Caer package, we'll have to pip install it:
RUN pip install caer# To execute the Python script:
CMD [ "python", "./]
FROM
instructs Docker what image the application is based on (a mouthful, I know)FROM
指示Docker应用程序基于什么映像(我知道这是一个完整的消息)RUN
executes any additional commands (such as a pip install)RUN
执行任何其他命令(例如pip安装)CMD
executes the commands when the image is loaded加载图像时,
CMD
执行命令
For this demonstration, I have used the caer
package that you can install with a simple pip install
在此演示中,我使用了caer
软件包,可以通过简单的pip install
Suppose, our hello_world_script.py
script looks like this:
假设我们的hello_world_script.py
脚本如下所示:
# Taken from the Caer Github repo
# https://github.com/jasmcaus/caerimport caerimg = caer.readImg('./img1.png')
# Caer version
print(caer.__version__)# Image shape
print(img.shape)# Image Array:
print(img)
To build the image from the Dockerfile, go ahead and run the following:
要从Dockerfile构建映像,请继续并运行以下命令:
docker build -t caer-readimg .
Once the image has been built, you’ll be able to run it as a container.
生成映像后,您就可以将其作为容器运行。
You’ll also notice a ‘caer-readimg’ image (you can view all your Docker image by running the docker images
command).
您还会注意到一个“ caer-readimg”映像(您可以通过运行docker images
命令来查看所有Docker映像)。
To run this image,
要运行此图像,
docker run caer-readimg
删除Docker映像 (Deleting a Docker Image)
# The image_id can be found when you run `docker images`docker rmi <image_id>
删除Docker容器 (Deleting a Docker Container)
# Retrieve the container ID:
docker ps -a # Deleting the container
docker rm <container_id>
That’s how easy it is to get started with Docker. Of course, you probably wouldn’t need to build a Docker image if your application is as simple as the code discussed above, but it makes sense especially if you’re working on the same project with multiple people.
这就是开始使用Docker的难易程度。 当然,如果您的应用程序与上面讨论的代码一样简单,则可能不需要构建Docker映像,但是这很有意义,尤其是当您与多个人一起在同一个项目中工作时。
Feel free to include your suggestions in the comments below.
欢迎在下面的评论中加入您的建议。
Until then, happy learning!
在那之前,学习愉快!
翻译自: https://towardsdatascience.com/you-should-start-using-docker-now-3c1a6b76a147